Added support for WNC M14A2A Cellular LTE Data Module.
Dependencies: WNC14A2AInterface
Dependents: http-example-wnc http-example-wnc-modified
MCR20Drv.c
00001 /*! 00002 * Copyright (c) 2015, Freescale Semiconductor, Inc. 00003 * All rights reserved. 00004 * 00005 * \file MCR20Drv.c 00006 * 00007 * Redistribution and use in source and binary forms, with or without modification, 00008 * are permitted provided that the following conditions are met: 00009 * 00010 * o Redistributions of source code must retain the above copyright notice, this list 00011 * of conditions and the following disclaimer. 00012 * 00013 * o Redistributions in binary form must reproduce the above copyright notice, this 00014 * list of conditions and the following disclaimer in the documentation and/or 00015 * other materials provided with the distribution. 00016 * 00017 * o Neither the name of Freescale Semiconductor, Inc. nor the names of its 00018 * contributors may be used to endorse or promote products derived from this 00019 * software without specific prior written permission. 00020 * 00021 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 00022 * ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 00023 * WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00024 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR 00025 * ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES 00026 * (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; 00027 * LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON 00028 * ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT 00029 * (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS 00030 * SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00031 */ 00032 00033 00034 /***************************************************************************** 00035 * INCLUDED HEADERS * 00036 *---------------------------------------------------------------------------* 00037 * Add to this section all the headers that this module needs to include. * 00038 *---------------------------------------------------------------------------* 00039 *****************************************************************************/ 00040 00041 #include "platform/arm_hal_interrupt.h" 00042 #include "MCR20Drv.h " 00043 #include "MCR20Reg.h" 00044 #include "XcvrSpi.h " 00045 00046 00047 /***************************************************************************** 00048 * PRIVATE VARIABLES * 00049 *---------------------------------------------------------------------------* 00050 * Add to this section all the variables and constants that have local * 00051 * (file) scope. * 00052 * Each of this declarations shall be preceded by the 'static' keyword. * 00053 * These variables / constants cannot be accessed outside this module. * 00054 *---------------------------------------------------------------------------* 00055 *****************************************************************************/ 00056 uint32_t mPhyIrqDisableCnt = 1; 00057 00058 /***************************************************************************** 00059 * PUBLIC VARIABLES * 00060 *---------------------------------------------------------------------------* 00061 * Add to this section all the variables and constants that have global * 00062 * (project) scope. * 00063 * These variables / constants can be accessed outside this module. * 00064 * These variables / constants shall be preceded by the 'extern' keyword in * 00065 * the interface header. * 00066 *---------------------------------------------------------------------------* 00067 *****************************************************************************/ 