Library that implements the CellularInterface using PPP and LWIP on the mbed MCU. May be used on the C027 and C030 (non-N2xx flavour) boards from mbed 5.5 onwards.
Dependents: example-ublox-cellular-interface HelloMQTT example-ublox-cellular-interface_r410M example-ublox-mbed-client
UbloxPPPCellularInterface Class Reference
UbloxPPPCellularInterface class. More...
#include <UbloxPPPCellularInterface.h>
Public Member Functions | |
UbloxPPPCellularInterface (PinName tx=MDMTXD, PinName rx=MDMRXD, int baud=MBED_CONF_UBLOX_CELL_BAUD_RATE, bool debug_on=false) | |
Constructor. | |
virtual void | set_credentials (const char *apn, const char *uname=0, const char *pwd=0) |
Set the cellular network credentials. | |
virtual void | set_sim_pin (const char *sim_pin) |
Set the PIN code for the SIM card. | |
virtual nsapi_error_t | connect (const char *sim_pin, const char *apn=0, const char *uname=0, const char *pwd=0) |
Connect to the cellular network and start the interface. | |
virtual nsapi_error_t | connect () |
Attempt to connect to the cellular network. | |
virtual nsapi_error_t | disconnect () |
Attempt to disconnect from the network. | |
nsapi_error_t | set_sim_pin_check (bool set, bool immediate=false, const char *sim_pin=NULL) |
Adds or removes a SIM facility lock. | |
nsapi_error_t | set_new_sim_pin (const char *new_pin, bool immediate=false, const char *old_pin=NULL) |
Change the PIN for the SIM card. | |
virtual bool | is_connected () |
Check if the connection is currently established or not. | |
virtual const char * | get_ip_address () |
Get the local IP address. | |
virtual const char * | get_netmask () |
Get the local network mask. | |
virtual const char * | get_gateway () |
Get the local gateways. | |
void | connection_status_cb (Callback< void(nsapi_event_t, intptr_t)> cb) |
Call back in case connection is lost. | |
Protected Member Functions | |
virtual NetworkStack * | get_stack () |
Provide access to the underlying stack. | |
void | get_next_credentials (const char **config) |
Get the next set of credentials from the database. | |
Protected Attributes | |
const char * | _apn |
The APN to use. | |
const char * | _uname |
The user name to use. | |
const char * | _pwd |
The password to use. | |
bool | _ppp_connection_up |
True if the PPP connection is active, otherwise false. |
Detailed Description
UbloxPPPCellularInterface class.
This class implements the network stack interface into the cellular modems on the C030 and C027 boards for 2G/3G/4G modules using LWIP running on the mbed MCU, connected to the modem via PPP.
Definition at line 46 of file UbloxPPPCellularInterface.h.
Constructor & Destructor Documentation
UbloxPPPCellularInterface | ( | PinName | tx = MDMTXD , |
PinName | rx = MDMRXD , |
||
int | baud = MBED_CONF_UBLOX_CELL_BAUD_RATE , |
||
bool | debug_on = false |
||
) |
Constructor.
- Parameters:
-
tx the UART TX data pin to which the modem is attached. rx the UART RX data pin to which the modem is attached. baud the UART baud rate. debug_on true to switch AT interface debug on, otherwise false.
Definition at line 99 of file UbloxPPPCellularInterface.cpp.
Member Function Documentation
nsapi_error_t connect | ( | const char * | sim_pin, |
const char * | apn = 0 , |
||
const char * | uname = 0 , |
||
const char * | pwd = 0 |
||
) | [virtual] |
Connect to the cellular network and start the interface.
Attempts to connect to a cellular network. Note: if init() has not been called beforehand, connect() will call it first.
- Parameters:
-
sim_pin PIN for the SIM card. apn optionally, access point name. uname optionally, username. pwd optionally, password.
- Returns:
- NSAPI_ERROR_OK on success, or negative error code on failure.
Definition at line 142 of file UbloxPPPCellularInterface.cpp.
nsapi_error_t connect | ( | ) | [virtual] |
Attempt to connect to the cellular network.
Brings up the network interface. Connects to the cellular radio network and then brings up the underlying network stack to be used by the cellular modem over PPP interface. Note: if init() has not been called beforehand, connect() will call it first. NOTE: even a failed attempt to connect will cause the modem to remain powered up. To power it down, call deinit().
For APN setup, default behaviour is to use 'internet' as APN string and assuming no authentication is required, i.e., user name and password are not set. Optionally, a database lookup can be requested by turning on the APN database lookup feature. The APN database is by no means exhaustive. It contains a short list of some public APNs with publicly available user names and passwords (if required) in some particular countries only. Lookup is done using IMSI (International mobile subscriber identifier).
The preferred method is to setup APN using 'set_credentials()' API.
If you find that the AT interface returns "CONNECT" but shortly afterwards drops the connection then 99% of the time this will be because the APN is incorrect.
