Library that implements the CellularInterface using PPP and LWIP on the mbed MCU. May be used on the C027 and C030 (non-N2xx flavour) boards from mbed 5.5 onwards.
Dependents: example-ublox-cellular-interface HelloMQTT example-ublox-cellular-interface_r410M example-ublox-mbed-client
UbloxPPPCellularInterface.h
00001 /* Copyright (c) 2017 ARM Limited 00002 * 00003 * Licensed under the Apache License, Version 2.0 (the "License"); 00004 * you may not use this file except in compliance with the License. 00005 * You may obtain a copy of the License at 00006 * 00007 * http://www.apache.org/licenses/LICENSE-2.0 00008 * 00009 * Unless required by applicable law or agreed to in writing, software 00010 * distributed under the License is distributed on an "AS IS" BASIS, 00011 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00012 * See the License for the specific language governing permissions and 00013 * limitations under the License. 00014 */ 00015 00016 #ifndef _UBLOX_PPP_CELLULAR_INTERFACE_ 00017 #define _UBLOX_PPP_CELLULAR_INTERFACE_ 00018 00019 #include "nsapi.h" 00020 #include "CellularBase.h" 00021 #include "NetworkStack.h" 00022 #include "InterruptIn.h" 00023 #include "UbloxCellularBase.h" 00024 00025 #if NSAPI_PPP_AVAILABLE 00026 00027 /********************************************************************** 00028 * CLASSES 00029 **********************************************************************/ 00030 00031 /* Forward declaration */ 00032 class NetworkStack; 00033 00034 /* 00035 * NOTE: order is important in the inheritance below! PAL takes this class 00036 * and casts it to CellularInterface and so CellularInterface has to be first 00037 * in the last for that to work. 00038 */ 00039 00040 /** UbloxPPPCellularInterface class. 00041 * 00042 * This class implements the network stack interface into the cellular 00043 * modems on the C030 and C027 boards for 2G/3G/4G modules using 00044 * LWIP running on the mbed MCU, connected to the modem via PPP. 00045 */ 00046 class UbloxPPPCellularInterface : public CellularBase, 00047 virtual public UbloxCellularBase { 00048 00049 public: 00050 /** Constructor. 00051 * 00052 * @param tx the UART TX data pin to which the modem is attached. 00053 * @param rx the UART RX data pin to which the modem is attached. 00054 * @param baud the UART baud rate. 00055 * @param debug_on true to switch AT interface debug on, otherwise false. 00056 */ 00057 UbloxPPPCellularInterface(PinName tx = MDMTXD, 00058 PinName rx = MDMRXD, 00059 int baud = MBED_CONF_UBLOX_CELL_BAUD_RATE, 00060 bool debug_on = false); 00061 00062 /* Destructor. 00063 */ 00064 virtual ~UbloxPPPCellularInterface(); 00065 00066 /** Set the cellular network credentials. 00067 * 00068 * Please check documentation of connect() for default behaviour of APN settings. 00069 * 00070 * @param apn Access point name. 00071 * @param uname optionally, username. 00072 * @param pwd optionally, password. 00073 */ 00074 virtual void set_credentials(const char *apn, const char *uname = 0, 00075 const char *pwd = 0); 00076 00077 /** Set the PIN code for the SIM card. 00078 * 00079 * @param sim_pin PIN for the SIM card. 00080 */ 00081 virtual void set_sim_pin(const char *sim_pin); 00082 00083 /** Connect to the cellular network and start the interface. 00084 * 00085 * Attempts to connect to a cellular network. Note: if init() has 00086 * not been called beforehand, connect() will call it first. 00087 * 00088 * @param sim_pin PIN for the SIM card. 00089 * @param apn optionally, access point name. 00090 * @param uname optionally, username. 00091 * @param pwd optionally, password. 00092 * @return NSAPI_ERROR_OK on success, or negative error code on failure. 00093 */ 00094 virtual nsapi_error_t connect(const char *sim_pin, const char *apn = 0, 00095 const char *uname = 0, const char *pwd = 0); 00096 00097 /** Attempt to connect to the cellular network. 00098 * 00099 * Brings up the network interface. Connects to the cellular radio 00100 * network and then brings up the underlying network stack to be used 00101 * by the cellular modem over PPP interface. Note: if init() has 00102 * not been called beforehand, connect() will call it first. 00103 * NOTE: even a failed attempt to connect will cause the modem to remain 00104 * powered up. To power it down, call deinit(). 00105 * 00106 * For APN setup, default behaviour is to use 'internet' as APN string and 00107 * assuming no authentication is required, i.e., user name and password are 00108 * not set. Optionally, a database lookup can be requested by turning on 00109 * the APN database lookup feature. The APN database is by no means exhaustive. 00110 * It contains a short list of some public APNs with publicly available 00111 * user names and passwords (if required) in some particular countries only. 00112 * Lookup is done using IMSI (International mobile subscriber identifier). 