00068 00069 /***************************************************************************** 00070 * PRIVATE FUNCTIONS PROTOTYPES * 00071 *---------------------------------------------------------------------------* 00072 * Add to this section all the functions prototypes that have local (file) * 00073 * scope. * 00074 * These functions cannot be accessed outside this module. * 00075 * These declarations shall be preceded by the 'static' keyword. * 00076 *---------------------------------------------------------------------------* 00077 *****************************************************************************/ 00078 00079 /***************************************************************************** 00080 * PRIVATE FUNCTIONS * 00081 *---------------------------------------------------------------------------* 00082 * Add to this section all the functions that have local (file) scope. * 00083 * These functions cannot be accessed outside this module. * 00084 * These definitions shall be preceded by the 'static' keyword. * 00085 *---------------------------------------------------------------------------* 00086 *****************************************************************************/ 00087 00088 00089 /***************************************************************************** 00090 * PUBLIC FUNCTIONS * 00091 *---------------------------------------------------------------------------* 00092 * Add to this section all the functions that have global (project) scope. * 00093 * These functions can be accessed outside this module. * 00094 * These functions shall have their declarations (prototypes) within the * 00095 * interface header file and shall be preceded by the 'extern' keyword. * 00096 *---------------------------------------------------------------------------* 00097 *****************************************************************************/ 00098 00099 /*--------------------------------------------------------------------------- 00100 * Name: MCR20Drv_Init 00101 * Description: - 00102 * Parameters: - 00103 * Return: - 00104 *---------------------------------------------------------------------------*/ 00105 void MCR20Drv_Init 00106 ( 00107 void 00108 ) 00109 { 00110 xcvr_spi_init(gXcvrSpiInstance_c); 00111 xcvr_spi_configure_speed(gXcvrSpiInstance_c, 8000000); 00112 00113 gXcvrDeassertCS_d(); 00114 MCR20Drv_RST_B_Deassert(); 00115 RF_IRQ_Init(); 00116 RF_IRQ_Disable(); 00117 mPhyIrqDisableCnt = 1; 00118 } 00119 00120 /*--------------------------------------------------------------------------- 00121 * Name: MCR20Drv_DirectAccessSPIWrite 00122 * Description: - 00123 * Parameters: - 00124 * Return: - 00125 *---------------------------------------------------------------------------*/ 00126 void MCR20Drv_DirectAccessSPIWrite 00127 ( 00128 uint8_t address, 00129 uint8_t value 00130 ) 00131 { 00132 uint16_t txData; 00133 00134 ProtectFromMCR20Interrupt(); 00135 00136 xcvr_spi_configure_speed(gXcvrSpiInstance_c, 16000000); 00137 00138 gXcvrAssertCS_d(); 00139 00140 txData = (address & TransceiverSPI_DirectRegisterAddressMask); 00141 txData |= value << 8; 00142 00143 xcvr_spi_transfer(gXcvrSpiInstance_c, (uint8_t *)&txData, 0, sizeof(txData)); 00144 00145 gXcvrDeassertCS_d(); 00146 UnprotectFromMCR20Interrupt(); 00147 } 00148 00149 /*--------------------------------------------------------------------------- 00150 * Name: MCR20Drv_DirectAccessSPIMultiByteWrite 00151 * Description: - 00152 * Parameters: - 00153 * Return: - 00154 *---------------------------------------------------------------------------*/ 