mbedtls ported to mbed-classic
Fork of mbedtls by
Embed:
(wiki syntax)
Show/hide line numbers
rsa.c
00001 /* 00002 * The RSA public-key cryptosystem 00003 * 00004 * Copyright (C) 2006-2015, ARM Limited, All Rights Reserved 00005 * SPDX-License-Identifier: Apache-2.0 00006 * 00007 * Licensed under the Apache License, Version 2.0 (the "License"); you may 00008 * not use this file except in compliance with the License. 00009 * You may obtain a copy of the License at 00010 * 00011 * http://www.apache.org/licenses/LICENSE-2.0 00012 * 00013 * Unless required by applicable law or agreed to in writing, software 00014 * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT 00015 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00016 * See the License for the specific language governing permissions and 00017 * limitations under the License. 00018 * 00019 * This file is part of mbed TLS (https://tls.mbed.org) 00020 */ 00021 /* 00022 * RSA was designed by Ron Rivest, Adi Shamir and Len Adleman. 00023 * 00024 * http://theory.lcs.mit.edu/~rivest/rsapaper.pdf 00025 * http://www.cacr.math.uwaterloo.ca/hac/about/chap8.pdf 00026 */ 00027 00028 #if !defined(MBEDTLS_CONFIG_FILE) 00029 #include "mbedtls/config.h" 00030 #else 00031 #include MBEDTLS_CONFIG_FILE 00032 #endif 00033 00034 #if defined(MBEDTLS_RSA_C) 00035 00036 #include "mbedtls/rsa.h" 00037 #include "mbedtls/oid.h" 00038 00039 #include <string.h> 00040 00041 #if defined(MBEDTLS_PKCS1_V21) 00042 #include "mbedtls/md.h" 00043 #endif 00044 00045 #if defined(MBEDTLS_PKCS1_V15) && !defined(__OpenBSD__) 00046 #include <stdlib.h> 00047 #endif 00048 00049 #if defined(MBEDTLS_PLATFORM_C) 00050 #include "mbedtls/platform.h" 00051 #else 00052 #include <stdio.h> 00053 #define mbedtls_printf printf 00054 #define mbedtls_calloc calloc 00055 #define mbedtls_free free 00056 #endif 00057 00058 /* 00059 * Initialize an RSA context 00060 */ 00061 void mbedtls_rsa_init( mbedtls_rsa_context *ctx, 00062 int padding, 00063 int hash_id ) 00064 { 00065 memset( ctx, 0, sizeof( mbedtls_rsa_context ) ); 00066 00067 mbedtls_rsa_set_padding( ctx, padding, hash_id ); 00068 00069 #if defined(MBEDTLS_THREADING_C) 00070 mbedtls_mutex_init( &ctx->mutex ); 00071 #endif 00072 } 00073 00074 /* 00075 * Set padding for an existing RSA context 00076 */ 00077 void mbedtls_rsa_set_padding( mbedtls_rsa_context *ctx, int padding, int hash_id ) 00078 { 00079 ctx->padding = padding; 00080 ctx->hash_id = hash_id; 00081 } 00082 00083 #if defined(MBEDTLS_GENPRIME) 00084 00085 /* 00086 * Generate an RSA keypair 00087 */ 00088 int mbedtls_rsa_gen_key( mbedtls_rsa_context *ctx, 00089 int (*f_rng)(void *, unsigned char *, size_t), 00090 void *p_rng, 00091 unsigned int nbits, int exponent ) 00092 { 00093 int ret; 00094 mbedtls_mpi P1, Q1, H, G; 00095 00096 if( f_rng == NULL || nbits < 128 || exponent < 3 ) 00097 return( MBEDTLS_ERR_RSA_BAD_INPUT_DATA ); 00098 00099 mbedtls_mpi_init( &P1 ); mbedtls_mpi_init( &Q1 ); mbedtls_mpi_init( &H ); mbedtls_mpi_init( &G ); 00100 00101 /* 00102 * find primes P and Q with Q < P so that: 00103 * GCD( E, (P-1)*(Q-1) ) == 1 00104 */ 00105 MBEDTLS_MPI_CHK( mbedtls_mpi_lset( &ctx->E , exponent ) ); 00106 00107 do 00108 { 00109 MBEDTLS_MPI_CHK( mbedtls_mpi_gen_prime( &ctx->P , ( nbits + 1 ) >> 1, 0, 00110 f_rng, p_rng ) ); 00111 00112 MBEDTLS_MPI_CHK( mbedtls_mpi_gen_prime( &ctx->Q , ( nbits + 1 ) >> 1, 0, 00113 f_rng, p_rng ) ); 00114 00115 if( mbedtls_mpi_cmp_mpi( &ctx->P , &ctx->Q ) < 0 ) 00116 mbedtls_mpi_swap( &ctx->P , &ctx->Q ); 00117 00118 if( mbedtls_mpi_cmp_mpi( &ctx->P , &ctx->Q ) == 0 ) 00119 continue; 00120 00121 MBEDTLS_MPI_CHK( mbedtls_mpi_mul_mpi( &ctx->N , &ctx->P , &ctx->Q ) ); 00122 if( mbedtls_mpi_bitlen( &ctx->N ) != nbits ) 00123 continue; 00124 00125 MBEDTLS_MPI_CHK( mbedtls_mpi_sub_int( &P1, &ctx->P , 1 ) ); 00126 MBEDTLS_MPI_CHK( mbedtls_mpi_sub_int( &Q1, &ctx->Q , 1 ) ); 00127 MBEDTLS_MPI_CHK( mbedtls_mpi_mul_mpi( &H, &P1, &Q1 ) ); 00128 MBEDTLS_MPI_CHK( mbedtls_mpi_gcd( &G, &ctx->E , &H ) ); 00129 } 00130 while( mbedtls_mpi_cmp_int( &G, 1 ) != 0 ); 00131 00132 /* 00133 * D = E^-1 mod ((P-1)*(Q-1)) 00134 * DP = D mod (P - 1) 00135 * DQ = D mod (Q - 1) 00136 * QP = Q^-1 mod P 00137 */ 00138 MBEDTLS_MPI_CHK( mbedtls_mpi_inv_mod( &ctx->D , &ctx->E , &H ) ); 00139 MBEDTLS_MPI_CHK( mbedtls_mpi_mod_mpi( &ctx->DP , &ctx->D , &P1 ) ); 00140 MBEDTLS_MPI_CHK( mbedtls_mpi_mod_mpi( &ctx->DQ , &ctx->D , &Q1 ) ); 00141 MBEDTLS_MPI_CHK( mbedtls_mpi_inv_mod( &ctx->QP , &ctx->Q , &ctx->P ) ); 00142 00143 ctx->len = ( mbedtls_mpi_bitlen( &ctx->N ) + 7 ) >> 3; 00144 00145 cleanup: 00146 00147 mbedtls_mpi_free( &P1 ); mbedtls_mpi_free( &Q1 ); mbedtls_mpi_free( &H ); mbedtls_mpi_free( &G ); 00148 00149 if( ret != 0 ) 00150 { 00151 mbedtls_rsa_free( ctx ); 00152 return( MBEDTLS_ERR_RSA_KEY_GEN_FAILED + ret ); 00153 } 00154 00155 return( 0 ); 00156 } 00157 00158 #endif /* MBEDTLS_GENPRIME */ 00159 00160 /* 00161 * Check a public RSA key 00162 */ 00163 int mbedtls_rsa_check_pubkey( const mbedtls_rsa_context *ctx ) 00164 { 00165 if( !ctx->N .p || !ctx->E .p ) 00166 return( MBEDTLS_ERR_RSA_KEY_CHECK_FAILED ); 00167 00168 if( ( ctx->N .p [0] & 1 ) == 0 || 00169 ( ctx->E .p [0] & 1 ) == 0 ) 00170 return( MBEDTLS_ERR_RSA_KEY_CHECK_FAILED ); 00171 00172 if( mbedtls_mpi_bitlen( &ctx->N ) < 128 || 00173 mbedtls_mpi_bitlen( &ctx->N ) > MBEDTLS_MPI_MAX_BITS ) 00174 return( MBEDTLS_ERR_RSA_KEY_CHECK_FAILED ); 00175 00176 if( mbedtls_mpi_bitlen( &ctx->E ) < 2 || 00177 mbedtls_mpi_cmp_mpi( &ctx->E , &ctx->N ) >= 0 ) 00178 return( MBEDTLS_ERR_RSA_KEY_CHECK_FAILED ); 00179 00180 return( 0 ); 00181 } 00182 00183 /* 00184 * Check a private RSA key 00185 */ 00186 int mbedtls_rsa_check_privkey( const mbedtls_rsa_context *ctx ) 00187 { 00188 int ret; 00189 mbedtls_mpi PQ, DE, P1, Q1, H, I, G, G2, L1, L2, DP, DQ, QP; 00190 00191 if( ( ret = mbedtls_rsa_check_pubkey( ctx ) ) != 0 ) 00192 return( ret ); 00193 00194 if( !ctx->P .p || !ctx->Q .p || !ctx->D .p ) 00195 return( MBEDTLS_ERR_RSA_KEY_CHECK_FAILED ); 00196 00197 mbedtls_mpi_init( &PQ ); mbedtls_mpi_init( &DE ); mbedtls_mpi_init( &P1 ); mbedtls_mpi_init( &Q1 ); 00198 mbedtls_mpi_init( &H ); mbedtls_mpi_init( &I ); mbedtls_mpi_init( &G ); mbedtls_mpi_init( &G2 ); 00199 mbedtls_mpi_init( &L1 ); mbedtls_mpi_init( &L2 ); mbedtls_mpi_init( &DP ); mbedtls_mpi_init( &DQ ); 00200 mbedtls_mpi_init( &QP ); 00201 00202 MBEDTLS_MPI_CHK( mbedtls_mpi_mul_mpi( &PQ, &ctx->P , &ctx->Q ) ); 00203 MBEDTLS_MPI_CHK( mbedtls_mpi_mul_mpi( &DE, &ctx->D , &ctx->E ) ); 00204 MBEDTLS_MPI_CHK( mbedtls_mpi_sub_int( &P1, &ctx->P , 1 ) ); 00205 MBEDTLS_MPI_CHK( mbedtls_mpi_sub_int( &Q1, &ctx->Q , 1 ) ); 00206 MBEDTLS_MPI_CHK( mbedtls_mpi_mul_mpi( &H, &P1, &Q1 ) ); 00207 MBEDTLS_MPI_CHK( mbedtls_mpi_gcd( &G, &ctx->E , &H ) ); 00208 00209 MBEDTLS_MPI_CHK( mbedtls_mpi_gcd( &G2, &P1, &Q1 ) ); 00210 MBEDTLS_MPI_CHK( mbedtls_mpi_div_mpi( &L1, &L2, &H, &G2 ) ); 00211 MBEDTLS_MPI_CHK( mbedtls_mpi_mod_mpi( &I, &DE, &L1 ) ); 00212 00213 MBEDTLS_MPI_CHK( mbedtls_mpi_mod_mpi( &DP, &ctx->D , &P1 ) ); 00214 MBEDTLS_MPI_CHK( mbedtls_mpi_mod_mpi( &DQ, &ctx->D , &Q1 ) ); 00215 MBEDTLS_MPI_CHK( mbedtls_mpi_inv_mod( &QP, &ctx->Q , &ctx->P ) ); 00216 /* 00217 * Check for a valid PKCS1v2 private key 00218 */ 00219 if( mbedtls_mpi_cmp_mpi( &PQ, &ctx->N ) != 0 || 00220 mbedtls_mpi_cmp_mpi( &DP, &ctx->DP ) != 0 || 00221 mbedtls_mpi_cmp_mpi( &DQ, &ctx->DQ ) != 0 || 00222 mbedtls_mpi_cmp_mpi( &QP, &ctx->QP ) != 0 || 00223 mbedtls_mpi_cmp_int( &L2, 0 ) != 0 || 00224 mbedtls_mpi_cmp_int( &I, 1 ) != 0 || 00225 mbedtls_mpi_cmp_int( &G, 1 ) != 0 ) 00226 { 00227 ret = MBEDTLS_ERR_RSA_KEY_CHECK_FAILED; 00228 } 00229 00230 cleanup: 00231 mbedtls_mpi_free( &PQ ); mbedtls_mpi_free( &DE ); mbedtls_mpi_free( &P1 ); mbedtls_mpi_free( &Q1 ); 00232 mbedtls_mpi_free( &H ); mbedtls_mpi_free( &I ); mbedtls_mpi_free( &G ); mbedtls_mpi_free( &G2 ); 00233 mbedtls_mpi_free( &L1 ); mbedtls_mpi_free( &L2 ); mbedtls_mpi_free( &DP ); mbedtls_mpi_free( &DQ ); 00234 mbedtls_mpi_free( &QP ); 00235 00236 if( ret == MBEDTLS_ERR_RSA_KEY_CHECK_FAILED ) 00237 return( ret ); 00238 00239 if( ret != 0 ) 00240 return( MBEDTLS_ERR_RSA_KEY_CHECK_FAILED + ret ); 00241 00242 return( 0 ); 00243 } 00244 00245 /* 00246 * Check if contexts holding a public and private key match 00247 */ 00248 int mbedtls_rsa_check_pub_priv( const mbedtls_rsa_context *pub, const mbedtls_rsa_context *prv ) 00249 { 00250 if( mbedtls_rsa_check_pubkey( pub ) != 0 || 00251 mbedtls_rsa_check_privkey( prv ) != 0 ) 00252 { 00253 return( MBEDTLS_ERR_RSA_KEY_CHECK_FAILED ); 00254 } 00255 00256 if( mbedtls_mpi_cmp_mpi( &pub->N , &prv->N ) != 0 || 00257 mbedtls_mpi_cmp_mpi( &pub->E , &prv->E ) != 0 ) 00258 { 00259 return( MBEDTLS_ERR_RSA_KEY_CHECK_FAILED ); 00260 } 00261 00262 return( 0 ); 00263 } 00264 00265 /* 00266 * Do an RSA public key operation 00267 */ 00268 int mbedtls_rsa_public( mbedtls_rsa_context *ctx, 00269 const unsigned char *input, 00270 unsigned char *output ) 00271 { 00272 int ret; 00273 size_t olen; 00274 mbedtls_mpi T; 00275 00276 mbedtls_mpi_init( &T ); 00277 00278 #if defined(MBEDTLS_THREADING_C) 00279 if( ( ret = mbedtls_mutex_lock( &ctx->mutex ) ) != 0 ) 00280 return( ret ); 00281 #endif 00282 00283 MBEDTLS_MPI_CHK( mbedtls_mpi_read_binary( &T, input, ctx->len ) ); 00284 00285 if( mbedtls_mpi_cmp_mpi( &T, &ctx->N ) >= 0 ) 00286 { 00287 ret = MBEDTLS_ERR_MPI_BAD_INPUT_DATA; 00288 goto cleanup; 00289 } 00290 00291 olen = ctx->len ; 00292 MBEDTLS_MPI_CHK( mbedtls_mpi_exp_mod( &T, &T, &ctx->E , &ctx->N , &ctx->RN ) ); 00293 MBEDTLS_MPI_CHK( mbedtls_mpi_write_binary( &T, output, olen ) ); 00294 00295 cleanup: 00296 #if defined(MBEDTLS_THREADING_C) 00297 if( mbedtls_mutex_unlock( &ctx->mutex ) != 0 ) 00298 return( MBEDTLS_ERR_THREADING_MUTEX_ERROR ); 00299 #endif 00300 00301 mbedtls_mpi_free( &T ); 00302 00303 if( ret != 0 ) 00304 return( MBEDTLS_ERR_RSA_PUBLIC_FAILED + ret ); 00305 00306 return( 0 ); 00307 } 00308 00309 /* 00310 * Generate or update blinding values, see section 10 of: 00311 * KOCHER, Paul C. Timing attacks on implementations of Diffie-Hellman, RSA, 00312 * DSS, and other systems. In : Advances in Cryptology-CRYPTO'96. Springer 00313 * Berlin Heidelberg, 1996. p. 104-113. 