Deprecated fork of old network stack source from github. Please use official library instead: https://mbed.org/users/mbed_official/code/EthernetInterface/
lwipopts.h
00001 /* Copyright (C) 2012 mbed.org, MIT License 00002 * 00003 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00004 * and associated documentation files (the "Software"), to deal in the Software without restriction, 00005 * including without limitation the rights to use, copy, modify, merge, publish, distribute, 00006 * sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is 00007 * furnished to do so, subject to the following conditions: 00008 * 00009 * The above copyright notice and this permission notice shall be included in all copies or 00010 * substantial portions of the Software. 00011 * 00012 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00013 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00014 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00015 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00016 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00017 */ 00018 00019 #ifndef LWIPOPTS_H 00020 #define LWIPOPTS_H 00021 00022 #include "lwipopts_conf.h" 00023 00024 // Operating System 00025 #define NO_SYS 0 00026 00027 #if NO_SYS == 0 00028 #include "cmsis_os.h" 00029 00030 #define SYS_LIGHTWEIGHT_PROT 1 00031 00032 #define LWIP_RAW 0 00033 00034 #define TCPIP_MBOX_SIZE 8 00035 #define DEFAULT_TCP_RECVMBOX_SIZE 8 00036 #define DEFAULT_UDP_RECVMBOX_SIZE 8 00037 #define DEFAULT_RAW_RECVMBOX_SIZE 8 00038 #define DEFAULT_ACCEPTMBOX_SIZE 8 00039 00040 #define TCPIP_THREAD_STACKSIZE 1024 00041 #define TCPIP_THREAD_PRIO (osPriorityNormal) 00042 00043 #define DEFAULT_THREAD_STACKSIZE 512 00044 00045 #define MEMP_NUM_SYS_TIMEOUT 16 00046 #endif 00047 00048 // 32-bit alignment 00049 #define MEM_ALIGNMENT 4 00050 00051 #define MEM_SIZE 16362 00052 00053 #define PBUF_POOL_SIZE 5 00054 #define MEMP_NUM_TCP_PCB_LISTEN 4 00055 #define MEMP_NUM_TCP_PCB 4 00056 #define MEMP_NUM_PBUF 8 00057 00058 #define TCP_QUEUE_OOSEQ 0 00059 #define TCP_OVERSIZE 0 00060 00061 #define LWIP_DHCP 1 00062 #define LWIP_DNS 1 00063 00064 // Support Multicast 00065 #include "stdlib.h" 00066 #define LWIP_IGMP 1 00067 #define LWIP_RAND() rand() 00068 00069 #define LWIP_COMPAT_SOCKETS 0 00070 #define LWIP_POSIX_SOCKETS_IO_NAMES 0 00071 #define LWIP_SO_RCVTIMEO 1 00072 #define LWIP_TCP_KEEPALIVE 1 00073 00074 // Debug Options 00075 // #define LWIP_DEBUG 00076 #define UDP_LPC_EMAC LWIP_DBG_OFF 00077 #define SYS_DEBUG LWIP_DBG_OFF 00078 #define PPP_DEBUG LWIP_DBG_OFF 00079 #define IP_DEBUG LWIP_DBG_OFF 00080 #define MEM_DEBUG LWIP_DBG_OFF 00081 #define MEMP_DEBUG LWIP_DBG_OFF 00082 #define PBUF_DEBUG LWIP_DBG_OFF 00083 #define API_LIB_DEBUG LWIP_DBG_OFF 00084 #define API_MSG_DEBUG LWIP_DBG_OFF 00085 #define TCPIP_DEBUG LWIP_DBG_OFF 00086 #define SOCKETS_DEBUG LWIP_DBG_OFF 00087 #define TCP_DEBUG LWIP_DBG_OFF 00088 #define TCP_INPUT_DEBUG LWIP_DBG_OFF 00089 #define TCP_FR_DEBUG LWIP_DBG_OFF 00090 #define TCP_RTO_DEBUG LWIP_DBG_OFF 00091 #define TCP_CWND_DEBUG LWIP_DBG_OFF 00092 #define TCP_WND_DEBUG LWIP_DBG_OFF 00093 #define TCP_OUTPUT_DEBUG LWIP_DBG_OFF 00094 #define TCP_RST_DEBUG LWIP_DBG_OFF 00095 #define TCP_QLEN_DEBUG LWIP_DBG_OFF 00096 #define ETHARP_DEBUG LWIP_DBG_OFF 00097 #define NETIF_DEBUG LWIP_DBG_OFF 00098 #define DHCP_DEBUG LWIP_DBG_OFF 00099 00100 #ifdef LWIP_DEBUG 00101 #define MEMP_OVERFLOW_CHECK 1 00102 #define MEMP_SANITY_CHECK 1 00103 #else 00104 #define LWIP_NOASSERT 1 00105 #define LWIP_STATS 0 00106 #endif 00107 00108 #define LWIP_PLATFORM_BYTESWAP 1 00109 00110 #if LWIP_TRANSPORT_ETHERNET 00111 00112 /* MSS should match the hardware packet size */ 00113 #define TCP_MSS 1460 00114 #define TCP_SND_BUF (2 * TCP_MSS) 00115 #define TCP_WND (2 * TCP_MSS) 00116 #define TCP_SND_QUEUELEN (2 * TCP_SND_BUF/TCP_MSS) 00117 00118 // Broadcast 00119 #define IP_SOF_BROADCAST 1 00120 #define IP_SOF_BROADCAST_RECV 1 00121 00122 #define LWIP_BROADCAST_PING 1 00123 00124 #define LWIP_CHECKSUM_ON_COPY 1 00125 00126 #define LWIP_NETIF_HOSTNAME 1 00127 #define LWIP_NETIF_STATUS_CALLBACK 1 00128 #define LWIP_NETIF_LINK_CALLBACK 1 00129 00130 #elif LWIP_TRANSPORT_PPP 00131 00132 #define TCP_SND_BUF (3 * 536) 00133 #define TCP_WND (2 * 536) 00134 00135 #define LWIP_ARP 0 00136 00137 #define PPP_SUPPORT 1 00138 #define CHAP_SUPPORT 1 00139 #define PAP_SUPPORT 1 00140 #define PPP_THREAD_STACKSIZE 4*192 00141 #define PPP_THREAD_PRIO 0 00142 00143 #define MAXNAMELEN 64 /* max length of hostname or name for auth */ 00144 #define MAXSECRETLEN 64 00145 00146 #else 00147 #error A transport mechanism (Ethernet or PPP) must be defined 00148 #endif 00149 00150 #endif /* LWIPOPTS_H_ */
Generated on Tue Jul 12 2022 23:10:19 by
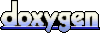