mbedtls ported to mbed-classic
Fork of mbedtls by
rsa.h File Reference
The RSA public-key cryptosystem. More...
Go to the source code of this file.
Data Structures | |
struct | mbedtls_rsa_context |
RSA context structure. More... | |
Functions | |
void | mbedtls_rsa_init (mbedtls_rsa_context *ctx, int padding, int hash_id) |
Initialize an RSA context. | |
void | mbedtls_rsa_set_padding (mbedtls_rsa_context *ctx, int padding, int hash_id) |
Set padding for an already initialized RSA context See mbedtls_rsa_init() for details. | |
int | mbedtls_rsa_gen_key (mbedtls_rsa_context *ctx, int(*f_rng)(void *, unsigned char *, size_t), void *p_rng, unsigned int nbits, int exponent) |
Generate an RSA keypair. | |
int | mbedtls_rsa_check_pubkey (const mbedtls_rsa_context *ctx) |
Check a public RSA key. | |
int | mbedtls_rsa_check_privkey (const mbedtls_rsa_context *ctx) |
Check a private RSA key. | |
int | mbedtls_rsa_check_pub_priv (const mbedtls_rsa_context *pub, const mbedtls_rsa_context *prv) |
Check a public-private RSA key pair. | |
int | mbedtls_rsa_public (mbedtls_rsa_context *ctx, const unsigned char *input, unsigned char *output) |
Do an RSA public key operation. | |
int | mbedtls_rsa_private (mbedtls_rsa_context *ctx, int(*f_rng)(void *, unsigned char *, size_t), void *p_rng, const unsigned char *input, unsigned char *output) |
Do an RSA private key operation. | |
int | mbedtls_rsa_pkcs1_encrypt (mbedtls_rsa_context *ctx, int(*f_rng)(void *, unsigned char *, size_t), void *p_rng, int mode, size_t ilen, const unsigned char *input, unsigned char *output) |
Generic wrapper to perform a PKCS#1 encryption using the mode from the context. | |
int | mbedtls_rsa_rsaes_pkcs1_v15_encrypt (mbedtls_rsa_context *ctx, int(*f_rng)(void *, unsigned char *, size_t), void *p_rng, int mode, size_t ilen, const unsigned char *input, unsigned char *output) |
Perform a PKCS#1 v1.5 encryption (RSAES-PKCS1-v1_5-ENCRYPT) | |
int | mbedtls_rsa_rsaes_oaep_encrypt (mbedtls_rsa_context *ctx, int(*f_rng)(void *, unsigned char *, size_t), void *p_rng, int mode, const unsigned char *label, size_t label_len, size_t ilen, const unsigned char *input, unsigned char *output) |
Perform a PKCS#1 v2.1 OAEP encryption (RSAES-OAEP-ENCRYPT) | |
int | mbedtls_rsa_pkcs1_decrypt (mbedtls_rsa_context *ctx, int(*f_rng)(void *, unsigned char *, size_t), void *p_rng, int mode, size_t *olen, const unsigned char *input, unsigned char *output, size_t output_max_len) |
Generic wrapper to perform a PKCS#1 decryption using the mode from the context. | |
int | mbedtls_rsa_rsaes_pkcs1_v15_decrypt (mbedtls_rsa_context *ctx, int(*f_rng)(void *, unsigned char *, size_t), void *p_rng, int mode, size_t *olen, const unsigned char *input, unsigned char *output, size_t output_max_len) |
Perform a PKCS#1 v1.5 decryption (RSAES-PKCS1-v1_5-DECRYPT) | |
int | mbedtls_rsa_rsaes_oaep_decrypt (mbedtls_rsa_context *ctx, int(*f_rng)(void *, unsigned char *, size_t), void *p_rng, int mode, const unsigned char *label, size_t label_len, size_t *olen, const unsigned char *input, unsigned char *output, size_t output_max_len) |
Perform a PKCS#1 v2.1 OAEP decryption (RSAES-OAEP-DECRYPT) | |
int | mbedtls_rsa_pkcs1_sign (mbedtls_rsa_context *ctx, int(*f_rng)(void *, unsigned char *, size_t), void *p_rng, int mode, mbedtls_md_type_t md_alg, unsigned int hashlen, const unsigned char *hash, unsigned char *sig) |
