
MPR121 demo code for 12 key Sparkfun touch keypad - sends key number to LEDs. Based on Sparkfun MPR121 code ported to mbed by Anthony Buckton
main.cpp
00001 /* 00002 Copyright (c) 2011 Anthony Buckton (abuckton [at] blackink [dot} net {dot} au) 00003 00004 Permission is hereby granted, free of charge, to any person obtaining a copy 00005 of this software and associated documentation files (the "Software"), to deal 00006 in the Software without restriction, including without limitation the rights 00007 to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00008 copies of the Software, and to permit persons to whom the Software is 00009 furnished to do so, subject to the following conditions: 00010 00011 The above copyright notice and this permission notice shall be included in 00012 all copies or substantial portions of the Software. 00013 00014 THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00015 IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00016 FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00017 AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00018 LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00019 OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00020 THE SOFTWARE. 00021 */ 00022 00023 #include <mbed.h> 00024 #include <string> 00025 #include <list> 00026 00027 #include <mpr121.h> 00028 00029 DigitalOut led1(LED1); 00030 DigitalOut led2(LED2); 00031 DigitalOut led3(LED3); 00032 DigitalOut led4(LED4); 00033 00034 // Create the interrupt receiver object on pin 26 00035 InterruptIn interrupt(p26); 00036 00037 // Setup the Serial to the PC for debugging 00038 Serial pc(USBTX, USBRX); 00039 00040 // Setup the i2c bus on pins 28 and 27 00041 I2C i2c(p9, p10); 00042 00043 // Setup the Mpr121: 00044 // constructor(i2c object, i2c address of the mpr121) 00045 Mpr121 mpr121(&i2c, Mpr121::ADD_VSS); 00046 00047 void fallInterrupt() { 00048 int key_code=0; 00049 int i=0; 00050 int value=mpr121.read(0x00); 00051 value +=mpr121.read(0x01)<<8; 00052 // LED demo mod by J. Hamblen 00053 //pc.printf("MPR value: %x \r\n", value); 00054 i=0; 00055 // puts key number out to LEDs for demo 00056 for (i=0; i<12; i++) { 00057 if (((value>>i)&0x01)==1) key_code=i+1; 00058 } 00059 led4=key_code & 0x01; 00060 led3=(key_code>>1) & 0x01; 00061 led2=(key_code>>2) & 0x01; 00062 led1=(key_code>>3) & 0x01; 00063 } 00064 int main() { 00065 00066 pc.printf("\nHello from the mbed & mpr121\n\r"); 00067 00068 unsigned char dataArray[2]; 00069 int key; 00070 int count = 0; 00071 00072 pc.printf("Test 1: read a value: \r\n"); 00073 dataArray[0] = mpr121.read(AFE_CFG); 00074 pc.printf("Read value=%x\r\n\n",dataArray[0]); 00075 00076 pc.printf("Test 2: read a value: \r\n"); 00077 dataArray[0] = mpr121.read(0x5d); 00078 pc.printf("Read value=%x\r\n\n",dataArray[0]); 00079 00080 pc.printf("Test 3: write & read a value: \r\n"); 00081 mpr121.read(ELE0_T); 00082 mpr121.write(ELE0_T,0x22); 00083 dataArray[0] = mpr121.read(ELE0_T); 00084 pc.printf("Read value=%x\r\n\n",dataArray[0]); 00085 00086 pc.printf("Test 4: Write many values: \r\n"); 00087 unsigned char data[] = {0x1,0x3,0x5,0x9,0x15,0x25,0x41}; 00088 mpr121.writeMany(0x42,data,7); 00089 00090 // Now read them back .. 00091 key = 0x42; 00092 count = 0; 00093 while (count < 7) { 00094 char result = mpr121.read(key); 00095 key++; 00096 count++; 00097 pc.printf("Read value: '%x'=%x\n\r",key,result); 00098 } 00099 00100 pc.printf("Test 5: Read Electrodes:\r\n"); 00101 key = ELE0_T; 00102 count = 0; 00103 while (count < 24) { 00104 char result = mpr121.read(key); 00105 pc.printf("Read key:%x value:%x\n\r",key,result); 00106 key++; 00107 count++; 00108 } 00109 pc.printf("--------- \r\n\n"); 00110 00111 // mpr121.setProximityMode(true); 00112 00113 pc.printf("ELE_CFG=%x", mpr121.read(ELE_CFG)); 00114 00115 interrupt.fall(&fallInterrupt); 00116 interrupt.mode(PullUp); 00117 00118 while (1) { 00119 wait(5); 00120 pc.printf("."); 00121 } 00122 } 00123 00124 00125
Generated on Wed Jul 13 2022 06:51:45 by
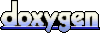