This module provides a simple API to the Maxim MAX7456 on-screen display chip
OSD7456 Class Reference
#include <OSD7456.h>
Public Member Functions | |
OSD7456 (PinName mosi, PinName miso, PinName sclk, const char *name, PinName cs, PinName rst, PinName vsync) | |
OSD7456 constructor. | |
OSD7456 (PinName mosi, PinName miso, PinName sclk, PinName cs, PinName rst, PinName vsync) | |
OSD7456 constructor. | |
int | print (int line, char *s) |
print() | |
int | print (int x, int y, char *s) |
print() | |
int | print (int line, char *s, char a) |
print() | |
int | print (int x, int y, char *s, char attrib) |
print() | |
void | clear (int line) |
clear() | |
template<typename T > | |
void | attach_vsync (T *tptr, void(T::*mptr)(void)) |
Attach a user callback object/method to call when the vsync signal activates. | |
void | attach_vsync (void(*fptr)(void)) |
Attach a user callback function pointer to call when the vsync signal activates. | |
__INLINE int | print0 (char *s) |
Data Fields | |
FunctionPointer | cb_vsync |
A callback object for the 1PPS user API. |
Detailed Description
OSD7456 module.
The OSD7456 is a wrapper around the MAX7456 that abstracts the hardware into a simple to use screen writer system. It provides buffered output. To ensure a "flicker free" display, the buffers are written to the MAX7456 chip at the start of the vertical sync period.
- See also:
- http://mbed.org/cookbook/
- example2.cpp
Example:
#include "mbed.h" #include "OSD7456.h" DigitalOut led1(LED1); OSD7456 *osd; int main() { osd = new OSD7456(p5, p6, p7, p8, p20, p15); osd->print(1, "Hello World"); osd->print2("This blinks", MAX7456::Blink); osd->print3("Background", MAX7456::LocalBG); osd->print(5, 4, "Positioned"); osd->print(5, 5, "Positioned", MAX7456::LocalBG); osd->print(3, 7, "Test"); osd->print(12, 7, "Test", MAX7456::LocalBG | MAX7456::Blink); while(1) { led1 = 1; wait(0.5); led1 = 0; wait(0.5); } }
Definition at line 81 of file OSD7456.h.
Constructor & Destructor Documentation
OSD7456 | ( | PinName | mosi, |
PinName | miso, | ||
PinName | sclk, | ||
const char * | name, | ||
PinName | cs, | ||
PinName | rst, | ||
PinName | vsync | ||
) |
OSD7456 | ( | PinName | mosi, |
PinName | miso, | ||
PinName | sclk, | ||
PinName | cs, | ||
PinName | rst, | ||
PinName | vsync | ||
) |
Member Function Documentation
void attach_vsync | ( | T * | tptr, |
void(T::*)(void) | mptr | ||
) |
void attach_vsync | ( | void(*)(void) | fptr ) |
void clear | ( | int | line ) |
Clear the line number supplied.
- Parameters:
-
line The line number to clear.
Definition at line 35 of file OSD7456.cpp.
int print | ( | int | x, |
int | y, | ||
char * | s, | ||
char | attrib | ||
) |
Print ASCII text on line y at position x
- Parameters:
-
x The line position to print at. y The line to print on. s A pointer to the null terminated string to print. a An attribute byte to apply to the string.
Definition at line 98 of file OSD7456.cpp.
int print | ( | int | line, |
char * | s | ||
) |
Print ASCII text at line.
- Parameters:
-
line The line number to print at. s A pointer to the null terminated string to print.
Definition at line 61 of file OSD7456.cpp.
int print | ( | int | x, |
int | y, | ||
char * | s | ||
) |
Print ASCII text on line y at position x
- Parameters:
-
x The line position to print at. y The line to print on. s A pointer to the null terminated string to print.
Definition at line 86 of file OSD7456.cpp.
int print | ( | int | line, |
char * | s, | ||
char | a | ||
) |
Print ASCII text on line y at position x with attribute.
- Parameters:
-
x The line position to print at. y The line to print on. s A pointer to the null terminated string to print. a An attribute byte to apply to the string.
Definition at line 73 of file OSD7456.cpp.
Field Documentation
Generated on Wed Jul 13 2022 11:53:43 by
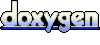