This class provides APIs to all of the registers of the TI BQ24295 battery charger chip, as used on the u-blox C030 board. This class is not required to charge a battery connected to the C030 board, charging will begin automatically when a battery is connected. This class is only required if the user wishes to monitor the charger's state or change the charger's settings. The caller should instantiate an I2C interface and pass this to init(), which will initialise the chip and place it into its lowest power state. The chip may then be configured using the API calls. Once the chip is configured, battery charging can be enabled. If battery charging is disabled the chip will once more be put into its lowest power state.
Dependents: example-battery-charger-bq24295 example-C030-out-of-box-demo example-C030-out-of-box-demo Amit
BatteryChargerBq24295 Class Reference
BQ27441 battery charger driver. More...
#include <battery_charger_bq24295.h>
Public Types | |
enum | ChargerState |
Charger state. More... | |
enum | ChargerFault { , CHARGER_FAULT_INPUT_FAULT = 0x10, CHARGER_FAULT_THERMAL_SHUTDOWN = 0x20, CHARGER_FAULT_CHARGE_TIMER_EXPIRED = 0x30 } |
Charger faults as a bitmap that matches the chip REG09 definitions. More... | |
Public Member Functions | |
BatteryChargerBq24295 (void) | |
Constructor. | |
~BatteryChargerBq24295 (void) | |
Destructor. | |
bool | init (I2C *pI2c, uint8_t address=BATTERY_CHARGER_BQ24295_ADDRESS) |
Initialise the BQ24295 chip. | |
ChargerState | getChargerState (void) |
Get the charger state. | |
bool | isExternalPowerPresent (void) |
Get whether external power is present or not. | |
bool | enableCharging (void) |
Enable charging. | |
bool | disableCharging (void) |
Disable charging. | |
bool | isChargingEnabled (void) |
Get the state of charging (enabled or disabled). | |
bool | enableOtg (void) |
Enable OTG charging. | |
bool | disableOtg (void) |
Disable OTG charging. | |
bool | isOtgEnabled (void) |
Determine whether OTG charging is enabled or not. | |
bool | setSystemVoltage (int32_t voltageMV) |
Set the system voltage (the voltage which the chip will attempt to maintain the system at). | |
bool | getSystemVoltage (int32_t *pVoltageMV) |
Get the system voltage. | |
bool | setFastChargingCurrentLimit (int32_t currentMA) |
Set the fast charging current limit. | |
bool | getFastChargingCurrentLimit (int32_t *pCurrentMA) |
Get the fast charging current limit. | |
bool | setFastChargingSafetyTimer (int32_t timerHours) |
Set the fast charging safety timer. | |
bool | getFastChargingSafetyTimer (int32_t *pTimerHours) |
Get the fast charging safety timer value. | |
bool | enableIcghIprechMargin (void) |
Set ICHG/IPRECH margin (see section 8.3.3.5 of the chip data sheet). | |
bool | disableIcghIprechMargin (void) |
Clear the ICHG/IPRECH margin (see section 8.3.3.5 of the chip data sheet). | |
bool | isIcghIprechMarginEnabled (void) |
Check if the ICHG/IPRECH margin is set (see section 8.3.3.5 of the chip data sheet). | |
bool | setChargingTerminationCurrent (int32_t currentMA) |
Set the charging termination current. | |
bool | getChargingTerminationCurrent (int32_t *pCurrentMA) |
Get the charging termination current. | |
bool | enableChargingTermination (void) |
Enable charging termination. | |
bool | disableChargingTermination (void) |
Disable charging termination. | |
bool | isChargingTerminationEnabled (void) |
Get the state of charging termination (enabled or disabled). | |
bool | setPrechargingCurrentLimit (int32_t currentMA) |
Set the pre-charging current limit. | |
bool | getPrechargingCurrentLimit (int32_t *pCurrentMA) |