Generic wrapper to perform a PKCS#1 signature using the mode from the context. | |
int | mbedtls_rsa_rsassa_pkcs1_v15_sign (mbedtls_rsa_context *ctx, int(*f_rng)(void *, unsigned char *, size_t), void *p_rng, int mode, mbedtls_md_type_t md_alg, unsigned int hashlen, const unsigned char *hash, unsigned char *sig) |
Perform a PKCS#1 v1.5 signature (RSASSA-PKCS1-v1_5-SIGN) | |
int | mbedtls_rsa_rsassa_pss_sign (mbedtls_rsa_context *ctx, int(*f_rng)(void *, unsigned char *, size_t), void *p_rng, int mode, mbedtls_md_type_t md_alg, unsigned int hashlen, const unsigned char *hash, unsigned char *sig) |
Perform a PKCS#1 v2.1 PSS signature (RSASSA-PSS-SIGN) | |
int | mbedtls_rsa_pkcs1_verify (mbedtls_rsa_context *ctx, int(*f_rng)(void *, unsigned char *, size_t), void *p_rng, int mode, mbedtls_md_type_t md_alg, unsigned int hashlen, const unsigned char *hash, const unsigned char *sig) |
Generic wrapper to perform a PKCS#1 verification using the mode from the context. | |
int | mbedtls_rsa_rsassa_pkcs1_v15_verify (mbedtls_rsa_context *ctx, int(*f_rng)(void *, unsigned char *, size_t), void *p_rng, int mode, mbedtls_md_type_t md_alg, unsigned int hashlen, const unsigned char *hash, const unsigned char *sig) |
Perform a PKCS#1 v1.5 verification (RSASSA-PKCS1-v1_5-VERIFY) | |
int | mbedtls_rsa_rsassa_pss_verify (mbedtls_rsa_context *ctx, int(*f_rng)(void *, unsigned char *, size_t), void *p_rng, int mode, mbedtls_md_type_t md_alg, unsigned int hashlen, const unsigned char *hash, const unsigned char *sig) |
Perform a PKCS#1 v2.1 PSS verification (RSASSA-PSS-VERIFY) (This is the "simple" version.) | |
int | mbedtls_rsa_rsassa_pss_verify_ext (mbedtls_rsa_context *ctx, int(*f_rng)(void *, unsigned char *, size_t), void *p_rng, int mode, mbedtls_md_type_t md_alg, unsigned int hashlen, const unsigned char *hash, mbedtls_md_type_t mgf1_hash_id, int expected_salt_len, const unsigned char *sig) |
Perform a PKCS#1 v2.1 PSS verification (RSASSA-PSS-VERIFY) (This is the version with "full" options.) | |
int | mbedtls_rsa_copy (mbedtls_rsa_context *dst, const mbedtls_rsa_context *src) |
Copy the components of an RSA context. | |
void | mbedtls_rsa_free (mbedtls_rsa_context *ctx) |
Free the components of an RSA key. | |
int | mbedtls_rsa_self_test (int verbose) |
Checkup routine. |
Detailed Description
The RSA public-key cryptosystem.
Copyright (C) 2006-2015, ARM Limited, All Rights Reserved SPDX-License-Identifier: Apache-2.0
Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance with the License. You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software distributed under the License is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions and limitations under the License.
This file is part of mbed TLS (https://tls.mbed.org)
Definition in file rsa.h.
Function Documentation
int mbedtls_rsa_check_privkey | ( | const mbedtls_rsa_context * | ctx ) |
int mbedtls_rsa_check_pub_priv | ( | const mbedtls_rsa_context * | pub, |
const mbedtls_rsa_context * | prv | ||
) |
int mbedtls_rsa_check_pubkey | ( | const mbedtls_rsa_context * | ctx ) |
int mbedtls_rsa_copy | ( | mbedtls_rsa_context * | dst, |
const mbedtls_rsa_context * | src | ||
) |
void mbedtls_rsa_free | ( | mbedtls_rsa_context * | ctx ) |
int mbedtls_rsa_gen_key | ( | mbedtls_rsa_context * | ctx, |
int(*)(void *, unsigned char *, size_t) | f_rng, | ||
void * | p_rng, | ||
unsigned int | nbits, | ||
int | exponent | ||
) |
Generate an RSA keypair.
