This library provides simplified I2C access to a Microchip MCP23x17 GPIO expender device, including a general interface for any GPIO expender
AbstractGpioExpender.h
00001 /* mbed simplified interface for GPIO expender (e.g. Microchip MCP23x17) 00002 * Copyright (c) 2014-2015 ygarcia, MIT License 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00005 * and associated documentation files (the "Software"), to deal in the Software without restriction, 00006 * including without limitation the rights to use, copy, modify, merge, publish, distribute, 00007 * sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is 00008 * furnished to do so, subject to the following conditions: 00009 * 00010 * The above copyright notice and this permission notice shall be included in all copies or 00011 * substantial portions of the Software. 00012 * 00013 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00014 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00015 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00016 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00017 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00018 */ 00019 #pragma once 00020 00021 #include <list> 00022 00023 #include <mbed.h> 00024 00025 #define GPA0 0 00026 #define GPA1 1 00027 #define GPA2 2 00028 #define GPA3 3 00029 #define GPA4 4 00030 #define GPA5 5 00031 #define GPA6 6 00032 #define GPA7 7 00033 #define GPB0 8 00034 #define GPB1 9 00035 #define GPB2 10 00036 #define GPB3 11 00037 #define GPB4 12 00038 #define GPB5 13 00039 #define GPB6 14 00040 #define GPB7 15 00041 #define GPIO_MAX GPB7 00042 #define GPIO_MED GPB0 00043 #define GPIO_SIZE 8 00044 00045 /** This class describes a simplified interface for GPIO expender (e.g. Microchip MCP23x17) 00046 */ 00047 class AbstractGpioExpender { 00048 public: 00049 /** Interrupt modes 00050 */ 00051 enum InterruptModes { 00052 OnChange = 0x00, 00053 OnRising = 0x01, 00054 OnFalling = 0x03 00055 }; 00056 00057 /** Pull modes 00058 */ 00059 enum PullModes { 00060 PullOff = 0x00, 00061 PullUp = 0x01, 00062 PullDown = 0x03 00063 }; 00064 00065 /** Reset the device 00066 */ 00067 virtual void reset() = 0; 00068 00069 /** Setup device interrupt mechanism 00070 * @param p_mirroring Set to 0x00 to disable INTA/B mirroring, 0x01 otherwise. Default: 0x00 00071 * @param p_openDrain Set to 0x00 for active driver output, 0x01 for opn drain output. Default: 0x00 00072 * @param p_polarity Set to 0x00 for interrupt active low, 0x01 otherwise. Default: 0x00 00073 */ 00074 virtual void setupInterrupts(const unsigned char p_mirroring = 0x00, const unsigned char p_openDrain = 0x00, const unsigned char p_polarity = 0x00) = 0; 00075 00076 /** Setup interrupt mode for a specific IO port 00077 * @param p_gpioId The IO port identifier 00078 * @param p_mode The interrupt mode 00079 * @return 0 on success, -1 on wrong parameters and -2 otherwise 00080 */ 00081 virtual int setupInterruptPin(const unsigned char p_gpioId, const InterruptModes p_mode = OnRising) = 0; 00082 00083 /** Setup pull mode for a specific IO port 00084 * @param p_gpioId The IO port identifier 00085 * @param p_mode The interrupt mode 00086 * @return 0 on success, -1 on wrong parameters and -2 otherwise 00087 */ 00088 virtual int setupPullPin(const unsigned char p_gpioId, const PullModes p_mode = PullOff) = 0; 00089 00090 /** Get interrupt information and clear it 00091 * @param p_gpioId The IO port identifier where the interrupt occured 00092 * @param p_value The logic value on the pin port where the interrupt occured 00093 * @return 0 on success, -1 on wrong parameters and -2 otherwise 00094 */ 00095 virtual int getLastInterruptPinAndValue(unsigned char * p_gpioId, unsigned char * p_value) = 0; 00096 00097 /** Attach a function to call when a interrupt occurs on the GPIOA input ports 00098 * @param p_fptr The pointer to the "C" callback function 00099 */ 00100 virtual void setIntrACallback(void (* p_fptr)(void)) = 0; 00101 00102 /** Attach a function to call when a interrupt occurs on the GPIOB input ports 00103 * @param p_fptr The pointer to the "C" callback function 00104 */ 00105 virtual void setIntrBCallback(void (* p_fptr)(void)) = 0; 00106 00107 /** Read the specific GPIO port pin 00108 * @param p_gpioId The GPIO port pin to be read 00109 * @param p_value The GPIO port pin value 00110 * @return 0 on success, -1 on wrong parameters and -2 otherwise 00111 */ 00112 virtual int read(const unsigned char p_gpioId, unsigned char * p_value) = 0; 00113 00114 /** Write value to the specific GPIO port pin 00115 * @param p_gpioId The GPIO port pin to be written 00116 * @param p_value The GPIO port pin value 00117 * @return 0 on success, -1 on wrong parameters and -2 otherwise 00118 */ 00119 virtual int write(const unsigned char p_gpioId, const unsigned char p_value) = 0; 00120 00121 virtual unsigned char createBus(const std::list<unsigned char> p_lines, const PinMode p_mode = PullNone) = 0; 00122 virtual void deleteBus(const unsigned char p_busId) = 0; 00123 virtual int busRead(const unsigned char p_busId, unsigned short * p_value) = 0; 00124 virtual int busWrite(const unsigned char p_busId, const unsigned short p_value) = 0; 00125 00126 protected: 00127 virtual bool writeRegister(const unsigned char p_registerId, const unsigned char p_value) = 0; 00128 virtual bool readRegister(const unsigned char p_registerId, unsigned char * p_value) = 0; 00129 00130 }; // End of class AbstractGpioExpender
Generated on Tue Jul 12 2022 15:16:48 by
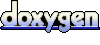