00314 */ 00315 static int rsa_prepare_blinding( mbedtls_rsa_context *ctx, 00316 int (*f_rng)(void *, unsigned char *, size_t), void *p_rng ) 00317 { 00318 int ret, count = 0; 00319 00320 if( ctx->Vf .p != NULL ) 00321 { 00322 /* We already have blinding values, just update them by squaring */ 00323 MBEDTLS_MPI_CHK( mbedtls_mpi_mul_mpi( &ctx->Vi , &ctx->Vi , &ctx->Vi ) ); 00324 MBEDTLS_MPI_CHK( mbedtls_mpi_mod_mpi( &ctx->Vi , &ctx->Vi , &ctx->N ) ); 00325 MBEDTLS_MPI_CHK( mbedtls_mpi_mul_mpi( &ctx->Vf , &ctx->Vf , &ctx->Vf ) ); 00326 MBEDTLS_MPI_CHK( mbedtls_mpi_mod_mpi( &ctx->Vf , &ctx->Vf , &ctx->N ) ); 00327 00328 goto cleanup; 00329 } 00330 00331 /* Unblinding value: Vf = random number, invertible mod N */ 00332 do { 00333 if( count++ > 10 ) 00334 return( MBEDTLS_ERR_RSA_RNG_FAILED ); 00335 00336 MBEDTLS_MPI_CHK( mbedtls_mpi_fill_random( &ctx->Vf , ctx->len - 1, f_rng, p_rng ) ); 00337 MBEDTLS_MPI_CHK( mbedtls_mpi_gcd( &ctx->Vi , &ctx->Vf , &ctx->N ) ); 00338 } while( mbedtls_mpi_cmp_int( &ctx->Vi , 1 ) != 0 ); 00339 00340 /* Blinding value: Vi = Vf^(-e) mod N */ 00341 MBEDTLS_MPI_CHK( mbedtls_mpi_inv_mod( &ctx->Vi , &ctx->Vf , &ctx->N ) ); 00342 MBEDTLS_MPI_CHK( mbedtls_mpi_exp_mod( &ctx->Vi , &ctx->Vi , &ctx->E , &ctx->N , &ctx->RN ) ); 00343 00344 00345 cleanup: 00346 return( ret ); 00347 } 00348 00349 /* 00350 * Do an RSA private key operation 00351 */ 00352 int mbedtls_rsa_private( mbedtls_rsa_context *ctx, 00353 int (*f_rng)(void *, unsigned char *, size_t), 00354 void *p_rng, 00355 const unsigned char *input, 00356 unsigned char *output ) 00357 { 00358 int ret; 00359 size_t olen; 00360 mbedtls_mpi T, T1, T2; 00361 00362 /* Make sure we have private key info, prevent possible misuse */ 00363 if( ctx->P .p == NULL || ctx->Q .p == NULL || ctx->D .p == NULL ) 00364 return( MBEDTLS_ERR_RSA_BAD_INPUT_DATA ); 00365 00366 mbedtls_mpi_init( &T ); mbedtls_mpi_init( &T1 ); mbedtls_mpi_init( &T2 ); 00367 00368 #if defined(MBEDTLS_THREADING_C) 00369 if( ( ret = mbedtls_mutex_lock( &ctx->mutex ) ) != 0 ) 00370 return( ret ); 00371 #endif 00372 00373 MBEDTLS_MPI_CHK( mbedtls_mpi_read_binary( &T, input, ctx->len ) ); 00374 if( mbedtls_mpi_cmp_mpi( &T, &ctx->N ) >= 0 ) 00375 { 00376 ret = MBEDTLS_ERR_MPI_BAD_INPUT_DATA; 00377 goto cleanup; 00378 } 00379 00380 if( f_rng != NULL ) 00381 { 00382 /* 00383 * Blinding 00384 * T = T * Vi mod N 00385 */ 00386 MBEDTLS_MPI_CHK( rsa_prepare_blinding( ctx, f_rng, p_rng ) ); 00387 MBEDTLS_MPI_CHK( mbedtls_mpi_mul_mpi( &T, &T, &ctx->Vi ) ); 00388 MBEDTLS_MPI_CHK( mbedtls_mpi_mod_mpi( &T, &T, &ctx->N ) ); 00389 } 00390 00391 #if defined(MBEDTLS_RSA_NO_CRT) 00392 MBEDTLS_MPI_CHK( mbedtls_mpi_exp_mod( &T, &T, &ctx->D , &ctx->N , &ctx->RN ) ); 00393 #else 00394 /* 00395 * faster decryption using the CRT 00396 * 00397 * T1 = input ^ dP mod P 00398 * T2 = input ^ dQ mod Q 00399 */ 00400 MBEDTLS_MPI_CHK( mbedtls_mpi_exp_mod( &T1, &T, &ctx->DP , &ctx->P , &ctx->RP ) ); 00401 MBEDTLS_MPI_CHK( mbedtls_mpi_exp_mod( &T2, &T, &ctx->DQ , &ctx->Q , &ctx->RQ ) ); 00402 00403 /* 00404 * T = (T1 - T2) * (Q^-1 mod P) mod P 00405 */ 00406 MBEDTLS_MPI_CHK( mbedtls_mpi_sub_mpi( &T, &T1, &T2 ) ); 00407 MBEDTLS_MPI_CHK( mbedtls_mpi_mul_mpi( &T1, &T, &ctx->QP ) ); 00408 MBEDTLS_MPI_CHK( mbedtls_mpi_mod_mpi( &T, &T1, &ctx->P ) ); 00409 00410 /* 00411 * T = T2 + T * Q 00412 */ 00413 MBEDTLS_MPI_CHK( mbedtls_mpi_mul_mpi( &T1, &T, &ctx->Q ) ); 00414 MBEDTLS_MPI_CHK( mbedtls_mpi_add_mpi( &T, &T2, &T1 ) ); 00415 #endif /* MBEDTLS_RSA_NO_CRT */ 00416 00417 if( f_rng != NULL ) 00418 { 00419 /* 00420 * Unblind 00421 * T = T * Vf mod N 00422 */ 00423 MBEDTLS_MPI_CHK( mbedtls_mpi_mul_mpi( &T, &T, &ctx->Vf ) ); 00424 MBEDTLS_MPI_CHK( mbedtls_mpi_mod_mpi( &T, &T, &ctx->N ) ); 00425 } 00426 00427 olen = ctx->len ; 00428 MBEDTLS_MPI_CHK( mbedtls_mpi_write_binary( &T, output, olen ) ); 00429 00430 cleanup: 00431 #if defined(MBEDTLS_THREADING_C) 00432 if( mbedtls_mutex_unlock( &ctx->mutex ) != 0 ) 00433 return( MBEDTLS_ERR_THREADING_MUTEX_ERROR ); 00434 #endif 00435 00436 mbedtls_mpi_free( &T ); mbedtls_mpi_free( &T1 ); mbedtls_mpi_free( &T2 ); 00437 00438 if( ret != 0 ) 00439 return( MBEDTLS_ERR_RSA_PRIVATE_FAILED + ret ); 00440 00441 return( 0 ); 00442 } 00443 00444 #if defined(MBEDTLS_PKCS1_V21) 00445 /** 00446 * Generate and apply the MGF1 operation (from PKCS#1 v2.1) to a buffer. 00447 * 00448 * \param dst buffer to mask 00449 * \param dlen length of destination buffer 00450 * \param src source of the mask generation 00451 * \param slen length of the source buffer 00452 * \param md_ctx message digest context to use 00453 */ 00454 static void mgf_mask( unsigned char *dst, size_t dlen, unsigned char *src, 00455 size_t slen, mbedtls_md_context_t *md_ctx ) 00456 { 00457 unsigned char mask[MBEDTLS_MD_MAX_SIZE]; 00458 unsigned char counter[4]; 00459 unsigned char *p; 00460 unsigned int hlen; 00461 size_t i, use_len; 00462 00463 memset( mask, 0, MBEDTLS_MD_MAX_SIZE ); 00464 memset( counter, 0, 4 ); 00465 00466 hlen = mbedtls_md_get_size( md_ctx->md_info ); 00467 00468 // Generate and apply dbMask 00469 // 00470 p = dst; 00471 00472 while( dlen > 0 ) 00473 { 00474 use_len = hlen; 00475 if( dlen < hlen ) 00476 use_len = dlen; 00477 00478 mbedtls_md_starts( md_ctx ); 00479 mbedtls_md_update( md_ctx, src, slen ); 00480 mbedtls_md_update( md_ctx, counter, 4 ); 00481 mbedtls_md_finish( md_ctx, mask ); 00482 00483 for( i = 0; i < use_len; ++i ) 00484 *p++ ^= mask[i]; 00485 00486 counter[3]++; 00487 00488 