mbed TLS library
Dependents: HTTPClient-SSL WS_SERVER
cipher.c
00001 /** 00002 * \file cipher.c 00003 * 00004 * \brief Generic cipher wrapper for mbed TLS 00005 * 00006 * \author Adriaan de Jong <dejong@fox-it.com> 00007 * 00008 * Copyright (C) 2006-2014, ARM Limited, All Rights Reserved 00009 * 00010 * This file is part of mbed TLS (https://tls.mbed.org) 00011 * 00012 * This program is free software; you can redistribute it and/or modify 00013 * it under the terms of the GNU General Public License as published by 00014 * the Free Software Foundation; either version 2 of the License, or 00015 * (at your option) any later version. 00016 * 00017 * This program is distributed in the hope that it will be useful, 00018 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00019 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00020 * GNU General Public License for more details. 00021 * 00022 * You should have received a copy of the GNU General Public License along 00023 * with this program; if not, write to the Free Software Foundation, Inc., 00024 * 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301 USA. 00025 */ 00026 00027 #if !defined(POLARSSL_CONFIG_FILE) 00028 #include "polarssl/config.h" 00029 #else 00030 #include POLARSSL_CONFIG_FILE 00031 #endif 00032 00033 #if defined(POLARSSL_CIPHER_C) 00034 00035 #include "polarssl/cipher.h" 00036 #include "polarssl/cipher_wrap.h" 00037 00038 #include <stdlib.h> 00039 #include <string.h> 00040 00041 #if defined(POLARSSL_GCM_C) 00042 #include "polarssl/gcm.h" 00043 #endif 00044 00045 #if defined(POLARSSL_CCM_C) 00046 #include "polarssl/ccm.h" 00047 #endif 00048 00049 #if defined(POLARSSL_ARC4_C) || defined(POLARSSL_CIPHER_NULL_CIPHER) 00050 #define POLARSSL_CIPHER_MODE_STREAM 00051 #endif 00052 00053 #if defined(_MSC_VER) && !defined strcasecmp && !defined(EFIX64) && \ 00054 !defined(EFI32) 00055 #define strcasecmp _stricmp 00056 #endif 00057 00058 /* Implementation that should never be optimized out by the compiler */ 00059 static void polarssl_zeroize( void *v, size_t n ) { 00060 volatile unsigned char *p = v; while( n-- ) *p++ = 0; 00061 } 00062 00063 static int supported_init = 0; 00064 00065 const int *cipher_list( void ) 00066 { 00067 const cipher_definition_t *def; 00068 int *type; 00069 00070 if( ! supported_init ) 00071 { 00072 def = cipher_definitions; 00073 type = supported_ciphers; 00074 00075 while( def->type != 0 ) 00076 *type++ = (*def++).type; 00077 00078 *type = 0; 00079 00080 supported_init = 1; 00081 } 00082 00083 return( supported_ciphers ); 00084 } 00085 00086 const cipher_info_t *cipher_info_from_type( const cipher_type_t cipher_type ) 00087 { 00088 const cipher_definition_t *def; 00089 00090 for( def = cipher_definitions; def->info != NULL; def++ ) 00091 if( def->type == cipher_type ) 00092 return( def->info ); 00093 00094 return( NULL ); 00095 } 00096 00097 const cipher_info_t *cipher_info_from_string( const char *cipher_name ) 00098 { 00099 const cipher_definition_t *def; 00100 00101 if( NULL == cipher_name ) 00102 return( NULL ); 00103 00104 for( def = cipher_definitions; def->info != NULL; def++ ) 00105 if( ! strcasecmp( def->info->name, cipher_name ) ) 00106 return( def->info ); 00107 00108 return( NULL ); 00109 } 00110 00111 const cipher_info_t *cipher_info_from_values( const