PokittoLib is the library needed for programming the Pokitto DIY game console (www.pokitto.com)
Dependents: YATTT sd_map_test cPong SnowDemo ... more
CHIP: LPC11u6x DMA Controller driver channel specific functions
[CHIP: LPC11u6x DMA Controller driver]
Functions | |
STATIC INLINE void | Chip_DMA_SetupChannelConfig (LPC_DMA_T *pDMA, DMA_CHID_T ch, uint32_t cfg) |
Setup a DMA channel configuration. | |
STATIC INLINE uint32_t | Chip_DMA_GetChannelStatus (LPC_DMA_T *pDMA, DMA_CHID_T ch) |
Returns channel specific status flags. | |
STATIC INLINE void | Chip_DMA_SetupChannelTransfer (LPC_DMA_T *pDMA, DMA_CHID_T ch, uint32_t cfg) |
Setup a DMA channel transfer configuration. | |
void | Chip_DMA_SetTranBits (LPC_DMA_T *pDMA, DMA_CHID_T ch, uint32_t mask) |
Set DMA transfer register interrupt bits (safe) | |
void | Chip_DMA_ClearTranBits (LPC_DMA_T *pDMA, DMA_CHID_T ch, uint32_t mask) |
Clear DMA transfer register interrupt bits (safe) | |
void | Chip_DMA_SetupChannelTransferSize (LPC_DMA_T *pDMA, DMA_CHID_T ch, uint32_t trans) |
Update the transfer size in an existing DMA channel transfer configuration. | |
STATIC INLINE void | Chip_DMA_SetChannelValid (LPC_DMA_T *pDMA, DMA_CHID_T ch) |
Sets a DMA channel configuration as valid. | |
STATIC INLINE void | Chip_DMA_SetChannelInValid (LPC_DMA_T *pDMA, DMA_CHID_T ch) |
Sets a DMA channel configuration as invalid. | |
STATIC INLINE void | Chip_DMA_SWTriggerChannel (LPC_DMA_T *pDMA, DMA_CHID_T ch) |
Performs a software trigger of the DMA channel. | |
bool | Chip_DMA_SetupTranChannel (LPC_DMA_T *pDMA, DMA_CHID_T ch, DMA_CHDESC_T *desc) |
Sets up a DMA channel with the passed DMA transfer descriptor. |
Function Documentation
void Chip_DMA_ClearTranBits | ( | LPC_DMA_T * | pDMA, |
DMA_CHID_T | ch, | ||
uint32_t | mask | ||
) |
Clear DMA transfer register interrupt bits (safe)
- Parameters:
-
pDMA : The base of DMA controller on the chip ch : DMA channel ID mask : Bits to clear
- Returns:
- Nothing
- Note:
- This function safely clears bits in the DMA channel specific XFERCFG register.
Definition at line 95 of file dma_11u6x.c.
STATIC INLINE uint32_t Chip_DMA_GetChannelStatus | ( | LPC_DMA_T * | pDMA, |
DMA_CHID_T | ch | ||
) |
Returns channel specific status flags.
- Parameters:
-
pDMA : The base of DMA controller on the chip ch : DMA channel ID
- Returns:
- AN Or'ed value of DMA_CTLSTAT_VALIDPENDING and DMA_CTLSTAT_TRIG
Definition at line 556 of file dma_11u6x.h.
STATIC INLINE void Chip_DMA_SetChannelInValid | ( | LPC_DMA_T * | pDMA, |
DMA_CHID_T | ch | ||
) |
Sets a DMA channel configuration as invalid.
- Parameters:
-
pDMA : The base of DMA controller on the chip ch : DMA channel ID
- Returns:
- Nothing
Definition at line 651 of file dma_11u6x.h.
STATIC INLINE void Chip_DMA_SetChannelValid | ( | LPC_DMA_T * | pDMA, |
DMA_CHID_T | ch | ||
) |
Sets a DMA channel configuration as valid.
- Parameters:
-
pDMA : The base of DMA controller on the chip ch : DMA channel ID
- Returns:
- Nothing
Definition at line 640 of file dma_11u6x.h.
void Chip_DMA_SetTranBits | ( | LPC_DMA_T * | pDMA, |
DMA_CHID_T | ch, | ||
uint32_t | mask | ||
) |
Set DMA transfer register interrupt bits (safe)
- Parameters:
-
pDMA : The base of DMA controller on the chip ch : DMA channel ID mask : Bits to set
- Returns:
- Nothing
- Note:
- This function safely sets bits in the DMA channel specific XFERCFG register.
STATIC INLINE void Chip_DMA_SetupChannelConfig | ( | LPC_DMA_T * | pDMA, |
DMA_CHID_T | ch, | ||
uint32_t | cfg | ||
) |
Setup a DMA channel configuration.
