This module provides a simple API to the Maxim MAX7456 on-screen display chip
MAX7456_API
Functions | |
MAX7456 (PinName mosi, PinName miso, PinName sclk, const char *name, PinName cs, PinName rst, PinName vsync) | |
MAX7456 constructor. | |
MAX7456 (PinName mosi, PinName miso, PinName sclk, PinName cs, PinName rst, PinName vsync) | |
MAX7456 constructor. | |
void | reset (void) |
reset() | |
void | clear_display (void) |
clear_display() | |
void | cursor (int x, int y) |
cursor(x,y) | |
int | string (char *s) |
string(*s) | |
int | stringxy (int x, int y, char *s) |
stringxy(x,y,s) | |
int | stringxy (int x, int y, char *s, uint8_t a) |
stringxy(x,y,s,a) | |
int | stringxy (int x, int y, char *s, char *a) |
stringxy(x,y,s,a) | |
void | stringl (int x, int y, char *s, int len) |
stringxy(x,y,s,len) | |
void | attributes_xyl (int x, int y, char *s, int len) |
attribute_xyl(x,y,s,len) | |
void | backGround (BGbrightness i) |
Set the background brightness. | |
void | blinkRate (BlinkTime bt, BlinkingDutyCycle dc) |
Set the character blink rate. | |
void | read_char_map (unsigned char address, unsigned char *data54) |
read_char_map(address,data54) | |
void | write_char_map (unsigned char address, const unsigned char *data54) |
write_char_map(address,data54) | |
template<typename T > | |
void | vsync_set_callback (T *tptr, void(T::*mptr)(void)) |
attach(tptr,mptr) | |
void | vsync_set_callback (void(*fptr)(void)) |
attach(tptr,mptr) |
Function Documentation
void attributes_xyl | ( | int | x, |
int | y, | ||
char * | s, | ||
int | len | ||
) | [inherited] |
attribute_xyl(x,y,s,len)
Write the character attribute bytes at x,y Since attributes can be zero (NULL), the string length must be provided.
- Parameters:
-
x The X position y The Y position s A char * pointer to the NULL terminated string of attributes. len The length of the string of attributes.
Definition at line 203 of file MAX7456.cpp.
void backGround | ( | BGbrightness | i ) | [inherited] |
void blinkRate | ( | BlinkTime | bt, |
BlinkingDutyCycle | dc | ||
) | [inherited] |
Set the character blink rate.
- See also:
- BlinkTime
- BlinkingDutyCycle
- Parameters:
-
bt The blink time dc The duty cycle
void clear_display | ( | void | ) | [inherited] |
void cursor | ( | int | x, |
int | y | ||
) | [inherited] |
cursor(x,y)
Moves the "cursor" to the screen X,Y position. Future writes to the screen ram will occur at this position.
- Parameters:
-
x The X position y The Y position
Definition at line 173 of file MAX7456.cpp.
MAX7456 | ( | PinName | mosi, |
PinName | miso, | ||
PinName | sclk, | ||
PinName | cs, | ||
PinName | rst, | ||
PinName | vsync | ||
) | [inherited] |
MAX7456 constructor.
The MAX7456 constructor is used to initialise the MAX7456 object.
Example 1
#include "mbed.h" #include "MAX7456.h" MAX7456 max(p5, p6, p7, p8, p20, p15); int main() { max.stringxy(1, 1, "Hello World"); while(1); }
Example 2
#include "mbed.h" #include "MAX7456.h" int main() { MAX7456 max = new MAX7456(p5, p6, p7, p8, p20, p15); max->stringxy(1, 1, "Hello World"); while(1); }
- Parameters:
-
miso PinName p5 or p11 mosi PinName p6 or p12 sclk PinName p7 pr p13 cs PinName CS signal rst PinName RESET signal vsync PinName Vertical sync signal
Definition at line 77 of file MAX7456.cpp.
MAX7456 | ( | PinName | mosi, |
PinName | miso, | ||
PinName | sclk, | ||
const char * | name, | ||
PinName | cs, | ||
PinName | rst, | ||
PinName | vsync | ||
) | [inherited] |
MAX7456 constructor.
