Board support library for C027
Dependents: IoTWorkshopLCD IoTWorkshopBuzzer IoTWorkshopSensors C027_USSDTest ... more
Fork of C027 by
C027.h
00001 /* Platform header file, for the u-blox C27-C20/U20/G35 00002 * mbed Internet of Things Starter Kit 00003 * http://mbed.org/platforms/u-blox-C027/ 00004 * 00005 */ 00006 00007 #pragma once 00008 00009 #include "mbed.h" 00010 00011 #if !defined(TARGET_UBLOX_C027) 00012 #warning "this library is indended to be used only with the C027 board" 00013 #else 00014 00015 //#define C027_REVA // select this define if you use revision A boards. 00016 00017 /** C027 Helper class to control the power supply and interface 00018 signals of the extended peripherials 00019 */ 00020 class C027 00021 { 00022 public: 00023 /** Constructor, this function initialized the control pins. 00024 */ 00025 C027(); 00026 00027 /** Enable the power supply of the Modem (MDM) 00028 */ 00029 void mdmPower(bool enable); 00030 00031 /** Activate the Usb Interface on LISA-C or LISA-U modems. For LISA-C this 00032 function must be called before its supply is enabled. 00033 \param enable true if supply should be truned on, false if off 00034 */ 00035 void mdmUsbEnable(bool enable); 00036 00037 /** Reset the Modem 00038 \attention use with care and only a last resort. 00039 */ 00040 void mdmReset(void); 00041 00042 /** Wakeup the Modem when in off mode. 00043 */ 00044 void mdmWakeup(void); 00045 00046 /** Some Modem can be commanded in sleep mode 00047 by setting the power pin low for a long period. 00048 */ 00049 void mdmSleep(void); 00050 00051 /** Enable the GPS Power supply 00052 \param enable true if supply should be truned on, false if off. 00053 */ 00054 void gpsPower(bool enable); 00055 00056 /** Reset the GPS 00057 \attention use with care and only a last resort. 00058 */ 00059 void gpsReset(void); 00060 00061 private: 00062 // modem pins 00063 DigitalOut mdmEn; //!< The modem LDO enable pin 00064 DigitalOut mdmRst; //!< The modem reset pin 00065 DigitalOut mdmPwrOn; //!< The power constrol pin of the modem 00066 DigitalOut mdmLvlOe; //!< The modem IO level shifter output enable (UART, GPIOs, active low) 00067 DigitalOut mdmILvlOe; //!< The modem I2C level shifter output enable (I2C, active high) 00068 DigitalOut mdmUsbDet; //!< The USB interface enable (active high) 00069 bool mdmIsEnabled; //!< Flag to remember if modem was enabled 00070 bool mdmUseUsb; //!< Flag to remember if USB pins were enabled 00071 // gps pins 00072 DigitalOut gpsEn; //!< The GPS LDO enable pin 00073 DigitalOut gpsRst; //!< The GPS reset pin 00074 bool gpsIsEnabled; //!< Flag to remember if GPS was enabled 00075 }; 00076 #endif
Generated on Tue Jul 12 2022 22:40:06 by
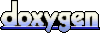