This class provides an API to assist with low power behaviour on an STM32F437 micro, as used on the u-blox C030 board. If you need to operate from battery for any significant period, or are mains powered and don't want to take the planet down with you, you should design your code with this in mind. This library uses the https://developer.mbed.org/users/Sissors/code/WakeUp/ library and so could be extended to support all of the MCUs that library supports.
Dependencies: WakeUp
Dependents: example-low-power-sleep aconnoCellularGnss
LowPower Class Reference
Low power library. More...
#include <low_power.h>
Public Member Functions | |
LowPower (void) | |
Constructor. | |
~LowPower (void) | |
Destructor. | |
void | exitDebugMode (void) |
Exit debug mode. | |
void | enterStop (uint32_t stopPeriodMilliseconds) |
Enter Stop mode. | |
void | enterStandby (uint32_t standbyPeriodMilliseconds, bool powerDownBackupSram=false) |
Enter Standby mode. | |
int32_t | numUserInterruptsEnabled (uint8_t *pList=NULL, uint32_t sizeOfList=0) |
Get the number of user interrupts that are enabled, sometimes helpful when debugging power-down modes. | |
Protected Member Functions | |
uint32_t | myNVIC_GetEnableIRQ (IRQn_Type IRQn) |
Check whether an interrupt is enabled or not. |
Detailed Description
Low power library.
Note: as it handles a hardware resource, there can only be one instance of this class; it is best to instantiate it statically at the top of your code or it can be instantiated once at the top of main().
Definition at line 42 of file low_power.h.
Constructor & Destructor Documentation
LowPower | ( | void | ) |
Constructor.
Definition at line 67 of file low_power.cpp.
~LowPower | ( | void | ) |
Destructor.
Definition at line 87 of file low_power.cpp.
Member Function Documentation
void enterStandby | ( | uint32_t | standbyPeriodMilliseconds, |
bool | powerDownBackupSram = false |
||
) |
Enter Standby mode.
Note that this function does NOT return. Or rather, if this function returns, there has been an error.
- Parameters:
-
standbyPeriodMilliseconds the amount of time to remain in Standby mode for. When the time has expired the processor will be reset and begin execution once more from main(). The values stored in BACKUP_SRAM will be retained, all other variables will be reset to their initial state. The RTOS is suspended on entry to Standby mode (i.e. no RTOS timers will run) and the RTOS will be reset on return to main(). The maximum delay is one calender month. It is up to the caller to ensure that the requested sleep time does not overflow the number of days in the current calender month. Note: Standby mode will only work on a standard mbed board if exitDebugMode has previously been called. Note: any enabled external interrupt can wake the processor from Standby mode, just as if the end of the period has expired. Note: if the watchdog is being used, the caller should set the watchdog timeout to longer period than the Standby period. powerDownBackupSram if true, backup SRAM will also be powered down in standby mode, otherwise it will be retained.
Definition at line 126 of file low_power.cpp.
void enterStop | ( | uint32_t | stopPeriodMilliseconds ) |
Enter Stop mode.
- Parameters:
-
stopPeriodMilliseconds the amount of time to remain in Stop mode for. When the time has expired the function will return. The maximum delay is one calender month. It is up to the caller to ensure that the requested sleep time does not overflow the number of days in the current calender month. This function will disable the RTOS tick and so any RTOS timers will be frozen for the duration of Stop mode; they will not tick and will not expire during this time. Any other enabled interrupts can bring the processor out of Stop mode. Note: during Stop mode the processor is running from a 32 kHz clock and so any interrupt that is triggered will run correspondingly slower. Note: if the watchdog is being used, the caller should set the watchdog timeout to longer period than the Stop period.
Definition at line 92 of file low_power.cpp.
void exitDebugMode | ( | void | ) |
Exit debug mode.
On a standard mbed board the host microcontroller is held in debug mode by the debug chip on the board. When the host microcontroller is in debug mode it cannot enter Standby mode normally. So to be able to use Standby mode, you must call this as the VERY FIRST THING you do on entry to main(); it will perform a soft reset of the microcontroller to cut the debug mode connection with the debug chip.
Definition at line 200 of file low_power.cpp.
uint32_t myNVIC_GetEnableIRQ | ( | IRQn_Type | IRQn ) | [protected] |
Check whether an interrupt is enabled or not.
Definition at line 53 of file low_power.cpp.
int32_t numUserInterruptsEnabled | ( | uint8_t * | pList = NULL , |
uint32_t | sizeOfList = 0 |
||
) |
Get the number of user interrupts that are enabled, sometimes helpful when debugging power-down modes.
User interrupts start at 0 and the number of them varies with the microcontroller.
- Parameters:
-
pList a pointer to an area in which the list of enabled user interrupts will be stored. sizeOfList the size of the memory pointer to be pList, in bytes.
- Returns:
- the number of enabled user interrupts.
Definition at line 168 of file low_power.cpp.
Generated on Tue Jul 12 2022 18:53:56 by
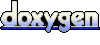