mbedtls ported to mbed-classic
Fork of mbedtls by
Embed:
(wiki syntax)
Show/hide line numbers
rsa.h
Go to the documentation of this file.
00001 /** 00002 * \file rsa.h 00003 * 00004 * \brief The RSA public-key cryptosystem 00005 * 00006 * Copyright (C) 2006-2015, ARM Limited, All Rights Reserved 00007 * SPDX-License-Identifier: Apache-2.0 00008 * 00009 * Licensed under the Apache License, Version 2.0 (the "License"); you may 00010 * not use this file except in compliance with the License. 00011 * You may obtain a copy of the License at 00012 * 00013 * http://www.apache.org/licenses/LICENSE-2.0 00014 * 00015 * Unless required by applicable law or agreed to in writing, software 00016 * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT 00017 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00018 * See the License for the specific language governing permissions and 00019 * limitations under the License. 00020 * 00021 * This file is part of mbed TLS (https://tls.mbed.org) 00022 */ 00023 #ifndef MBEDTLS_RSA_H 00024 #define MBEDTLS_RSA_H 00025 00026 #if !defined(MBEDTLS_CONFIG_FILE) 00027 #include "config.h" 00028 #else 00029 #include MBEDTLS_CONFIG_FILE 00030 #endif 00031 00032 #include "bignum.h" 00033 #include "md.h" 00034 00035 #if defined(MBEDTLS_THREADING_C) 00036 #include "threading.h" 00037 #endif 00038 00039 /* 00040 * RSA Error codes 00041 */ 00042 #define MBEDTLS_ERR_RSA_BAD_INPUT_DATA -0x4080 /**< Bad input parameters to function. */ 00043 #define MBEDTLS_ERR_RSA_INVALID_PADDING -0x4100 /**< Input data contains invalid padding and is rejected. */ 00044 #define MBEDTLS_ERR_RSA_KEY_GEN_FAILED -0x4180 /**< Something failed during generation of a key. */ 00045 #define MBEDTLS_ERR_RSA_KEY_CHECK_FAILED -0x4200 /**< Key failed to pass the library's validity check. */ 00046 #define MBEDTLS_ERR_RSA_PUBLIC_FAILED -0x4280 /**< The public key operation failed. */ 00047 #define MBEDTLS_ERR_RSA_PRIVATE_FAILED -0x4300 /**< The private key operation failed. */ 00048 #define MBEDTLS_ERR_RSA_VERIFY_FAILED -0x4380 /**< The PKCS#1 verification failed. */ 00049 #define MBEDTLS_ERR_RSA_OUTPUT_TOO_LARGE -0x4400 /**< The output buffer for decryption is not large enough. */ 00050 #define MBEDTLS_ERR_RSA_RNG_FAILED -0x4480 /**< The random generator failed to generate non-zeros. */ 00051 00052 /* 00053 * RSA constants 00054 */ 00055 #define MBEDTLS_RSA_PUBLIC 0 00056 #define MBEDTLS_RSA_PRIVATE 1 00057 00058 #define MBEDTLS_RSA_PKCS_V15 0 00059 #define MBEDTLS_RSA_PKCS_V21 1 00060 00061 #define MBEDTLS_RSA_SIGN 1 00062 #define MBEDTLS_RSA_CRYPT 2 00063 00064 #define MBEDTLS_RSA_SALT_LEN_ANY -1 00065 00066 /* 00067 * The above constants may be used even if the RSA module is compile out, 00068 * eg for alternative (PKCS#11) RSA implemenations in the PK layers. 00069 */ 00070 #if defined(MBEDTLS_RSA_C) 00071 00072 #ifdef __cplusplus 00073 extern "C" { 00074 #endif 00075 00076 /** 00077 * \brief RSA context structure 00078 */ 00079 typedef struct 00080 { 00081 int ver ; /*!< always 0 */ 00082 size_t len ; /*!< size(N) in chars */ 00083 00084 mbedtls_mpi N ; /*!< public modulus */ 00085 mbedtls_mpi E ; /*!