- Parameters:
-
ctx RSA context that will hold the key f_rng RNG function p_rng RNG parameter nbits size of the public key in bits exponent public exponent (e.g., 65537)
- Note:
- mbedtls_rsa_init() must be called beforehand to setup the RSA context.
- Returns:
- 0 if successful, or an MBEDTLS_ERR_RSA_XXX error code
void mbedtls_rsa_init | ( | mbedtls_rsa_context * | ctx, |
int | padding, | ||
int | hash_id | ||
) |
Initialize an RSA context.
Note: Set padding to MBEDTLS_RSA_PKCS_V21 for the RSAES-OAEP encryption scheme and the RSASSA-PSS signature scheme.
- Parameters:
-
ctx RSA context to be initialized padding MBEDTLS_RSA_PKCS_V15 or MBEDTLS_RSA_PKCS_V21 hash_id MBEDTLS_RSA_PKCS_V21 hash identifier
- Note:
- The hash_id parameter is actually ignored when using MBEDTLS_RSA_PKCS_V15 padding.
- Choice of padding mode is strictly enforced for private key operations, since there might be security concerns in mixing padding modes. For public key operations it's merely a default value, which can be overriden by calling specific rsa_rsaes_xxx or rsa_rsassa_xxx functions.
- The chosen hash is always used for OEAP encryption. For PSS signatures, it's always used for making signatures, but can be overriden (and always is, if set to MBEDTLS_MD_NONE) for verifying them.
int mbedtls_rsa_pkcs1_decrypt | ( | mbedtls_rsa_context * | ctx, |
int(*)(void *, unsigned char *, size_t) | f_rng, | ||
void * | p_rng, | ||
int | mode, | ||
size_t * | olen, | ||
const unsigned char * | input, | ||
unsigned char * | output, | ||
size_t | output_max_len | ||
) |
Generic wrapper to perform a PKCS#1 decryption using the mode from the context.
Do an RSA operation, then remove the message padding
- Parameters:
-
ctx RSA context f_rng RNG function (Only needed for MBEDTLS_RSA_PRIVATE) p_rng RNG parameter mode MBEDTLS_RSA_PUBLIC or MBEDTLS_RSA_PRIVATE olen will contain the plaintext length input buffer holding the encrypted data output buffer that will hold the plaintext output_max_len maximum length of the output buffer
- Returns:
- 0 if successful, or an MBEDTLS_ERR_RSA_XXX error code
- Note:
- The output buffer must be as large as the size of ctx->N (eg. 128 bytes if RSA-1024 is used) otherwise an error is thrown.
int mbedtls_rsa_pkcs1_encrypt | ( | mbedtls_rsa_context * | ctx, |
int(*)(void *, unsigned char *, size_t) | f_rng, | ||
void * | p_rng, | ||
int | mode, | ||
size_t | ilen, | ||
const unsigned char * | input, | ||
unsigned char * | output | ||
) |
Generic wrapper to perform a PKCS#1 encryption using the mode from the context.
Add the message padding, then do an RSA operation.
- Parameters:
-
ctx RSA context f_rng RNG function (Needed for padding and PKCS#1 v2.1 encoding and MBEDTLS_RSA_PRIVATE) p_rng RNG parameter mode MBEDTLS_RSA_PUBLIC or MBEDTLS_RSA_PRIVATE ilen contains the plaintext length input buffer holding the data to be encrypted output buffer that will hold the ciphertext
- Returns:
- 0 if successful, or an MBEDTLS_ERR_RSA_XXX error code
- Note:
- The output buffer must be as large as the size of ctx->N (eg. 128 bytes if RSA-1024 is used).
int mbedtls_rsa_pkcs1_sign | ( | mbedtls_rsa_context * | ctx, |
int(*)(void *, unsigned char *, size_t) | f_rng, | ||
void * | p_rng, | ||
int | mode, | ||
mbedtls_md_type_t | md_alg, | ||
unsigned int | hashlen, | ||
const unsigned char * | hash, | ||
unsigned char * | sig | ||
) |
Generic wrapper to perform a PKCS#1 signature using the mode from the context.