00155 void MCR20Drv_DirectAccessSPIMultiByteWrite 00156 ( 00157 uint8_t startAddress, 00158 uint8_t * byteArray, 00159 uint8_t numOfBytes 00160 ) 00161 { 00162 uint8_t txData; 00163 00164 if( (numOfBytes == 0) || (byteArray == 0) ) 00165 { 00166 return; 00167 } 00168 00169 ProtectFromMCR20Interrupt(); 00170 00171 xcvr_spi_configure_speed(gXcvrSpiInstance_c, 16000000); 00172 00173 gXcvrAssertCS_d(); 00174 00175 txData = (startAddress & TransceiverSPI_DirectRegisterAddressMask); 00176 00177 xcvr_spi_transfer(gXcvrSpiInstance_c, &txData, 0, sizeof(txData)); 00178 xcvr_spi_transfer(gXcvrSpiInstance_c, byteArray, 0, numOfBytes); 00179 00180 gXcvrDeassertCS_d(); 00181 UnprotectFromMCR20Interrupt(); 00182 } 00183 00184 /*--------------------------------------------------------------------------- 00185 * Name: MCR20Drv_PB_SPIByteWrite 00186 * Description: - 00187 * Parameters: - 00188 * Return: - 00189 *---------------------------------------------------------------------------*/ 00190 void MCR20Drv_PB_SPIByteWrite 00191 ( 00192 uint8_t address, 00193 uint8_t value 00194 ) 00195 { 00196 uint32_t txData; 00197 00198 ProtectFromMCR20Interrupt(); 00199 00200 xcvr_spi_configure_speed(gXcvrSpiInstance_c, 16000000); 00201 00202 gXcvrAssertCS_d(); 00203 00204 txData = TransceiverSPI_WriteSelect | 00205 TransceiverSPI_PacketBuffAccessSelect | 00206 TransceiverSPI_PacketBuffByteModeSelect; 00207 txData |= (address) << 8; 00208 txData |= (value) << 16; 00209 00210 xcvr_spi_transfer(gXcvrSpiInstance_c, (uint8_t*)&txData, 0, 3); 00211 00212 gXcvrDeassertCS_d(); 00213 UnprotectFromMCR20Interrupt(); 00214 } 00215 00216 /*--------------------------------------------------------------------------- 00217 * Name: MCR20Drv_PB_SPIBurstWrite 00218 * Description: - 00219 * Parameters: - 00220 * Return: - 00221 *---------------------------------------------------------------------------*/ 00222 void MCR20Drv_PB_SPIBurstWrite 00223 ( 00224 uint8_t * byteArray, 00225 uint8_t numOfBytes 00226 ) 00227 { 00228 uint8_t txData; 00229 00230 if( (numOfBytes == 0) || (byteArray == 0) ) 00231 { 00232 return; 00233 } 00234 00235 ProtectFromMCR20Interrupt(); 00236 00237 xcvr_spi_configure_speed(gXcvrSpiInstance_c, 16000000); 00238 00239 gXcvrAssertCS_d(); 00240 00241 txData = TransceiverSPI_WriteSelect | 00242 TransceiverSPI_PacketBuffAccessSelect | 00243 TransceiverSPI_PacketBuffBurstModeSelect; 00244 00245 xcvr_spi_transfer(gXcvrSpiInstance_c, &txData, 0, 1); 00246 xcvr_spi_transfer(gXcvrSpiInstance_c, byteArray, 0, numOfBytes); 00247 00248 gXcvrDeassertCS_d(); 00249 UnprotectFromMCR20Interrupt(); 00250 } 00251 00252 /*--------------------------------------------------------------------------- 00253 * Name: MCR20Drv_DirectAccessSPIRead 00254 * Description: - 00255 * Parameters: - 00256 * Return: - 00257 *---------------------------------------------------------------------------*/ 00258 00259 uint8_t MCR20Drv_DirectAccessSPIRead 00260 ( 00261 uint8_t address 00262 ) 00263 { 00264 uint8_t txData; 00265 uint8_t rxData; 00266 00267 ProtectFromMCR20Interrupt(); 00268 00269 xcvr_spi_configure_speed(gXcvrSpiInstance_c, 8000000); 00270 00271 gXcvrAssertCS_d(); 00272 00273 txData = (address & TransceiverSPI_DirectRegisterAddressMask) | 00274 TransceiverSPI_ReadSelect; 00275 00276 xcvr_spi_transfer(gXcvrSpiInstance_c, &txData, 0, sizeof(txData)); 00277 xcvr_spi_transfer(gXcvrSpiInstance_c, 0, &rxData, sizeof(rxData)); 00278 00279 gXcvrDeassertCS_d(); 00280 UnprotectFromMCR20Interrupt(); 00281 00282 return rxData; 00283 00284 } 00285 00286 /*--------------------------------------------------------------------------- 