< public exponent */ 00086 00087 mbedtls_mpi D ; /*!< private exponent */ 00088 mbedtls_mpi P ; /*!< 1st prime factor */ 00089 mbedtls_mpi Q ; /*!< 2nd prime factor */ 00090 mbedtls_mpi DP ; /*!< D % (P - 1) */ 00091 mbedtls_mpi DQ ; /*!< D % (Q - 1) */ 00092 mbedtls_mpi QP ; /*!< 1 / (Q % P) */ 00093 00094 mbedtls_mpi RN ; /*!< cached R^2 mod N */ 00095 mbedtls_mpi RP ; /*!< cached R^2 mod P */ 00096 mbedtls_mpi RQ ; /*!< cached R^2 mod Q */ 00097 00098 mbedtls_mpi Vi ; /*!< cached blinding value */ 00099 mbedtls_mpi Vf ; /*!< cached un-blinding value */ 00100 00101 int padding; /*!< MBEDTLS_RSA_PKCS_V15 for 1.5 padding and 00102 RSA_PKCS_v21 for OAEP/PSS */ 00103 int hash_id; /*!< Hash identifier of mbedtls_md_type_t as 00104 specified in the mbedtls_md.h header file 00105 for the EME-OAEP and EMSA-PSS 00106 encoding */ 00107 #if defined(MBEDTLS_THREADING_C) 00108 mbedtls_threading_mutex_t mutex ; /*!< Thread-safety mutex */ 00109 #endif 00110 } 00111 mbedtls_rsa_context; 00112 00113 /** 00114 * \brief Initialize an RSA context 00115 * 00116 * Note: Set padding to MBEDTLS_RSA_PKCS_V21 for the RSAES-OAEP 00117 * encryption scheme and the RSASSA-PSS signature scheme. 00118 * 00119 * \param ctx RSA context to be initialized 00120 * \param padding MBEDTLS_RSA_PKCS_V15 or MBEDTLS_RSA_PKCS_V21 00121 * \param hash_id MBEDTLS_RSA_PKCS_V21 hash identifier 00122 * 00123 * \note The hash_id parameter is actually ignored 00124 * when using MBEDTLS_RSA_PKCS_V15 padding. 00125 * 00126 * \note Choice of padding mode is strictly enforced for private key 00127 * operations, since there might be security concerns in 00128 * mixing padding modes. For public key operations it's merely 00129 * a default value, which can be overriden by calling specific 00130 * rsa_rsaes_xxx or rsa_rsassa_xxx functions. 00131 * 00132 * \note The chosen hash is always used for OEAP encryption. 00133 * For PSS signatures, it's always used for making signatures, 00134 * but can be overriden (and always is, if set to 00135 * MBEDTLS_MD_NONE) for verifying them. 00136 */ 00137 void mbedtls_rsa_init( mbedtls_rsa_context *ctx, 00138 int padding, 00139 int hash_id); 00140 00141 /** 00142 * \brief Set padding for an already initialized RSA context 00143 * See \c mbedtls_rsa_init() for details. 00144 * 00145 * \param ctx RSA context to be set 00146 * \param padding MBEDTLS_RSA_PKCS_V15 or MBEDTLS_RSA_PKCS_V21 00147 * \param hash_id MBEDTLS_RSA_PKCS_V21 hash identifier 00148 */ 00149 void mbedtls_rsa_set_padding( mbedtls_rsa_context *ctx, int padding, int hash_id); 00150 00151 /** 00152 * \brief Generate an RSA keypair 00153 * 00154 * \param ctx RSA context that will hold the key 00155 * \param f_rng RNG function 00156 * \param p_rng RNG parameter 00157 * \param nbits size of the public key in bits 00158 * \param exponent public exponent (e.g., 65537) 00159 * 00160 * \note mbedtls_rsa_init() must be called beforehand to setup 00161 * the RSA context. 