Do a private RSA operation to sign a message digest
- Parameters:
-
ctx RSA context f_rng RNG function (Needed for PKCS#1 v2.1 encoding and for MBEDTLS_RSA_PRIVATE) p_rng RNG parameter mode MBEDTLS_RSA_PUBLIC or MBEDTLS_RSA_PRIVATE md_alg a MBEDTLS_MD_XXX (use MBEDTLS_MD_NONE for signing raw data) hashlen message digest length (for MBEDTLS_MD_NONE only) hash buffer holding the message digest sig buffer that will hold the ciphertext
- Returns:
- 0 if the signing operation was successful, or an MBEDTLS_ERR_RSA_XXX error code
- Note:
- The "sig" buffer must be as large as the size of ctx->N (eg. 128 bytes if RSA-1024 is used).
- In case of PKCS#1 v2.1 encoding, see comments on
-
mbedtls_rsa_rsassa_pss_sign()
for details on md_alg and hash_id.
int mbedtls_rsa_pkcs1_verify | ( | mbedtls_rsa_context * | ctx, |
int(*)(void *, unsigned char *, size_t) | f_rng, | ||
void * | p_rng, | ||
int | mode, | ||
mbedtls_md_type_t | md_alg, | ||
unsigned int | hashlen, | ||
const unsigned char * | hash, | ||
const unsigned char * | sig | ||
) |
Generic wrapper to perform a PKCS#1 verification using the mode from the context.
Do a public RSA operation and check the message digest
- Parameters:
-
ctx points to an RSA public key f_rng RNG function (Only needed for MBEDTLS_RSA_PRIVATE) p_rng RNG parameter mode MBEDTLS_RSA_PUBLIC or MBEDTLS_RSA_PRIVATE md_alg a MBEDTLS_MD_XXX (use MBEDTLS_MD_NONE for signing raw data) hashlen message digest length (for MBEDTLS_MD_NONE only) hash buffer holding the message digest sig buffer holding the ciphertext
- Returns:
- 0 if the verify operation was successful, or an MBEDTLS_ERR_RSA_XXX error code
- Note:
- The "sig" buffer must be as large as the size of ctx->N (eg. 128 bytes if RSA-1024 is used).
-
In case of PKCS#1 v2.1 encoding, see comments on
mbedtls_rsa_rsassa_pss_verify()
about md_alg and hash_id.
int mbedtls_rsa_private | ( | mbedtls_rsa_context * | ctx, |
int(*)(void *, unsigned char *, size_t) | f_rng, | ||
void * | p_rng, | ||
const unsigned char * | input, | ||
unsigned char * | output | ||
) |
Do an RSA private key operation.
- Parameters:
-
ctx RSA context f_rng RNG function (Needed for blinding) p_rng RNG parameter input input buffer output output buffer
- Returns:
- 0 if successful, or an MBEDTLS_ERR_RSA_XXX error code
- Note:
- The input and output buffers must be large enough (eg. 128 bytes if RSA-1024 is used).
int mbedtls_rsa_public | ( | mbedtls_rsa_context * | ctx, |
const unsigned char * | input, | ||
unsigned char * | output | ||
) |
Do an RSA public key operation.
- Parameters:
-
ctx RSA context input input buffer output output buffer
- Returns:
- 0 if successful, or an MBEDTLS_ERR_RSA_XXX error code
- Note:
- This function does NOT take care of message padding. Also, be sure to set input[0] = 0 or assure that input is smaller than N.
