mbed TLS library
Dependents: HTTPClient-SSL WS_SERVER
ctr_drbg.h
00001 /** 00002 * \file ctr_drbg.h 00003 * 00004 * \brief CTR_DRBG based on AES-256 (NIST SP 800-90) 00005 * 00006 * Copyright (C) 2006-2014, ARM Limited, All Rights Reserved 00007 * 00008 * This file is part of mbed TLS (https://tls.mbed.org) 00009 * 00010 * This program is free software; you can redistribute it and/or modify 00011 * it under the terms of the GNU General Public License as published by 00012 * the Free Software Foundation; either version 2 of the License, or 00013 * (at your option) any later version. 00014 * 00015 * This program is distributed in the hope that it will be useful, 00016 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00017 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00018 * GNU General Public License for more details. 00019 * 00020 * You should have received a copy of the GNU General Public License along 00021 * with this program; if not, write to the Free Software Foundation, Inc., 00022 * 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301 USA. 00023 */ 00024 #ifndef POLARSSL_CTR_DRBG_H 00025 #define POLARSSL_CTR_DRBG_H 00026 00027 #include "aes.h" 00028 00029 #define POLARSSL_ERR_CTR_DRBG_ENTROPY_SOURCE_FAILED -0x0034 /**< The entropy source failed. */ 00030 #define POLARSSL_ERR_CTR_DRBG_REQUEST_TOO_BIG -0x0036 /**< Too many random requested in single call. */ 00031 #define POLARSSL_ERR_CTR_DRBG_INPUT_TOO_BIG -0x0038 /**< Input too large (Entropy + additional). */ 00032 #define POLARSSL_ERR_CTR_DRBG_FILE_IO_ERROR -0x003A /**< Read/write error in file. */ 00033 00034 #define CTR_DRBG_BLOCKSIZE 16 /**< Block size used by the cipher */ 00035 #define CTR_DRBG_KEYSIZE 32 /**< Key size used by the cipher */ 00036 #define CTR_DRBG_KEYBITS ( CTR_DRBG_KEYSIZE * 8 ) 00037 #define CTR_DRBG_SEEDLEN ( CTR_DRBG_KEYSIZE + CTR_DRBG_BLOCKSIZE ) 00038 /**< The seed length (counter + AES key) */ 00039 00040 /** 00041 * \name SECTION: Module settings 00042 * 00043 * The configuration options you can set for this module are in this section. 00044 * Either change them in config.h or define them on the compiler command line. 00045 * \{ 00046 */ 00047 00048 #if !defined(CTR_DRBG_ENTROPY_LEN) 00049 #if defined(POLARSSL_SHA512_C) && !defined(POLARSSL_ENTROPY_FORCE_SHA256) 00050 #define CTR_DRBG_ENTROPY_LEN 48 /**< Amount of entropy used per seed by default (48 with SHA-512, 32 with SHA-256) */ 00051 #else 00052 #define CTR_DRBG_ENTROPY_LEN 32 /**< Amount of entropy used per seed by default (48 with SHA-512, 32 with SHA-256) */ 00053 #endif 00054 #endif 00055 00056 #if !defined(CTR_DRBG_RESEED_INTERVAL) 00057 #define CTR_DRBG_RESEED_INTERVAL 10000 /**< Interval before reseed is performed by default */ 00058 #endif 00059 00060 #if !defined(CTR_DRBG_MAX_INPUT) 00061 #define CTR_DRBG_MAX_INPUT 256 /**< Maximum number of additional input bytes */ 00062 #endif 00063 00064 #if !defined(CTR_DRBG_MAX_REQUEST) 00065 #define CTR_DRBG_MAX_REQUEST 1024 /**< Maximum number of requested bytes per call */ 00066 #endif 00067 00068 #if !defined(CTR_DRBG_MAX_SEED_INPUT) 00069 #define CTR_DRBG_MAX_SEED_INPUT 384 /**< Maximum size of (re)seed buffer */ 00070 #endif 00071 00072 /* \} name SECTION: Module settings */ 00073 00074 #define CTR_DRBG_PR_OFF 0 /**< No prediction resistance */ 00075 #define CTR_DRBG_PR_ON 1 /**< Prediction resistance enabled */ 00076 00077 #ifdef __cplusplus 00078 extern "C" { 00079 #endif 00080 00081 /** 00082 * \brief CTR_DRBG context structure 00083 */ 00084 typedef struct 00085 { 00086 unsigned char counter[16]; /*!