00162 * 00163 * \return 0 if successful, or an MBEDTLS_ERR_RSA_XXX error code 00164 */ 00165 int mbedtls_rsa_gen_key( mbedtls_rsa_context *ctx, 00166 int (*f_rng)(void *, unsigned char *, size_t), 00167 void *p_rng, 00168 unsigned int nbits, int exponent ); 00169 00170 /** 00171 * \brief Check a public RSA key 00172 * 00173 * \param ctx RSA context to be checked 00174 * 00175 * \return 0 if successful, or an MBEDTLS_ERR_RSA_XXX error code 00176 */ 00177 int mbedtls_rsa_check_pubkey( const mbedtls_rsa_context *ctx ); 00178 00179 /** 00180 * \brief Check a private RSA key 00181 * 00182 * \param ctx RSA context to be checked 00183 * 00184 * \return 0 if successful, or an MBEDTLS_ERR_RSA_XXX error code 00185 */ 00186 int mbedtls_rsa_check_privkey( const mbedtls_rsa_context *ctx ); 00187 00188 /** 00189 * \brief Check a public-private RSA key pair. 00190 * Check each of the contexts, and make sure they match. 00191 * 00192 * \param pub RSA context holding the public key 00193 * \param prv RSA context holding the private key 00194 * 00195 * \return 0 if successful, or an MBEDTLS_ERR_RSA_XXX error code 00196 */ 00197 int mbedtls_rsa_check_pub_priv( const mbedtls_rsa_context *pub, const mbedtls_rsa_context *prv ); 00198 00199 /** 00200 * \brief Do an RSA public key operation 00201 * 00202 * \param ctx RSA context 00203 * \param input input buffer 00204 * \param output output buffer 00205 * 00206 * \return 0 if successful, or an MBEDTLS_ERR_RSA_XXX error code 00207 * 00208 * \note This function does NOT take care of message 00209 * padding. Also, be sure to set input[0] = 0 or assure that 00210 * input is smaller than N. 00211 * 00212 * \note The input and output buffers must be large 00213 * enough (eg. 128 bytes if RSA-1024 is used). 00214 */ 00215 int mbedtls_rsa_public( mbedtls_rsa_context *ctx, 00216 const unsigned char *input, 00217 unsigned char *output ); 00218 00219 /** 00220 * \brief Do an RSA private key operation 00221 * 00222 * \param ctx RSA context 00223 * \param f_rng RNG function (Needed for blinding) 00224 * \param p_rng RNG parameter 00225 * \param input input buffer 00226 * \param output output buffer 00227 * 00228 * \return 0 if successful, or an MBEDTLS_ERR_RSA_XXX error code 00229 * 00230 * \note The input and output buffers must be large 00231 * enough (eg. 128 bytes if RSA-1024 is used). 00232 */ 00233 int mbedtls_rsa_private( mbedtls_rsa_context *ctx, 00234 int (*f_rng)(void *, unsigned char *, size_t), 00235 void *p_rng, 00236 const unsigned char *input, 00237 unsigned char *output ); 00238 00239 /** 00240 * \brief Generic wrapper to perform a PKCS#1 encryption using the 00241 * mode from the context. Add the message padding, then do an 00242 * RSA operation. 00243 * 00244 * \param ctx RSA context 00245 * \param f_rng RNG function (Needed for padding and PKCS#1 v2.1 encoding 00246 * and MBEDTLS_RSA_PRIVATE) 00247 * \param p_rng RNG parameter 00248 * \param mode MBEDTLS_RSA_PUBLIC or MBEDTLS_RSA_PRIVATE 00249 * \param ilen contains the plaintext length 00250 * \param input buffer holding the data to be encrypted 00251 * \param output buffer that will hold the ciphertext 00252 * 00253 * \return 0 if successful, or an MBEDTLS_ERR_RSA_XXX error code 00254 * 00255 * \note The output buffer must be as large as the size 00256 * of ctx->N (eg. 128 bytes if RSA-1024 is used). 