cipher_id_t cipher_id, 00112 int key_length, 00113 const cipher_mode_t mode ) 00114 { 00115 const cipher_definition_t *def; 00116 00117 for( def = cipher_definitions; def->info != NULL; def++ ) 00118 if( def->info->base->cipher == cipher_id && 00119 def->info->key_length == (unsigned) key_length && 00120 def->info->mode == mode ) 00121 return( def->info ); 00122 00123 return( NULL ); 00124 } 00125 00126 void cipher_init( cipher_context_t *ctx ) 00127 { 00128 memset( ctx, 0, sizeof( cipher_context_t ) ); 00129 } 00130 00131 void cipher_free( cipher_context_t *ctx ) 00132 { 00133 if( ctx == NULL ) 00134 return; 00135 00136 if( ctx->cipher_ctx ) 00137 ctx->cipher_info->base->ctx_free_func( ctx->cipher_ctx ); 00138 00139 polarssl_zeroize( ctx, sizeof(cipher_context_t) ); 00140 } 00141 00142 int cipher_init_ctx( cipher_context_t *ctx, const cipher_info_t *cipher_info ) 00143 { 00144 if( NULL == cipher_info || NULL == ctx ) 00145 return( POLARSSL_ERR_CIPHER_BAD_INPUT_DATA ); 00146 00147 memset( ctx, 0, sizeof( cipher_context_t ) ); 00148 00149 if( NULL == ( ctx->cipher_ctx = cipher_info->base->ctx_alloc_func() ) ) 00150 return( POLARSSL_ERR_CIPHER_ALLOC_FAILED ); 00151 00152 ctx->cipher_info = cipher_info; 00153 00154 #if defined(POLARSSL_CIPHER_MODE_WITH_PADDING) 00155 /* 00156 * Ignore possible errors caused by a cipher mode that doesn't use padding 00157 */ 00158 #if defined(POLARSSL_CIPHER_PADDING_PKCS7) 00159 (void) cipher_set_padding_mode( ctx, POLARSSL_PADDING_PKCS7 ); 00160 #else 00161 (void) cipher_set_padding_mode( ctx, POLARSSL_PADDING_NONE ); 00162 #endif 00163 #endif /* POLARSSL_CIPHER_MODE_WITH_PADDING */ 00164 00165 return( 0 ); 00166 } 00167 00168 #if ! defined(POLARSSL_DEPRECATED_REMOVED) 00169 int cipher_free_ctx( cipher_context_t *ctx ) 00170 { 00171 cipher_free( ctx ); 00172 00173 return( 0 ); 00174 } 00175 #endif 00176 00177 int cipher_setkey( cipher_context_t *ctx, const unsigned char *key, 00178 int key_length, const operation_t operation ) 00179 { 00180 if( NULL == ctx || NULL == ctx->cipher_info ) 00181 return( POLARSSL_ERR_CIPHER_BAD_INPUT_DATA ); 00182 00183 if( ( ctx->cipher_info->flags & POLARSSL_CIPHER_VARIABLE_KEY_LEN ) == 0 && 00184 (int) ctx->cipher_info->key_length != key_length ) 00185 { 00186 return( POLARSSL_ERR_CIPHER_BAD_INPUT_DATA ); 00187 } 00188 00189 ctx->key_length = key_length; 00190 ctx->operation = operation; 00191 00192 /* 00193 * For CFB and CTR mode always use the encryption key schedule 00194 */ 00195 if( POLARSSL_ENCRYPT == operation || 00196 POLARSSL_MODE_CFB == ctx->cipher_info->mode || 00197 POLARSSL_MODE_CTR == ctx->cipher_info->mode ) 00198 { 00199 return ctx->cipher_info->base->setkey_enc_func( ctx->cipher_ctx, key, 00200 ctx->key_length ); 00201 } 00202 00203 if( POLARSSL_DECRYPT == operation ) 00204 return ctx->cipher_info->base->setkey_dec_func( ctx->cipher_ctx, key, 00205 ctx->key_length ); 00206 00207 return( POLARSSL_ERR_CIPHER_BAD_INPUT_DATA ); 00208 } 00209 00210 int cipher_set_iv( cipher_context_t *ctx, 00211 const unsigned char *iv, size_t iv_len ) 00212 { 00213 size_t actual_iv_size; 00214 00215 if( NULL == ctx || NULL == ctx->cipher_info || NULL == iv ) 00216 return( POLARSSL_ERR_CIPHER_BAD_INPUT_DATA ); 00217 00218 /* avoid buffer overflow in ctx->iv */ 00219 if( iv_len > POLARSSL_MAX_IV_LENGTH ) 