00113 * 00114 * The preferred method is to setup APN using 'set_credentials()' API. 00115 * 00116 * If you find that the AT interface returns "CONNECT" but shortly afterwards drops the connection 00117 * then 99% of the time this will be because the APN is incorrect. 00118 * 00119 * @return 0 on success, negative error code on failure. 00120 */ 00121 virtual nsapi_error_t connect(); 00122 00123 /** Attempt to disconnect from the network. 00124 * 00125 * Brings down the network interface. Shuts down the PPP interface 00126 * of the underlying network stack. Does not bring down the Radio network. 00127 * 00128 * @return 0 on success, negative error code on failure. 00129 */ 00130 virtual nsapi_error_t disconnect(); 00131 00132 /** Adds or removes a SIM facility lock. 00133 * 00134 * Can be used to enable or disable SIM PIN check at device startup. 00135 * 00136 * @param set can be set to true if the SIM PIN check is supposed 00137 * to be enabled and vice versa. 00138 * @param immediate if true, change the SIM PIN now, else set a flag 00139 * and make the change only when connect() is called. 00140 * If this is true and init() has not been called previously, 00141 * it will be called first. 00142 * @param sim_pin the current SIM PIN, must be a const. If this is not 00143 * provided, the SIM PIN must have previously been set by a 00144 * call to set_sim_pin(). 00145 * @return 0 on success, negative error code on failure. 00146 */ 00147 nsapi_error_t set_sim_pin_check(bool set, bool immediate = false, 00148 const char *sim_pin = NULL); 00149 00150 /** Change the PIN for the SIM card. 00151 * 00152 * Provide the new PIN for your SIM card with this API. It is ONLY possible to 00153 * change the SIM PIN when SIM PIN checking is ENABLED. 00154 * 00155 * @param new_pin new PIN to be used in string format, must be a const. 00156 * @param immediate if true, change the SIM PIN now, else set a flag 00157 * and make the change only when connect() is called. 00158 * If this is true and init() has not been called previously, 00159 * it will be called first. 00160 * @param old_pin old PIN, must be a const. If this is not provided, the SIM PIN 00161 * must have previously been set by a call to set_sim_pin(). 00162 * @return 0 on success, negative error code on failure. 00163 */ 00164 nsapi_error_t set_new_sim_pin(const char *new_pin, bool immediate = false, 00165 const char *old_pin = NULL); 00166 00167 /** Check if the connection is currently established or not. 00168 * 00169 * @return true/false If the cellular module have successfully acquired a carrier and is 00170 * connected to an external packet data network using PPP, true 00171 * is returned, otherwise false is returned. 00172 */ 00173 virtual bool is_connected(); 00174 00175 /** Get the local IP address 00176 * 00177 * @return Null-terminated representation of the local IP address 00178 * or null if no IP address has been received. 00179 */ 00180 virtual const char *get_ip_address(); 00181 00182 /** Get the local network mask. 00183 * 00184 * @return Null-terminated representation of the local network mask 00185 * or null if no network mask has been received. 00186 */ 00187 virtual const char *get_netmask(); 00188 00189 /** Get the local gateways. 00190 * 00191 * @return Null-terminated representation of the local gateway 00192 * or null if no network mask has been received. 00193 */ 00194 virtual const char *get_gateway(); 00195 00196 /** Call back in case connection is lost. 00197 * 00198 * @param fptr the function to call. 00199 */ 00200 void connection_status_cb(Callback<void(nsapi_event_t, intptr_t)> cb); 00201 00202 protected: 00203 00204 /** The APN to use. 00205 */ 00206 const char *_apn; 00207 00208 /** The user name to use. 00209 */ 00210 const char *_uname; 00211 00212 /** The password to use. 00213 */ 00214 const char *_pwd; 00215 00216 /** True if the PPP connection is active, otherwise false. 00217 */ 00218 bool _ppp_connection_up; 00219 00220 /** Provide access to the underlying stack. 00221 * 00222 * @return The underlying network stack. 00223 */ 00224 virtual NetworkStack *get_stack(); 00225 00226 /** Get the next set of credentials from the database. 00227 */ 00228 void get_next_credentials(const char ** config); 00229 00230 private: 00231 bool _sim_pin_check_change_pending; 00232 bool _sim_pin_check_change_pending_enabled_value; 00233 bool _sim_pin_change_pending; 00234 const char *_sim_pin_change_pending_new_pin_value; 00235 Callback<void(nsapi_event_t, intptr_t)> _connection_status_cb; 00236 nsapi_error_t setup_context_and_credentials(); 00237 bool set_atd(); 00238 }; 00239 00240 #endif // NSAPI_PPP_AVAILABLE 00241 #endif // _UBLOX_PPP_CELLULAR_INTERFACE_ 00242
Generated on Wed Jul 13 2022 20:24:27 by
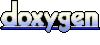