mbed TLS library
Dependents: HTTPClient-SSL WS_SERVER
cipher.c File Reference
Generic cipher wrapper for mbed TLS. More...
Go to the source code of this file.
Functions | |
const int * | cipher_list (void) |
Returns the list of ciphers supported by the generic cipher module. | |
const cipher_info_t * | cipher_info_from_type (const cipher_type_t cipher_type) |
Returns the cipher information structure associated with the given cipher type. | |
const cipher_info_t * | cipher_info_from_string (const char *cipher_name) |
Returns the cipher information structure associated with the given cipher name. | |
const cipher_info_t * | cipher_info_from_values (const cipher_id_t cipher_id, int key_length, const cipher_mode_t mode) |
Returns the cipher information structure associated with the given cipher id, key size and mode. | |
void | cipher_init (cipher_context_t *ctx) |
Initialize a cipher_context (as NONE) | |
void | cipher_free (cipher_context_t *ctx) |
Free and clear the cipher-specific context of ctx. | |
int | cipher_init_ctx (cipher_context_t *ctx, const cipher_info_t *cipher_info) |
Initialises and fills the cipher context structure with the appropriate values. | |
int | cipher_free_ctx (cipher_context_t *ctx) |
Free the cipher-specific context of ctx. | |
int | cipher_setkey (cipher_context_t *ctx, const unsigned char *key, int key_length, const operation_t operation) |
Set the key to use with the given context. | |
int | cipher_set_iv (cipher_context_t *ctx, const unsigned char *iv, size_t iv_len) |
Set the initialization vector (IV) or nonce. | |
int | cipher_reset (cipher_context_t *ctx) |
Finish preparation of the given context. | |
int | cipher_update_ad (cipher_context_t *ctx, const unsigned char *ad, size_t ad_len) |
Add additional data (for AEAD ciphers). | |
int | cipher_update (cipher_context_t *ctx, const unsigned char *input, size_t ilen, unsigned char *output, size_t *olen) |
Generic cipher update function. | |
int | cipher_finish (cipher_context_t *ctx, unsigned char *output, size_t *olen) |
Generic cipher finalisation function. | |
int | cipher_set_padding_mode (cipher_context_t *ctx, cipher_padding_t mode) |
Set padding mode, for cipher modes that use padding. | |
int | cipher_write_tag (cipher_context_t *ctx, unsigned char *tag, size_t tag_len) |
Write tag for AEAD ciphers. | |
int | cipher_check_tag (cipher_context_t *ctx, const unsigned char *tag, size_t tag_len) |
Check tag for AEAD ciphers. | |
int | cipher_crypt (cipher_context_t *ctx, const unsigned char *iv, size_t iv_len, const unsigned char *input, size_t ilen, unsigned char *output, size_t *olen) |
Generic all-in-one encryption/decryption (for all ciphers except AEAD constructs). | |
int | cipher_auth_encrypt (cipher_context_t *ctx, const unsigned char *iv, size_t iv_len, const unsigned char *ad, size_t ad_len, const unsigned char *input, size_t ilen, unsigned char *output, size_t *olen, unsigned char *tag, size_t tag_len) |
Generic autenticated encryption (AEAD ciphers). | |
int | cipher_auth_decrypt (cipher_context_t *ctx, const unsigned char *iv, size_t iv_len, const unsigned char *ad, size_t ad_len, const unsigned char *input, size_t ilen, unsigned char *output, size_t *olen, const unsigned char *tag, size_t tag_len) |
Generic autenticated decryption (AEAD ciphers). | |
int | cipher_self_test (int verbose) |
Checkup routine. |
Detailed Description
Generic cipher wrapper for mbed TLS.
Copyright (C) 2006-2014, ARM Limited, All Rights Reserved
This file is part of mbed TLS (https://tls.mbed.org)
This program is free software; you can redistribute it and/or modify it under the terms of the GNU General Public License as published by the Free Software Foundation; either version 2 of the License, or (at your option) any later version.
This program is distributed in the hope that it will be useful, but WITHOUT ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU General Public License for more details.
You should have received a copy of the GNU General Public License along with this program; if not, write to the Free Software Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301 USA.
Definition in file cipher.c.
Function Documentation
int cipher_auth_decrypt | ( | cipher_context_t * | ctx, |
const unsigned char * | iv, | ||
size_t | iv_len, | ||
const unsigned char * | ad, | ||
size_t | ad_len, | ||
const unsigned char * | input, | ||
size_t | ilen, | ||
unsigned char * | output, | ||
size_t * | olen, | ||
const unsigned char * | tag, | ||
size_t | tag_len | ||
) |
Generic autenticated decryption (AEAD ciphers).