< counter (V) */ 00087 int reseed_counter; /*!< reseed counter */ 00088 int prediction_resistance; /*!< enable prediction resistance (Automatic 00089 reseed before every random generation) */ 00090 size_t entropy_len; /*!< amount of entropy grabbed on each 00091 (re)seed */ 00092 int reseed_interval; /*!< reseed interval */ 00093 00094 aes_context aes_ctx; /*!< AES context */ 00095 00096 /* 00097 * Callbacks (Entropy) 00098 */ 00099 int (*f_entropy)(void *, unsigned char *, size_t); 00100 00101 void *p_entropy; /*!< context for the entropy function */ 00102 } 00103 ctr_drbg_context; 00104 00105 /** 00106 * \brief CTR_DRBG initialization 00107 * 00108 * Note: Personalization data can be provided in addition to the more generic 00109 * entropy source to make this instantiation as unique as possible. 00110 * 00111 * \param ctx CTR_DRBG context to be initialized 00112 * \param f_entropy Entropy callback (p_entropy, buffer to fill, buffer 00113 * length) 00114 * \param p_entropy Entropy context 00115 * \param custom Personalization data (Device specific identifiers) 00116 * (Can be NULL) 00117 * \param len Length of personalization data 00118 * 00119 * \return 0 if successful, or 00120 * POLARSSL_ERR_CTR_DRBG_ENTROPY_SOURCE_FAILED 00121 */ 00122 int ctr_drbg_init( ctr_drbg_context *ctx, 00123 int (*f_entropy)(void *, unsigned char *, size_t), 00124 void *p_entropy, 00125 const unsigned char *custom, 00126 size_t len ); 00127 00128 /** 00129 * \brief Clear CTR_CRBG context data 00130 * 00131 * \param ctx CTR_DRBG context to clear 00132 */ 00133 void ctr_drbg_free( ctr_drbg_context *ctx ); 00134 00135 /** 00136 * \brief Enable / disable prediction resistance (Default: Off) 00137 * 00138 * Note: If enabled, entropy is used for ctx->entropy_len before each call! 00139 * Only use this if you have ample supply of good entropy! 00140 * 00141 * \param ctx CTR_DRBG context 00142 * \param resistance CTR_DRBG_PR_ON or CTR_DRBG_PR_OFF 00143 */ 00144 void ctr_drbg_set_prediction_resistance( ctr_drbg_context *ctx, 00145 int resistance ); 00146 00147 /** 00148 * \brief Set the amount of entropy grabbed on each (re)seed 00149 * (Default: CTR_DRBG_ENTROPY_LEN) 00150 * 00151 * \param ctx CTR_DRBG context 00152 * \param len Amount of entropy to grab 00153 */ 00154 void ctr_drbg_set_entropy_len( ctr_drbg_context *ctx, 00155 size_t len ); 00156 00157 /** 00158 * \brief Set the reseed interval 00159 * (Default: CTR_DRBG_RESEED_INTERVAL) 00160 * 00161 * \param ctx CTR_DRBG context 00162 * \param interval Reseed interval 00163 */ 00164 void ctr_drbg_set_reseed_interval( ctr_drbg_context *ctx, 00165 int interval ); 00166 00167 /** 00168 * \brief CTR_DRBG reseeding (extracts data from entropy source) 00169 * 00170 * \param ctx CTR_DRBG context 00171 * \param additional Additional data to add to state (Can be NULL) 00172 * \param len Length of additional data 00173 * 00174 * \return 0 if successful, or 00175 * POLARSSL_ERR_CTR_DRBG_ENTROPY_SOURCE_FAILED 00176 */ 00177 int ctr_drbg_reseed( ctr_drbg_context *ctx, 00178 const unsigned char *additional, size_t len ); 00179 00180 /** 00181 * \brief CTR_DRBG update state 00182 * 00183 * \param ctx CTR_DRBG context 00184 * \param additional Additional data to update state with 00185 * \param add_len Length of additional data 00186 * 00187 * \note If add_len is greater than CTR_DRBG_MAX_SEED_INPUT, 00188 * only the first CTR_DRBG_MAX_SEED_INPUT bytes are used, 00189 * the remaining ones are silently discarded. 