This library provides simplified I2C access to a Microchip MCP23x17 GPIO expender device, including a general interface for any GPIO expender
AbstractGpioExpender Class Reference
This class describes a simplified interface for GPIO expender (e.g. More...
#include <AbstractGpioExpender.h>
Inherited by CMCP23017_I2C.
Public Types | |
enum | InterruptModes |
Interrupt modes. More... | |
enum | PullModes |
Pull modes. More... | |
Public Member Functions | |
virtual void | reset ()=0 |
Reset the device. | |
virtual void | setupInterrupts (const unsigned char p_mirroring=0x00, const unsigned char p_openDrain=0x00, const unsigned char p_polarity=0x00)=0 |
Setup device interrupt mechanism. | |
virtual int | setupInterruptPin (const unsigned char p_gpioId, const InterruptModes p_mode=OnRising)=0 |
Setup interrupt mode for a specific IO port. | |
virtual int | setupPullPin (const unsigned char p_gpioId, const PullModes p_mode=PullOff)=0 |
Setup pull mode for a specific IO port. | |
virtual int | getLastInterruptPinAndValue (unsigned char *p_gpioId, unsigned char *p_value)=0 |
Get interrupt information and clear it. | |
virtual void | setIntrACallback (void(*p_fptr)(void))=0 |
Attach a function to call when a interrupt occurs on the GPIOA input ports. | |
virtual void | setIntrBCallback (void(*p_fptr)(void))=0 |
Attach a function to call when a interrupt occurs on the GPIOB input ports. | |
virtual int | read (const unsigned char p_gpioId, unsigned char *p_value)=0 |
Read the specific GPIO port pin. | |
virtual int | write (const unsigned char p_gpioId, const unsigned char p_value)=0 |
Write value to the specific GPIO port pin. |
Detailed Description
This class describes a simplified interface for GPIO expender (e.g.
Microchip MCP23x17)
Definition at line 47 of file AbstractGpioExpender.h.
Member Enumeration Documentation
enum InterruptModes |
Interrupt modes.
Definition at line 51 of file AbstractGpioExpender.h.
enum PullModes |
Pull modes.
Definition at line 59 of file AbstractGpioExpender.h.
Member Function Documentation
virtual int getLastInterruptPinAndValue | ( | unsigned char * | p_gpioId, |
unsigned char * | p_value | ||
) | [pure virtual] |
Get interrupt information and clear it.
- Parameters:
-
p_gpioId The IO port identifier where the interrupt occured p_value The logic value on the pin port where the interrupt occured
- Returns:
- 0 on success, -1 on wrong parameters and -2 otherwise
Implemented in CMCP23017_I2C.
virtual int read | ( | const unsigned char | p_gpioId, |
unsigned char * | p_value | ||
) | [pure virtual] |
Read the specific GPIO port pin.
- Parameters:
-
p_gpioId The GPIO port pin to be read p_value The GPIO port pin value
- Returns:
- 0 on success, -1 on wrong parameters and -2 otherwise
Implemented in CMCP23017_I2C.
virtual void reset | ( | ) | [pure virtual] |
Reset the device.
Implemented in CMCP23017_I2C.
virtual void setIntrACallback | ( | void(*)(void) | p_fptr ) | [pure virtual] |
Attach a function to call when a interrupt occurs on the GPIOA input ports.
- Parameters:
-
p_fptr The pointer to the "C" callback function
Implemented in CMCP23017_I2C.
virtual void setIntrBCallback | ( | void(*)(void) | p_fptr ) | [pure virtual] |
Attach a function to call when a interrupt occurs on the GPIOB input ports.
- Parameters:
-
p_fptr The pointer to the "C" callback function
Implemented in CMCP23017_I2C.
virtual int setupInterruptPin | ( | const unsigned char | p_gpioId, |
const InterruptModes | p_mode = OnRising |
||
) | [pure virtual] |
Setup interrupt mode for a specific IO port.
- Parameters:
-
p_gpioId The IO port identifier p_mode The interrupt mode
- Returns:
- 0 on success, -1 on wrong parameters and -2 otherwise
Implemented in CMCP23017_I2C.
virtual void setupInterrupts | ( | const unsigned char | p_mirroring = 0x00 , |
const unsigned char | p_openDrain = 0x00 , |
||
const unsigned char | p_polarity = 0x00 |
||
) | [pure virtual] |
Setup device interrupt mechanism.
- Parameters:
-
p_mirroring Set to 0x00 to disable INTA/B mirroring, 0x01 otherwise. Default: 0x00 p_openDrain Set to 0x00 for active driver output, 0x01 for opn drain output. Default: 0x00 p_polarity Set to 0x00 for interrupt active low, 0x01 otherwise. Default: 0x00
Implemented in CMCP23017_I2C.
virtual int setupPullPin | ( | const unsigned char | p_gpioId, |
const PullModes | p_mode = PullOff |
||
) | [pure virtual] |
Setup pull mode for a specific IO port.
- Parameters:
-
p_gpioId The IO port identifier p_mode The interrupt mode
- Returns:
- 0 on success, -1 on wrong parameters and -2 otherwise
Implemented in CMCP23017_I2C.
virtual int write | ( | const unsigned char | p_gpioId, |
const unsigned char | p_value | ||
) | [pure virtual] |
Write value to the specific GPIO port pin.
- Parameters:
-
p_gpioId The GPIO port pin to be written p_value The GPIO port pin value
- Returns:
- 0 on success, -1 on wrong parameters and -2 otherwise
Implemented in CMCP23017_I2C.
Generated on Tue Jul 12 2022 15:16:48 by
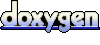