
MPR121 demo code for 12 key Sparkfun touch keypad - sends key number to LEDs. Based on Sparkfun MPR121 code ported to mbed by Anthony Buckton
mpr121.cpp
00001 /* 00002 Copyright (c) 2011 Anthony Buckton (abuckton [at] blackink [dot} net {dot} au) 00003 00004 Permission is hereby granted, free of charge, to any person obtaining a copy 00005 of this software and associated documentation files (the "Software"), to deal 00006 in the Software without restriction, including without limitation the rights 00007 to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00008 copies of the Software, and to permit persons to whom the Software is 00009 furnished to do so, subject to the following conditions: 00010 00011 The above copyright notice and this permission notice shall be included in 00012 all copies or substantial portions of the Software. 00013 00014 THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00015 IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00016 FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00017 AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00018 LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00019 OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00020 THE SOFTWARE. 00021 */ 00022 00023 #include <mbed.h> 00024 #include <sstream> 00025 #include <string> 00026 #include <list> 00027 00028 #include <mpr121.h> 00029 00030 Mpr121::Mpr121(I2C *i2c, Address i2cAddress) 00031 { 00032 this->i2c = i2c; 00033 00034 address = i2cAddress; 00035 00036 // Configure the MPR121 settings to default 00037 this->configureSettings(); 00038 } 00039 00040 00041 void Mpr121::configureSettings() 00042 { 00043 // Put the MPR into setup mode 00044 this->write(ELE_CFG,0x00); 00045 00046 // Electrode filters for when data is > baseline 00047 unsigned char gtBaseline[] = { 00048 0x01, //MHD_R 00049 0x01, //NHD_R 00050 0x00, //NCL_R 00051 0x00 //FDL_R 00052 }; 00053 00054 writeMany(MHD_R,gtBaseline,4); 00055 00056 // Electrode filters for when data is < baseline 00057 unsigned char ltBaseline[] = { 00058 0x01, //MHD_F 00059 0x01, //NHD_F 00060 0xFF, //NCL_F 00061 0x02 //FDL_F 00062 }; 00063 00064 writeMany(MHD_F,ltBaseline,4); 00065 00066 // Electrode touch and release thresholds 00067 unsigned char electrodeThresholds[] = { 00068 E_THR_T, // Touch Threshhold 00069 E_THR_R // Release Threshold 00070 }; 00071 00072 for(int i=0; i<12; i++){ 00073 int result = writeMany((ELE0_T+(i*2)),electrodeThresholds,2); 00074 } 00075 00076 // Proximity Settings 00077 unsigned char proximitySettings[] = { 00078 0xff, //MHD_Prox_R 00079 0xff, //NHD_Prox_R 00080 0x00, //NCL_Prox_R 00081 0x00, //FDL_Prox_R 00082 0x01, //MHD_Prox_F 00083 0x01, //NHD_Prox_F 00084 0xFF, //NCL_Prox_F 00085 0xff, //FDL_Prox_F 00086 0x00, //NHD_Prox_T 00087 0x00, //NCL_Prox_T 00088 0x00 //NFD_Prox_T 00089 }; 00090 writeMany(MHDPROXR,proximitySettings,11); 00091 00092 unsigned char proxThresh[] = { 00093 PROX_THR_T, // Touch Threshold 00094 PROX_THR_R // Release Threshold 00095 }; 00096 writeMany(EPROXTTH,proxThresh,2); 00097 00098 this->write(FIL_CFG,0x04); 00099 00100 // Set the electrode config to transition to active mode 00101 this->write(ELE_CFG,0x0c); 00102 } 00103 00104 void Mpr121::setElectrodeThreshold(int electrode, unsigned char touch, unsigned char release){ 00105 00106 if(electrode > 11) return; 00107 00108 // Get the current mode 00109 unsigned char mode = this->read(ELE_CFG); 00110 00111 // Put the MPR into setup mode 00112 this->write(ELE_CFG,0x00); 00113 00114 // Write the new threshold 00115 this->write((ELE0_T+(electrode*2)), touch); 00116 this->write((ELE0_T+(electrode*2)+1), release); 00117 00118 //Restore the operating mode 00119 this->write(ELE_CFG, mode); 00120 } 00121 00122 00123 unsigned char Mpr121::read(int key){ 00124 00125 unsigned char data[2]; 00126 00127 //Start the command 00128 i2c->start(); 00129 00130 // Address the target (Write mode) 00131 int ack1= i2c->write(address); 00132 00133 // Set the register key to read 00134 int ack2 = i2c->write(key); 00135 00136 // Re-start for read of data 00137 i2c->start(); 00138 00139 // Re-send the target address in read mode 00140 int ack3 = i2c->write(address+1); 00141 00142 // Read in the result 00143 data[0] = i2c->read(0); 00144 00145 // Reset the bus 00146 i2c->stop(); 00147 00148 return data[0]; 00149 } 00150 00151 00152 int Mpr121::write(int key, unsigned char value){ 00153 00154 //Start the command 00155 i2c->start(); 00156 00157 // Address the target (Write mode) 00158 int ack1= i2c->write(address); 00159 00160 // Set the register key to write 00161 int ack2 = i2c->write(key); 00162 00163 // Read in the result 00164 int ack3 = i2c->write(value); 00165 00166 // Reset the bus 00167 i2c->stop(); 00168 00169 return (ack1+ack2+ack3)-3; 00170 } 00171 00172 00173 int Mpr121::writeMany(int start, unsigned char* dataSet, int length){ 00174 //Start the command 00175 i2c->start(); 00176 00177 // Address the target (Write mode) 00178 int ack= i2c->write(address); 00179 if(ack!=1){ 00180 return -1; 00181 } 00182 00183 // Set the register key to write 00184 ack = i2c->write(start); 00185 if(ack!=1){ 00186 return -1; 00187 } 00188 00189 // Write the date set 00190 int count = 0; 00191 while(ack==1 && (count < length)){ 00192 ack = i2c->write(dataSet[count]); 00193 count++; 00194 } 00195 // Stop the cmd 00196 i2c->stop(); 00197 00198 return count; 00199 } 00200 00201 00202 bool Mpr121::getProximityMode(){ 00203 if(this->read(ELE_CFG) > 0x0c) 00204 return true; 00205 else 00206 return false; 00207 } 00208 00209 void Mpr121::setProximityMode(bool mode){ 00210 this->write(ELE_CFG,0x00); 00211 if(mode){ 00212 this->write(ELE_CFG,0x30); //Sense proximity from ALL pads 00213 } else { 00214 this->write(ELE_CFG,0x0c); //Sense touch, all 12 pads active. 00215 } 00216 } 00217 00218 00219 int Mpr121::readTouchData(){ 00220 return this->read(0x00); 00221 }
Generated on Wed Jul 13 2022 06:51:45 by
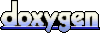