A class that converts byte streams into MIDI messages, and stores them in a FIFO. This is useful if you wish to read MIDI messages via polling instead of interrupts. The class supports every type of MIDI message, and System Realtime messages can be interleaved with regular ones.
MyBuffer.h
00001 00002 /** 00003 * @file Buffer.h 00004 * @brief Software Buffer - Templated Ring Buffer for most data types 00005 * @author sam grove 00006 * @version 1.0 00007 * @see 00008 * 00009 * Copyright (c) 2013 00010 * 00011 * Licensed under the Apache License, Version 2.0 (the "License"); 00012 * you may not use this file except in compliance with the License. 00013 * You may obtain a copy of the License at 00014 * 00015 * http://www.apache.org/licenses/LICENSE-2.0 00016 * 00017 * Unless required by applicable law or agreed to in writing, software 00018 * distributed under the License is distributed on an "AS IS" BASIS, 00019 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00020 * See the License for the specific language governing permissions and 00021 * limitations under the License. 00022 */ 00023 00024 #ifndef MYBUFFER_H 00025 #define MYBUFFER_H 00026 00027 #include <stdint.h> 00028 #include <string.h> 00029 00030 /** A templated software ring buffer 00031 * 00032 * Example: 00033 * @code 00034 * #include "mbed.h" 00035 * #include "MyBuffer.h" 00036 * 00037 * MyBuffer <char> buf; 00038 * 00039 * int main() 00040 * { 00041 * buf = 'a'; 00042 * buf.put('b'); 00043 * char *head = buf.head(); 00044 * puts(head); 00045 * 00046 * char whats_in_there[2] = {0}; 00047 * int pos = 0; 00048 * 00049 * while(buf.available()) 00050 * { 00051 * whats_in_there[pos++] = buf; 00052 * } 00053 * printf("%c %c\n", whats_in_there[0], whats_in_there[1]); 00054 * buf.clear(); 00055 * error("done\n\n\n"); 00056 * } 00057 * @endcode 00058 */ 00059 00060 template <typename T> 00061 class MyBuffer 00062 { 00063 private: 00064 T *_buf; 00065 volatile uint32_t _wloc; 00066 volatile uint32_t _rloc; 00067 uint32_t _size; 00068 00069 public: 00070 /** Create a Buffer and allocate memory for it 00071 * @param size The size of the buffer 00072 */ 00073 MyBuffer(uint32_t size = 0x100); 00074 00075 /** Get the size of the ring buffer 00076 * @return the size of the ring buffer 00077 */ 00078 uint32_t getSize(); 00079 00080 /** Destry a Buffer and release it's allocated memory 00081 */ 00082 ~MyBuffer(); 00083 00084 /** Add a data element into the buffer 00085 * @param data Something to add to the buffer 00086 */ 00087 void put(T data); 00088 00089 /** Remove a data element from the buffer 00090 * @return Pull the oldest element from the buffer 00091 */ 00092 T get(void); 00093 00094 /** Get the address to the head of the buffer 00095 * @return The address of element 0 in the buffer 00096 */ 00097 T *head(void); 00098 00099 /** Reset the buffer to 0. Useful if using head() to parse packeted data 00100 */ 00101 void clear(void); 00102 00103 /** Determine if anything is readable in the buffer 00104 * @return 1 if something can be read, 0 otherwise 00105 */ 00106 uint32_t available(void); 00107 00108 uint32_t size(); 00109 00110 /** Overloaded operator for writing to the buffer 00111 * @param data Something to put in the buffer 00112 * @return 00113 */ 00114 MyBuffer &operator= (T data) 00115 { 00116 put(data); 00117 return *this; 00118 } 00119 00120 /** Overloaded operator for reading from the buffer 00121 * @return Pull the oldest element from the buffer 00122 */ 00123 operator int(void) 00124 { 00125 return get(); 00126 } 00127 00128 uint32_t peek(char c); 00129 00130 }; 00131 00132 template <class T> 00133 inline void MyBuffer<T>::put(T data) 00134 { 00135 _buf[_wloc++] = data; 00136 _wloc %= (_size-1); 00137 00138 return; 00139 } 00140 00141 template <class T> 00142 inline T MyBuffer<T>::get(void) 00143 { 00144 T data_pos = _buf[_rloc++]; 00145 _rloc %= (_size-1); 00146 00147 return data_pos; 00148 } 00149 00150 template <class T> 00151 inline T *MyBuffer<T>::head(void) 00152 { 00153 T *data_pos = &_buf[0]; 00154 00155 return data_pos; 00156 } 00157 00158 template <class T> 00159 inline uint32_t MyBuffer<T>::available(void) 00160 { 00161 return (_wloc == _rloc) ? 0 : 1; 00162 } 00163 00164 template <class T> 00165 inline uint32_t MyBuffer<T>::size(void) 00166 { 00167 return _wloc - _rloc; 00168 } 00169 00170 #endif 00171
Generated on Tue Jul 12 2022 21:41:49 by
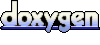