Fixed custom headers and Basic authorization, added support for redirection, functional file download interface can be used for SW updates and more.
Dependents: Sample_HTTPClient Sample_HTTPClient LWM2M_NanoService_Ethernet LWM2M_NanoService_Ethernet ... more
Fork of HTTPClient by
HTTPFile Class Reference
A file handling mechanism - downloads files to a locally accessible file system. More...
#include <HTTPFile.h>
Inherits IHTTPDataIn.
Public Member Functions | |
HTTPFile (char *filename) | |
Instantiate HTTPFile with a specified file on a locally accessible file system. | |
void | close () |
Closes the file, should be called once the http connection is closed. | |
Protected Member Functions | |
virtual void | writeReset () |
Reset stream to its beginning Called by the HTTPClient on each new request. | |
virtual int | write (const char *buf, size_t len) |
Write a piece of data transmitted by the server. | |
virtual void | setDataType (const char *type) |
Set MIME type. | |
virtual void | setIsChunked (bool chunked) |
Determine whether the data is chunked Recovered from Transfer-Encoding header. | |
virtual void | setDataLen (size_t len) |
If the data is not chunked, set its size From Content-Length header. | |
Friends | |
class | HTTPClient |
Detailed Description
A file handling mechanism - downloads files to a locally accessible file system.
Definition at line 8 of file HTTPFile.h.
Constructor & Destructor Documentation
HTTPFile | ( | char * | filename ) |
Instantiate HTTPFile with a specified file on a locally accessible file system.
HTTPFile latest("/local/status.txt"); HTTPErrorCode = http.get("http://server.dom/path/serverstatus.txt", &latest); if (HTTPErrorCode == HTTP_OK) { ... // file successfully downloaded }
- Parameters:
-
filename is the fully qualified filename to create.
Definition at line 17 of file HTTPFile.cpp.
Member Function Documentation
void close | ( | ) |
Closes the file, should be called once the http connection is closed.
Definition at line 23 of file HTTPFile.cpp.
void setDataLen | ( | size_t | len ) | [protected, virtual] |
If the data is not chunked, set its size From Content-Length header.
- Parameters:
-
[in] len defines the size of the non-chunked transfer.
Implements IHTTPDataIn.
Definition at line 65 of file HTTPFile.cpp.
void setDataType | ( | const char * | type ) | [protected, virtual] |
Set MIME type.
- Parameters:
-
[in] type Internet media type from Content-Type header
Implements IHTTPDataIn.
Definition at line 52 of file HTTPFile.cpp.
void setIsChunked | ( | bool | chunked ) | [protected, virtual] |
Determine whether the data is chunked Recovered from Transfer-Encoding header.
- Parameters:
-
[in] chunked indicates the transfer is chunked.
Implements IHTTPDataIn.
Definition at line 60 of file HTTPFile.cpp.
int write | ( | const char * | buf, |
size_t | len | ||
) | [protected, virtual] |
Write a piece of data transmitted by the server.
- Parameters:
-
[in] buf Pointer to the buffer from which to copy the data [in] len Length of the buffer
- Returns:
- number of bytes written.
Implements IHTTPDataIn.
Definition at line 38 of file HTTPFile.cpp.
void writeReset | ( | ) | [protected, virtual] |
Reset stream to its beginning Called by the HTTPClient on each new request.
Implements IHTTPDataIn.
Definition at line 31 of file HTTPFile.cpp.
Generated on Tue Jul 12 2022 17:30:36 by
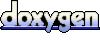