00190 */ 00191 void ctr_drbg_update( ctr_drbg_context *ctx, 00192 const unsigned char *additional, size_t add_len ); 00193 00194 /** 00195 * \brief CTR_DRBG generate random with additional update input 00196 * 00197 * Note: Automatically reseeds if reseed_counter is reached. 00198 * 00199 * \param p_rng CTR_DRBG context 00200 * \param output Buffer to fill 00201 * \param output_len Length of the buffer 00202 * \param additional Additional data to update with (Can be NULL) 00203 * \param add_len Length of additional data 00204 * 00205 * \return 0 if successful, or 00206 * POLARSSL_ERR_CTR_DRBG_ENTROPY_SOURCE_FAILED, or 00207 * POLARSSL_ERR_CTR_DRBG_REQUEST_TOO_BIG 00208 */ 00209 int ctr_drbg_random_with_add( void *p_rng, 00210 unsigned char *output, size_t output_len, 00211 const unsigned char *additional, size_t add_len ); 00212 00213 /** 00214 * \brief CTR_DRBG generate random 00215 * 00216 * Note: Automatically reseeds if reseed_counter is reached. 00217 * 00218 * \param p_rng CTR_DRBG context 00219 * \param output Buffer to fill 00220 * \param output_len Length of the buffer 00221 * 00222 * \return 0 if successful, or 00223 * POLARSSL_ERR_CTR_DRBG_ENTROPY_SOURCE_FAILED, or 00224 * POLARSSL_ERR_CTR_DRBG_REQUEST_TOO_BIG 00225 */ 00226 int ctr_drbg_random( void *p_rng, 00227 unsigned char *output, size_t output_len ); 00228 00229 #if defined(POLARSSL_FS_IO) 00230 /** 00231 * \brief Write a seed file 00232 * 00233 * \param ctx CTR_DRBG context 00234 * \param path Name of the file 00235 * 00236 * \return 0 if successful, 00237 * POLARSSL_ERR_CTR_DRBG_FILE_IO_ERROR on file error, or 00238 * POLARSSL_ERR_CTR_DRBG_ENTROPY_SOURCE_FAILED 00239 */ 00240 int ctr_drbg_write_seed_file( ctr_drbg_context *ctx, const char *path ); 00241 00242 /** 00243 * \brief Read and update a seed file. Seed is added to this 00244 * instance 00245 * 00246 * \param ctx CTR_DRBG context 00247 * \param path Name of the file 00248 * 00249 * \return 0 if successful, 00250 * POLARSSL_ERR_CTR_DRBG_FILE_IO_ERROR on file error, 00251 * POLARSSL_ERR_CTR_DRBG_ENTROPY_SOURCE_FAILED or 00252 * POLARSSL_ERR_CTR_DRBG_INPUT_TOO_BIG 00253 */ 00254 int ctr_drbg_update_seed_file( ctr_drbg_context *ctx, const char *path ); 00255 #endif /* POLARSSL_FS_IO */ 00256 00257 /** 00258 * \brief Checkup routine 00259 * 00260 * \return 0 if successful, or 1 if the test failed 00261 */ 00262 int ctr_drbg_self_test( int verbose ); 00263 00264 /* Internal functions (do not call directly) */ 00265 int ctr_drbg_init_entropy_len( ctr_drbg_context *, 00266 int (*)(void *, unsigned char *, size_t), void *, 00267 const unsigned char *, size_t, size_t ); 00268 00269 #ifdef __cplusplus 00270 } 00271 #endif 00272 00273 #endif /* ctr_drbg.h */ 00274
Generated on Tue Jul 12 2022 13:50:37 by
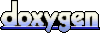