00220 return( POLARSSL_ERR_CIPHER_FEATURE_UNAVAILABLE ); 00221 00222 if( ( ctx->cipher_info->flags & POLARSSL_CIPHER_VARIABLE_IV_LEN ) != 0 ) 00223 actual_iv_size = iv_len; 00224 else 00225 { 00226 actual_iv_size = ctx->cipher_info->iv_size; 00227 00228 /* avoid reading past the end of input buffer */ 00229 if( actual_iv_size > iv_len ) 00230 return( POLARSSL_ERR_CIPHER_BAD_INPUT_DATA ); 00231 } 00232 00233 memcpy( ctx->iv, iv, actual_iv_size ); 00234 ctx->iv_size = actual_iv_size; 00235 00236 return( 0 ); 00237 } 00238 00239 int cipher_reset( cipher_context_t *ctx ) 00240 { 00241 if( NULL == ctx || NULL == ctx->cipher_info ) 00242 return( POLARSSL_ERR_CIPHER_BAD_INPUT_DATA ); 00243 00244 ctx->unprocessed_len = 0; 00245 00246 return( 0 ); 00247 } 00248 00249 #if defined(POLARSSL_GCM_C) 00250 int cipher_update_ad( cipher_context_t *ctx, 00251 const unsigned char *ad, size_t ad_len ) 00252 { 00253 if( NULL == ctx || NULL == ctx->cipher_info ) 00254 return( POLARSSL_ERR_CIPHER_BAD_INPUT_DATA ); 00255 00256 if( POLARSSL_MODE_GCM == ctx->cipher_info->mode ) 00257 { 00258 return gcm_starts( (gcm_context *) ctx->cipher_ctx, ctx->operation, 00259 ctx->iv, ctx->iv_size, ad, ad_len ); 00260 } 00261 00262 return( 0 ); 00263 } 00264 #endif /* POLARSSL_GCM_C */ 00265 00266 int cipher_update( cipher_context_t *ctx, const unsigned char *input, 00267 size_t ilen, unsigned char *output, size_t *olen ) 00268 { 00269 int ret; 00270 00271 if( NULL == ctx || NULL == ctx->cipher_info || NULL == olen ) 00272 { 00273 return( POLARSSL_ERR_CIPHER_BAD_INPUT_DATA ); 00274 } 00275 00276 *olen = 0; 00277 00278 if( ctx->cipher_info->mode == POLARSSL_MODE_ECB ) 00279 { 00280 if( ilen != cipher_get_block_size( ctx ) ) 00281 return( POLARSSL_ERR_CIPHER_FULL_BLOCK_EXPECTED ); 00282 00283 *olen = ilen; 00284 00285 if( 0 != ( ret = ctx->cipher_info->base->ecb_func( ctx->cipher_ctx, 00286 ctx->operation, input, output ) ) ) 00287 { 00288 return( ret ); 00289 } 00290 00291 return( 0 ); 00292 } 00293 00294 #if defined(POLARSSL_GCM_C) 00295 if( ctx->cipher_info->mode == POLARSSL_MODE_GCM ) 00296 { 00297 *olen = ilen; 00298 return gcm_update( (gcm_context *) ctx->cipher_ctx, ilen, input, 00299 output ); 00300 } 00301 #endif 00302 00303 if( input == output && 00304 ( ctx->unprocessed_len != 0 || ilen % cipher_get_block_size( ctx ) ) ) 00305 { 00306 return( POLARSSL_ERR_CIPHER_BAD_INPUT_DATA ); 00307 } 00308 00309 #if defined(POLARSSL_CIPHER_MODE_CBC) 00310 if( ctx->cipher_info->mode == POLARSSL_MODE_CBC ) 00311 { 00312 size_t copy_len = 0; 00313 00314 /* 00315 * If there is not enough data for a full block, cache it. 00316 */ 00317 if( ( ctx->operation == POLARSSL_DECRYPT && 00318 ilen + ctx->unprocessed_len <= cipher_get_block_size( ctx ) ) || 00319 ( ctx->operation == POLARSSL_ENCRYPT && 00320 ilen + ctx->unprocessed_len < cipher_get_block_size( ctx ) ) ) 00321 { 00322 memcpy( &( ctx->unprocessed_data[ctx->unprocessed_len] ), input, 00323 ilen ); 00324 00325 ctx->unprocessed_len += ilen; 00326 return( 0 ); 00327 } 00328 00329 /* 00330 * Process cached data first 00331 */ 00332 if( ctx->unprocessed_len != 0 ) 00333 { 00334 copy_len = cipher_get_block_size( ctx ) - ctx->unprocessed_len; 00335 00336 memcpy( &( ctx->unprocessed_data[ctx->unprocessed_len] ), input, 00337 copy_len ); 00338 00339 if( 0 != ( ret = ctx->cipher_info->base->cbc_func( ctx->cipher_ctx, 00340 ctx->operation, cipher_get_block_size( ctx ), ctx->iv, 00341 ctx->unprocessed_data, output ) ) ) 00342 { 00343 return( ret ); 00344 } 00345 00346 *olen += cipher_get_block_size( ctx ); 00347 output += cipher_get_block_size( ctx ); 00348 ctx->unprocessed_len = 0; 00349 00350 input += copy_len; 00351 ilen -= copy_len; 00352 } 00353 00354 /* 00355 * Cache final, incomplete block 00356 */ 00357 if( 0 != ilen ) 00358 { 00359 copy_len = ilen % cipher_get_block_size( ctx ); 00360 if( copy_len == 0 && ctx->operation == POLARSSL_DECRYPT ) 00361 copy_len = cipher_get_block_size( ctx ); 00362 00363 memcpy( ctx->unprocessed_data, &( input[ilen - copy_len] ), 00364 copy_len ); 00365 00366 ctx->unprocessed_len += copy_len; 00367 ilen -= copy_len; 00368 } 00369 00370 /* 00371 * Process remaining full blocks 00372 */ 00373 if( ilen ) 00374 { 00375 if( 0 != ( ret = ctx->cipher_info->base->cbc_func( ctx->cipher_ctx, 00376 ctx->operation, ilen, ctx->iv, input, output ) ) ) 00377 { 00378 return( ret ); 00379 } 00380 00381 *olen += ilen; 00382 } 00383 00384 return( 0 ); 00385 } 00386 #endif /* POLARSSL_CIPHER_MODE_CBC */ 00387 00388 #if defined(POLARSSL_CIPHER_MODE_CFB) 00389 if( ctx->cipher_info->mode == POLARSSL_MODE_CFB ) 00390 { 00391 if( 0 != ( ret = ctx->cipher_info->base->cfb_func( ctx->cipher_ctx, 00392 ctx->operation, ilen, &ctx->unprocessed_len, ctx->iv, 00393 input, output ) ) ) 00394 { 00395 return( ret ); 00396 } 00397 00398 *olen = ilen; 00399 00400 return( 0 ); 00401 } 00402 #endif /* POLARSSL_CIPHER_MODE_CFB */ 00403 00404 #if defined(POLARSSL_CIPHER_MODE_CTR) 00405 if( ctx->cipher_info->mode == POLARSSL_MODE_CTR ) 00406 { 00407 if( 0 != ( ret = ctx->cipher_info->base->ctr_func( ctx->cipher_ctx, 00408 ilen, &ctx->unprocessed_len, ctx->iv, 00409 ctx->unprocessed_data, input, output ) ) ) 00410 { 00411 return( ret ); 00412 } 00413 00414 *olen = ilen; 00415 00416 return( 0 ); 00417 } 00418 #endif /* POLARSSL_CIPHER_MODE_CTR */ 00419 00420 #if defined(POLARSSL_CIPHER_MODE_STREAM) 00421 if( ctx->cipher_info->mode == POLARSSL_MODE_STREAM ) 00422 { 00423 if( 0 != ( ret = ctx->cipher_info->base->stream_func( ctx->cipher_ctx, 00424 ilen, input, output ) ) ) 00425 { 00426 return( ret ); 00427 } 00428 00429 *olen = ilen; 00430 00431 return( 0 ); 00432 } 00433 #endif /* POLARSSL_CIPHER_MODE_STREAM */ 00434 00435 return( POLARSSL_ERR_CIPHER_FEATURE_UNAVAILABLE ); 00436 } 00437 00438 #if defined(POLARSSL_CIPHER_MODE_WITH_PADDING) 00439 #if defined(POLARSSL_CIPHER_PADDING_PKCS7) 00440 /* 00441 * PKCS7 (and PKCS5) padding: fill with ll bytes, with ll = padding_len 00442 */ 00443 static void add_pkcs_padding( unsigned char *output, size_t output_len, 00444 size_t data_len ) 00445 { 00446 size_t padding_len = output_len - data_len; 00447 unsigned char i; 00448 00449 for( i = 0; i < padding_len; i++ ) 00450 output[data_len + i] = (unsigned char) padding_len; 00451 } 00452 00453 static int get_pkcs_padding( unsigned char *input, size_t input_len, 00454 size_t *data_len ) 00455 { 00456 size_t i, pad_idx; 00457 unsigned char padding_len, bad = 0; 00458 00459 if( NULL == input || NULL == data_len ) 00460 return( POLARSSL_ERR_CIPHER_BAD_INPUT_DATA ); 00461 00462 padding_len = input[input_len - 1]; 00463 *data_len = input_len - padding_len; 00464 00465 /* Avoid logical || since it results in a branch */ 00466 bad |= padding_len > input_len; 00467 bad |= padding_len == 0; 00468 00469 /* The number of bytes checked must be independent of padding_len, 00470 * so pick input_len, which is usually 8 or 16 (one block) */ 00471 pad_idx = input_len - padding_len; 00472 for( i = 0; i < input_len; i++ ) 00473 bad |= ( input[i] ^ padding_len ) * ( i >= pad_idx ); 00474 00475 return( POLARSSL_ERR_CIPHER_INVALID_PADDING * ( bad != 0 ) ); 00476 } 00477 #endif /* POLARSSL_CIPHER_PADDING_PKCS7 */ 00478 00479 #if defined(POLARSSL_CIPHER_PADDING_ONE_AND_ZEROS) 00480 /* 00481 * One and zeros padding: fill with 80 00 ... 00 00482 */ 00483 static void add_one_and_zeros_padding( unsigned char *output, 00484 size_t output_len, size_t data_len ) 00485 { 00486 size_t padding_len = output_len - data_len; 00487 unsigned char i = 0; 00488 00489 output[data_len] = 0x80; 00490 for( i = 1; i < padding_len; i++ ) 00491 output[data_len + i] = 0x00; 00492 } 00493 00494 static int get_one_and_zeros_padding( unsigned char *input, size_t input_len, 00495 size_t *data_len ) 00496 { 00497 size_t i; 00498 unsigned char done = 0, prev_done, bad; 00499 00500 if( NULL == input || NULL == data_len ) 00501 return( POLARSSL_ERR_CIPHER_BAD_INPUT_DATA ); 00502 00503 bad = 0xFF; 00504 *data_len = 0; 00505 for( i = input_len; i > 0; i-- ) 00506 { 00507 prev_done = done; 00508 done |= ( input[i-1] != 0 ); 00509 *data_len |= ( i - 1 ) * ( done != prev_done ); 00510 bad &= ( input[i-1] ^ 0x80 ) | ( done == prev_done ); 00511 } 00512 00513 return( POLARSSL_ERR_CIPHER_INVALID_PADDING * ( bad != 0 ) ); 00514 00515 } 00516 #endif /* POLARSSL_CIPHER_PADDING_ONE_AND_ZEROS */ 00517 00518 #if defined(POLARSSL_CIPHER_PADDING_ZEROS_AND_LEN) 00519 /* 00520 * Zeros and len padding: fill with 00 ... 00 ll, where ll is padding length 00521 */ 00522 static void add_zeros_and_len_padding( unsigned char *output, 00523 size_t output_len, size_t data_len ) 00524 { 00525 size_t padding_len = output_len - data_len; 00526 unsigned char i = 0; 00527 00528 for( i = 1; i < padding_len; i++ ) 00529 output[data_len + i - 1] = 0x00; 00530 output[output_len - 1] = (unsigned char) padding_len; 00531 } 00532 00533 static int get_zeros_and_len_padding( unsigned char *input, size_t input_len, 00534 size_t *data_len ) 00535 { 00536 size_t i, pad_idx; 00537 unsigned char padding_len, bad = 0; 00538 00539 if( NULL == input || NULL == data_len ) 00540 return( POLARSSL_ERR_CIPHER_BAD_INPUT_DATA ); 00541 00542 padding_len = input[input_len - 1]; 00543 *data_len = input_len - padding_len; 00544 00545 /* Avoid logical || since it results in a branch */ 00546 bad |= padding_len > input_len; 00547 bad |= padding_len == 0; 00548 00549 /* The number of bytes checked must be independent of padding_len */ 00550 pad_idx = input_len - padding_len; 00551 for( i = 0; i < input_len - 1; i++ ) 00552 bad |= input[i] * ( i >= pad_idx ); 00553 00554 return( POLARSSL_ERR_CIPHER_INVALID_PADDING * ( bad != 0 ) ); 00555 } 00556 #endif /* POLARSSL_CIPHER_PADDING_ZEROS_AND_LEN */ 00557 00558 #if defined(POLARSSL_CIPHER_PADDING_ZEROS) 00559 /* 00560 * Zero padding: fill with 00 ... 