00287 * Name: MCR20Drv_DirectAccessSPIMultyByteRead 00288 * Description: - 00289 * Parameters: - 00290 * Return: - 00291 *---------------------------------------------------------------------------*/ 00292 uint8_t MCR20Drv_DirectAccessSPIMultiByteRead 00293 ( 00294 uint8_t startAddress, 00295 uint8_t * byteArray, 00296 uint8_t numOfBytes 00297 ) 00298 { 00299 uint8_t txData; 00300 uint8_t phyIRQSTS1; 00301 00302 if( (numOfBytes == 0) || (byteArray == 0) ) 00303 { 00304 return 0; 00305 } 00306 00307 ProtectFromMCR20Interrupt(); 00308 00309 xcvr_spi_configure_speed(gXcvrSpiInstance_c, 8000000); 00310 00311 gXcvrAssertCS_d(); 00312 00313 txData = (startAddress & TransceiverSPI_DirectRegisterAddressMask) | 00314 TransceiverSPI_ReadSelect; 00315 00316 xcvr_spi_transfer(gXcvrSpiInstance_c, &txData, &phyIRQSTS1, sizeof(txData)); 00317 xcvr_spi_transfer(gXcvrSpiInstance_c, 0, byteArray, numOfBytes); 00318 00319 gXcvrDeassertCS_d(); 00320 UnprotectFromMCR20Interrupt(); 00321 00322 return phyIRQSTS1; 00323 } 00324 00325 /*--------------------------------------------------------------------------- 00326 * Name: MCR20Drv_PB_SPIBurstRead 00327 * Description: - 00328 * Parameters: - 00329 * Return: - 00330 *---------------------------------------------------------------------------*/ 00331 uint8_t MCR20Drv_PB_SPIBurstRead 00332 ( 00333 uint8_t * byteArray, 00334 uint8_t numOfBytes 00335 ) 00336 { 00337 uint8_t txData; 00338 uint8_t phyIRQSTS1; 00339 00340 if( (numOfBytes == 0) || (byteArray == 0) ) 00341 { 00342 return 0; 00343 } 00344 00345 ProtectFromMCR20Interrupt(); 00346 00347 xcvr_spi_configure_speed(gXcvrSpiInstance_c, 8000000); 00348 00349 gXcvrAssertCS_d(); 00350 00351 txData = TransceiverSPI_ReadSelect | 00352 TransceiverSPI_PacketBuffAccessSelect | 00353 TransceiverSPI_PacketBuffBurstModeSelect; 00354 00355 xcvr_spi_transfer(gXcvrSpiInstance_c, &txData, &phyIRQSTS1, sizeof(txData)); 00356 xcvr_spi_transfer(gXcvrSpiInstance_c, 0, byteArray, numOfBytes); 00357 00358 gXcvrDeassertCS_d(); 00359 UnprotectFromMCR20Interrupt(); 00360 00361 return phyIRQSTS1; 00362 } 00363 00364 /*--------------------------------------------------------------------------- 00365 * Name: MCR20Drv_IndirectAccessSPIWrite 00366 * Description: - 00367 * Parameters: - 00368 * Return: - 00369 *---------------------------------------------------------------------------*/ 00370 void MCR20Drv_IndirectAccessSPIWrite 00371 ( 00372 uint8_t address, 00373 uint8_t value 00374 ) 00375 { 00376 uint32_t txData; 00377 00378 ProtectFromMCR20Interrupt(); 00379 00380 xcvr_spi_configure_speed(gXcvrSpiInstance_c, 16000000); 00381 00382 gXcvrAssertCS_d(); 00383 00384 txData = TransceiverSPI_IARIndexReg; 00385 txData |= (address) << 8; 00386 txData |= (value) << 16; 00387 00388 xcvr_spi_transfer(gXcvrSpiInstance_c, (uint8_t*)&txData, 0, 3); 00389 00390 gXcvrDeassertCS_d(); 00391 UnprotectFromMCR20Interrupt(); 00392 } 00393 00394 /*--------------------------------------------------------------------------- 00395 * Name: MCR20Drv_IndirectAccessSPIMultiByteWrite 00396 * Description: - 00397 * Parameters: - 00398 * Return: - 00399 *---------------------------------------------------------------------------*/ 00400 void MCR20Drv_IndirectAccessSPIMultiByteWrite 00401 ( 00402 uint8_t startAddress, 00403 uint8_t * byteArray, 00404 uint8_t numOfBytes 00405 ) 00406 { 00407 uint16_t txData; 00408 00409 if( (numOfBytes == 0) || (byteArray == 0) ) 00410 { 00411 return; 00412 } 00413 00414 ProtectFromMCR20Interrupt(); 00415 00416 xcvr_spi_configure_speed(gXcvrSpiInstance_c, 16000000); 00417 00418 