00257 */ 00258 int mbedtls_rsa_pkcs1_encrypt( mbedtls_rsa_context *ctx, 00259 int (*f_rng)(void *, unsigned char *, size_t), 00260 void *p_rng, 00261 int mode, size_t ilen, 00262 const unsigned char *input, 00263 unsigned char *output ); 00264 00265 /** 00266 * \brief Perform a PKCS#1 v1.5 encryption (RSAES-PKCS1-v1_5-ENCRYPT) 00267 * 00268 * \param ctx RSA context 00269 * \param f_rng RNG function (Needed for padding and MBEDTLS_RSA_PRIVATE) 00270 * \param p_rng RNG parameter 00271 * \param mode MBEDTLS_RSA_PUBLIC or MBEDTLS_RSA_PRIVATE 00272 * \param ilen contains the plaintext length 00273 * \param input buffer holding the data to be encrypted 00274 * \param output buffer that will hold the ciphertext 00275 * 00276 * \return 0 if successful, or an MBEDTLS_ERR_RSA_XXX error code 00277 * 00278 * \note The output buffer must be as large as the size 00279 * of ctx->N (eg. 128 bytes if RSA-1024 is used). 00280 */ 00281 int mbedtls_rsa_rsaes_pkcs1_v15_encrypt( mbedtls_rsa_context *ctx, 00282 int (*f_rng)(void *, unsigned char *, size_t), 00283 void *p_rng, 00284 int mode, size_t ilen, 00285 const unsigned char *input, 00286 unsigned char *output ); 00287 00288 /** 00289 * \brief Perform a PKCS#1 v2.1 OAEP encryption (RSAES-OAEP-ENCRYPT) 00290 * 00291 * \param ctx RSA context 00292 * \param f_rng RNG function (Needed for padding and PKCS#1 v2.1 encoding 00293 * and MBEDTLS_RSA_PRIVATE) 00294 * \param p_rng RNG parameter 00295 * \param mode MBEDTLS_RSA_PUBLIC or MBEDTLS_RSA_PRIVATE 00296 * \param label buffer holding the custom label to use 00297 * \param label_len contains the label length 00298 * \param ilen contains the plaintext length 00299 * \param input buffer holding the data to be encrypted 00300 * \param output buffer that will hold the ciphertext 00301 * 00302 * \return 0 if successful, or an MBEDTLS_ERR_RSA_XXX error code 00303 * 00304 * \note The output buffer must be as large as the size 00305 * of ctx->N (eg. 128 bytes if RSA-1024 is used). 00306 */ 00307 int mbedtls_rsa_rsaes_oaep_encrypt( mbedtls_rsa_context *ctx, 00308 int (*f_rng)(void *, unsigned char *, size_t), 00309 void *p_rng, 00310 int mode, 00311 const unsigned char *label, size_t label_len, 00312 size_t ilen, 00313 const unsigned char *input, 00314 unsigned char *output ); 00315 00316 /** 00317 * \brief Generic wrapper to perform a PKCS#1 decryption using the 00318 * mode from the context. Do an RSA operation, then remove 00319 * the message padding 00320 * 00321 * \param ctx RSA context 00322 * \param f_rng RNG function (Only needed for MBEDTLS_RSA_PRIVATE) 00323 * \param p_rng RNG parameter 00324 * \param mode MBEDTLS_RSA_PUBLIC or MBEDTLS_RSA_PRIVATE 00325 * \param olen will contain the plaintext length 00326 * \param input buffer holding the encrypted data 00327 * \param output buffer that will hold the plaintext 00328 * \param output_max_len maximum length of the output buffer 00329 * 00330 * \return 0 if successful, or an MBEDTLS_ERR_RSA_XXX error code 00331 * 00332 * \note The output buffer must be as large as the size 00333 * of ctx->N (eg. 128 bytes if RSA-1024 is used) otherwise 00334 * an error is thrown. 