
Satellite Observers Workbench. NOT yet complete, just published for forum posters to \"cherry pick\" pieces of code as requiered as an example.
gpioirq.c
00001 /**************************************************************************** 00002 * Copyright 2010 Andy Kirkham, Stellar Technologies Ltd 00003 * 00004 * This file is part of the Satellite Observers Workbench (SOWB). 00005 * 00006 * SOWB is free software: you can redistribute it and/or modify 00007 * it under the terms of the GNU General Public License as published by 00008 * the Free Software Foundation, either version 3 of the License, or 00009 * (at your option) any later version. 00010 * 00011 * SOWB is distributed in the hope that it will be useful, 00012 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00013 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00014 * GNU General Public License for more details. 00015 * 00016 * You should have received a copy of the GNU General Public License 00017 * along with SOWB. If not, see <http://www.gnu.org/licenses/>. 00018 * 00019 * $Id: main.cpp 5 2010-07-12 20:51:11Z ajk $ 00020 * 00021 ***************************************************************************/ 00022 00023 #include "sowb.h" 00024 #include "debug.h" 00025 #include "gpioirq.h" 00026 00027 /* Declare the external interrupt callback functions. */ 00028 extern void MAX7456_vsync_rise(void); 00029 extern void MAX7456_vsync_fall(void); 00030 //extern void max7456_los_rise(void); 00031 //extern void max7456_los_fall(void); 00032 extern void gps_pps_rise(void); 00033 extern void gps_pps_fall(void); 00034 00035 /** EINT3_IRQHandler 00036 */ 00037 extern "C" void GPIOIRQ_IRQHandler (void) { 00038 00039 /* Test for IRQ on Port0. */ 00040 if (LPC_GPIOINT->IntStatus & 0x1) { 00041 00042 /* GPS PPS is connected to MBED P29 (Port0.5) */ 00043 //if (LPC_GPIOINT->IO0IntStatR & (1 << 5)) gps_pps_rise(); 00044 00045 /* GPS PPS is connected to MBED P29 (Port0.5) */ 00046 if (LPC_GPIOINT->IO0IntStatF & (1 << 5)) gps_pps_fall(); 00047 00048 /* MAX7456 Vertical Sync is connected to MBED P15 (Port0.23) */ 00049 if (LPC_GPIOINT->IO0IntStatF & (1 << 23)) MAX7456_vsync_fall(); 00050 00051 /* MAX7456 LOS is connected to P17 (Port0.25) */ 00052 //if (LPC_GPIOINT->IO0IntStatR & (1 << 25)) max7456_los_rise(); 00053 00054 LPC_GPIOINT->IO0IntClr = (LPC_GPIOINT->IO0IntStatR | LPC_GPIOINT->IO0IntStatF); 00055 } 00056 00057 /* Test for IRQ on Port2. */ 00058 if (LPC_GPIOINT->IntStatus & 0x4) { 00059 00060 LPC_GPIOINT->IO2IntClr = (LPC_GPIOINT->IO2IntStatR | LPC_GPIOINT->IO2IntStatF); 00061 } 00062 } 00063 00064 /** gpioirq_init 00065 */ 00066 void gpioirq_init(void) { 00067 00068 DEBUG_INIT_START; 00069 00070 /* Enable the interrupts for connected signals. 00071 For bit definitions see the ISR function above. */ 00072 LPC_GPIOINT->IO0IntEnR |= ( (1UL << 5) | (1UL << 25) | (1UL << 23) ); 00073 LPC_GPIOINT->IO0IntEnF |= ( (1UL << 5) | (1UL << 25) | (1UL << 23) ); 00074 //LPC_GPIOINT->IO2IntEnR |= ( (1UL << 5) ); 00075 //LPC_GPIOINT->IO2IntEnF |= ( (1UL << 5) ); 00076 00077 NVIC_SetVector(EINT3_IRQn, (uint32_t)GPIOIRQ_IRQHandler); 00078 NVIC_EnableIRQ(EINT3_IRQn); 00079 00080 DEBUG_INIT_END; 00081 } 00082 00083 void gpioirq_process(void) { 00084 /* Does nothing, no periodic house keeping required. */ 00085 } 00086 00087 00088
Generated on Tue Jul 12 2022 18:05:35 by
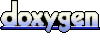