A class that converts byte streams into MIDI messages, and stores them in a FIFO. This is useful if you wish to read MIDI messages via polling instead of interrupts. The class supports every type of MIDI message, and System Realtime messages can be interleaved with regular ones.
MyBuffer< T > Class Template Reference
A templated software ring buffer. More...
#include <MyBuffer.h>
Public Member Functions | |
MyBuffer (uint32_t size=0x100) | |
Create a Buffer and allocate memory for it. | |
uint32_t | getSize () |
Get the size of the ring buffer. | |
~MyBuffer () | |
Destry a Buffer and release it's allocated memory. | |
void | put (T data) |
Add a data element into the buffer. | |
T | get (void) |
Remove a data element from the buffer. | |
T * | head (void) |
Get the address to the head of the buffer. | |
void | clear (void) |
Reset the buffer to 0. | |
uint32_t | available (void) |
Determine if anything is readable in the buffer. | |
MyBuffer & | operator= (T data) |
Overloaded operator for writing to the buffer. | |
operator int (void) | |
Overloaded operator for reading from the buffer. |
Detailed Description
template<typename T>
class MyBuffer< T >
A templated software ring buffer.
Example:
#include "mbed.h" #include "MyBuffer.h" MyBuffer <char> buf; int main() { buf = 'a'; buf.put('b'); char *head = buf.head(); puts(head); char whats_in_there[2] = {0}; int pos = 0; while(buf.available()) { whats_in_there[pos++] = buf; } printf("%c %c\n", whats_in_there[0], whats_in_there[1]); buf.clear(); error("done\n\n\n"); }
Definition at line 61 of file MyBuffer.h.
Constructor & Destructor Documentation
MyBuffer | ( | uint32_t | size = 0x100 ) |
Create a Buffer and allocate memory for it.
- Parameters:
-
size The size of the buffer
Definition at line 27 of file MyBuffer.cpp.
~MyBuffer | ( | ) |
Destry a Buffer and release it's allocated memory.
Definition at line 37 of file MyBuffer.cpp.
Member Function Documentation
uint32_t available | ( | void | ) |
Determine if anything is readable in the buffer.
- Returns:
- 1 if something can be read, 0 otherwise
Definition at line 159 of file MyBuffer.h.
void clear | ( | void | ) |
Reset the buffer to 0.
Useful if using head() to parse packeted data
Definition at line 51 of file MyBuffer.cpp.
T get | ( | void | ) |
Remove a data element from the buffer.
- Returns:
- Pull the oldest element from the buffer
Definition at line 142 of file MyBuffer.h.
uint32_t getSize | ( | ) |
Get the size of the ring buffer.
- Returns:
- the size of the ring buffer
Definition at line 45 of file MyBuffer.cpp.
T * head | ( | void | ) |
Get the address to the head of the buffer.
- Returns:
- The address of element 0 in the buffer
Definition at line 151 of file MyBuffer.h.
operator int | ( | void | ) |
Overloaded operator for reading from the buffer.
- Returns:
- Pull the oldest element from the buffer
Definition at line 123 of file MyBuffer.h.
MyBuffer& operator= | ( | T | data ) |
Overloaded operator for writing to the buffer.
- Parameters:
-
data Something to put in the buffer
- Returns:
Definition at line 114 of file MyBuffer.h.
void put | ( | T | data ) |
Add a data element into the buffer.
- Parameters:
-
data Something to add to the buffer
Definition at line 133 of file MyBuffer.h.
Generated on Tue Jul 12 2022 21:41:49 by
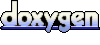