Get the pre-charging current limit. | |
bool | setChargingVoltageLimit (int32_t voltageMV) |
Set the charging voltage limit. | |
bool | getChargingVoltageLimit (int32_t *pVoltageMV) |
Get the charging voltage limit. | |
bool | setFastChargingVoltageThreshold (int32_t voltageMV) |
Set the pre-charge to fast-charge voltage threshold. | |
bool | getFastChargingVoltageThreshold (int32_t *pVoltageMV) |
Get the pre-charge to fast-charge voltage threshold. | |
bool | setRechargingVoltageThreshold (int32_t voltageMV) |
Set the recharging voltage threshold. | |
bool | getRechargingVoltageThreshold (int32_t *pVoltageMV) |
Get the recharging voltage threshold. | |
bool | setBoostVoltage (int32_t voltageMV) |
Set the boost voltage. | |
bool | getBoostVoltage (int32_t *pVoltageMV) |
Get the boost voltage. | |
bool | setBoostUpperTemperatureLimit (int32_t temperatureC) |
Set the boost mode upper temperature limit. | |
bool | getBoostUpperTemperatureLimit (int32_t *pTemperatureC) |
Get the boost mode upper temperature limit. | |
bool | isBoostUpperTemperatureLimitEnabled (void) |
Check whether the boost mode upper temperature limit is enabled. | |
bool | disableBoostUpperTemperatureLimit (void) |
Disable the boost mode upper temperature limit. | |
bool | setBoostLowerTemperatureLimit (int32_t temperatureC) |
Set the boost mode low temperature limit. | |
bool | getBoostLowerTemperatureLimit (int32_t *pTemperatureC) |
Get the boost mode low temperature limit. | |
bool | setInputVoltageLimit (int32_t voltageMV) |
Set the input voltage limit. | |
bool | getInputVoltageLimit (int32_t *pVoltageMV) |
Get the input voltage limit. | |
bool | setInputCurrentLimit (int32_t currentMA) |
Set the input current limit. | |
bool | getInputCurrentLimit (int32_t *pCurrentMA) |
Get the input current limit. | |
bool | enableInputLimits (void) |
Enable input voltage and current limits. | |
bool | disableInputLimits (void) |
Remove any input voltage or current limits. | |
bool | areInputLimitsEnabled (void) |
Check whether input limits are enabled. | |
bool | setChipThermalRegulationThreshold (int32_t temperatureC) |
Set the thermal regulation threshold for the chip. | |
bool | getChipThermalRegulationThreshold (int32_t *pTemperatureC) |
Get the thermal regulation threshold for the chip. | |
char | getChargerFaults (void) |
Get the charger faults. | |
bool | feedWatchdog (void) |
Feed the watchdog timer. | |
bool | getWatchdog (int32_t *pWatchdogS) |
Get the watchdog timer of the BQ24295 chip. | |
bool | setWatchdog (int32_t watchdogS) |
Set the watchdog timer of the BQ24295 chip. | |
bool | enableShippingMode (void) |
Enable shipping mode. | |
bool | disableShippingMode (void) |
Disable shipping mode. | |
bool | isShippingModeEnabled (void) |
Check whether shipping mode is enabled. | |
bool | advancedGet (char address, char *pValue) |
Advanced function to read a register on the chip. | |
bool | advancedSet (char address, char value) |
Advanced function to set a register on the chip. | |
Protected Member Functions | |
bool | getRegister (char address, char *pValue) |
Read a register. | |
bool | setRegister (char address, char value) |
Set a register. | |
bool | setRegisterBits (char address, char mask) |
Set a mask of bits in a register. | |
bool | clearRegisterBits (char address, char mask) |
Clear a mask of bits in a register. | |
Protected Attributes | |
I2C * | gpI2c |
Pointer to the I2C interface. | |
uint8_t | gAddress |
The address of the device. | |
bool | gReady |
Flag to indicate device is ready. |
Detailed Description
BQ27441 battery charger driver.
Definition at line 37 of file battery_charger_bq24295.h.