00335 */ 00336 int mbedtls_rsa_pkcs1_decrypt( mbedtls_rsa_context *ctx, 00337 int (*f_rng)(void *, unsigned char *, size_t), 00338 void *p_rng, 00339 int mode, size_t *olen, 00340 const unsigned char *input, 00341 unsigned char *output, 00342 size_t output_max_len ); 00343 00344 /** 00345 * \brief Perform a PKCS#1 v1.5 decryption (RSAES-PKCS1-v1_5-DECRYPT) 00346 * 00347 * \param ctx RSA context 00348 * \param f_rng RNG function (Only needed for MBEDTLS_RSA_PRIVATE) 00349 * \param p_rng RNG parameter 00350 * \param mode MBEDTLS_RSA_PUBLIC or MBEDTLS_RSA_PRIVATE 00351 * \param olen will contain the plaintext length 00352 * \param input buffer holding the encrypted data 00353 * \param output buffer that will hold the plaintext 00354 * \param output_max_len maximum length of the output buffer 00355 * 00356 * \return 0 if successful, or an MBEDTLS_ERR_RSA_XXX error code 00357 * 00358 * \note The output buffer must be as large as the size 00359 * of ctx->N (eg. 128 bytes if RSA-1024 is used) otherwise 00360 * an error is thrown. 00361 */ 00362 int mbedtls_rsa_rsaes_pkcs1_v15_decrypt( mbedtls_rsa_context *ctx, 00363 int (*f_rng)(void *, unsigned char *, size_t), 00364 void *p_rng, 00365 int mode, size_t *olen, 00366 const unsigned char *input, 00367 unsigned char *output, 00368 size_t output_max_len ); 00369 00370 /** 00371 * \brief Perform a PKCS#1 v2.1 OAEP decryption (RSAES-OAEP-DECRYPT) 00372 * 00373 * \param ctx RSA context 00374 * \param f_rng RNG function (Only needed for MBEDTLS_RSA_PRIVATE) 00375 * \param p_rng RNG parameter 00376 * \param mode MBEDTLS_RSA_PUBLIC or MBEDTLS_RSA_PRIVATE 00377 * \param label buffer holding the custom label to use 00378 * \param label_len contains the label length 00379 * \param olen will contain the plaintext length 00380 * \param input buffer holding the encrypted data 00381 * \param output buffer that will hold the plaintext 00382 * \param output_max_len maximum length of the output buffer 00383 * 00384 * \return 0 if successful, or an MBEDTLS_ERR_RSA_XXX error code 00385 * 00386 * \note The output buffer must be as large as the size 00387 * of ctx->N (eg. 128 bytes if RSA-1024 is used) otherwise 00388 * an error is thrown. 00389 */ 00390 int mbedtls_rsa_rsaes_oaep_decrypt( mbedtls_rsa_context *ctx, 00391 int (*f_rng)(void *, unsigned char *, size_t), 00392 void *p_rng, 00393 int mode, 00394 const unsigned char *label, size_t label_len, 00395 size_t *olen, 00396 const unsigned char *input, 00397 unsigned char *output, 00398 size_t output_max_len ); 00399 00400 /** 00401 * \brief Generic wrapper to perform a PKCS#1 signature using the 00402 * mode from the context. Do a private RSA operation to sign 00403 * a message digest 00404 * 00405 * \param ctx RSA context 00406 * \param f_rng RNG function (Needed for PKCS#1 v2.1 encoding and for 00407 * MBEDTLS_RSA_PRIVATE) 00408 * \param p_rng RNG parameter 00409 * \param mode MBEDTLS_RSA_PUBLIC or MBEDTLS_RSA_PRIVATE 00410 * \param md_alg a MBEDTLS_MD_XXX (use MBEDTLS_MD_NONE for signing raw data) 00411 * \param hashlen message digest length (for MBEDTLS_MD_NONE only) 00412 * \param hash buffer holding the message digest 00413 * \param sig buffer that will hold the ciphertext 00414 * 00415 * \return 0 if the signing operation was successful, 00416 * or an MBEDTLS_ERR_RSA_XXX error code 00417 * 00418 * \note The "sig" buffer must be as large as the size 00419 * of ctx->N (eg. 128 bytes if RSA-1024 is used). 