Extends DigitalIn to DebounceIn to provide mechanical switch debouncing.
Dependents: AVC_20110423 Pushbutton_NoBounce_Demo FinalTime AVC_2012 ... more
DebounceIn.h
00001 /* 00002 Copyright (c) 2010 Andy Kirkham 00003 00004 Permission is hereby granted, free of charge, to any person obtaining a copy 00005 of this software and associated documentation files (the "Software"), to deal 00006 in the Software without restriction, including without limitation the rights 00007 to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00008 copies of the Software, and to permit persons to whom the Software is 00009 furnished to do so, subject to the following conditions: 00010 00011 The above copyright notice and this permission notice shall be included in 00012 all copies or substantial portions of the Software. 00013 00014 THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00015 IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00016 FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00017 AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00018 LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00019 OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00020 THE SOFTWARE. 00021 */ 00022 00023 #ifndef DEBOUNCEIN_H 00024 #define DEBOUNCEIN_H 00025 00026 #include "mbed.h" 00027 00028 /** DebounceIn adds mechanical switch debouncing to DigitialIn. 00029 * 00030 * Example: 00031 * @code 00032 * #include "mbed.h" 00033 * #include "DebounceIn.h" 00034 * 00035 * DebounceIn d(p5); 00036 * DigitialOut led1(LED1); 00037 * DigitialOut led2(LED2); 00038 * 00039 * int main() { 00040 * while(1) { 00041 * led1 = d; 00042 * led2 = d.read(); 00043 * } 00044 * } 00045 * @endcode 00046 * 00047 * @see set_debounce_us() To change the sampling frequency. 00048 * @see set_samples() To alter the number of samples. 00049 * 00050 * Users of this library may also be interested in PinDetect library:- 00051 * @see http://mbed.org/users/AjK/libraries/PinDetect/latest 00052 * 00053 * This example shows one input displayed by two outputs. The input 00054 * is debounced by the default 10ms. 00055 */ 00056 00057 class DebounceIn : public DigitalIn { 00058 public: 00059 00060 /** set_debounce_us 00061 * 00062 * Sets the debounce sample period time in microseconds, default is 1000 (1ms) 00063 * 00064 * @param int i The debounce sample period time to set. 00065 */ 00066 void set_debounce_us(int i) { _ticker.attach_us(this, &DebounceIn::_callback, i); } 00067 00068 /** set_samples 00069 * 00070 * Defines the number of samples before switching the shadow 00071 * definition of the pin. 00072 * 00073 * @param int i The number of samples. 00074 */ 00075 void set_samples(int i) { _samples = i; } 00076 00077 /** read 00078 * 00079 * Read the value of the debounced pin. 00080 */ 00081 int read(void) { return _shadow; } 00082 00083 #ifdef MBED_OPERATORS 00084 /** operator int() 00085 * 00086 * Read the value of the debounced pin. 00087 */ 00088 operator int() { return read(); } 00089 #endif 00090 00091 /** Constructor 00092 * 00093 * @param PinName pin The pin to assign as an input. 00094 */ 00095 DebounceIn(PinName pin) : DigitalIn(pin) { _counter = 0; _samples = 10; set_debounce_us(1000); }; 00096 00097 protected: 00098 void _callback(void) { 00099 if (DigitalIn::read()) { 00100 if (_counter < _samples) _counter++; 00101 if (_counter == _samples) _shadow = 1; 00102 } 00103 else { 00104 if (_counter > 0) _counter--; 00105 if (_counter == 0) _shadow = 0; 00106 } 00107 } 00108 00109 Ticker _ticker; 00110 int _shadow; 00111 int _counter; 00112 int _samples; 00113 }; 00114 00115 #endif 00116
Generated on Tue Jul 12 2022 21:29:50 by
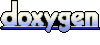