00 00561 */ 00562 static void add_zeros_padding( unsigned char *output, 00563 size_t output_len, size_t data_len ) 00564 { 00565 size_t i; 00566 00567 for( i = data_len; i < output_len; i++ ) 00568 output[i] = 0x00; 00569 } 00570 00571 static int get_zeros_padding( unsigned char *input, size_t input_len, 00572 size_t *data_len ) 00573 { 00574 size_t i; 00575 unsigned char done = 0, prev_done; 00576 00577 if( NULL == input || NULL == data_len ) 00578 return( POLARSSL_ERR_CIPHER_BAD_INPUT_DATA ); 00579 00580 *data_len = 0; 00581 for( i = input_len; i > 0; i-- ) 00582 { 00583 prev_done = done; 00584 done |= ( input[i-1] != 0 ); 00585 *data_len |= i * ( done != prev_done ); 00586 } 00587 00588 return( 0 ); 00589 } 00590 #endif /* POLARSSL_CIPHER_PADDING_ZEROS */ 00591 00592 /* 00593 * No padding: don't pad :) 00594 * 00595 * There is no add_padding function (check for NULL in cipher_finish) 00596 * but a trivial get_padding function 00597 */ 00598 static int get_no_padding( unsigned char *input, size_t input_len, 00599 size_t *data_len ) 00600 { 00601 if( NULL == input || NULL == data_len ) 00602 return( POLARSSL_ERR_CIPHER_BAD_INPUT_DATA ); 00603 00604 *data_len = input_len; 00605 00606 return( 0 ); 00607 } 00608 #endif /* POLARSSL_CIPHER_MODE_WITH_PADDING */ 00609 00610 int cipher_finish( cipher_context_t *ctx, 00611 unsigned char *output, size_t *olen ) 00612 { 00613 if( NULL == ctx || NULL == ctx->cipher_info || NULL == olen ) 00614 return( POLARSSL_ERR_CIPHER_BAD_INPUT_DATA ); 00615 00616 *olen = 0; 00617 00618 if( POLARSSL_MODE_CFB == ctx->cipher_info->mode || 00619 POLARSSL_MODE_CTR == ctx->cipher_info->mode || 00620 POLARSSL_MODE_GCM == ctx->cipher_info->mode || 00621 POLARSSL_MODE_STREAM == ctx->cipher_info->mode ) 00622 { 00623 return( 0 ); 00624 } 00625 00626 if( POLARSSL_MODE_ECB == ctx->cipher_info->mode ) 00627 { 00628 if( ctx->unprocessed_len != 0 ) 00629 return( POLARSSL_ERR_CIPHER_FULL_BLOCK_EXPECTED ); 00630 00631 return( 0 ); 00632 } 00633 00634 #if defined(POLARSSL_CIPHER_MODE_CBC) 00635 if( POLARSSL_MODE_CBC == ctx->cipher_info->mode ) 00636 { 00637 int ret = 0; 00638 00639 if( POLARSSL_ENCRYPT == ctx->operation ) 00640 { 00641 /* check for 'no padding' mode */ 00642 if( NULL == ctx->add_padding ) 00643 { 00644 if( 0 != ctx->unprocessed_len ) 00645 return( POLARSSL_ERR_CIPHER_FULL_BLOCK_EXPECTED ); 00646 00647 return( 0 ); 00648 } 00649 00650 ctx->add_padding( ctx->unprocessed_data, cipher_get_iv_size( ctx ), 00651 ctx->unprocessed_len ); 00652 } 00653 else if( cipher_get_block_size( ctx ) != ctx->unprocessed_len ) 00654 { 00655 /* 00656 * For decrypt operations, expect a full block, 00657 * or an empty block if no padding 00658 */ 00659 if( NULL == ctx->add_padding && 0 == ctx->unprocessed_len ) 00660 return( 0 ); 00661 00662 return( POLARSSL_ERR_CIPHER_FULL_BLOCK_EXPECTED ); 00663 } 00664 00665 /* cipher block */ 00666 if( 0 != ( ret = ctx->cipher_info->base->cbc_func( ctx->cipher_ctx, 00667 ctx->operation, cipher_get_block_size( ctx ), ctx->iv, 00668 ctx->unprocessed_data, output ) ) ) 00669 { 00670 return( ret ); 00671 } 00672 00673 /* Set output size for decryption */ 00674 if( POLARSSL_DECRYPT == ctx->operation ) 00675 return ctx->get_padding( output, cipher_get_block_size( ctx ), 00676 olen ); 00677 00678 /* Set output size for encryption */ 00679 *olen = cipher_get_block_size( ctx ); 00680 return( 0 ); 00681 } 00682 #else 00683 ((void) output); 