- Parameters:
-
pDMA : The base of DMA controller on the chip ch : DMA channel ID cfg : An Or'ed value of DMA_CFG_* values that define the channel's configuration
- Returns:
- Nothing
- Note:
- This function sets up all configurable options for the DMA channel. These options are usually set once for a channel and then unchanged.
The following example show how to configure the channel for peripheral DMA requests, burst transfer size of 1 (in 'transfers', not bytes), continuous reading of the same source address, incrementing destination address, and highest channel priority.
Example: Chip_DMA_SetupChannelConfig(pDMA, SSP0_RX_DMA, (DMA_CFG_PERIPHREQEN | DMA_CFG_TRIGBURST_BURST | DMA_CFG_BURSTPOWER_1 | DMA_CFG_SRCBURSTWRAP | DMA_CFG_CHPRIORITY(0)));
The following example show how to configure the channel for an external trigger from the imput mux with low edge polarity, a burst transfer size of 8, incrementing source and destination addresses, and lowest channel priority.
Example: Chip_DMA_SetupChannelConfig(pDMA, DMA_CH14, (DMA_CFG_HWTRIGEN | DMA_CFG_TRIGPOL_LOW | DMA_CFG_TRIGTYPE_EDGE | DMA_CFG_TRIGBURST_BURST | DMA_CFG_BURSTPOWER_8 | DMA_CFG_CHPRIORITY(3)));
For non-peripheral DMA triggering (DMA_CFG_HWTRIGEN definition), use the DMA input mux functions to configure the DMA trigger source for a DMA channel.
Definition at line 541 of file dma_11u6x.h.
STATIC INLINE void Chip_DMA_SetupChannelTransfer | ( | LPC_DMA_T * | pDMA, |
DMA_CHID_T | ch, | ||
uint32_t | cfg | ||
) |
Setup a DMA channel transfer configuration.
- Parameters:
-
pDMA : The base of DMA controller on the chip ch : DMA channel ID cfg : An Or'ed value of DMA_XFERCFG_* values that define the channel's transfer configuration
- Returns:
- Nothing
- Note:
- This function sets up the transfer configuration for the DMA channel.
The following example show how to configure the channel's transfer for multiple transfer descriptors (ie, ping-pong), interrupt 'A' trigger on transfer descriptor completion, 128 byte size transfers, and source and destination address increment.
Example: Chip_DMA_SetupChannelTransfer(pDMA, SSP0_RX_DMA, (DMA_XFERCFG_CFGVALID | DMA_XFERCFG_RELOAD | DMA_XFERCFG_SETINTA | DMA_XFERCFG_WIDTH_8 | DMA_XFERCFG_SRCINC_1 | DMA_XFERCFG_DSTINC_1 | DMA_XFERCFG_XFERCOUNT(128)));
Definition at line 598 of file dma_11u6x.h.
void Chip_DMA_SetupChannelTransferSize | ( | LPC_DMA_T * | pDMA, |
DMA_CHID_T | ch, | ||
uint32_t | trans | ||
) |
Update the transfer size in an existing DMA channel transfer configuration.
- Parameters:
-
pDMA : The base of DMA controller on the chip ch : DMA channel ID trans : Number of transfers to update the transfer configuration to (1 - 1023)
- Returns:
- Nothing
Definition at line 107 of file dma_11u6x.c.
bool Chip_DMA_SetupTranChannel | ( | LPC_DMA_T * | pDMA, |
DMA_CHID_T | ch, | ||
DMA_CHDESC_T * | desc | ||
) |
Sets up a DMA channel with the passed DMA transfer descriptor.
- Parameters:
-
pDMA : The base of DMA controller on the chip ch : DMA channel ID desc : Pointer to DMA transfer descriptor
- Returns:
- false if the DMA channel was active, otherwise true
- Note:
- This function will set the DMA descriptor in the SRAM table to the the passed descriptor. This function is only meant to be used when the DMA channel is not active and can be used to setup the initial transfer for a linked list or ping-pong buffer or just a single transfer without a next descriptor.
If using this function to write the initial transfer descriptor in a linked list or ping-pong buffer configuration, it should contain a non-NULL 'next' field pointer.
Definition at line 114 of file dma_11u6x.c.
STATIC INLINE void Chip_DMA_SWTriggerChannel | ( | LPC_DMA_T * | pDMA, |
DMA_CHID_T | ch | ||
) |
Performs a software trigger of the DMA channel.
- Parameters:
-
pDMA : The base of DMA controller on the chip ch : DMA channel ID
- Returns:
- Nothing
Definition at line 662 of file dma_11u6x.h.
Generated on Tue Jul 12 2022 11:20:46 by
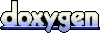