A class that converts byte streams into MIDI messages, and stores them in a FIFO. This is useful if you wish to read MIDI messages via polling instead of interrupts. The class supports every type of MIDI message, and System Realtime messages can be interleaved with regular ones.
MyBuffer.cpp
00001 00002 /** 00003 * @file Buffer.cpp 00004 * @brief Software Buffer - Templated Ring Buffer for most data types 00005 * @author sam grove 00006 * @version 1.0 00007 * @see 00008 * 00009 * Copyright (c) 2013 00010 * 00011 * Licensed under the Apache License, Version 2.0 (the "License"); 00012 * you may not use this file except in compliance with the License. 00013 * You may obtain a copy of the License at 00014 * 00015 * http://www.apache.org/licenses/LICENSE-2.0 00016 * 00017 * Unless required by applicable law or agreed to in writing, software 00018 * distributed under the License is distributed on an "AS IS" BASIS, 00019 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00020 * See the License for the specific language governing permissions and 00021 * limitations under the License. 00022 */ 00023 00024 #include "MyBuffer.h" 00025 00026 template <class T> 00027 MyBuffer<T>::MyBuffer(uint32_t size) 00028 { 00029 _buf = new T [size]; 00030 _size = size; 00031 clear(); 00032 00033 return; 00034 } 00035 00036 template <class T> 00037 MyBuffer<T>::~MyBuffer() 00038 { 00039 delete [] _buf; 00040 00041 return; 00042 } 00043 00044 template <class T> 00045 uint32_t MyBuffer<T>::getSize() 00046 { 00047 return this->_size; 00048 } 00049 00050 template <class T> 00051 void MyBuffer<T>::clear(void) 00052 { 00053 _wloc = 0; 00054 _rloc = 0; 00055 memset(_buf, 0, _size); 00056 00057 return; 00058 } 00059 00060 template <class T> 00061 uint32_t MyBuffer<T>::peek(char c) 00062 { 00063 return 1; 00064 } 00065 00066 // make the linker aware of some possible types 00067 template class MyBuffer<uint8_t> ; 00068 template class MyBuffer<int8_t>; 00069 template class MyBuffer<uint16_t>; 00070 template class MyBuffer<int16_t>; 00071 template class MyBuffer<uint32_t>; 00072 template class MyBuffer<int32_t>; 00073 template class MyBuffer<uint64_t>; 00074 template class MyBuffer<int64_t>; 00075 template class MyBuffer<char>; 00076 template class MyBuffer<wchar_t>;
Generated on Tue Jul 12 2022 21:41:49 by
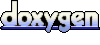