This library provides simplified I2C access to a Microchip MCP23x17 GPIO expender device, including a general interface for any GPIO expender
CMCP23017_I2C Class Reference
This class provides simplified I2C access to a Microchip 2MCP28x17 GPIO expender. More...
#include <MCP23017_I2C.h>
Inherits AbstractGpioExpender.
Public Types | |
enum | InterruptModes |
Interrupt modes. More... | |
enum | PullModes |
Pull modes. More... | |
Public Member Functions | |
CMCP23017_I2C (const PinName p_sda, const PinName p_scl, const unsigned char p_address, const PinName p_intA=NC, const PinName p_intB=NC, const PinName p_reset=NC, const bool p_internalPullUp=true, const unsigned int p_frequency=400000) | |
Constructor with Write Protect command pin wired. | |
virtual | ~CMCP23017_I2C () |
Destructor. | |
bool | Initialize (const unsigned char p_gpioAFlags=0x00, const unsigned char p_gpioBFlags=0x00) |
Initialize the module, configuring the module and starting the clock. | |
const I2C * | operator* () |
Used to return the unique instance of I2C instance. | |
virtual void | reset () |
Reset the device. | |
virtual void | setupInterrupts (const unsigned char mirroring=0x00, const unsigned char p_openDrain=0x00, const unsigned char polarity=0x00) |
Setup device interrupt mechanism. | |
virtual int | setupInterruptPin (const unsigned char p_gpioId, const InterruptModes p_mode=OnRising) |
Setup interrupt for a specific IO port. | |
virtual int | setupPullPin (const unsigned char p_gpioId, const PullModes p_mode=PullOff) |
Setup pull mode for a specific IO port. | |
virtual int | getLastInterruptPinAndValue (unsigned char *p_gpioId, unsigned char *p_value) |
Get interrupt information and clear it. | |
virtual int | read (const unsigned char p_gpioId, unsigned char *p_value) |
Read the specific GPIO port pin. | |
virtual int | write (const unsigned char p_gpioId, const unsigned char p_value) |
Write value to the specific GPIO port pin. | |
virtual void | setIntrACallback (void(*p_fptr)(void)) |
Attach a function to call when a interrupt occurs on the GPIOA input ports. | |
virtual void | setIntrBCallback (void(*p_fptr)(void)) |
Attach a function to call when a interrupt occurs on the GPIOB input ports. | |
template<typename T > | |
void | setIntrACallback (const T *p_tptr, void(T::*p_mptr)(void)) |
Attach a member function to call when a interrupt occurs on the GPIOA input ports. | |
template<typename T > | |
void | setIntrBCallback (const T *p_tptr, void(T::*p_mptr)(void)) |
Attach a member function to call when a rising edge occurs on the input. | |
Protected Member Functions | |
virtual bool | writeRegister (const unsigned char p_registerId, const unsigned char p_value) |
Write value to a register. |
Detailed Description
This class provides simplified I2C access to a Microchip 2MCP28x17 GPIO expender.
V0.0.0.1
Note that if the LPC1768 is powered in 3.3V and Microchip MCP28x17 GPIO expender device could be powered at 3.3V or 5V. In this case, you shall use a bi-directional level shifter for I2C-bus. Please refer to AN97055 (http://ics.nxp.com/support/documents/interface/pdf/an97055.pdf) Microchip MCP28x17 GPIO expender device reference: DS21952B
Note that for I2C details, please visit http://www.datelec.fr/fiches/I2C.htm
Note that this header file include following headers:
- <string>
- <vector>
- <mbed.h>
Definition at line 43 of file MCP23017_I2C.h.
Member Enumeration Documentation
enum InterruptModes [inherited] |
Interrupt modes.
Definition at line 51 of file AbstractGpioExpender.h.
enum PullModes [inherited] |
Pull modes.
Definition at line 59 of file AbstractGpioExpender.h.
Constructor & Destructor Documentation
CMCP23017_I2C | ( | const PinName | p_sda, |
const PinName | p_scl, | ||
const unsigned char | p_address, | ||
const PinName | p_intA = NC , |
||
const PinName | p_intB = NC , |
||
const PinName | p_reset = NC , |
||
const bool | p_internalPullUp = true , |
||
const unsigned int | p_frequency = 400000 |
||
) |
Constructor with Write Protect command pin wired.
Use it to manage the first I2C module on 3.3V or 5V network
- Parameters:
-
p_sda,: MBed pin for SDA p_scl,: MBed pin for SCL p_address,: Device address input: A0, A1, A2 (Pins <1,3>) p_intA,: MBed pin to manage interrupt on GPIOA, default value is NC, not connected p_intB,: MBed pin to manage device reset. If NC, WP is not managed, default value is NC, not connected p_reset,: MBed pin to manage Write Protect input. If NC, WP is not managed, default value is NC, not connected p_internalPullUp,: Set to true to use internal pull-up resistor, default value is true p_frequency,: Frequency of the I2C interface (SCL), default value is 400KHz Example: - If A1 and A2 pins are tired to Vdd and A0 is tired to Vss, address shall '00000110'B
- If A0 and A1 pins are tired to Vss and A2 is tired to Vdd, address shall '00000100'B
Definition at line 51 of file MCP23017_I2C.cpp.
~CMCP23017_I2C | ( | ) | [virtual] |
Destructor.
