Interface library for the nRF24L01+ chip (specifically the Transceiver nRF24L01+ Module with Chip Antenna from SparkFun, talking to their Nordic Serial Interface Board, but should work with any nRF24L01+).
Dependents: nRF24L01P_Hello_World NerfGun_nRF24L01P_RX NerfGun_nRF24L01P_TX idd_hw3_AngieWangAntonioDeLimaFernandesDanielLim_BladeSymphony ... more
nRF24L01P.h
00001 /** 00002 * @file nRF24L01P.h 00003 * 00004 * @author Owen Edwards 00005 * 00006 * @section LICENSE 00007 * 00008 * Copyright (c) 2010 Owen Edwards 00009 * 00010 * This program is free software: you can redistribute it and/or modify 00011 * it under the terms of the GNU General Public License as published by 00012 * the Free Software Foundation, either version 3 of the License, or 00013 * (at your option) any later version. 00014 * 00015 * This program is distributed in the hope that it will be useful, 00016 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00017 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00018 * GNU General Public License for more details. 00019 * 00020 * You should have received a copy of the GNU General Public License 00021 * along with this program. If not, see <http://www.gnu.org/licenses/>. 00022 * 00023 * The above copyright notice and this permission notice shall be included in 00024 * all copies or substantial portions of the Software. 00025 * 00026 * @section DESCRIPTION 00027 * 00028 * nRF24L01+ Single Chip 2.4GHz Transceiver from Nordic Semiconductor. 00029 * 00030 * Datasheet: 00031 * 00032 * http://www.nordicsemi.no/files/Product/data_sheet/nRF24L01P_Product_Specification_1_0.pdf 00033 */ 00034 00035 #ifndef __NRF24L01P_H__ 00036 #define __NRF24L01P_H__ 00037 00038 /** 00039 * Includes 00040 */ 00041 #include "mbed.h" 00042 00043 /** 00044 * Defines 00045 */ 00046 #define NRF24L01P_TX_PWR_ZERO_DB 0 00047 #define NRF24L01P_TX_PWR_MINUS_6_DB -6 00048 #define NRF24L01P_TX_PWR_MINUS_12_DB -12 00049 #define NRF24L01P_TX_PWR_MINUS_18_DB -18 00050 00051 #define NRF24L01P_DATARATE_250_KBPS 250 00052 #define NRF24L01P_DATARATE_1_MBPS 1000 00053 #define NRF24L01P_DATARATE_2_MBPS 2000 00054 00055 #define NRF24L01P_CRC_NONE 0 00056 #define NRF24L01P_CRC_8_BIT 8 00057 #define NRF24L01P_CRC_16_BIT 16 00058 00059 #define NRF24L01P_MIN_RF_FREQUENCY 2400 00060 #define NRF24L01P_MAX_RF_FREQUENCY 2525 00061 00062 #define NRF24L01P_PIPE_P0 0 00063 #define NRF24L01P_PIPE_P1 1 00064 #define NRF24L01P_PIPE_P2 2 00065 #define NRF24L01P_PIPE_P3 3 00066 #define NRF24L01P_PIPE_P4 4 00067 #define NRF24L01P_PIPE_P5 5 00068 00069 /** 00070 * Default setup for the nRF24L01+, based on the Sparkfun "Nordic Serial Interface Board" 00071 * for evaluation (http://www.sparkfun.com/products/9019) 00072 */ 00073 #define DEFAULT_NRF24L01P_ADDRESS ((unsigned long long) 0xE7E7E7E7E7 ) 00074 #define DEFAULT_NRF24L01P_ADDRESS_WIDTH 5 00075 #define DEFAULT_NRF24L01P_CRC NRF24L01P_CRC_8_BIT 00076 #define DEFAULT_NRF24L01P_RF_FREQUENCY (NRF24L01P_MIN_RF_FREQUENCY + 2) 00077 #define DEFAULT_NRF24L01P_DATARATE NRF24L01P_DATARATE_1_MBPS 00078 #define DEFAULT_NRF24L01P_TX_PWR NRF24L01P_TX_PWR_ZERO_DB 00079 #define DEFAULT_NRF24L01P_TRANSFER_SIZE 4 00080 00081 /** 00082 * nRF24L01+ Single Chip 2.4GHz Transceiver from Nordic Semiconductor. 00083 */ 00084 class nRF24L01P { 00085 00086 public: 00087 00088 /** 00089 * Constructor. 00090 * 00091 * @param mosi mbed pin to use for MOSI line of SPI interface. 00092 * @param miso mbed pin to use for MISO line of SPI interface. 00093 * @param sck mbed pin to use for SCK line of SPI interface. 00094 * @param csn mbed pin to use for not chip select line of SPI interface. 