Member Enumeration Documentation
enum ChargerFault |
Charger faults as a bitmap that matches the chip REG09 definitions.
- Enumerator:
Definition at line 52 of file battery_charger_bq24295.h.
enum ChargerState |
Charger state.
Definition at line 40 of file battery_charger_bq24295.h.
Constructor & Destructor Documentation
BatteryChargerBq24295 | ( | void | ) |
Constructor.
Definition at line 126 of file bq24295.cpp.
~BatteryChargerBq24295 | ( | void | ) |
Destructor.
Definition at line 133 of file bq24295.cpp.
Member Function Documentation
bool advancedGet | ( | char | address, |
char * | pValue | ||
) |
Advanced function to read a register on the chip.
- Parameters:
-
address the address to read from. pValue a place to put the returned value.
- Returns:
- true if successful, otherwise false.
Definition at line 1843 of file bq24295.cpp.
bool advancedSet | ( | char | address, |
char | value | ||
) |
Advanced function to set a register on the chip.
- Parameters:
-
address the address to write to. value the value to write.
- Returns:
- true if successful, otherwise false.
Definition at line 1868 of file bq24295.cpp.
bool areInputLimitsEnabled | ( | void | ) |
Check whether input limits are enabled.
- Returns:
- true if input limits are enabled, otherwise false.
Definition at line 1543 of file bq24295.cpp.
bool clearRegisterBits | ( | char | address, |
char | mask | ||
) | [protected] |
Clear a mask of bits in a register.
Note: gpI2c should be locked before this is called.
- Parameters:
-
address the address to write to. mask the mask of bits to clear.
- Returns:
- true if successful, otherwise false.
Definition at line 106 of file bq24295.cpp.
bool disableBoostUpperTemperatureLimit | ( | void | ) |
Disable the boost mode upper temperature limit.
Default is disabled.
- Returns:
- true if successful, otherwise false.
Definition at line 1253 of file bq24295.cpp.
bool disableCharging | ( | void | ) |
Disable charging.
Default is disabled.
- Returns:
- true if successful, otherwise false.
Definition at line 286 of file bq24295.cpp.
bool disableChargingTermination | ( | void | ) |
Disable charging termination.
Default is enabled.
- Returns:
- true if successful, otherwise false.
Definition at line 783 of file bq24295.cpp.
bool disableIcghIprechMargin | ( | void | ) |
Clear the ICHG/IPRECH margin (see section 8.3.3.5 of the chip data sheet).
Default is disabled.
- Returns:
- true if successful, otherwise false.
Definition at line 560 of file bq24295.cpp.
bool disableInputLimits | ( | void | ) |
Remove any input voltage or current limits.
Default is disabled.
- Returns:
- true if successful, otherwise false.
Definition at line 1523 of file bq24295.cpp.
bool disableOtg | ( | void | ) |
Disable OTG charging.
Default is enabled.
- Returns:
- true if successful, otherwise false.
Definition at line 354 of file bq24295.cpp.
bool disableShippingMode | ( | void | ) |
Disable shipping mode.
In shipping mode the battery is disconnected from the system to avoid leakage. Default is disabled.
- Returns:
- true if successful, otherwise false.
Definition at line 1789 of file bq24295.cpp.
bool enableCharging | ( | void | ) |
Enable charging.
Default is disabled.
- Returns:
- true if successful, otherwise false.
Definition at line 266 of file bq24295.cpp.
bool enableChargingTermination | ( | void | ) |
Enable charging termination.
Default is enabled.
- Returns:
- true if successful, otherwise false.
Definition at line 763 of file bq24295.cpp.
bool enableIcghIprechMargin | ( | void | ) |
Set ICHG/IPRECH margin (see section 8.3.3.5 of the chip data sheet).
Default is disabled.
- Returns:
- true if successful, otherwise false.
Definition at line 540 of file bq24295.cpp.
bool enableInputLimits | ( | void | ) |
Enable input voltage and current limits.