- Parameters:
-
ctx generic cipher context iv IV to use (or NONCE_COUNTER for CTR-mode ciphers) iv_len IV length for ciphers with variable-size IV; discarded by ciphers with fixed-size IV. ad Additional data to be authenticated. ad_len Length of ad. input buffer holding the input data ilen length of the input data output buffer for the output data. Should be able to hold at least ilen. olen length of the output data, will be filled with the actual number of bytes written. tag buffer holding the authentication tag tag_len length of the authentication tag
- Returns:
- 0 on success, or POLARSSL_ERR_CIPHER_BAD_INPUT_DATA, or POLARSSL_ERR_CIPHER_AUTH_FAILED if data isn't authentic, or a cipher specific error code.
- Note:
- If the data is not authentic, then the output buffer is zeroed out to prevent the unauthentic plaintext to be used by mistake, making this interface safer.
int cipher_auth_encrypt | ( | cipher_context_t * | ctx, |
const unsigned char * | iv, | ||
size_t | iv_len, | ||
const unsigned char * | ad, | ||
size_t | ad_len, | ||
const unsigned char * | input, | ||
size_t | ilen, | ||
unsigned char * | output, | ||
size_t * | olen, | ||
unsigned char * | tag, | ||
size_t | tag_len | ||
) |
Generic autenticated encryption (AEAD ciphers).
- Parameters:
-
ctx generic cipher context iv IV to use (or NONCE_COUNTER for CTR-mode ciphers) iv_len IV length for ciphers with variable-size IV; discarded by ciphers with fixed-size IV. ad Additional data to authenticate. ad_len Length of ad. input buffer holding the input data ilen length of the input data output buffer for the output data. Should be able to hold at least ilen. olen length of the output data, will be filled with the actual number of bytes written. tag buffer for the authentication tag tag_len desired tag length
- Returns:
- 0 on success, or POLARSSL_ERR_CIPHER_BAD_INPUT_DATA, or a cipher specific error code.
int cipher_check_tag | ( | cipher_context_t * | ctx, |
const unsigned char * | tag, | ||
size_t | tag_len | ||
) |
Check tag for AEAD ciphers.
Currently only supported with GCM. Must be called after cipher_finish().
- Parameters:
-
ctx Generic cipher context tag Buffer holding the tag tag_len Length of the tag to check
- Returns:
- 0 on success, or a specific error code.
int cipher_crypt | ( | cipher_context_t * | ctx, |
const unsigned char * | iv, | ||
size_t | iv_len, | ||
const unsigned char * | input, | ||
size_t | ilen, | ||
unsigned char * | output, | ||
size_t * | olen | ||
) |
Generic all-in-one encryption/decryption (for all ciphers except AEAD constructs).
- Parameters:
-
ctx generic cipher context iv IV to use (or NONCE_COUNTER for CTR-mode ciphers) iv_len IV length for ciphers with variable-size IV; discarded by ciphers with fixed-size IV. input buffer holding the input data ilen length of the input data output buffer for the output data. Should be able to hold at least ilen + block_size. Cannot be the same buffer as input! olen length of the output data, will be filled with the actual number of bytes written.
- Note:
- Some ciphers don't use IVs nor NONCE. For these ciphers, use iv = NULL and iv_len = 0.
- Returns:
- 0 on success, or POLARSSL_ERR_CIPHER_BAD_INPUT_DATA, or POLARSSL_ERR_CIPHER_FULL_BLOCK_EXPECTED if decryption expected a full block but was not provided one, or POLARSSL_ERR_CIPHER_INVALID_PADDING on invalid padding while decrypting, or a cipher specific error code.
int cipher_finish | ( | cipher_context_t * | ctx, |
unsigned char * | output, | ||
size_t * | olen | ||
) |
Generic cipher finalisation function.
If data still needs to be flushed from an incomplete block, data contained within it will be padded with the size of the last block, and written to the output buffer.
- Parameters:
-
ctx Generic cipher context output buffer to write data to. Needs block_size available. olen length of the data written to the output buffer.
- Returns:
- 0 on success, POLARSSL_ERR_CIPHER_BAD_INPUT_DATA if parameter verification fails, POLARSSL_ERR_CIPHER_FULL_BLOCK_EXPECTED if decryption expected a full block but was not provided one, POLARSSL_ERR_CIPHER_INVALID_PADDING on invalid padding while decrypting or a cipher specific error code.
void cipher_free | ( | cipher_context_t * | ctx ) |
int cipher_free_ctx | ( | cipher_context_t * | ctx ) |
const cipher_info_t* cipher_info_from_string | ( | const char * | cipher_name ) |
const cipher_info_t* cipher_info_from_type | ( | const cipher_type_t | cipher_type ) |
const cipher_info_t* cipher_info_from_values | ( | const cipher_id_t | cipher_id, |
int | key_length, | ||
const cipher_mode_t | mode | ||
) |
Returns the cipher information structure associated with the given cipher id, key size and mode.
