
David's line following code from the LVBots competition, 2015.
Dependencies: GeneralDebouncer Pacer PololuEncoder mbed
Fork of DeadReckoning by
turn_sensor.h
00001 #pragma once 00002 00003 #include <mbed.h> 00004 00005 class TurnSensor 00006 { 00007 // TODO: for production code, you would want a way to set the gyro offset 00008 00009 public: 00010 00011 void reset(); 00012 void start(); 00013 void update(); 00014 00015 int32_t getAngle() 00016 { 00017 return (int32_t)angleUnsigned; 00018 } 00019 00020 uint32_t getAngleUnsigned() 00021 { 00022 return angleUnsigned; 00023 } 00024 00025 int16_t getAngleDegrees() 00026 { 00027 return (((int32_t)angleUnsigned >> 16) * 360) >> 16; 00028 } 00029 00030 int16_t getRate() 00031 { 00032 return rate; 00033 } 00034 00035 private: 00036 00037 Timer timer; 00038 uint32_t angleUnsigned; 00039 int16_t rate; 00040 uint16_t gyroLastUpdate; 00041 }; 00042 00043 00044 // This constant represents a turn of 45 degrees. 00045 const int32_t turnAngle45 = 0x20000000; 00046 00047 // This constant represents a turn of 90 degrees. 00048 const int32_t turnAngle90 = turnAngle45 * 2; 00049 00050 // This constant represents a turn of approximately 1 degree. 00051 const int32_t turnAngle1 = (turnAngle45 + 22) / 45;
Generated on Sun Jul 17 2022 15:55:09 by
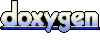