Definition at line 101 of file MCP23017_I2C.cpp.
Member Function Documentation
int getLastInterruptPinAndValue | ( | unsigned char * | p_gpioId, |
unsigned char * | p_value | ||
) | [virtual] |
Get interrupt information and clear it.
- Parameters:
-
p_gpioId The IO port identifier where the interrupt occured p_value The logic value on the pin port where the interrupt occured
- Returns:
- 0 on success, -1 on wrong parameters and -2 otherwise
Implements AbstractGpioExpender.
Definition at line 404 of file MCP23017_I2C.cpp.
bool Initialize | ( | const unsigned char | p_gpioAFlags = 0x00 , |
const unsigned char | p_gpioBFlags = 0x00 |
||
) |
Initialize the module, configuring the module and starting the clock.
- Parameters:
-
p_gpioAFlags GPIO A port configuration: bit set for input mode, 0 for output mode. Default: ports as outputs p_gpioBFlags GPIO B port configuration: bit set for input mode, 0 for output mode. Default: ports as outputs
- Returns:
- true on success, false otherwise
Definition at line 125 of file MCP23017_I2C.cpp.
const I2C* operator* | ( | ) |
Used to return the unique instance of I2C instance.
Definition at line 116 of file MCP23017_I2C.h.
int read | ( | const unsigned char | p_gpioId, |
unsigned char * | p_value | ||
) | [virtual] |
Read the specific GPIO port pin.
- Parameters:
-
p_gpioId The GPIO port pin to be read p_value The GPIO port pin value
- Returns:
- 0 on success, -1 on wrong parameters and -2 otherwise
Implements AbstractGpioExpender.
Definition at line 450 of file MCP23017_I2C.cpp.
void reset | ( | ) | [virtual] |
void setIntrACallback | ( | const T * | p_tptr, |
void(T::*)(void) | p_mptr | ||
) |
Attach a member function to call when a interrupt occurs on the GPIOA input ports.
Definition at line 219 of file MCP23017_I2C.cpp.
void setIntrACallback | ( | void(*)(void) | p_fptr ) | [virtual] |
Attach a function to call when a interrupt occurs on the GPIOA input ports.
- Parameters:
-
p_fptr The pointer to the "C" callback function
Implements AbstractGpioExpender.
Definition at line 190 of file MCP23017_I2C.cpp.
void setIntrBCallback | ( | void(*)(void) | p_fptr ) | [virtual] |
Attach a function to call when a interrupt occurs on the GPIOB input ports.
- Parameters:
-
p_fptr The pointer to the "C" callback function
Implements AbstractGpioExpender.
Definition at line 204 of file MCP23017_I2C.cpp.
void setIntrBCallback | ( | const T * | p_tptr, |
void(T::*)(void) | p_mptr | ||
) |
Attach a member function to call when a rising edge occurs on the input.
Definition at line 234 of file MCP23017_I2C.cpp.
int setupInterruptPin | ( | const unsigned char | p_gpioId, |
const InterruptModes | p_mode = OnRising |
||
) | [virtual] |
Setup interrupt for a specific IO port.
- Parameters:
-
p_pinId The IO port identifier p_mode The interrupt mode
- Returns:
- 0 on success, -1 on wrong parameters and -2 otherwise
Implements AbstractGpioExpender.
Definition at line 272 of file MCP23017_I2C.cpp.
void setupInterrupts | ( | const unsigned char | mirroring = 0x00 , |
const unsigned char | p_openDrain = 0x00 , |
||
const unsigned char | polarity = 0x00 |
||
) | [virtual] |
Setup device interrupt mechanism.
- Parameters:
-
p_mirroring Set to 0x00 to disable INTA/B mirroring, 0x01 otherwise. Default: 0x00 p_openDrain Set to 0x00 for active driver output, 0x01 for opn drain output. Default: 0x00 p_polarity Set to 0x00 for interrupt active low, 0x01 otherwise. Default: 0x00
Implements AbstractGpioExpender.
Definition at line 246 of file MCP23017_I2C.cpp.
int setupPullPin | ( | const unsigned char | p_gpioId, |
const PullModes | p_mode = PullOff |
||
) | [virtual] |
Setup pull mode for a specific IO port.
- Parameters:
-
p_gpioId The IO port identifier p_mode The interrupt mode
- Returns:
- 0 on success, -1 on wrong parameters and -2 otherwise
Implements AbstractGpioExpender.
Definition at line 347 of file MCP23017_I2C.cpp.
int write | ( | const unsigned char | p_gpioId, |
const unsigned char | p_value | ||
) | [virtual] |
Write value to the specific GPIO port pin.
- Parameters:
-
p_gpioId The GPIO port pin to be written p_value The GPIO port pin value
- Returns:
- 0 on success, -1 on wrong parameters and -2 otherwise
Implements AbstractGpioExpender.
Definition at line 493 of file MCP23017_I2C.cpp.
bool writeRegister | ( | const unsigned char | p_registerId, |
const unsigned char | p_value | ||
) | [protected, virtual] |
Write value to a register.
- Parameters:
-
p_address The register address p_byte The value to write to the register
- Returns:
- 1 on success, false otherwise
Implements AbstractGpioExpender.
Definition at line 601 of file MCP23017_I2C.cpp.
Generated on Tue Jul 12 2022 15:16:48 by
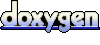