- Parameters:
-
cipher_id Id of the cipher to search for (e.g. POLARSSL_CIPHER_ID_AES) key_length Length of the key in bits mode Cipher mode (e.g. POLARSSL_MODE_CBC)
- Returns:
- the cipher information structure associated with the given cipher_type, or NULL if not found.
void cipher_init | ( | cipher_context_t * | ctx ) |
int cipher_init_ctx | ( | cipher_context_t * | ctx, |
const cipher_info_t * | cipher_info | ||
) |
Initialises and fills the cipher context structure with the appropriate values.
- Note:
- Currently also clears structure. In future versions you will be required to call cipher_init() on the structure first.
- Parameters:
-
ctx context to initialise. May not be NULL. cipher_info cipher to use.
- Returns:
- 0 on success, POLARSSL_ERR_CIPHER_BAD_INPUT_DATA on parameter failure, POLARSSL_ERR_CIPHER_ALLOC_FAILED if allocation of the cipher-specific context failed.
const int* cipher_list | ( | void | ) |
int cipher_reset | ( | cipher_context_t * | ctx ) |
int cipher_self_test | ( | int | verbose ) |
int cipher_set_iv | ( | cipher_context_t * | ctx, |
const unsigned char * | iv, | ||
size_t | iv_len | ||
) |
Set the initialization vector (IV) or nonce.
- Parameters:
-
ctx generic cipher context iv IV to use (or NONCE_COUNTER for CTR-mode ciphers) iv_len IV length for ciphers with variable-size IV; discarded by ciphers with fixed-size IV.
- Returns:
- 0 on success, or POLARSSL_ERR_CIPHER_BAD_INPUT_DATA
- Note:
- Some ciphers don't use IVs nor NONCE. For these ciphers, this function has no effect.
int cipher_set_padding_mode | ( | cipher_context_t * | ctx, |
cipher_padding_t | mode | ||
) |
Set padding mode, for cipher modes that use padding.
(Default: PKCS7 padding.)
- Parameters:
-
ctx generic cipher context mode padding mode
- Returns:
- 0 on success, POLARSSL_ERR_CIPHER_FEATURE_UNAVAILABLE if selected padding mode is not supported, or POLARSSL_ERR_CIPHER_BAD_INPUT_DATA if the cipher mode does not support padding.
int cipher_setkey | ( | cipher_context_t * | ctx, |
const unsigned char * | key, | ||
int | key_length, | ||
const operation_t | operation | ||
) |
Set the key to use with the given context.
- Parameters:
-
ctx generic cipher context. May not be NULL. Must have been initialised using cipher_context_from_type or cipher_context_from_string. key The key to use. key_length key length to use, in bits. operation Operation that the key will be used for, either POLARSSL_ENCRYPT or POLARSSL_DECRYPT.
- Returns:
- 0 on success, POLARSSL_ERR_CIPHER_BAD_INPUT_DATA if parameter verification fails or a cipher specific error code.
int cipher_update | ( | cipher_context_t * | ctx, |
const unsigned char * | input, | ||
size_t | ilen, | ||
unsigned char * | output, | ||
size_t * | olen | ||
) |
Generic cipher update function.
Encrypts/decrypts using the given cipher context. Writes as many block size'd blocks of data as possible to output. Any data that cannot be written immediately will either be added to the next block, or flushed when cipher_final is called. Exception: for POLARSSL_MODE_ECB, expects single block in size (e.g. 16 bytes for AES)
- Parameters:
-
ctx generic cipher context input buffer holding the input data ilen length of the input data output buffer for the output data. Should be able to hold at least ilen + block_size. Cannot be the same buffer as input! olen length of the output data, will be filled with the actual number of bytes written.
- Returns:
- 0 on success, POLARSSL_ERR_CIPHER_BAD_INPUT_DATA if parameter verification fails, POLARSSL_ERR_CIPHER_FEATURE_UNAVAILABLE on an unsupported mode for a cipher or a cipher specific error code.
- Note:
- If the underlying cipher is GCM, all calls to this function, except the last one before cipher_finish(), must have ilen a multiple of the block size.
int cipher_update_ad | ( | cipher_context_t * | ctx, |
const unsigned char * | ad, | ||
size_t | ad_len | ||
) |
Add additional data (for AEAD ciphers).
Currently only supported with GCM. Must be called exactly once, after cipher_reset().
- Parameters:
-
ctx generic cipher context ad Additional data to use. ad_len Length of ad.
- Returns:
- 0 on success, or a specific error code.
int cipher_write_tag | ( | cipher_context_t * | ctx, |
unsigned char * | tag, | ||
size_t | tag_len | ||
) |
Write tag for AEAD ciphers.
Currently only supported with GCM. Must be called after cipher_finish().
- Parameters:
-
ctx Generic cipher context tag buffer to write the tag tag_len Length of the tag to write
- Returns:
- 0 on success, or a specific error code.
Generated on Tue Jul 12 2022 13:50:39 by
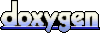