gXcvrAssertCS_d(); 00419 00420 txData = TransceiverSPI_IARIndexReg; 00421 txData |= (startAddress) << 8; 00422 00423 xcvr_spi_transfer(gXcvrSpiInstance_c, (uint8_t*)&txData, 0, sizeof(txData)); 00424 xcvr_spi_transfer(gXcvrSpiInstance_c, (uint8_t*)byteArray, 0, numOfBytes); 00425 00426 gXcvrDeassertCS_d(); 00427 UnprotectFromMCR20Interrupt(); 00428 } 00429 00430 /*--------------------------------------------------------------------------- 00431 * Name: MCR20Drv_IndirectAccessSPIRead 00432 * Description: - 00433 * Parameters: - 00434 * Return: - 00435 *---------------------------------------------------------------------------*/ 00436 uint8_t MCR20Drv_IndirectAccessSPIRead 00437 ( 00438 uint8_t address 00439 ) 00440 { 00441 uint16_t txData; 00442 uint8_t rxData; 00443 00444 ProtectFromMCR20Interrupt(); 00445 00446 xcvr_spi_configure_speed(gXcvrSpiInstance_c, 8000000); 00447 00448 gXcvrAssertCS_d(); 00449 00450 txData = TransceiverSPI_IARIndexReg | TransceiverSPI_ReadSelect; 00451 txData |= (address) << 8; 00452 00453 xcvr_spi_transfer(gXcvrSpiInstance_c, (uint8_t*)&txData, 0, sizeof(txData)); 00454 xcvr_spi_transfer(gXcvrSpiInstance_c, 0, &rxData, sizeof(rxData)); 00455 00456 gXcvrDeassertCS_d(); 00457 UnprotectFromMCR20Interrupt(); 00458 00459 return rxData; 00460 } 00461 00462 /*--------------------------------------------------------------------------- 00463 * Name: MCR20Drv_IndirectAccessSPIMultiByteRead 00464 * Description: - 00465 * Parameters: - 00466 * Return: - 00467 *---------------------------------------------------------------------------*/ 00468 void MCR20Drv_IndirectAccessSPIMultiByteRead 00469 ( 00470 uint8_t startAddress, 00471 uint8_t * byteArray, 00472 uint8_t numOfBytes 00473 ) 00474 { 00475 uint16_t txData; 00476 00477 if( (numOfBytes == 0) || (byteArray == 0) ) 00478 { 00479 return; 00480 } 00481 00482 ProtectFromMCR20Interrupt(); 00483 00484 xcvr_spi_configure_speed(gXcvrSpiInstance_c, 8000000); 00485 00486 gXcvrAssertCS_d(); 00487 00488 txData = (TransceiverSPI_IARIndexReg | TransceiverSPI_ReadSelect); 00489 txData |= (startAddress) << 8; 00490 00491 xcvr_spi_transfer(gXcvrSpiInstance_c, (uint8_t*)&txData, 0, sizeof(txData)); 00492 xcvr_spi_transfer(gXcvrSpiInstance_c, 0, byteArray, numOfBytes); 00493 00494 gXcvrDeassertCS_d(); 00495 UnprotectFromMCR20Interrupt(); 00496 } 00497 00498 /*--------------------------------------------------------------------------- 00499 * Name: MCR20Drv_IsIrqPending 00500 * Description: - 00501 * Parameters: - 00502 * Return: - 00503 *---------------------------------------------------------------------------*/ 00504 uint32_t MCR20Drv_IsIrqPending 00505 ( 00506 void 00507 ) 00508 { 00509 return RF_isIRQ_Pending(); 00510 } 00511 00512 /*--------------------------------------------------------------------------- 00513 * Name: MCR20Drv_IRQ_Disable 00514 * Description: - 00515 * Parameters: - 00516 * Return: - 00517 *---------------------------------------------------------------------------*/ 00518 void MCR20Drv_IRQ_Disable 00519 ( 00520 void 00521 ) 00522 { 00523 platform_enter_critical(); 00524 00525 if( mPhyIrqDisableCnt == 0 ) 00526 { 00527 RF_IRQ_Disable(); 00528 } 00529 00530 mPhyIrqDisableCnt++; 00531 00532 platform_exit_critical(); 00533 } 00534 00535 /*--------------------------------------------------------------------------- 00536 * Name: MCR20Drv_IRQ_Enable 00537 * Description: - 00538 * Parameters: - 00539 * Return: - 00540 *---------------------------------------------------------------------------*/ 00541 void MCR20Drv_IRQ_Enable 00542 ( 00543 void 00544 ) 00545 { 00546 platform_enter_critical(); 