00420 * 00421 * \note In case of PKCS#1 v2.1 encoding, see comments on 00422 * \note \c mbedtls_rsa_rsassa_pss_sign() for details on md_alg and hash_id. 00423 */ 00424 int mbedtls_rsa_pkcs1_sign( mbedtls_rsa_context *ctx, 00425 int (*f_rng)(void *, unsigned char *, size_t), 00426 void *p_rng, 00427 int mode, 00428 mbedtls_md_type_t md_alg, 00429 unsigned int hashlen, 00430 const unsigned char *hash, 00431 unsigned char *sig ); 00432 00433 /** 00434 * \brief Perform a PKCS#1 v1.5 signature (RSASSA-PKCS1-v1_5-SIGN) 00435 * 00436 * \param ctx RSA context 00437 * \param f_rng RNG function (Only needed for MBEDTLS_RSA_PRIVATE) 00438 * \param p_rng RNG parameter 00439 * \param mode MBEDTLS_RSA_PUBLIC or MBEDTLS_RSA_PRIVATE 00440 * \param md_alg a MBEDTLS_MD_XXX (use MBEDTLS_MD_NONE for signing raw data) 00441 * \param hashlen message digest length (for MBEDTLS_MD_NONE only) 00442 * \param hash buffer holding the message digest 00443 * \param sig buffer that will hold the ciphertext 00444 * 00445 * \return 0 if the signing operation was successful, 00446 * or an MBEDTLS_ERR_RSA_XXX error code 00447 * 00448 * \note The "sig" buffer must be as large as the size 00449 * of ctx->N (eg. 128 bytes if RSA-1024 is used). 00450 */ 00451 int mbedtls_rsa_rsassa_pkcs1_v15_sign( mbedtls_rsa_context *ctx, 00452 int (*f_rng)(void *, unsigned char *, size_t), 00453 void *p_rng, 00454 int mode, 00455 mbedtls_md_type_t md_alg, 00456 unsigned int hashlen, 00457 const unsigned char *hash, 00458 unsigned char *sig ); 00459 00460 /** 00461 * \brief Perform a PKCS#1 v2.1 PSS signature (RSASSA-PSS-SIGN) 00462 * 00463 * \param ctx RSA context 00464 * \param f_rng RNG function (Needed for PKCS#1 v2.1 encoding and for 00465 * MBEDTLS_RSA_PRIVATE) 00466 * \param p_rng RNG parameter 00467 * \param mode MBEDTLS_RSA_PUBLIC or MBEDTLS_RSA_PRIVATE 00468 * \param md_alg a MBEDTLS_MD_XXX (use MBEDTLS_MD_NONE for signing raw data) 00469 * \param hashlen message digest length (for MBEDTLS_MD_NONE only) 00470 * \param hash buffer holding the message digest 00471 * \param sig buffer that will hold the ciphertext 00472 * 00473 * \return 0 if the signing operation was successful, 00474 * or an MBEDTLS_ERR_RSA_XXX error code 00475 * 00476 * \note The "sig" buffer must be as large as the size 00477 * of ctx->N (eg. 128 bytes if RSA-1024 is used). 00478 * 00479 * \note The hash_id in the RSA context is the one used for the 00480 * encoding. md_alg in the function call is the type of hash 00481 * that is encoded. According to RFC 3447 it is advised to 00482 * keep both hashes the same. 00483 */ 00484 int mbedtls_rsa_rsassa_pss_sign( mbedtls_rsa_context *ctx, 00485 int (*f_rng)(void *, unsigned char *, size_t), 00486 void *p_rng, 00487 int mode, 00488 mbedtls_md_type_t md_alg, 00489 unsigned int hashlen, 00490 const unsigned char *hash, 00491 unsigned char *sig ); 00492 00493 /** 00494 * \brief Generic wrapper to perform a PKCS#1 verification using the 00495 * mode from the context. Do a public RSA operation and check 00496 * the message digest 00497 * 00498 * \param ctx points to an RSA public key 00499 * \param f_rng RNG function (Only needed for MBEDTLS_RSA_PRIVATE) 00500 * \param p_rng RNG parameter 00501 * \param mode MBEDTLS_RSA_PUBLIC or MBEDTLS_RSA_PRIVATE 00502 * \param md_alg a MBEDTLS_MD_XXX (use MBEDTLS_MD_NONE for signing raw data) 00503 * \param hashlen message digest length (for MBEDTLS_MD_NONE only) 00504 * \param hash buffer holding the message digest 00505 * \param sig buffer holding the ciphertext 00506 * 00507 * \return 0 if the verify operation was successful, 00508 * or an MBEDTLS_ERR_RSA_XXX error code 00509 * 00510 * \note The "sig" buffer must be as large as the size 00511 * of ctx->N (eg. 128 bytes if RSA-1024 is used). 00512 * 00513 * \note In case of PKCS#1 v2.1 encoding, see comments on 00514 * \c mbedtls_rsa_rsassa_pss_verify() about md_alg and hash_id. 00515 */ 00516 int mbedtls_rsa_pkcs1_verify( mbedtls_rsa_context *ctx, 00517 int (*f_rng)(void *, unsigned char *, size_t), 00518 void *p_rng, 00519 int mode, 00520 mbedtls_md_type_t md_alg, 00521 unsigned int hashlen, 00522 const unsigned char *hash, 00523 const unsigned char *sig ); 00524 00525 /** 00526 * \brief Perform a PKCS#1 v1.5 verification (RSASSA-PKCS1-v1_5-VERIFY) 00527 * 00528 * \param ctx points to an RSA public key 00529 * \param f_rng RNG function (Only needed for MBEDTLS_RSA_PRIVATE) 00530 * \param p_rng RNG parameter 00531 * \param mode MBEDTLS_RSA_PUBLIC or MBEDTLS_RSA_PRIVATE 00532 * \param md_alg a MBEDTLS_MD_XXX (use MBEDTLS_MD_NONE for signing raw data) 00533 * \param hashlen message digest length (for MBEDTLS_MD_NONE only) 00534 * \param hash buffer holding the message digest 00535 * \param sig buffer holding the ciphertext 00536 * 00537 * \return 0 if the verify operation was successful, 00538 * or an MBEDTLS_ERR_RSA_XXX error code 00539 * 00540 * \note The "sig" buffer must be as large as the size 00541 * of ctx->N (eg. 128 bytes if RSA-1024 is used). 00542 */ 00543 int mbedtls_rsa_rsassa_pkcs1_v15_verify( mbedtls_rsa_context *ctx, 00544 int (*f_rng)(void *, unsigned char *, size_t), 00545 void *p_rng, 00546 int mode, 00547 mbedtls_md_type_t md_alg, 00548 unsigned int hashlen, 00549 const unsigned char *hash, 00550 const unsigned char *sig ); 00551 00552 /** 00553 * \brief Perform a PKCS#1 v2.1 PSS verification (RSASSA-PSS-VERIFY) 00554 * (This is the "simple" version.) 00555 * 00556 * \param ctx points to an RSA public key 00557 * \param f_rng RNG function (Only needed for MBEDTLS_RSA_PRIVATE) 00558 * \param p_rng RNG parameter 00559 * \param mode MBEDTLS_RSA_PUBLIC or MBEDTLS_RSA_PRIVATE 00560 * \param md_alg a MBEDTLS_MD_XXX (use MBEDTLS_MD_NONE for signing raw data) 00561 * \param hashlen message digest length (for MBEDTLS_MD_NONE only) 00562 * \param hash buffer holding the message digest 00563 * \param sig buffer holding the ciphertext 00564 * 00565 * \return 0 if the verify operation was successful, 00566 * or an MBEDTLS_ERR_RSA_XXX error code 00567 * 00568 * \note The "sig" buffer must be as large as the size 00569 * of ctx->N (eg. 128 bytes if RSA-1024 is used). 