Deprecated fork of old network stack source from github. Please use official library instead: https://mbed.org/users/mbed_official/code/EthernetInterface/
Dynamic memory manager. More...
Go to the source code of this file.
Functions | |
void * | mem_malloc (mem_size_t size) |
Allocate memory: determine the smallest pool that is big enough to contain an element of 'size' and get an element from that pool. | |
void | mem_free (void *rmem) |
Free memory previously allocated by mem_malloc. | |
static void | plug_holes (struct mem *mem) |
"Plug holes" by combining adjacent empty struct mems. | |
void | mem_init (void) |
Zero the heap and initialize start, end and lowest-free. | |
void * | mem_trim (void *rmem, mem_size_t newsize) |
Shrink memory returned by mem_malloc(). | |
void * | mem_calloc (mem_size_t count, mem_size_t size) |
Contiguously allocates enough space for count objects that are size bytes of memory each and returns a pointer to the allocated memory. | |
Variables | |
u8_t ram_heap[MEM_SIZE_ALIGNED+(2 *SIZEOF_STRUCT_MEM)+MEM_ALIGNMENT | ETHMEM_SECTION ) |
the heap. | |
static u8_t * | ram |
pointer to the heap (ram_heap): for alignment, ram is now a pointer instead of an array | |
static struct mem * | ram_end |
the last entry, always unused! | |
static struct mem * | lfree |
pointer to the lowest free block, this is used for faster search | |
static sys_mutex_t | mem_mutex |
concurrent access protection |
Detailed Description
Dynamic memory manager.
This is a lightweight replacement for the standard C library malloc().
If you want to use the standard C library malloc() instead, define MEM_LIBC_MALLOC to 1 in your lwipopts.h
To let mem_malloc() use pools (prevents fragmentation and is much faster than a heap but might waste some memory), define MEM_USE_POOLS to 1, define MEM_USE_CUSTOM_POOLS to 1 and create a file "lwippools.h" that includes a list of pools like this (more pools can be added between _START and _END):
Define three pools with sizes 256, 512, and 1512 bytes LWIP_MALLOC_MEMPOOL_START LWIP_MALLOC_MEMPOOL(20, 256) LWIP_MALLOC_MEMPOOL(10, 512) LWIP_MALLOC_MEMPOOL(5, 1512) LWIP_MALLOC_MEMPOOL_END
Definition in file mem.c.
Function Documentation
void* mem_calloc | ( | mem_size_t | count, |
mem_size_t | size | ||
) |
Contiguously allocates enough space for count objects that are size bytes of memory each and returns a pointer to the allocated memory.
The allocated memory is filled with bytes of value zero.
- Parameters:
-
count number of objects to allocate size size of the objects to allocate
- Returns:
- pointer to allocated memory / NULL pointer if there is an error
void mem_free | ( | void * | rmem ) |
Free memory previously allocated by mem_malloc.
Put a struct mem back on the heap.
Loads the pool number and calls memp_free with that pool number to put the element back into its pool
- Parameters:
-
rmem the memory element to free rmem is the data portion of a struct mem as returned by a previous call to mem_malloc()
void mem_init | ( | void | ) |
void * mem_malloc | ( | mem_size_t | size ) |
Allocate memory: determine the smallest pool that is big enough to contain an element of 'size' and get an element from that pool.
Adam's mem_malloc() plus solution for bug #17922 Allocate a block of memory with a minimum of 'size' bytes.
- Parameters:
-
size the size in bytes of the memory needed
- Returns:
- a pointer to the allocated memory or NULL if the pool is empty
- Parameters:
-
size is the minimum size of the requested block in bytes.
- Returns:
- pointer to allocated memory or NULL if no free memory was found.
Note that the returned value will always be aligned (as defined by MEM_ALIGNMENT).
Try a bigger pool if this one is empty!
void* mem_trim | ( | void * | rmem, |
mem_size_t | newsize | ||
) |
Shrink memory returned by mem_malloc().
- Parameters:
-
rmem pointer to memory allocated by mem_malloc the is to be shrinked newsize required size after shrinking (needs to be smaller than or equal to the previous size)
- Returns:
- for compatibility reasons: is always == rmem, at the moment or NULL if newsize is > old size, in which case rmem is NOT touched or freed!
static void plug_holes | ( | struct mem * | mem ) | [static] |
Variable Documentation
u8_t ram_heap [MEM_SIZE_ALIGNED + (2*SIZEOF_STRUCT_MEM) + MEM_ALIGNMENT ETHMEM_SECTION) |
struct mem* lfree [static] |
u8_t* ram [static] |
Generated on Tue Jul 12 2022 23:10:20 by
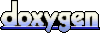