00547 00548 if( mPhyIrqDisableCnt ) 00549 { 00550 mPhyIrqDisableCnt--; 00551 00552 if( mPhyIrqDisableCnt == 0 ) 00553 { 00554 RF_IRQ_Enable(); 00555 } 00556 } 00557 00558 platform_exit_critical(); 00559 } 00560 00561 /*--------------------------------------------------------------------------- 00562 * Name: MCR20Drv_RST_Assert 00563 * Description: - 00564 * Parameters: - 00565 * Return: - 00566 *---------------------------------------------------------------------------*/ 00567 void MCR20Drv_RST_B_Assert 00568 ( 00569 void 00570 ) 00571 { 00572 RF_RST_Set(0); 00573 } 00574 00575 /*--------------------------------------------------------------------------- 00576 * Name: MCR20Drv_RST_Deassert 00577 * Description: - 00578 * Parameters: - 00579 * Return: - 00580 *---------------------------------------------------------------------------*/ 00581 void MCR20Drv_RST_B_Deassert 00582 ( 00583 void 00584 ) 00585 { 00586 RF_RST_Set(1); 00587 } 00588 00589 /*--------------------------------------------------------------------------- 00590 * Name: MCR20Drv_SoftRST_Assert 00591 * Description: - 00592 * Parameters: - 00593 * Return: - 00594 *---------------------------------------------------------------------------*/ 00595 void MCR20Drv_SoftRST_Assert 00596 ( 00597 void 00598 ) 00599 { 00600 MCR20Drv_IndirectAccessSPIWrite(SOFT_RESET, (0x80)); 00601 } 00602 00603 /*--------------------------------------------------------------------------- 00604 * Name: MCR20Drv_SoftRST_Deassert 00605 * Description: - 00606 * Parameters: - 00607 * Return: - 00608 *---------------------------------------------------------------------------*/ 00609 void MCR20Drv_SoftRST_Deassert 00610 ( 00611 void 00612 ) 00613 { 00614 MCR20Drv_IndirectAccessSPIWrite(SOFT_RESET, (0x00)); 00615 } 00616 00617 /*--------------------------------------------------------------------------- 00618 * Name: MCR20Drv_Soft_RESET 00619 * Description: - 00620 * Parameters: - 00621 * Return: - 00622 *---------------------------------------------------------------------------*/ 00623 void MCR20Drv_Soft_RESET 00624 ( 00625 void 00626 ) 00627 { 00628 //assert SOG_RST 00629 MCR20Drv_IndirectAccessSPIWrite(SOFT_RESET, (0x80)); 00630 00631 //deassert SOG_RST 00632 MCR20Drv_IndirectAccessSPIWrite(SOFT_RESET, (0x00)); 00633 } 00634 00635 /*--------------------------------------------------------------------------- 00636 * Name: MCR20Drv_RESET 00637 * Description: - 00638 * Parameters: - 00639 * Return: - 00640 *---------------------------------------------------------------------------*/ 00641 void MCR20Drv_RESET 00642 ( 00643 void 00644 ) 00645 { 00646 volatile uint32_t delay = 1000; 00647 //assert RST_B 00648 MCR20Drv_RST_B_Assert(); 00649 00650 while(delay--); 00651 00652 //deassert RST_B 00653 MCR20Drv_RST_B_Deassert(); 00654 } 00655 00656 /*--------------------------------------------------------------------------- 00657 * Name: MCR20Drv_Set_CLK_OUT_Freq 00658 * Description: - 00659 * Parameters: - 00660 * Return: - 00661 *---------------------------------------------------------------------------*/ 00662 void MCR20Drv_Set_CLK_OUT_Freq 00663 ( 00664 uint8_t freqDiv 00665 ) 00666 { 00667 uint8_t clkOutCtrlReg = (freqDiv & cCLK_OUT_DIV_Mask) | cCLK_OUT_EN | cCLK_OUT_EXTEND; 00668 00669 if(freqDiv == gCLK_OUT_FREQ_DISABLE) 00670 { 00671 clkOutCtrlReg = (cCLK_OUT_EXTEND | gCLK_OUT_FREQ_4_MHz); //reset value with clock out disabled 00672 } 00673 00674 MCR20Drv_DirectAccessSPIWrite((uint8_t) CLK_OUT_CTRL, clkOutCtrlReg); 00675 }
Generated on Tue Jul 12 2022 17:40:25 by
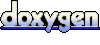