00684 #endif /* POLARSSL_CIPHER_MODE_CBC */ 00685 00686 return( POLARSSL_ERR_CIPHER_FEATURE_UNAVAILABLE ); 00687 } 00688 00689 #if defined(POLARSSL_CIPHER_MODE_WITH_PADDING) 00690 int cipher_set_padding_mode( cipher_context_t *ctx, cipher_padding_t mode ) 00691 { 00692 if( NULL == ctx || 00693 POLARSSL_MODE_CBC != ctx->cipher_info->mode ) 00694 { 00695 return( POLARSSL_ERR_CIPHER_BAD_INPUT_DATA ); 00696 } 00697 00698 switch( mode ) 00699 { 00700 #if defined(POLARSSL_CIPHER_PADDING_PKCS7) 00701 case POLARSSL_PADDING_PKCS7: 00702 ctx->add_padding = add_pkcs_padding; 00703 ctx->get_padding = get_pkcs_padding; 00704 break; 00705 #endif 00706 #if defined(POLARSSL_CIPHER_PADDING_ONE_AND_ZEROS) 00707 case POLARSSL_PADDING_ONE_AND_ZEROS: 00708 ctx->add_padding = add_one_and_zeros_padding; 00709 ctx->get_padding = get_one_and_zeros_padding; 00710 break; 00711 #endif 00712 #if defined(POLARSSL_CIPHER_PADDING_ZEROS_AND_LEN) 00713 case POLARSSL_PADDING_ZEROS_AND_LEN: 00714 ctx->add_padding = add_zeros_and_len_padding; 00715 ctx->get_padding = get_zeros_and_len_padding; 00716 break; 00717 #endif 00718 #if defined(POLARSSL_CIPHER_PADDING_ZEROS) 00719 case POLARSSL_PADDING_ZEROS: 00720 ctx->add_padding = add_zeros_padding; 00721 ctx->get_padding = get_zeros_padding; 00722 break; 00723 #endif 00724 case POLARSSL_PADDING_NONE: 00725 ctx->add_padding = NULL; 00726 ctx->get_padding = get_no_padding; 00727 break; 00728 00729 default: 00730 return( POLARSSL_ERR_CIPHER_FEATURE_UNAVAILABLE ); 00731 } 00732 00733 return( 0 ); 00734 } 00735 #endif /* POLARSSL_CIPHER_MODE_WITH_PADDING */ 00736 00737 #if defined(POLARSSL_GCM_C) 00738 int cipher_write_tag( cipher_context_t *ctx, 00739 unsigned char *tag, size_t tag_len ) 00740 { 00741 if( NULL == ctx || NULL == ctx->cipher_info || NULL == tag ) 00742 return( POLARSSL_ERR_CIPHER_BAD_INPUT_DATA ); 00743 00744 if( POLARSSL_ENCRYPT != ctx->operation ) 00745 return( POLARSSL_ERR_CIPHER_BAD_INPUT_DATA ); 00746 00747 if( POLARSSL_MODE_GCM == ctx->cipher_info->mode ) 00748 return gcm_finish( (gcm_context *) ctx->cipher_ctx, tag, tag_len ); 00749 00750 return( 0 ); 00751 } 00752 00753 int cipher_check_tag( cipher_context_t *ctx, 00754 const unsigned char *tag, size_t tag_len ) 00755 { 00756 int ret; 00757 00758 if( NULL == ctx || NULL == ctx->cipher_info || 00759 POLARSSL_DECRYPT != ctx->operation ) 00760 { 00761 return( POLARSSL_ERR_CIPHER_BAD_INPUT_DATA ); 00762 } 00763 00764 if( POLARSSL_MODE_GCM == ctx->cipher_info->mode ) 00765 { 00766 unsigned char check_tag[16]; 00767 size_t i; 00768 int diff; 00769 00770 if( tag_len > sizeof( check_tag ) ) 00771 return( POLARSSL_ERR_CIPHER_BAD_INPUT_DATA ); 00772 00773 if( 0 != ( ret = gcm_finish( (gcm_context *) ctx->cipher_ctx, 00774 check_tag, tag_len ) ) ) 00775 { 00776 return( ret ); 00777 } 00778 00779 /* Check the tag in "constant-time" */ 00780 for( diff = 0, i = 0; i < tag_len; i++ ) 00781 diff |= tag[i] ^ check_tag[i]; 00782 00783 if( diff != 0 ) 00784 return( POLARSSL_ERR_CIPHER_AUTH_FAILED ); 00785 00786 return( 0 ); 00787 } 00788 00789 return( 0 ); 00790 } 00791 #endif /* POLARSSL_GCM_C */ 00792 00793 /* 00794 * Packet-oriented wrapper for non-AEAD modes 00795 */ 00796 int cipher_crypt( cipher_context_t *ctx, 00797 const unsigned char *iv, size_t iv_len, 00798 const unsigned char *input, size_t