dlen -= use_len; 00489 } 00490 } 00491 #endif /* MBEDTLS_PKCS1_V21 */ 00492 00493 #if defined(MBEDTLS_PKCS1_V21) 00494 /* 00495 * Implementation of the PKCS#1 v2.1 RSAES-OAEP-ENCRYPT function 00496 */ 00497 int mbedtls_rsa_rsaes_oaep_encrypt( mbedtls_rsa_context *ctx, 00498 int (*f_rng)(void *, unsigned char *, size_t), 00499 void *p_rng, 00500 int mode, 00501 const unsigned char *label, size_t label_len, 00502 size_t ilen, 00503 const unsigned char *input, 00504 unsigned char *output ) 00505 { 00506 size_t olen; 00507 int ret; 00508 unsigned char *p = output; 00509 unsigned int hlen; 00510 const mbedtls_md_info_t *md_info; 00511 mbedtls_md_context_t md_ctx; 00512 00513 if( mode == MBEDTLS_RSA_PRIVATE && ctx->padding != MBEDTLS_RSA_PKCS_V21 ) 00514 return( MBEDTLS_ERR_RSA_BAD_INPUT_DATA ); 00515 00516 if( f_rng == NULL ) 00517 return( MBEDTLS_ERR_RSA_BAD_INPUT_DATA ); 00518 00519 md_info = mbedtls_md_info_from_type( (mbedtls_md_type_t) ctx->hash_id ); 00520 if( md_info == NULL ) 00521 return( MBEDTLS_ERR_RSA_BAD_INPUT_DATA ); 00522 00523 olen = ctx->len ; 00524 hlen = mbedtls_md_get_size( md_info ); 00525 00526 if( olen < ilen + 2 * hlen + 2 ) 00527 return( MBEDTLS_ERR_RSA_BAD_INPUT_DATA ); 00528 00529 memset( output, 0, olen ); 00530 00531 *p++ = 0; 00532 00533 // Generate a random octet string seed 00534 // 00535 if( ( ret = f_rng( p_rng, p, hlen ) ) != 0 ) 00536 return( MBEDTLS_ERR_RSA_RNG_FAILED + ret ); 00537 00538 p += hlen; 00539 00540 // Construct DB 00541 // 00542 mbedtls_md( md_info, label, label_len, p ); 00543 p += hlen; 00544 p += olen - 2 * hlen - 2 - ilen; 00545 *p++ = 1; 00546 memcpy( p, input, ilen ); 00547 00548 mbedtls_md_init( &md_ctx ); 00549 mbedtls_md_setup( &md_ctx, md_info, 0 ); 00550 00551 // maskedDB: Apply dbMask to DB 00552 // 00553 mgf_mask( output + hlen + 1, olen - hlen - 1, output + 1, hlen, 00554 &md_ctx ); 00555 00556 // maskedSeed: Apply seedMask to seed 00557 // 00558 mgf_mask( output + 1, hlen, output + hlen + 1, olen - hlen - 1, 00559 &md_ctx ); 00560 00561 mbedtls_md_free( &md_ctx ); 00562 00563 return( ( mode == MBEDTLS_RSA_PUBLIC ) 00564 ? mbedtls_rsa_public( ctx, output, output ) 00565 : mbedtls_rsa_private( ctx, f_rng, p_rng, output, output ) ); 00566 } 00567 #endif /* MBEDTLS_PKCS1_V21 */ 00568 00569 #if defined(MBEDTLS_PKCS1_V15) 00570 /* 00571 * Implementation of the PKCS#1 v2.1 RSAES-PKCS1-V1_5-ENCRYPT function 00572 */ 00573 int mbedtls_rsa_rsaes_pkcs1_v15_encrypt( mbedtls_rsa_context *ctx, 00574 int (*f_rng)(void *, unsigned char *, size_t), 00575 void *p_rng, 00576 int mode, size_t ilen, 00577 const unsigned char *input, 00578 unsigned char *output ) 00579 { 00580 size_t nb_pad, olen; 00581 int ret; 00582 unsigned char *p = output; 00583 00584 if( mode == MBEDTLS_RSA_PRIVATE && ctx->padding != MBEDTLS_RSA_PKCS_V15 ) 00585 return( MBEDTLS_ERR_RSA_BAD_INPUT_DATA ); 00586 00587 if( f_rng == NULL ) 00588 return( MBEDTLS_ERR_RSA_BAD_INPUT_DATA ); 00589 00590 olen = ctx->len ; 00591 00592 if( olen < ilen + 11 ) 00593 return( MBEDTLS_ERR_RSA_BAD_INPUT_DATA ); 00594 00595 nb_pad = olen - 3 - ilen; 00596 00597 *p++ = 0; 00598 if( mode == MBEDTLS_RSA_PUBLIC ) 00599 { 00600 *p++ = MBEDTLS_RSA_CRYPT; 00601 00602 while( nb_pad-- > 0 ) 00603 { 00604 int rng_dl = 100; 00605 00606 do { 00607 ret = f_rng( p_rng, p, 1 ); 00608 } while( *p == 0 && --rng_dl && ret == 0 ); 00609 00610 // Check if RNG failed to generate data 00611 // 00612 if( rng_dl == 0 || ret != 0 ) 00613 return( MBEDTLS_ERR_RSA_RNG_FAILED + ret ); 00614 00615 p++; 00616 } 00617 } 00618 else 00619 { 00620 *p++ = MBEDTLS_RSA_SIGN; 00621 00622 while( nb_pad-- > 0 ) 00623 *p++ = 0xFF; 00624 } 00625 00626 *p++ = 0; 00627 memcpy( p, input, ilen ); 00628 00629 return( ( mode == MBEDTLS_RSA_PUBLIC ) 00630 ? mbedtls_rsa_public( ctx, output, output ) 00631 : mbedtls_rsa_private( ctx, f_rng, p_rng, output, output ) ); 00632 } 00633 #endif /* MBEDTLS_PKCS1_V15 */ 00634 00635 /* 00636 * Add the message padding, then do an RSA operation 00637 */ 00638 int mbedtls_rsa_pkcs1_encrypt( mbedtls_rsa_context *ctx, 00639 int (*f_rng)(void *, unsigned char *, size_t), 00640 void *p_rng, 00641 int mode, size_t ilen, 00642 const unsigned char *input, 00643 unsigned char *output ) 00644 { 00645 switch( ctx->padding ) 00646 { 00647 #if defined(MBEDTLS_PKCS1_V15) 00648 case MBEDTLS_RSA_PKCS_V15: 00649 return mbedtls_rsa_rsaes_pkcs1_v15_encrypt( ctx, f_rng, p_rng, mode, ilen, 00650 input, output ); 00651 #endif 00652 00653 #if defined(MBEDTLS_PKCS1_V21) 00654 case MBEDTLS_RSA_PKCS_V21: 00655 return mbedtls_rsa_rsaes_oaep_encrypt( ctx, f_rng, p_rng, mode, NULL, 0, 00656 ilen, input, output ); 00657 #endif 00658 00659 default: 00660 return( MBEDTLS_ERR_RSA_INVALID_PADDING ); 00661 } 00662 } 00663 00664 #if defined(MBEDTLS_PKCS1_V21) 00665 /* 00666 * Implementation of the PKCS#1 v2.1 RSAES-OAEP-DECRYPT function 00667 */ 00668 int mbedtls_rsa_rsaes_oaep_decrypt( mbedtls_rsa_context *ctx, 00669 int (*f_rng)(void *, unsigned char *, size_t), 00670 void *p_rng, 00671 int mode, 00672 const unsigned char *label, size_t label_len, 00673 size_t *olen, 00674 const unsigned char *input, 00675 unsigned char *output, 00676 size_t output_max_len ) 00677 { 00678 int ret; 00679 size_t