00570 * 00571 * \note The hash_id in the RSA context is the one used for the 00572 * verification. md_alg in the function call is the type of 00573 * hash that is verified. According to RFC 3447 it is advised to 00574 * keep both hashes the same. If hash_id in the RSA context is 00575 * unset, the md_alg from the function call is used. 00576 */ 00577 int mbedtls_rsa_rsassa_pss_verify( mbedtls_rsa_context *ctx, 00578 int (*f_rng)(void *, unsigned char *, size_t), 00579 void *p_rng, 00580 int mode, 00581 mbedtls_md_type_t md_alg, 00582 unsigned int hashlen, 00583 const unsigned char *hash, 00584 const unsigned char *sig ); 00585 00586 /** 00587 * \brief Perform a PKCS#1 v2.1 PSS verification (RSASSA-PSS-VERIFY) 00588 * (This is the version with "full" options.) 00589 * 00590 * \param ctx points to an RSA public key 00591 * \param f_rng RNG function (Only needed for MBEDTLS_RSA_PRIVATE) 00592 * \param p_rng RNG parameter 00593 * \param mode MBEDTLS_RSA_PUBLIC or MBEDTLS_RSA_PRIVATE 00594 * \param md_alg a MBEDTLS_MD_XXX (use MBEDTLS_MD_NONE for signing raw data) 00595 * \param hashlen message digest length (for MBEDTLS_MD_NONE only) 00596 * \param hash buffer holding the message digest 00597 * \param mgf1_hash_id message digest used for mask generation 00598 * \param expected_salt_len Length of the salt used in padding, use 00599 * MBEDTLS_RSA_SALT_LEN_ANY to accept any salt length 00600 * \param sig buffer holding the ciphertext 00601 * 00602 * \return 0 if the verify operation was successful, 00603 * or an MBEDTLS_ERR_RSA_XXX error code 00604 * 00605 * \note The "sig" buffer must be as large as the size 00606 * of ctx->N (eg. 128 bytes if RSA-1024 is used). 00607 * 00608 * \note The hash_id in the RSA context is ignored. 00609 */ 00610 int mbedtls_rsa_rsassa_pss_verify_ext( mbedtls_rsa_context *ctx, 00611 int (*f_rng)(void *, unsigned char *, size_t), 00612 void *p_rng, 00613 int mode, 00614 mbedtls_md_type_t md_alg, 00615 unsigned int hashlen, 00616 const unsigned char *hash, 00617 mbedtls_md_type_t mgf1_hash_id, 00618 int expected_salt_len, 00619 const unsigned char *sig ); 00620 00621 /** 00622 * \brief Copy the components of an RSA context 00623 * 00624 * \param dst Destination context 00625 * \param src Source context 00626 * 00627 * \return 0 on success, 00628 * MBEDTLS_ERR_MPI_ALLOC_FAILED on memory allocation failure 00629 */ 00630 int mbedtls_rsa_copy( mbedtls_rsa_context *dst, const mbedtls_rsa_context *src ); 00631 00632 /** 00633 * \brief Free the components of an RSA key 00634 * 00635 * \param ctx RSA Context to free 00636 */ 00637 void mbedtls_rsa_free( mbedtls_rsa_context *ctx ); 00638 00639 /** 00640 * \brief Checkup routine 00641 * 00642 * \return 0 if successful, or 1 if the test failed 00643 */ 00644 int mbedtls_rsa_self_test( int verbose ); 00645 00646 #ifdef __cplusplus 00647 } 00648 #endif 00649 00650 #endif /* MBEDTLS_RSA_C */ 00651 00652 #endif /* rsa.h */
Generated on Tue Jul 12 2022 12:52:46 by
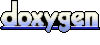