Default is disabled.
- Returns:
- true if successful, otherwise false.
Definition at line 1503 of file bq24295.cpp.
bool enableOtg | ( | void | ) |
Enable OTG charging.
Default is enabled.
- Returns:
- true if successful, otherwise false.
Definition at line 334 of file bq24295.cpp.
bool enableShippingMode | ( | void | ) |
Enable shipping mode.
In shipping mode the battery is disconnected from the system to avoid leakage. Default is disabled.
- Returns:
- true if successful, otherwise false.
Definition at line 1766 of file bq24295.cpp.
bool feedWatchdog | ( | void | ) |
Feed the watchdog timer.
Use this if it is necessary to keep the BQ24295 chip in Host mode (see section 8.4.1 of the data sheet for details).
- Returns:
- true if successful, otherwise false.
Definition at line 1669 of file bq24295.cpp.
bool getBoostLowerTemperatureLimit | ( | int32_t * | pTemperatureC ) |
Get the boost mode low temperature limit.
- Parameters:
-
pTemperatureC a place to put the temperature.
- Returns:
- true if successful, otherwise false.
Definition at line 1300 of file bq24295.cpp.
bool getBoostUpperTemperatureLimit | ( | int32_t * | pTemperatureC ) |
Get the boost mode upper temperature limit.
If the boost mode upper temperature limit is not enabled then pTemperatureC will remain untouched and false will be returned.
- Parameters:
-
pTemperatureC a place to put the temperature.
- Returns:
- true if successful and a limit was set, otherwise false.
Definition at line 1185 of file bq24295.cpp.
bool getBoostVoltage | ( | int32_t * | pVoltageMV ) |
Get the boost voltage.
- Parameters:
-
pVoltageMV a place to put the boost voltage, in milliVolts.
- Returns:
- true if successful, otherwise false.
Definition at line 1120 of file bq24295.cpp.
char getChargerFaults | ( | void | ) |
Get the charger faults.
Note: as with all the other API functions here, this should not be called from an interrupt function as the comms with the chip over I2C will take too long.
- Returns:
- a bit-map of that can be tested against ChargerFault.
Definition at line 1648 of file bq24295.cpp.
BatteryChargerBq24295::ChargerState getChargerState | ( | void | ) |
Get the charger state.
Note: on a u-blox C030 board with no battery connected this will report CHARGER_STATE_COMPLETE or CHARGER_STATE_FAST_CHARGE rather than CHARGER_STATE_NOT_CHARGING.
- Returns:
- the charge state.
Definition at line 170 of file bq24295.cpp.
bool getChargingTerminationCurrent | ( | int32_t * | pCurrentMA ) |
Get the charging termination current.
- Parameters:
-
pCurrentMA a place to put the charging termination current.
- Returns:
- true if successful, otherwise false.
Definition at line 734 of file bq24295.cpp.
bool getChargingVoltageLimit | ( | int32_t * | pVoltageMV ) |
Get the charging voltage limit.
- Parameters:
-
pVoltageMV a place to put the charging voltage limit, in milliVolts.
- Returns:
- true if successful, otherwise false.
Definition at line 933 of file bq24295.cpp.
bool getChipThermalRegulationThreshold | ( | int32_t * | pTemperatureC ) |
Get the thermal regulation threshold for the chip.
- Parameters:
-
pTemperatureC a place to put the temperature.
- Returns:
- true if successful, otherwise false.
TREG is in bits 0 & 1 of the boost voltage/thermal regulation control register
Definition at line 1608 of file bq24295.cpp.
bool getFastChargingCurrentLimit | ( | int32_t * | pCurrentMA ) |
Get the fast charging current limit.
- Parameters:
-
pCurrentMA a place to put the fast charging current limit.
- Returns:
- true if successful, otherwise false.
Definition at line 510 of file bq24295.cpp.
bool getFastChargingSafetyTimer | ( | int32_t * | pTimerHours ) |
Get the fast charging safety timer value.