ilen, i, pad_len; 00680 unsigned char *p, bad, pad_done; 00681 unsigned char buf[MBEDTLS_MPI_MAX_SIZE]; 00682 unsigned char lhash[MBEDTLS_MD_MAX_SIZE]; 00683 unsigned int hlen; 00684 const mbedtls_md_info_t *md_info; 00685 mbedtls_md_context_t md_ctx; 00686 00687 /* 00688 * Parameters sanity checks 00689 */ 00690 if( mode == MBEDTLS_RSA_PRIVATE && ctx->padding != MBEDTLS_RSA_PKCS_V21 ) 00691 return( MBEDTLS_ERR_RSA_BAD_INPUT_DATA ); 00692 00693 ilen = ctx->len ; 00694 00695 if( ilen < 16 || ilen > sizeof( buf ) ) 00696 return( MBEDTLS_ERR_RSA_BAD_INPUT_DATA ); 00697 00698 md_info = mbedtls_md_info_from_type( (mbedtls_md_type_t) ctx->hash_id ); 00699 if( md_info == NULL ) 00700 return( MBEDTLS_ERR_RSA_BAD_INPUT_DATA ); 00701 00702 /* 00703 * RSA operation 00704 */ 00705 ret = ( mode == MBEDTLS_RSA_PUBLIC ) 00706 ? mbedtls_rsa_public( ctx, input, buf ) 00707 : mbedtls_rsa_private( ctx, f_rng, p_rng, input, buf ); 00708 00709 if( ret != 0 ) 00710 return( ret ); 00711 00712 /* 00713 * Unmask data and generate lHash 00714 */ 00715 hlen = mbedtls_md_get_size( md_info ); 00716 00717 mbedtls_md_init( &md_ctx ); 00718 mbedtls_md_setup( &md_ctx, md_info, 0 ); 00719 00720 /* Generate lHash */ 00721 mbedtls_md( md_info, label, label_len, lhash ); 00722 00723 /* seed: Apply seedMask to maskedSeed */ 00724 mgf_mask( buf + 1, hlen, buf + hlen + 1, ilen - hlen - 1, 00725 &md_ctx ); 00726 00727 /* DB: Apply dbMask to maskedDB */ 00728 mgf_mask( buf + hlen + 1, ilen - hlen - 1, buf + 1, hlen, 00729 &md_ctx ); 00730 00731 mbedtls_md_free( &md_ctx ); 00732 00733 /* 00734 * Check contents, in "constant-time" 00735 */ 00736 p = buf; 00737 bad = 0; 00738 00739 bad |= *p++; /* First byte must be 0 */ 00740 00741 p += hlen; /* Skip seed */ 00742 00743 /* Check lHash */ 00744 for( i = 0; i < hlen; i++ ) 00745 bad |= lhash[i] ^ *p++; 00746 00747 /* Get zero-padding len, but always read till end of buffer 00748 * (minus one, for the 01 byte) */ 00749 pad_len = 0; 00750 pad_done = 0; 00751 for( i = 0; i < ilen - 2 * hlen - 2; i++ ) 00752 { 00753 pad_done |= p[i]; 00754 pad_len += ((pad_done | (unsigned char)-pad_done) >> 7) ^ 1; 00755 } 00756 00757 p += pad_len; 00758 bad |= *p++ ^ 0x01; 00759 00760 /* 00761 * The only information "leaked" is whether the padding was correct or not 00762 * (eg, no data is copied if it was not correct). This meets the 00763 * recommendations in PKCS#1 v2.2: an opponent cannot distinguish between 00764 * the different error conditions. 00765 */ 00766 if( bad != 0 ) 00767 return( MBEDTLS_ERR_RSA_INVALID_PADDING ); 00768 00769 if( ilen - ( p - buf ) > output_max_len ) 00770 return( MBEDTLS_ERR_RSA_OUTPUT_TOO_LARGE ); 00771 00772 *olen = ilen - (p - buf); 00773 memcpy( output, p, *olen ); 00774 00775 return( 0 ); 00776 } 00777 #endif /* MBEDTLS_PKCS1_V21 */ 00778 00779 #if defined(MBEDTLS_PKCS1_V15) 00780 /* 00781 * Implementation of the PKCS#1 v2.1 RSAES-PKCS1-V1_5-DECRYPT function 00782 */ 00783 int mbedtls_rsa_rsaes_pkcs1_v15_decrypt( mbedtls_rsa_context *ctx, 00784 int (*f_rng)(void *, unsigned char *, size_t), 00785 void *p_rng, 00786 int mode, size_t *olen, 00787 const unsigned char *input, 00788 unsigned char *output, 00789 size_t output_max_len) 00790 { 00791 int ret; 00792 size_t ilen, pad_count = 0, i; 00793 unsigned char *p, bad, pad_done = 0; 00794 unsigned char buf[MBEDTLS_MPI_MAX_SIZE]; 00795 00796 if( mode == MBEDTLS_RSA_PRIVATE && ctx->padding != MBEDTLS_RSA_PKCS_V15 ) 00797 return( MBEDTLS_ERR_RSA_BAD_INPUT_DATA ); 00798 00799 ilen = ctx->len ; 00800 00801 if( ilen < 16 || ilen > sizeof( buf ) ) 00802 return( MBEDTLS_ERR_RSA_BAD_INPUT_DATA ); 00803 00804 ret = ( mode == MBEDTLS_RSA_PUBLIC ) 00805 ? mbedtls_rsa_public( ctx, input, buf ) 00806 : mbedtls_rsa_private( ctx, f_rng, p_rng, input, buf ); 00807 00808 if( ret != 0 ) 00809 return( ret ); 00810 00811 p = buf; 00812 bad = 0; 00813 00814 /* 00815 * Check and get padding len in "constant-time" 00816 */ 00817 bad |= *p++; /* First byte must be 0 */ 00818 00819 /* This test does not depend on secret data */ 00820 if( mode == MBEDTLS_RSA_PRIVATE ) 00821 { 00822 bad |= *p++ ^ MBEDTLS_RSA_CRYPT; 00823 00824 /* Get padding len, but always read till end of buffer 00825 * (minus one, for the 00 byte) */ 00826 for( i = 0; i < ilen - 3; i++ ) 00827 { 00828 pad_done |= ((p[i] | (unsigned char)-p[i]) >> 7) ^ 1; 00829 pad_count += ((pad_done | (unsigned char)-pad_done) >> 7) ^ 1; 00830 } 00831 00832 p += pad_count; 00833 bad |= *p++; /* Must be zero */ 00834 } 00835 else 00836 { 00837 bad |= *p++ ^ MBEDTLS_RSA_SIGN; 00838 00839 /* Get padding len, but always read till end of buffer 00840 * (minus one, for the 00 byte) */ 00841 for( i = 0; i < ilen - 3; i++ ) 00842 { 00843 pad_done |= ( p[i] != 0xFF ); 00844 pad_count += ( pad_done == 0 ); 00845 } 00846 00847 p += pad_count; 00848 bad |= *p++; /* Must be zero */ 00849 } 00850 00851 if( bad ) 00852 return( MBEDTLS_ERR_RSA_INVALID_PADDING ); 00853 00854 if( ilen - ( p - buf ) > output_max_len ) 00855 return( MBEDTLS_ERR_RSA_OUTPUT_TOO_LARGE ); 00856 00857 *olen = ilen - (p - buf); 00858 memcpy( output, p, *olen ); 00859 00860 return( 0 ); 00861 } 00862 #endif /* MBEDTLS_PKCS1_V15 */ 00863 00864 /* 00865 * Do an RSA operation, then remove the