ilen, 00799 unsigned char *output, size_t *olen ) 00800 { 00801 int ret; 00802 size_t finish_olen; 00803 00804 if( ( ret = cipher_set_iv( ctx, iv, iv_len ) ) != 0 ) 00805 return( ret ); 00806 00807 if( ( ret = cipher_reset( ctx ) ) != 0 ) 00808 return( ret ); 00809 00810 if( ( ret = cipher_update( ctx, input, ilen, output, olen ) ) != 0 ) 00811 return( ret ); 00812 00813 if( ( ret = cipher_finish( ctx, output + *olen, &finish_olen ) ) != 0 ) 00814 return( ret ); 00815 00816 *olen += finish_olen; 00817 00818 return( 0 ); 00819 } 00820 00821 #if defined(POLARSSL_CIPHER_MODE_AEAD) 00822 /* 00823 * Packet-oriented encryption for AEAD modes 00824 */ 00825 int cipher_auth_encrypt( cipher_context_t *ctx, 00826 const unsigned char *iv, size_t iv_len, 00827 const unsigned char *ad, size_t ad_len, 00828 const unsigned char *input, size_t ilen, 00829 unsigned char *output, size_t *olen, 00830 unsigned char *tag, size_t tag_len ) 00831 { 00832 #if defined(POLARSSL_GCM_C) 00833 if( POLARSSL_MODE_GCM == ctx->cipher_info->mode ) 00834 { 00835 *olen = ilen; 00836 return( gcm_crypt_and_tag( ctx->cipher_ctx, GCM_ENCRYPT, ilen, 00837 iv, iv_len, ad, ad_len, input, output, 00838 tag_len, tag ) ); 00839 } 00840 #endif /* POLARSSL_GCM_C */ 00841 #if defined(POLARSSL_CCM_C) 00842 if( POLARSSL_MODE_CCM == ctx->cipher_info->mode ) 00843 { 00844 *olen = ilen; 00845 return( ccm_encrypt_and_tag( ctx->cipher_ctx, ilen, 00846 iv, iv_len, ad, ad_len, input, output, 00847 tag, tag_len ) ); 00848 } 00849 #endif /* POLARSSL_CCM_C */ 00850 00851 return( POLARSSL_ERR_CIPHER_FEATURE_UNAVAILABLE ); 00852 } 00853 00854 /* 00855 * Packet-oriented decryption for AEAD modes 00856 */ 00857 int cipher_auth_decrypt( cipher_context_t *ctx, 00858 const unsigned char *iv, size_t iv_len, 00859 const unsigned char *ad, size_t ad_len, 00860 const unsigned char *input, size_t ilen, 00861 unsigned char *output, size_t *olen, 00862 const unsigned char *tag, size_t tag_len ) 00863 { 00864 #if defined(POLARSSL_GCM_C) 00865 if( POLARSSL_MODE_GCM == ctx->cipher_info->mode ) 00866 { 00867 int ret; 00868 00869 *olen = ilen; 00870 ret = gcm_auth_decrypt( ctx->cipher_ctx, ilen, 00871 iv, iv_len, ad, ad_len, 00872 tag, tag_len, input, output ); 00873 00874 if( ret == POLARSSL_ERR_GCM_AUTH_FAILED ) 00875 ret = POLARSSL_ERR_CIPHER_AUTH_FAILED; 00876 00877 return( ret ); 00878 } 00879 #endif /* POLARSSL_GCM_C */ 00880 #if defined(POLARSSL_CCM_C) 00881 if( POLARSSL_MODE_CCM == ctx->cipher_info->mode ) 00882 { 00883 int ret; 00884 00885 *olen = ilen; 00886 ret = ccm_auth_decrypt( ctx->cipher_ctx, ilen, 00887 iv, iv_len, ad, ad_len, 00888 input, output, tag, tag_len ); 00889 00890 if( ret == POLARSSL_ERR_CCM_AUTH_FAILED ) 00891 ret = POLARSSL_ERR_CIPHER_AUTH_FAILED; 00892 00893 return( ret ); 00894 } 00895 #endif /* POLARSSL_CCM_C */ 00896 00897 return( POLARSSL_ERR_CIPHER_FEATURE_UNAVAILABLE ); 00898 } 00899 #endif /* POLARSSL_CIPHER_MODE_AEAD */ 00900 00901 00902 #if defined(POLARSSL_SELF_TEST) 00903 00904 /* 00905 * Checkup routine 00906 */ 00907 int cipher_self_test( int verbose ) 00908 { 00909 ((void) verbose); 00910 00911 return( 0 ); 00912 } 00913 00914 #endif /* POLARSSL_SELF_TEST */ 00915 00916 #endif /* POLARSSL_CIPHER_C */ 00917
Generated on Tue Jul 12 2022 13:50:36 by
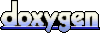