- The input and output buffers must be large enough (eg. 128 bytes if RSA-1024 is used).
int mbedtls_rsa_rsaes_oaep_decrypt | ( | mbedtls_rsa_context * | ctx, |
int(*)(void *, unsigned char *, size_t) | f_rng, | ||
void * | p_rng, | ||
int | mode, | ||
const unsigned char * | label, | ||
size_t | label_len, | ||
size_t * | olen, | ||
const unsigned char * | input, | ||
unsigned char * | output, | ||
size_t | output_max_len | ||
) |
Perform a PKCS#1 v2.1 OAEP decryption (RSAES-OAEP-DECRYPT)
- Parameters:
-
ctx RSA context f_rng RNG function (Only needed for MBEDTLS_RSA_PRIVATE) p_rng RNG parameter mode MBEDTLS_RSA_PUBLIC or MBEDTLS_RSA_PRIVATE label buffer holding the custom label to use label_len contains the label length olen will contain the plaintext length input buffer holding the encrypted data output buffer that will hold the plaintext output_max_len maximum length of the output buffer
- Returns:
- 0 if successful, or an MBEDTLS_ERR_RSA_XXX error code
- Note:
- The output buffer must be as large as the size of ctx->N (eg. 128 bytes if RSA-1024 is used) otherwise an error is thrown.
int mbedtls_rsa_rsaes_oaep_encrypt | ( | mbedtls_rsa_context * | ctx, |
int(*)(void *, unsigned char *, size_t) | f_rng, | ||
void * | p_rng, | ||
int | mode, | ||
const unsigned char * | label, | ||
size_t | label_len, | ||
size_t | ilen, | ||
const unsigned char * | input, | ||
unsigned char * | output | ||
) |
Perform a PKCS#1 v2.1 OAEP encryption (RSAES-OAEP-ENCRYPT)
- Parameters:
-
ctx RSA context f_rng RNG function (Needed for padding and PKCS#1 v2.1 encoding and MBEDTLS_RSA_PRIVATE) p_rng RNG parameter mode MBEDTLS_RSA_PUBLIC or MBEDTLS_RSA_PRIVATE label buffer holding the custom label to use label_len contains the label length ilen contains the plaintext length input buffer holding the data to be encrypted output buffer that will hold the ciphertext
- Returns:
- 0 if successful, or an MBEDTLS_ERR_RSA_XXX error code
- Note:
- The output buffer must be as large as the size of ctx->N (eg. 128 bytes if RSA-1024 is used).
int mbedtls_rsa_rsaes_pkcs1_v15_decrypt | ( | mbedtls_rsa_context * | ctx, |
int(*)(void *, unsigned char *, size_t) | f_rng, | ||
void * | p_rng, | ||
int | mode, | ||
size_t * | olen, | ||
const unsigned char * | input, | ||
unsigned char * | output, | ||
size_t | output_max_len | ||
) |
Perform a PKCS#1 v1.5 decryption (RSAES-PKCS1-v1_5-DECRYPT)
- Parameters:
-
ctx RSA context f_rng RNG function (Only needed for MBEDTLS_RSA_PRIVATE) p_rng RNG parameter mode MBEDTLS_RSA_PUBLIC or MBEDTLS_RSA_PRIVATE olen will contain the plaintext length input buffer holding the encrypted data output buffer that will hold the plaintext output_max_len maximum length of the output buffer
- Returns:
- 0 if successful, or an MBEDTLS_ERR_RSA_XXX error code
- Note:
- The output buffer must be as large as the size of ctx->N (eg. 128 bytes if RSA-1024 is used) otherwise an error is thrown.
int mbedtls_rsa_rsaes_pkcs1_v15_encrypt | ( | mbedtls_rsa_context * | ctx, |
int(*)(void *, unsigned char *, size_t) | f_rng, | ||
void * | p_rng, | ||
int | mode, | ||
size_t | ilen, | ||
const unsigned char * | input, | ||
unsigned char * | output | ||
) |
Perform a PKCS#1 v1.5 encryption (RSAES-PKCS1-v1_5-ENCRYPT)
- Parameters:
-
ctx RSA context f_rng RNG function (Needed for padding and MBEDTLS_RSA_PRIVATE) p_rng RNG parameter mode MBEDTLS_RSA_PUBLIC or MBEDTLS_RSA_PRIVATE ilen contains the plaintext length input buffer holding the data to be encrypted output buffer that will hold the ciphertext
- Returns:
- 0 if successful, or an MBEDTLS_ERR_RSA_XXX error code
- Note:
- The output buffer must be as large as the size of ctx->N (eg. 128 bytes if RSA-1024 is used).