message padding 00866 */ 00867 int mbedtls_rsa_pkcs1_decrypt( mbedtls_rsa_context *ctx, 00868 int (*f_rng)(void *, unsigned char *, size_t), 00869 void *p_rng, 00870 int mode, size_t *olen, 00871 const unsigned char *input, 00872 unsigned char *output, 00873 size_t output_max_len) 00874 { 00875 switch( ctx->padding ) 00876 { 00877 #if defined(MBEDTLS_PKCS1_V15) 00878 case MBEDTLS_RSA_PKCS_V15: 00879 return mbedtls_rsa_rsaes_pkcs1_v15_decrypt( ctx, f_rng, p_rng, mode, olen, 00880 input, output, output_max_len ); 00881 #endif 00882 00883 #if defined(MBEDTLS_PKCS1_V21) 00884 case MBEDTLS_RSA_PKCS_V21: 00885 return mbedtls_rsa_rsaes_oaep_decrypt( ctx, f_rng, p_rng, mode, NULL, 0, 00886 olen, input, output, 00887 output_max_len ); 00888 #endif 00889 00890 default: 00891 return( MBEDTLS_ERR_RSA_INVALID_PADDING ); 00892 } 00893 } 00894 00895 #if defined(MBEDTLS_PKCS1_V21) 00896 /* 00897 * Implementation of the PKCS#1 v2.1 RSASSA-PSS-SIGN function 00898 */ 00899 int mbedtls_rsa_rsassa_pss_sign( mbedtls_rsa_context *ctx, 00900 int (*f_rng)(void *, unsigned char *, size_t), 00901 void *p_rng, 00902 int mode, 00903 mbedtls_md_type_t md_alg, 00904 unsigned int hashlen, 00905 const unsigned char *hash, 00906 unsigned char *sig ) 00907 { 00908 size_t olen; 00909 unsigned char *p = sig; 00910 unsigned char salt[MBEDTLS_MD_MAX_SIZE]; 00911 unsigned int slen, hlen, offset = 0; 00912 int ret; 00913 size_t msb; 00914 const mbedtls_md_info_t *md_info; 00915 mbedtls_md_context_t md_ctx; 00916 00917 if( mode == MBEDTLS_RSA_PRIVATE && ctx->padding != MBEDTLS_RSA_PKCS_V21 ) 00918 return( MBEDTLS_ERR_RSA_BAD_INPUT_DATA ); 00919 00920 if( f_rng == NULL ) 00921 return( MBEDTLS_ERR_RSA_BAD_INPUT_DATA ); 00922 00923 olen = ctx->len ; 00924 00925 if( md_alg != MBEDTLS_MD_NONE ) 00926 { 00927 // Gather length of hash to sign 00928 // 00929 md_info = mbedtls_md_info_from_type( md_alg ); 00930 if( md_info == NULL ) 00931 return( MBEDTLS_ERR_RSA_BAD_INPUT_DATA ); 00932 00933 hashlen = mbedtls_md_get_size( md_info ); 00934 } 00935 00936 md_info = mbedtls_md_info_from_type( (mbedtls_md_type_t) ctx->hash_id ); 00937 if( md_info == NULL ) 00938 return( MBEDTLS_ERR_RSA_BAD_INPUT_DATA ); 00939 00940 hlen = mbedtls_md_get_size( md_info ); 00941 slen = hlen; 00942 00943 if( olen < hlen + slen + 2 ) 00944 return( MBEDTLS_ERR_RSA_BAD_INPUT_DATA ); 00945 00946 memset( sig, 0, olen ); 00947 00948 // Generate salt of length slen 00949 // 00950 if( ( ret = f_rng( p_rng, salt, slen ) ) != 0 ) 00951 return( MBEDTLS_ERR_RSA_RNG_FAILED + ret ); 00952 00953 // Note: EMSA-PSS encoding is over the length of N - 1 bits 00954 // 00955 msb = mbedtls_mpi_bitlen( &ctx->N ) - 1; 00956 p += olen - hlen * 2 - 2; 00957 *p++ = 0x01; 00958 memcpy( p, salt, slen ); 00959 p += slen; 00960 00961 mbedtls_md_init( &md_ctx ); 00962 mbedtls_md_setup( &md_ctx, md_info, 0 ); 00963 00964 // Generate H = Hash( M' ) 00965 // 00966 mbedtls_md_starts( &md_ctx ); 00967 mbedtls_md_update( &md_ctx, p, 8 ); 00968 mbedtls_md_update( &md_ctx, hash, hashlen ); 00969 mbedtls_md_update( &md_ctx, salt, slen ); 00970 mbedtls_md_finish( &md_ctx, p ); 00971 00972 // Compensate for boundary condition when applying mask 00973 // 00974 if( msb % 8 == 0 ) 00975 offset = 1; 00976 00977 // maskedDB: Apply dbMask to DB 00978 // 00979 mgf_mask( sig + offset, olen - hlen - 1 - offset, p, hlen, &md_ctx ); 00980 00981 mbedtls_md_free( &md_ctx ); 00982 00983 msb = mbedtls_mpi_bitlen( &ctx->N ) - 1; 00984 sig[0] &= 0xFF >> ( olen * 8 - msb ); 00985 00986 p += hlen; 00987 *p++ = 0xBC; 00988 00989 return( ( mode == MBEDTLS_RSA_PUBLIC ) 00990 ? mbedtls_rsa_public( ctx, sig, sig ) 00991 : mbedtls_rsa_private( ctx, f_rng, p_rng, sig, sig ) ); 00992 } 00993 #endif /* MBEDTLS_PKCS1_V21 */ 00994 00995 #if defined(MBEDTLS_PKCS1_V15) 00996 /* 00997 * Implementation of the PKCS#1 v2.1 RSASSA-PKCS1-V1_5-SIGN function 00998 */ 00999 /* 01000 * Do an RSA operation to sign the message digest 01001 */ 01002 int mbedtls_rsa_rsassa_pkcs1_v15_sign( mbedtls_rsa_context *ctx, 01003 int (*f_rng)(void *, unsigned char *, size_t), 01004 void *p_rng, 01005 int mode, 01006 mbedtls_md_type_t md_alg, 01007 unsigned int hashlen, 01008 const unsigned char *hash, 01009 unsigned char *sig ) 01010 { 01011 size_t nb_pad, olen, oid_size = 0; 01012 unsigned char *p = sig; 01013 const char *oid = NULL; 01014 unsigned char *sig_try = NULL, *verif = NULL; 01015 size_t i; 01016 unsigned char diff; 01017 volatile unsigned char diff_no_optimize; 01018 int ret; 01019 01020 if( mode == MBEDTLS_RSA_PRIVATE && ctx->padding != MBEDTLS_RSA_PKCS_V15 ) 01021 return( MBEDTLS_ERR_RSA_BAD_INPUT_DATA ); 01022 01023 olen = ctx->len ; 01024 nb_pad = olen - 3; 01025 01026 if( md_alg != MBEDTLS_MD_NONE ) 01027 { 01028 const mbedtls_md_info_t *md_info = mbedtls_md_info_from_type( md_alg ); 01029 if( md_info == NULL ) 01030 return( MBEDTLS_ERR_RSA_BAD_INPUT_DATA ); 01031 01032 if( mbedtls_oid_get_oid_by_md( md_alg, &oid, &oid_size ) != 0 ) 01033 return( MBEDTLS_ERR_RSA_BAD_INPUT_DATA ); 01034 01035 nb_pad -= 10 + oid_size; 01036 01037 hashlen = mbedtls_md_get_size( md_info ); 01038 } 01039 01040 nb_pad -= hashlen; 01041 01042 if( ( nb_pad < 8 ) || ( nb_pad > olen ) ) 01043 return( MBEDTLS_ERR_RSA_BAD_INPUT_DATA ); 01044 01045 *p++ = 0; 01046 *p++ = MBEDTLS_RSA_SIGN; 01047 memset( p, 0xFF, nb_pad ); 01048 p += nb_pad; 01049 *p++ = 0; 01050 01051 if( md_alg == MBEDTLS_MD_NONE ) 01052 { 01053 memcpy( p, hash, hashlen ); 01054 } 01055 else 01056 { 01057 /* 01058 * DigestInfo ::= SEQUENCE { 01059 * digestAlgorithm DigestAlgorithmIdentifier, 01060 * digest Digest } 01061 * 01062 * DigestAlgorithmIdentifier ::= AlgorithmIdentifier 01063 * 01064 * Digest ::= OCTET STRING 01065 */ 01066 *p++ = MBEDTLS_ASN1_SEQUENCE | MBEDTLS_ASN1_CONSTRUCTED; 01067 *p++ = (unsigned char) ( 0x08 + oid_size + hashlen ); 01068 *p++ = MBEDTLS_ASN1_SEQUENCE | MBEDTLS_ASN1_CONSTRUCTED; 01069 *p++ = (unsigned char) ( 0x04 + oid_size ); 01070 *p++ = MBEDTLS_ASN1_OID; 01071 *p++ = oid_size & 0xFF; 01072 memcpy( p, oid, oid_size ); 01073 p += oid_size; 01074 *p++ = MBEDTLS_ASN1_NULL; 01075 *p++ = 0x00; 01076 *p++ = MBEDTLS_ASN1_OCTET_STRING; 01077 *p++ = hashlen; 01078 memcpy( p, hash, hashlen ); 01079 } 01080 01081 if( mode == MBEDTLS_RSA_PUBLIC ) 01082 return( mbedtls_rsa_public( ctx, sig, sig ) ); 01083 01084 /* 01085 * In order to prevent Lenstra's attack, make the signature in a 01086 * temporary buffer and check it before returning it. 01087 */ 01088 sig_try = mbedtls_calloc( 1, ctx->len ); 01089 verif = mbedtls_calloc( 1, ctx->len ); 01090 if( sig_try == NULL || verif == NULL ) 01091 return( MBEDTLS_ERR_MPI_ALLOC_FAILED ); 01092 01093 MBEDTLS_MPI_CHK( mbedtls_rsa_private( ctx, f_rng, p_rng, sig, sig_try ) ); 01094 MBEDTLS_MPI_CHK( mbedtls_rsa_public( ctx, sig_try, verif ) ); 01095 01096 /* Compare in constant time just in case */ 01097 for( diff = 0, i = 0; i < ctx->len ; i++ ) 01098 diff |= verif[i] ^ sig[i]; 01099 diff_no_optimize = diff; 01100 01101 if( diff_no_optimize != 0 ) 01102 { 01103 ret = MBEDTLS_ERR_RSA_PRIVATE_FAILED; 01104 goto cleanup; 01105 } 01106 01107 memcpy( sig, sig_try, ctx->len ); 01108 01109 cleanup: 01110 mbedtls_free( sig_try ); 01111 mbedtls_free( verif ); 01112 01113 return( ret ); 01114 } 01115 #endif /* MBEDTLS_PKCS1_V15 */ 01116 01117 /* 01118 * Do an RSA operation to sign the message digest 01119 */ 01120 int mbedtls_rsa_pkcs1_sign( mbedtls_rsa_context *ctx, 01121 int (*f_rng)(void *, unsigned char *, size_t), 01122 void *p_rng, 01123 int mode, 01124 mbedtls_md_type_t md_alg, 01125 unsigned int hashlen, 01126 const unsigned char *hash, 01127 unsigned char *sig ) 01128 { 01129 switch( ctx->padding ) 01130 { 01131 #if defined(MBEDTLS_PKCS1_V15) 01132 case MBEDTLS_RSA_PKCS_V15: 01133 return mbedtls_rsa_rsassa_pkcs1_v15_sign( ctx, f_rng, p_rng, mode, md_alg, 01134 hashlen, hash, sig ); 01135 #endif 01136 01137 #if defined(MBEDTLS_PKCS1_V21) 01138 case MBEDTLS_RSA_PKCS_V21: 01139 return mbedtls_rsa_rsassa_pss_sign( ctx, f_rng, p_rng, mode, md_alg, 01140 hashlen, hash, sig ); 01141 #endif 01142 01143 default: 01144 return( MBEDTLS_ERR_RSA_INVALID_PADDING ); 01145 } 01146 } 01147 01148 #if defined(MBEDTLS_PKCS1_V21) 01149 /* 01150 * Implementation of the PKCS#1 v2.1 RSASSA-PSS-VERIFY function 01151 */ 01152 int mbedtls_rsa_rsassa_pss_verify_ext( mbedtls_rsa_context *ctx, 01153 int (*f_rng)(void *, unsigned char *, size_t), 01154 void *p_rng, 01155 int mode, 01156 mbedtls_md_type_t md_alg, 01157 unsigned int hashlen, 01158 const unsigned char *hash, 01159 mbedtls_md_type_t mgf1_hash_id, 01160 int expected_salt_len, 01161 const unsigned char *sig ) 01162 { 01163 int ret; 01164 size_t siglen; 01165 unsigned char *p; 01166 unsigned char buf[MBEDTLS_MPI_MAX_SIZE]; 01167 unsigned char result[MBEDTLS_MD_MAX_SIZE]; 01168 unsigned char zeros[8]; 01169 unsigned int hlen; 01170 size_t slen, msb; 01171 const mbedtls_md_info_t *md_info; 01172 mbedtls_md_context_t md_ctx; 01173 01174 if( mode == MBEDTLS_RSA_PRIVATE && ctx->padding != MBEDTLS_RSA_PKCS_V21 ) 01175 return( MBEDTLS_ERR_RSA_BAD_INPUT_DATA ); 01176 01177 siglen = ctx->len ; 01178 01179 if( siglen < 16 || siglen > sizeof( buf ) ) 01180 return( MBEDTLS_ERR_RSA_BAD_INPUT_DATA ); 01181 01182 ret = ( mode == MBEDTLS_RSA_PUBLIC ) 01183 ? mbedtls_rsa_public( ctx, sig, buf ) 01184 : mbedtls_rsa_private( ctx, f_rng, p_rng, sig, buf ); 01185 01186 if( ret != 0 ) 01187 return( ret ); 01188 01189 p = buf; 01190 01191 if( buf[siglen - 1] != 0xBC ) 01192 return( MBEDTLS_ERR_RSA_INVALID_PADDING ); 01193 01194 if( md_alg != MBEDTLS_MD_NONE ) 01195 { 01196 // Gather length of hash to sign 01197 // 01198 md_info = mbedtls_md_info_from_type( md_alg ); 01199 if( md_info == NULL ) 01200 return( MBEDTLS_ERR_RSA_BAD_INPUT_DATA ); 01201 01202 hashlen = mbedtls_md_get_size( md_info ); 01203 } 01204 01205 md_info = mbedtls_md_info_from_type( mgf1_hash_id ); 01206 if( md_info == NULL ) 01207 return( MBEDTLS_ERR_RSA_BAD_INPUT_DATA ); 01208 01209 hlen = mbedtls_md_get_size( md_info ); 01210 slen = siglen - hlen - 1; /* Currently length of salt + padding */ 01211 01212 memset( zeros, 0, 8 ); 01213 01214 // Note: EMSA-PSS verification is over the length of N - 1 bits 01215 // 01216 msb = mbedtls_mpi_bitlen( &ctx->N ) - 1; 01217 01218 // Compensate for boundary condition when applying mask 01219 // 01220 if( msb % 8 == 0 ) 01221 { 01222 p++; 01223 siglen -= 1; 01224 } 01225 if( buf[0] >> ( 8 - siglen * 8 + msb ) ) 01226 