Fixed custom headers and Basic authorization, added support for redirection, functional file download interface can be used for SW updates and more.
Dependents: Sample_HTTPClient Sample_HTTPClient LWM2M_NanoService_Ethernet LWM2M_NanoService_Ethernet ... more
Fork of HTTPClient by
HTTPFile.cpp
00001 #include "HTTPFile.h" 00002 00003 //#define DEBUG "HTfi" 00004 #include <cstdio> 00005 #if (defined(DEBUG) && !defined(TARGET_LPC11U24)) 00006 #define DBG(x, ...) std::printf("[DBG %s %4d] "x"\r\n", DEBUG, __LINE__, ##__VA_ARGS__); 00007 #define WARN(x, ...) std::printf("[WRN %s %4d] "x"\r\n", DEBUG, __LINE__, ##__VA_ARGS__); 00008 #define ERR(x, ...) std::printf("[ERR %s %4d] "x"\r\n", DEBUG, __LINE__, ##__VA_ARGS__); 00009 #define INFO(x, ...) std::printf("[INF %s %4d] "x"\r\n", DEBUG, __LINE__, ##__VA_ARGS__); 00010 #else 00011 #define DBG(x, ...) 00012 #define WARN(x, ...) 00013 #define ERR(x, ...) 00014 #define INFO(x, ...) 00015 #endif 00016 00017 HTTPFile::HTTPFile(char* filename) { 00018 INFO("HTTPFile %s", filename); 00019 file = fopen(filename, "w"); 00020 m_chunked = false; 00021 } 00022 00023 void HTTPFile::close() { 00024 INFO("close()"); 00025 if (file) { 00026 fclose(file); 00027 file = NULL; 00028 } 00029 } 00030 00031 void HTTPFile::writeReset() { 00032 INFO("writeReset()"); 00033 if (file) { 00034 rewind(file); 00035 } 00036 } 00037 00038 int HTTPFile::write(const char* buf, size_t len) { 00039 size_t written; 00040 INFO("write(%d,%s) m_len(%d), chunk %d", len, buf, m_len, m_chunked); 00041 if (file) { 00042 written = fwrite(buf, 1, len, file); 00043 INFO(" writ:%d, ftell: %d", written, ftell(file)); 00044 if ((!m_chunked && ((size_t)ftell(file) >= m_len)) || (m_chunked && !written)) { 00045 INFO("closing"); 00046 close(); 00047 } 00048 } 00049 return len; 00050 } 00051 00052 void HTTPFile::setDataType(const char* type) { 00053 INFO("setDataType(%s)", type); 00054 } 00055 00056 //void HTTPFile::setLocation(const char * location) { 00057 // 00058 //} 00059 00060 void HTTPFile::setIsChunked(bool chunked) { 00061 INFO("setIsChunked(%d)", chunked); 00062 m_chunked = chunked; 00063 } 00064 00065 void HTTPFile::setDataLen(size_t len) { 00066 INFO("setDataLen(%d)", len); 00067 m_len = len; 00068 }
Generated on Tue Jul 12 2022 17:30:35 by
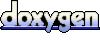