int mbedtls_rsa_rsassa_pkcs1_v15_sign | ( | mbedtls_rsa_context * | ctx, |
int(*)(void *, unsigned char *, size_t) | f_rng, | ||
void * | p_rng, | ||
int | mode, | ||
mbedtls_md_type_t | md_alg, | ||
unsigned int | hashlen, | ||
const unsigned char * | hash, | ||
unsigned char * | sig | ||
) |
Perform a PKCS#1 v1.5 signature (RSASSA-PKCS1-v1_5-SIGN)
- Parameters:
-
ctx RSA context f_rng RNG function (Only needed for MBEDTLS_RSA_PRIVATE) p_rng RNG parameter mode MBEDTLS_RSA_PUBLIC or MBEDTLS_RSA_PRIVATE md_alg a MBEDTLS_MD_XXX (use MBEDTLS_MD_NONE for signing raw data) hashlen message digest length (for MBEDTLS_MD_NONE only) hash buffer holding the message digest sig buffer that will hold the ciphertext
- Returns:
- 0 if the signing operation was successful, or an MBEDTLS_ERR_RSA_XXX error code
- Note:
- The "sig" buffer must be as large as the size of ctx->N (eg. 128 bytes if RSA-1024 is used).
int mbedtls_rsa_rsassa_pkcs1_v15_verify | ( | mbedtls_rsa_context * | ctx, |
int(*)(void *, unsigned char *, size_t) | f_rng, | ||
void * | p_rng, | ||
int | mode, | ||
mbedtls_md_type_t | md_alg, | ||
unsigned int | hashlen, | ||
const unsigned char * | hash, | ||
const unsigned char * | sig | ||
) |
Perform a PKCS#1 v1.5 verification (RSASSA-PKCS1-v1_5-VERIFY)
- Parameters:
-
ctx points to an RSA public key f_rng RNG function (Only needed for MBEDTLS_RSA_PRIVATE) p_rng RNG parameter mode MBEDTLS_RSA_PUBLIC or MBEDTLS_RSA_PRIVATE md_alg a MBEDTLS_MD_XXX (use MBEDTLS_MD_NONE for signing raw data) hashlen message digest length (for MBEDTLS_MD_NONE only) hash buffer holding the message digest sig buffer holding the ciphertext
- Returns:
- 0 if the verify operation was successful, or an MBEDTLS_ERR_RSA_XXX error code
- Note:
- The "sig" buffer must be as large as the size of ctx->N (eg. 128 bytes if RSA-1024 is used).
int mbedtls_rsa_rsassa_pss_sign | ( | mbedtls_rsa_context * | ctx, |
int(*)(void *, unsigned char *, size_t) | f_rng, | ||
void * | p_rng, | ||
int | mode, | ||
mbedtls_md_type_t | md_alg, | ||
unsigned int | hashlen, | ||
const unsigned char * | hash, | ||
unsigned char * | sig | ||
) |
Perform a PKCS#1 v2.1 PSS signature (RSASSA-PSS-SIGN)
- Parameters:
-
ctx RSA context f_rng RNG function (Needed for PKCS#1 v2.1 encoding and for MBEDTLS_RSA_PRIVATE) p_rng RNG parameter mode MBEDTLS_RSA_PUBLIC or MBEDTLS_RSA_PRIVATE md_alg a MBEDTLS_MD_XXX (use MBEDTLS_MD_NONE for signing raw data) hashlen message digest length (for MBEDTLS_MD_NONE only) hash buffer holding the message digest sig buffer that will hold the ciphertext
- Returns:
- 0 if the signing operation was successful, or an MBEDTLS_ERR_RSA_XXX error code
- Note:
- The "sig" buffer must be as large as the size of ctx->N (eg. 128 bytes if RSA-1024 is used).
