
David's line following code from the LVBots competition, 2015.
Dependencies: GeneralDebouncer Pacer PololuEncoder mbed
Fork of DeadReckoning by
logger.h
00001 #pragma once 00002 00003 #include <stdint.h> 00004 00005 #define LOGGER_SIZE 2000 00006 00007 struct LogEntry 00008 { 00009 int16_t turnAngle; 00010 int16_t x; 00011 int16_t y; 00012 }; 00013 00014 class Logger 00015 { 00016 public: 00017 Logger(); 00018 void log(struct LogEntry *); 00019 void dump(); 00020 bool isFull(); 00021 int32_t getSize() { return entryIndex; } 00022 00023 LogEntry entries[LOGGER_SIZE]; 00024 00025 // The index of the next entry to write to. 00026 uint32_t entryIndex; 00027 }; 00028
Generated on Sun Jul 17 2022 15:55:09 by
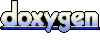