- Parameters:
-
pTimerHours a place to put the charging safety timer value. Returned value is zero if the fast charging safety timer is disabled.
- Returns:
- true if charging termination is enabled, otherwise false.
Definition at line 653 of file bq24295.cpp.
bool getFastChargingVoltageThreshold | ( | int32_t * | pVoltageMV ) |
Get the pre-charge to fast-charge voltage threshold.
- Parameters:
-
pVoltageMV a place to put the threshold, in milliVolts.
- Returns:
- true if successful, otherwise false.
Definition at line 989 of file bq24295.cpp.
bool getInputCurrentLimit | ( | int32_t * | pCurrentMA ) |
Get the input current limit.
- Parameters:
-
pCurrentMA a place to put the input current limit.
- Returns:
- true if successful, otherwise false.
Definition at line 1449 of file bq24295.cpp.
bool getInputVoltageLimit | ( | int32_t * | pVoltageMV ) |
Get the input voltage limit.
- Parameters:
-
pVoltageMV a place to put the input voltage limit.
- Returns:
- true if successful, otherwise false.
Definition at line 1368 of file bq24295.cpp.
bool getPrechargingCurrentLimit | ( | int32_t * | pCurrentMA ) |
Get the pre-charging current limit.
- Parameters:
-
pCurrentMA a place to put the pre-charging current limit.
- Returns:
- true if successful, otherwise false.
Definition at line 867 of file bq24295.cpp.
bool getRechargingVoltageThreshold | ( | int32_t * | pVoltageMV ) |
Get the recharging voltage threshold.
- Parameters:
-
pVoltageMV a place to put the charging voltage threshold, in milliVolts.
- Returns:
- true if successful, otherwise false.
Definition at line 1047 of file bq24295.cpp.
bool getRegister | ( | char | address, |
char * | pValue | ||
) | [protected] |
Read a register.
Note: gpI2c should be locked before this is called.
- Parameters:
-
address the address to read from. pValue a place to put the returned value.
- Returns:
- true if successful, otherwise false.
Definition at line 45 of file bq24295.cpp.
bool getSystemVoltage | ( | int32_t * | pVoltageMV ) |
Get the system voltage.
- Parameters:
-
pVoltageMV a place to put the system voltage limit.
- Returns:
- true if successful, otherwise false.
Definition at line 444 of file bq24295.cpp.
bool getWatchdog | ( | int32_t * | pWatchdogS ) |
Get the watchdog timer of the BQ24295 chip.
- Parameters:
-
pWatchdogS a place to put the watchdog timer (in seconds).
- Returns:
- true if successful, otherwise false.
Definition at line 1690 of file bq24295.cpp.
bool init | ( | I2C * | pI2c, |
uint8_t | address = BATTERY_CHARGER_BQ24295_ADDRESS |
||
) |
Initialise the BQ24295 chip.
After initialisation the chip will be put into its lowest power state and should be configured if the default settings are not satisfactory. Note: the BQ24295 charging chip will automonously charge a LiPo cell that is connected to it, without host interaction. This class is only required where the configuration of the chip needs to be changed or the charger state is to be monitored.
- Parameters:
-
pI2c a pointer to the I2C instance to use. address 7-bit I2C address of the battery charger chip.
- Returns:
- true if successful, otherwise false.
Definition at line 138 of file bq24295.cpp.
bool isBoostUpperTemperatureLimitEnabled | ( | void | ) |
Check whether the boost mode upper temperature limit is enabled.
- Returns:
- true if successful, otherwise false.
Definition at line 1225 of file bq24295.cpp.
bool isChargingEnabled | ( | void | ) |
Get the state of charging (enabled or disabled).
- Returns:
- true if charging is enabled, otherwise false.
Definition at line 306 of file bq24295.cpp.
bool isChargingTerminationEnabled | ( | void | ) |
Get the state of charging termination (enabled or disabled).
- Returns:
- true if charging termination is enabled, otherwise false.