- The hash_id in the RSA context is the one used for the encoding. md_alg in the function call is the type of hash that is encoded. According to RFC 3447 it is advised to keep both hashes the same.
int mbedtls_rsa_rsassa_pss_verify | ( | mbedtls_rsa_context * | ctx, |
int(*)(void *, unsigned char *, size_t) | f_rng, | ||
void * | p_rng, | ||
int | mode, | ||
mbedtls_md_type_t | md_alg, | ||
unsigned int | hashlen, | ||
const unsigned char * | hash, | ||
const unsigned char * | sig | ||
) |
Perform a PKCS#1 v2.1 PSS verification (RSASSA-PSS-VERIFY) (This is the "simple" version.)
- Parameters:
-
ctx points to an RSA public key f_rng RNG function (Only needed for MBEDTLS_RSA_PRIVATE) p_rng RNG parameter mode MBEDTLS_RSA_PUBLIC or MBEDTLS_RSA_PRIVATE md_alg a MBEDTLS_MD_XXX (use MBEDTLS_MD_NONE for signing raw data) hashlen message digest length (for MBEDTLS_MD_NONE only) hash buffer holding the message digest sig buffer holding the ciphertext
- Returns:
- 0 if the verify operation was successful, or an MBEDTLS_ERR_RSA_XXX error code
- Note:
- The "sig" buffer must be as large as the size of ctx->N (eg. 128 bytes if RSA-1024 is used).
- The hash_id in the RSA context is the one used for the verification. md_alg in the function call is the type of hash that is verified. According to RFC 3447 it is advised to keep both hashes the same. If hash_id in the RSA context is unset, the md_alg from the function call is used.
int mbedtls_rsa_rsassa_pss_verify_ext | ( | mbedtls_rsa_context * | ctx, |
int(*)(void *, unsigned char *, size_t) | f_rng, | ||
void * | p_rng, | ||
int | mode, | ||
mbedtls_md_type_t | md_alg, | ||
unsigned int | hashlen, | ||
const unsigned char * | hash, | ||
mbedtls_md_type_t | mgf1_hash_id, | ||
int | expected_salt_len, | ||
const unsigned char * | sig | ||
) |
Perform a PKCS#1 v2.1 PSS verification (RSASSA-PSS-VERIFY) (This is the version with "full" options.)
- Parameters:
-
ctx points to an RSA public key f_rng RNG function (Only needed for MBEDTLS_RSA_PRIVATE) p_rng RNG parameter mode MBEDTLS_RSA_PUBLIC or MBEDTLS_RSA_PRIVATE md_alg a MBEDTLS_MD_XXX (use MBEDTLS_MD_NONE for signing raw data) hashlen message digest length (for MBEDTLS_MD_NONE only) hash buffer holding the message digest mgf1_hash_id message digest used for mask generation expected_salt_len Length of the salt used in padding, use MBEDTLS_RSA_SALT_LEN_ANY to accept any salt length sig buffer holding the ciphertext
- Returns:
- 0 if the verify operation was successful, or an MBEDTLS_ERR_RSA_XXX error code
- Note:
- The "sig" buffer must be as large as the size of ctx->N (eg. 128 bytes if RSA-1024 is used).
- The hash_id in the RSA context is ignored.
int mbedtls_rsa_self_test | ( | int | verbose ) |
void mbedtls_rsa_set_padding | ( | mbedtls_rsa_context * | ctx, |
int | padding, | ||
int | hash_id | ||
) |
Set padding for an already initialized RSA context See mbedtls_rsa_init()
for details.
- Parameters:
-
ctx RSA context to be set padding MBEDTLS_RSA_PKCS_V15 or MBEDTLS_RSA_PKCS_V21 hash_id MBEDTLS_RSA_PKCS_V21 hash identifier
Generated on Tue Jul 12 2022 12:52:52 by
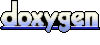