The MAX7456 constructor is used to initialise the MAX7456 object.
Example 1
#include "mbed.h" #include "MAX7456.h" MAX7456 max(p5, p6, p7, NULL, p8, p20, p15); int main() { max.stringxy(1, 1, "Hello World"); while(1); }
Example 2
#include "mbed.h" #include "MAX7456.h" int main() { MAX7456 max = new MAX7456(p5, p6, p7, NULL, p8, p20, p15); max->stringxy(1, 1, "Hello World"); while(1); }
- Parameters:
-
miso PinName p5 or p11 mosi PinName p6 or p12 sclk PinName p7 pr p13 name Optional const char * SSP object name cs PinName CS signal rst PinName RESET signal vsync PinName Vertical sync signal
Definition at line 72 of file MAX7456.cpp.
void read_char_map | ( | unsigned char | address, |
unsigned char * | data54 | ||
) | [inherited] |
read_char_map(address,data54)
Read the 54byte character map from MAX7456 ROM.
- Parameters:
-
address The uchar address to read from (which character) data54 A char * pointer to a buffer where to write to.
Definition at line 262 of file MAX7456.cpp.
void reset | ( | void | ) | [inherited] |
int string | ( | char * | s ) | [inherited] |
string(*s)
Print the string pointed to by s at the current cursor position. The string should be an ASCII NULL terminated string.
- Parameters:
-
s A char * pointer to the NULL terminated string to print.
Definition at line 190 of file MAX7456.cpp.
void stringl | ( | int | x, |
int | y, | ||
char * | s, | ||
int | len | ||
) | [inherited] |
stringxy(x,y,s,len)
Print the string pointed to by s at the position supplied by x,y. The string should be an ASCII terminated string. len determines the length of the string to print (not the term NULL).
- Parameters:
-
x The X position y The Y position s A char * pointer to the NULL terminated string to print. len The length of teh string to print.
Definition at line 250 of file MAX7456.cpp.
int stringxy | ( | int | x, |
int | y, | ||
char * | s, | ||
uint8_t | a | ||
) | [inherited] |
stringxy(x,y,s,a)
Print the string pointed to by s at the position supplied by x,y. The string should be an ASCII NULL terminated string.
- Parameters:
-
x The X position y The Y position s A char * pointer to the NULL terminated string to print. a An attribute byte to appply to the string.
- Returns:
- int The length of the string written.
Definition at line 223 of file MAX7456.cpp.
int stringxy | ( | int | x, |
int | y, | ||
char * | s, | ||
char * | a | ||
) | [inherited] |
stringxy(x,y,s,a)
Print the string pointed to by s at the position supplied by x,y. The string should be an ASCII NULL terminated string.
- Parameters:
-
x The X position y The Y position s A char * pointer to the NULL terminated string to print. a A char * pointer to the 30byte attribute string.
- Returns:
- int The length of the string written.
Definition at line 240 of file MAX7456.cpp.
int stringxy | ( | int | x, |
int | y, | ||
char * | s | ||
) | [inherited] |
stringxy(x,y,s)
Print the string pointed to by s at the position supplied by x,y. The string should be an ASCII NULL terminated string.
- Parameters:
-
x The X position y The Y position s A char * pointer to the NULL terminated string to print.
- Returns:
- int The length of the string written.
Definition at line 216 of file MAX7456.cpp.
void vsync_set_callback | ( | T * | tptr, |
void(T::*)(void) | mptr | ||
) | [inherited] |
void vsync_set_callback | ( | void(*)(void) | fptr ) | [inherited] |
void write_char_map | ( | unsigned char | address, |
const unsigned char * | data54 | ||
) | [inherited] |
write_char_map(address,data54)
Write the 54byte character map to the MAX7456 ROM.
- Parameters:
-
address The uchar address to write to data54 A char * pointer to a buffer where to read from.
Definition at line 274 of file MAX7456.cpp.
Generated on Wed Jul 13 2022 11:53:43 by
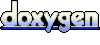