Definition at line 803 of file bq24295.cpp.
bool isExternalPowerPresent | ( | void | ) |
Get whether external power is present or not.
- Returns:
- true if external power is present, otherwise false.
Definition at line 237 of file bq24295.cpp.
bool isIcghIprechMarginEnabled | ( | void | ) |
Check if the ICHG/IPRECH margin is set (see section 8.3.3.5 of the chip data sheet).
- Returns:
- true if the ICHG/IPRECH margin is enabled, otherwise false.
Definition at line 580 of file bq24295.cpp.
bool isOtgEnabled | ( | void | ) |
Determine whether OTG charging is enabled or not.
- Returns:
- true if OTG charging is enabled, otherwise false.
Definition at line 374 of file bq24295.cpp.
bool isShippingModeEnabled | ( | void | ) |
Check whether shipping mode is enabled.
- Returns:
- true if input limits are enabled, otherwise false.
Definition at line 1812 of file bq24295.cpp.
bool setBoostLowerTemperatureLimit | ( | int32_t | temperatureC ) |
Set the boost mode low temperature limit.
- Parameters:
-
temperatureC the temperature in C. Values will be translated to the nearest (higher) of -10 C and -20 C, default is -10 C.
- Returns:
- true if successful, otherwise false.
Definition at line 1274 of file bq24295.cpp.
bool setBoostUpperTemperatureLimit | ( | int32_t | temperatureC ) |
Set the boost mode upper temperature limit.
- Parameters:
-
temperatureC the temperature in C. Values will be translated to the nearest (lower) of 55 C, 60 C and 65 C (disabled by default).
- Returns:
- true if successful, otherwise false.
Definition at line 1150 of file bq24295.cpp.
bool setBoostVoltage | ( | int32_t | voltageMV ) |
Set the boost voltage.
- Parameters:
-
voltageMV the boost voltage, in milliVolts. Range is 4550 mV to 5510 mV, default is 5126 mV.
- Returns:
- true if successful, otherwise false.
Definition at line 1079 of file bq24295.cpp.
bool setChargingTerminationCurrent | ( | int32_t | currentMA ) |
Set the charging termination current.
- Parameters:
-
currentMA the charging termination current, in milliAmps. Range is 128 mA to 2048 mA, default is 256 mA.
- Returns:
- true if successful, otherwise false.
Definition at line 698 of file bq24295.cpp.
bool setChargingVoltageLimit | ( | int32_t | voltageMV ) |
Set the charging voltage limit.
- Parameters:
-
voltageMV the charging voltage limit, in milliVolts. Range is 3504 mV to 4400 mV, default is 4208 mV.
- Returns:
- true if successful, otherwise false.
Definition at line 897 of file bq24295.cpp.
bool setChipThermalRegulationThreshold | ( | int32_t | temperatureC ) |
Set the thermal regulation threshold for the chip.
- Parameters:
-
temperatureC the temperature in C. Values will be translated to the nearest (lower) of 60 C, 80 C, 100 C and 120 C, default 120 C.
- Returns:
- true if successful, otherwise false.
Definition at line 1571 of file bq24295.cpp.
bool setFastChargingCurrentLimit | ( | int32_t | currentMA ) |
Set the fast charging current limit.
- Parameters:
-
currentMA the fast charging current limit, in milliAmps. Range is 512 mA to 3008 mA, default 1024 mA.
- Returns:
- true if successful, otherwise false.
Definition at line 474 of file bq24295.cpp.
bool setFastChargingSafetyTimer | ( | int32_t | timerHours ) |
Set the fast charging safety timer.
- Parameters:
-
timerHours the charging safety timer value. Use a value of 0 to indicate that the timer should be disabled. Timer values will be translated to the nearest (lower) value out of 5, 8, 12, and 20 hours, default 12 hours.
- Returns:
- true if successful, otherwise false.