00095 * @param ce mbed pin to use for the chip enable line. 00096 * @param irq mbed pin to use for the interrupt request line. 00097 */ 00098 nRF24L01P(PinName mosi, PinName miso, PinName sck, PinName csn, PinName ce, PinName irq = NC); 00099 00100 /** 00101 * Set the RF frequency. 00102 * 00103 * @param frequency the frequency of RF transmission in MHz (2400..2525). 00104 */ 00105 void setRfFrequency(int frequency = DEFAULT_NRF24L01P_RF_FREQUENCY); 00106 00107 /** 00108 * Get the RF frequency. 00109 * 00110 * @return the frequency of RF transmission in MHz (2400..2525). 00111 */ 00112 int getRfFrequency(void); 00113 00114 /** 00115 * Set the RF output power. 00116 * 00117 * @param power the RF output power in dBm (0, -6, -12 or -18). 00118 */ 00119 void setRfOutputPower(int power = DEFAULT_NRF24L01P_TX_PWR); 00120 00121 /** 00122 * Get the RF output power. 00123 * 00124 * @return the RF output power in dBm (0, -6, -12 or -18). 00125 */ 00126 int getRfOutputPower(void); 00127 00128 /** 00129 * Set the Air data rate. 00130 * 00131 * @param rate the air data rate in kbps (250, 1M or 2M). 00132 */ 00133 void setAirDataRate(int rate = DEFAULT_NRF24L01P_DATARATE); 00134 00135 /** 00136 * Get the Air data rate. 00137 * 00138 * @return the air data rate in kbps (250, 1M or 2M). 00139 */ 00140 int getAirDataRate(void); 00141 00142 /** 00143 * Set the CRC width. 00144 * 00145 * @param width the number of bits for the CRC (0, 8 or 16). 00146 */ 00147 void setCrcWidth(int width = DEFAULT_NRF24L01P_CRC); 00148 00149 /** 00150 * Get the CRC width. 00151 * 00152 * @return the number of bits for the CRC (0, 8 or 16). 00153 */ 00154 int getCrcWidth(void); 00155 00156 /** 00157 * Set the Receive address. 00158 * 00159 * @param address address associated with the particular pipe 00160 * @param width width of the address in bytes (3..5) 00161 * @param pipe pipe to associate the address with (0..5, default 0) 00162 * 00163 * Note that Pipes 0 & 1 have 3, 4 or 5 byte addresses, 00164 * while Pipes 2..5 only use the lowest byte (bits 7..0) of the 00165 * address provided here, and use 2, 3 or 4 bytes from Pipe 1's address. 00166 * The width parameter is ignored for Pipes 2..5. 00167 */ 00168 void setRxAddress(unsigned long long address = DEFAULT_NRF24L01P_ADDRESS, int width = DEFAULT_NRF24L01P_ADDRESS_WIDTH, int pipe = NRF24L01P_PIPE_P0); 00169 00170 void setRxAddress(unsigned long msb_address, unsigned long lsb_address, int width, int pipe = NRF24L01P_PIPE_P0); 00171 00172 /** 00173 * Set the Transmit address. 00174 * 00175 * @param address address for transmission 00176 * @param width width of the address in bytes (3..5) 00177 * 00178 * Note that the address width is shared with the Receive pipes, 00179 * so a change to that address width affect transmissions. 00180 */ 00181 void setTxAddress(unsigned long long address = DEFAULT_NRF24L01P_ADDRESS, int width = DEFAULT_NRF24L01P_ADDRESS_WIDTH); 00182 00183 void setTxAddress(unsigned long msb_address, unsigned long lsb_address, int width); 00184 00185 /** 00186 * Get the Receive address. 00187 * 00188 * @param pipe pipe to get the address from (0..5, default 0) 00189 * @return the address associated with the particular pipe 00190 */ 00191 unsigned long long getRxAddress(int pipe = NRF24L01P_PIPE_P0); 00192 00193 /** 00194 * Get the Transmit address. 00195 * 00196 * @return address address for transmission 00197 */ 00198 unsigned long long getTxAddress(void); 00199 00200 /** 00201 * Set the transfer size. 00202 * 00203 * @param size the size of the transfer, in bytes (1..32) 00204 * @param pipe pipe for the transfer (0..5, default 0) 00205 */ 00206 void setTransferSize(int size = DEFAULT_NRF24L01P_TRANSFER_SIZE, int pipe = NRF24L01P_PIPE_P0); 00207 00208 /** 00209 * Get the transfer size. 00210 * 00211 * @return the size of the transfer, in bytes (1..32). 