- Returns:
- 0 on success, negative error code on failure.
Definition at line 171 of file UbloxPPPCellularInterface.cpp.
void connection_status_cb | ( | Callback< void(nsapi_event_t, intptr_t)> | cb ) |
Call back in case connection is lost.
- Parameters:
-
fptr the function to call.
Definition at line 347 of file UbloxPPPCellularInterface.cpp.
nsapi_error_t disconnect | ( | ) | [virtual] |
Attempt to disconnect from the network.
Brings down the network interface. Shuts down the PPP interface of the underlying network stack. Does not bring down the Radio network.
- Returns:
- 0 on success, negative error code on failure.
Definition at line 248 of file UbloxPPPCellularInterface.cpp.
const char * get_gateway | ( | ) | [virtual] |
Get the local gateways.
- Returns:
- Null-terminated representation of the local gateway or null if no network mask has been received.
Definition at line 341 of file UbloxPPPCellularInterface.cpp.
const char * get_ip_address | ( | ) | [virtual] |
Get the local IP address.
- Returns:
- Null-terminated representation of the local IP address or null if no IP address has been received.
Definition at line 329 of file UbloxPPPCellularInterface.cpp.
const char * get_netmask | ( | ) | [virtual] |
Get the local network mask.
- Returns:
- Null-terminated representation of the local network mask or null if no network mask has been received.
Definition at line 335 of file UbloxPPPCellularInterface.cpp.
void get_next_credentials | ( | const char ** | config ) | [protected] |
Get the next set of credentials from the database.
Definition at line 81 of file UbloxPPPCellularInterface.cpp.
NetworkStack * get_stack | ( | ) | [protected, virtual] |
Provide access to the underlying stack.
- Returns:
- The underlying network stack.
Definition at line 75 of file UbloxPPPCellularInterface.cpp.
bool is_connected | ( | ) | [virtual] |
Check if the connection is currently established or not.
- Returns:
- true/false If the cellular module have successfully acquired a carrier and is connected to an external packet data network using PPP, true is returned, otherwise false is returned.
Definition at line 323 of file UbloxPPPCellularInterface.cpp.
void set_credentials | ( | const char * | apn, |
const char * | uname = 0 , |
||
const char * | pwd = 0 |
||
) | [virtual] |
Set the cellular network credentials.
Please check documentation of connect() for default behaviour of APN settings.
- Parameters:
-
apn Access point name. uname optionally, username. pwd optionally, password.
Definition at line 127 of file UbloxPPPCellularInterface.cpp.
nsapi_error_t set_new_sim_pin | ( | const char * | new_pin, |
bool | immediate = false , |
||
const char * | old_pin = NULL |
||
) |
Change the PIN for the SIM card.
Provide the new PIN for your SIM card with this API. It is ONLY possible to change the SIM PIN when SIM PIN checking is ENABLED.
- Parameters:
-
new_pin new PIN to be used in string format, must be a const. immediate if true, change the SIM PIN now, else set a flag and make the change only when connect() is called. If this is true and init() has not been called previously, it will be called first. old_pin old PIN, must be a const. If this is not provided, the SIM PIN must have previously been set by a call to set_sim_pin().
- Returns:
- 0 on success, negative error code on failure.
Definition at line 295 of file UbloxPPPCellularInterface.cpp.
void set_sim_pin | ( | const char * | sim_pin ) | [virtual] |
Set the PIN code for the SIM card.
- Parameters:
-
sim_pin PIN for the SIM card.
Definition at line 137 of file UbloxPPPCellularInterface.cpp.
nsapi_error_t set_sim_pin_check | ( | bool | set, |
bool | immediate = false , |
||
const char * | sim_pin = NULL |
||
) |
Adds or removes a SIM facility lock.
Can be used to enable or disable SIM PIN check at device startup.
- Parameters:
-
set can be set to true if the SIM PIN check is supposed to be enabled and vice versa. immediate if true, change the SIM PIN now, else set a flag and make the change only when connect() is called. If this is true and init() has not been called previously, it will be called first. sim_pin the current SIM PIN, must be a const. If this is not provided, the SIM PIN must have previously been set by a call to set_sim_pin().
- Returns:
- 0 on success, negative error code on failure.
Definition at line 267 of file UbloxPPPCellularInterface.cpp.
Field Documentation
const char* _apn [protected] |
The APN to use.
Definition at line 206 of file UbloxPPPCellularInterface.h.
bool _ppp_connection_up [protected] |
True if the PPP connection is active, otherwise false.
Definition at line 218 of file UbloxPPPCellularInterface.h.
const char* _pwd [protected] |
The password to use.
Definition at line 214 of file UbloxPPPCellularInterface.h.
const char* _uname [protected] |
The user name to use.
Definition at line 210 of file UbloxPPPCellularInterface.h.
Generated on Wed Jul 13 2022 20:24:27 by
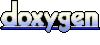