Definition at line 608 of file bq24295.cpp.
bool setFastChargingVoltageThreshold | ( | int32_t | voltageMV ) |
Set the pre-charge to fast-charge voltage threshold.
- Parameters:
-
voltageMV the threshold, in milliVolts. Values will be translated to the nearest (highest) voltage out of 2800 mV and 3000 mV, default is 3000 mV.
- Returns:
- true if successful, otherwise false.
Definition at line 963 of file bq24295.cpp.
bool setInputCurrentLimit | ( | int32_t | currentMA ) |
Set the input current limit.
If the current drawn goes above this limit then charging will be ramped down. The limit does not take effect until enableInputLimits() is called (default setting is disabled).
- Parameters:
-
currentMA the input current limit, in milliAmps. Range is 100 mA to 3000 mA, default depends upon hardware configuration, see section 8.3.1.4.3 of the data sheet.
- Returns:
- true if successful, otherwise false.
Definition at line 1398 of file bq24295.cpp.
bool setInputVoltageLimit | ( | int32_t | voltageMV ) |
Set the input voltage limit.
If the input falls below this level then charging will be ramped down. The limit does not take effect until enableInputLimits() is called (default setting is disabled).
- Parameters:
-
voltageMV the input voltage limit, in milliVolts. Range is 3880 mV to 5080 mV, default is 4760 mV.
- Returns:
- true if successful, otherwise false.
Definition at line 1332 of file bq24295.cpp.
bool setPrechargingCurrentLimit | ( | int32_t | currentMA ) |
Set the pre-charging current limit.
- Parameters:
-
currentMA the pre-charging current limit, in milliAmps. Range is 128 mA to 2048 mA, default is 256 mA.
- Returns:
- true if successful, otherwise false.
Definition at line 831 of file bq24295.cpp.
bool setRechargingVoltageThreshold | ( | int32_t | voltageMV ) |
Set the recharging voltage threshold.
- Parameters:
-
voltageMV the recharging voltage threshold, in milliVolts. Values will be translated to the nearest (highest) voltage out of 100 mV and 300 mV, default is 100 mV.
- Returns:
- true if successful, otherwise false.
Definition at line 1021 of file bq24295.cpp.
bool setRegister | ( | char | address, |
char | value | ||
) | [protected] |
Set a register.
Note: gpI2c should be locked before this is called.
- Parameters:
-
address the address to write to. value the value to write.
- Returns:
- true if successful, otherwise false.
Definition at line 70 of file bq24295.cpp.
bool setRegisterBits | ( | char | address, |
char | mask | ||
) | [protected] |
Set a mask of bits in a register.
Note: gpI2c should be locked before this is called.
- Parameters:
-
address the address to write to. mask the mask of bits to set.
- Returns:
- true if successful, otherwise false.
Definition at line 90 of file bq24295.cpp.
bool setSystemVoltage | ( | int32_t | voltageMV ) |
Set the system voltage (the voltage which the chip will attempt to maintain the system at).
- Parameters:
-
voltageMV the voltage limit, in milliVolts. Range is 3000 mV to 3700 mV, default 3500 mV.
- Returns:
- true if successful, otherwise false.
Definition at line 402 of file bq24295.cpp.
bool setWatchdog | ( | int32_t | watchdogS ) |
Set the watchdog timer of the BQ24295 chip.
- Parameters:
-
watchdogS the watchdog timer (in seconds), 0 to disable, max 160 seconds.
- Returns:
- true if successful, otherwise false.
Definition at line 1731 of file bq24295.cpp.
Field Documentation
uint8_t gAddress [protected] |
The address of the device.
Definition at line 463 of file battery_charger_bq24295.h.
I2C* gpI2c [protected] |
Pointer to the I2C interface.
Definition at line 461 of file battery_charger_bq24295.h.
bool gReady [protected] |
Flag to indicate device is ready.
Definition at line 465 of file battery_charger_bq24295.h.
Generated on Thu Jul 14 2022 05:28:56 by
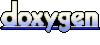