00212 */ 00213 int getTransferSize(int pipe = NRF24L01P_PIPE_P0); 00214 00215 00216 /** 00217 * Get the RPD (Received Power Detector) state. 00218 * 00219 * @return true if the received power exceeded -64dBm 00220 */ 00221 bool getRPD(void); 00222 00223 /** 00224 * Put the nRF24L01+ into Receive mode 00225 */ 00226 void setReceiveMode(void); 00227 00228 /** 00229 * Put the nRF24L01+ into Transmit mode 00230 */ 00231 void setTransmitMode(void); 00232 00233 /** 00234 * Power up the nRF24L01+ into Standby mode 00235 */ 00236 void powerUp(void); 00237 00238 /** 00239 * Power down the nRF24L01+ into Power Down mode 00240 */ 00241 void powerDown(void); 00242 00243 /** 00244 * Enable the nRF24L01+ to Receive or Transmit (using the CE pin) 00245 */ 00246 void enable(void); 00247 00248 /** 00249 * Disable the nRF24L01+ to Receive or Transmit (using the CE pin) 00250 */ 00251 void disable(void); 00252 00253 /** 00254 * Transmit data 00255 * 00256 * @param pipe is ignored (included for consistency with file write routine) 00257 * @param data pointer to an array of bytes to write 00258 * @param count the number of bytes to send (1..32) 00259 * @return the number of bytes actually written, or -1 for an error 00260 */ 00261 int write(int pipe, char *data, int count); 00262 00263 /** 00264 * Receive data 00265 * 00266 * @param pipe the receive pipe to get data from 00267 * @param data pointer to an array of bytes to store the received data 00268 * @param count the number of bytes to receive (1..32) 00269 * @return the number of bytes actually received, 0 if none are received, or -1 for an error 00270 */ 00271 int read(int pipe, char *data, int count); 00272 00273 /** 00274 * Determine if there is data available to read 00275 * 00276 * @param pipe the receive pipe to check for data 00277 * @return true if the is data waiting in the given pipe 00278 */ 00279 bool readable(int pipe = NRF24L01P_PIPE_P0); 00280 00281 /** 00282 * Disable all receive pipes 00283 * 00284 * Note: receive pipes are enabled when their address is set. 00285 */ 00286 void disableAllRxPipes(void); 00287 00288 /** 00289 * Disable AutoAcknowledge function 00290 */ 00291 void disableAutoAcknowledge(void); 00292 00293 /** 00294 * Enable AutoAcknowledge function 00295 * 00296 * @param pipe the receive pipe 00297 */ 00298 void enableAutoAcknowledge(int pipe = NRF24L01P_PIPE_P0); 00299 00300 /** 00301 * Disable AutoRetransmit function 00302 */ 00303 void disableAutoRetransmit(void); 00304 00305 /** 00306 * Enable AutoRetransmit function 00307 * 00308 * @param delay the delay between restransmits, in uS (250uS..4000uS) 00309 * @param count number of retransmits before generating an error (1..15) 00310 */ 00311 void enableAutoRetransmit(int delay, int count); 00312 00313 private: 00314 00315 /** 00316 * Get the contents of an addressable register. 00317 * 00318 * @param regAddress address of the register 00319 * @return the contents of the register 00320 */ 00321 int getRegister(int regAddress); 00322 00323 /** 00324 * Set the contents of an addressable register. 00325 * 00326 * @param regAddress address of the register 00327 * @param regData data to write to the register 00328 */ 00329 void setRegister(int regAddress, int regData); 00330 00331 /** 00332 * Get the contents of the status register. 00333 * 00334 * @return the contents of the status register 00335 */ 00336 int getStatusRegister(void); 00337 00338 SPI spi_; 00339 DigitalOut nCS_; 00340 DigitalOut ce_; 00341 InterruptIn nIRQ_; 00342 00343 int mode; 00344 00345 }; 00346 00347 #endif /* __NRF24L01P_H__ */
Generated on Tue Jul 12 2022 17:37:08 by
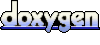