return( MBEDTLS_ERR_RSA_BAD_INPUT_DATA ); 01227 01228 mbedtls_md_init( &md_ctx ); 01229 mbedtls_md_setup( &md_ctx, md_info, 0 ); 01230 01231 mgf_mask( p, siglen - hlen - 1, p + siglen - hlen - 1, hlen, &md_ctx ); 01232 01233 buf[0] &= 0xFF >> ( siglen * 8 - msb ); 01234 01235 while( p < buf + siglen && *p == 0 ) 01236 p++; 01237 01238 if( p == buf + siglen || 01239 *p++ != 0x01 ) 01240 { 01241 mbedtls_md_free( &md_ctx ); 01242 return( MBEDTLS_ERR_RSA_INVALID_PADDING ); 01243 } 01244 01245 /* Actual salt len */ 01246 slen -= p - buf; 01247 01248 if( expected_salt_len != MBEDTLS_RSA_SALT_LEN_ANY && 01249 slen != (size_t) expected_salt_len ) 01250 { 01251 mbedtls_md_free( &md_ctx ); 01252 return( MBEDTLS_ERR_RSA_INVALID_PADDING ); 01253 } 01254 01255 // Generate H = Hash( M' ) 01256 // 01257 mbedtls_md_starts( &md_ctx ); 01258 mbedtls_md_update( &md_ctx, zeros, 8 ); 01259 mbedtls_md_update( &md_ctx, hash, hashlen ); 01260 mbedtls_md_update( &md_ctx, p, slen ); 01261 mbedtls_md_finish( &md_ctx, result ); 01262 01263 mbedtls_md_free( &md_ctx ); 01264 01265 if( memcmp( p + slen, result, hlen ) == 0 ) 01266 return( 0 ); 01267 else 01268 return( MBEDTLS_ERR_RSA_VERIFY_FAILED ); 01269 } 01270 01271 /* 01272 * Simplified PKCS#1 v2.1 RSASSA-PSS-VERIFY function 01273 */ 01274 int mbedtls_rsa_rsassa_pss_verify( mbedtls_rsa_context *ctx, 01275 int (*f_rng)(void *, unsigned char *, size_t), 01276 void *p_rng, 01277 int mode, 01278 mbedtls_md_type_t md_alg, 01279 unsigned int hashlen, 01280 const unsigned char *hash, 01281 const unsigned char *sig ) 01282 { 01283 mbedtls_md_type_t mgf1_hash_id = ( ctx->hash_id != MBEDTLS_MD_NONE ) 01284 ? (mbedtls_md_type_t) ctx->hash_id 01285 : md_alg; 01286 01287 return( mbedtls_rsa_rsassa_pss_verify_ext( ctx, f_rng, p_rng, mode, 01288 md_alg, hashlen, hash, 01289 mgf1_hash_id, MBEDTLS_RSA_SALT_LEN_ANY, 01290 sig ) ); 01291 01292 } 01293 #endif /* MBEDTLS_PKCS1_V21 */ 01294 01295 #if defined(MBEDTLS_PKCS1_V15) 01296 /* 01297 * Implementation of the PKCS#1 v2.1 RSASSA-PKCS1-v1_5-VERIFY function 01298 */ 01299 int mbedtls_rsa_rsassa_pkcs1_v15_verify( mbedtls_rsa_context *ctx, 01300 int (*f_rng)(void *, unsigned char *, size_t), 01301 void *p_rng, 01302 int mode, 01303 mbedtls_md_type_t md_alg, 01304 unsigned int hashlen, 01305 const unsigned char *hash, 01306 const unsigned char *sig ) 01307 { 01308 int ret; 01309 size_t len, siglen, asn1_len; 01310 unsigned char *p, *end; 01311 unsigned char buf[MBEDTLS_MPI_MAX_SIZE]; 01312 mbedtls_md_type_t msg_md_alg; 01313 const mbedtls_md_info_t *md_info; 01314 mbedtls_asn1_buf oid; 01315 01316 if( mode == MBEDTLS_RSA_PRIVATE && ctx->padding != MBEDTLS_RSA_PKCS_V15 ) 01317 return( MBEDTLS_ERR_RSA_BAD_INPUT_DATA ); 01318 01319 siglen = ctx->len ; 01320 01321 if( siglen < 16 || siglen > sizeof( buf ) ) 01322 return( MBEDTLS_ERR_RSA_BAD_INPUT_DATA ); 01323 01324 ret = ( mode == MBEDTLS_RSA_PUBLIC ) 01325 ? mbedtls_rsa_public( ctx, sig, buf ) 01326 : mbedtls_rsa_private( ctx, f_rng, p_rng, sig, buf ); 01327 01328 if( ret != 0 ) 01329 return( ret ); 01330 01331 p = buf; 01332 01333 if( *p++ != 0 || *p++ != MBEDTLS_RSA_SIGN ) 01334 return( MBEDTLS_ERR_RSA_INVALID_PADDING ); 01335 01336 while( *p != 0 ) 01337 { 01338 if( p >= buf + siglen - 1 || *p != 0xFF ) 01339 return( MBEDTLS_ERR_RSA_INVALID_PADDING ); 01340 p++; 01341 } 01342 p++; 01343 01344 len = siglen - ( p - buf ); 01345 01346 if( len == hashlen && md_alg == MBEDTLS_MD_NONE ) 01347 { 01348 if( memcmp( p, hash, hashlen ) == 0 ) 01349 return( 0 ); 01350 else 01351 return( MBEDTLS_ERR_RSA_VERIFY_FAILED ); 01352 } 01353 01354 md_info = mbedtls_md_info_from_type( md_alg ); 01355 if( md_info == NULL ) 01356 return( MBEDTLS_ERR_RSA_BAD_INPUT_DATA ); 01357 hashlen = mbedtls_md_get_size( md_info ); 01358 01359 end = p + len; 01360 01361 // Parse the ASN.1 structure inside the PKCS#1 v1.5 structure 01362 // 01363 if( ( ret = mbedtls_asn1_get_tag( &p, end, &asn1_len, 01364 MBEDTLS_ASN1_CONSTRUCTED | MBEDTLS_ASN1_SEQUENCE ) ) != 0 ) 01365 return( MBEDTLS_ERR_RSA_VERIFY_FAILED ); 01366 01367 if( asn1_len + 2 != len ) 01368 return( MBEDTLS_ERR_RSA_VERIFY_FAILED ); 01369 01370 if( ( ret = mbedtls_asn1_get_tag( &p, end, &asn1_len, 01371 MBEDTLS_ASN1_CONSTRUCTED | MBEDTLS_ASN1_SEQUENCE ) ) != 0 ) 01372 return( MBEDTLS_ERR_RSA_VERIFY_FAILED ); 01373 01374 if( asn1_len + 6 + hashlen != len ) 01375 return( MBEDTLS_ERR_RSA_VERIFY_FAILED ); 01376 01377 if( ( ret = mbedtls_asn1_get_tag( &p, end, &oid.len, MBEDTLS_ASN1_OID ) ) != 0 ) 01378 return( MBEDTLS_ERR_RSA_VERIFY_FAILED ); 01379 01380 oid.p = p; 01381 p += oid.len; 01382 01383 if( mbedtls_oid_get_md_alg( &oid, &msg_md_alg ) != 0 ) 01384 return( MBEDTLS_ERR_RSA_VERIFY_FAILED ); 01385 01386 if( md_alg != msg_md_alg ) 01387 return( MBEDTLS_ERR_RSA_VERIFY_FAILED ); 01388 01389 /* 01390 * assume the algorithm parameters must be NULL 01391 */ 01392 if( ( ret = mbedtls_asn1_get_tag( &p, end, &asn1_len, MBEDTLS_ASN1_NULL ) ) != 0 ) 01393 return( MBEDTLS_ERR_RSA_VERIFY_FAILED ); 01394 01395 if( ( ret = mbedtls_asn1_get_tag( &p, end, &asn1_len, MBEDTLS_ASN1_OCTET_STRING ) ) != 0 ) 01396 return( MBEDTLS_ERR_RSA_VERIFY_FAILED ); 01397 01398 if( asn1_len != hashlen ) 01399 return( MBEDTLS_ERR_RSA_VERIFY_FAILED ); 01400 01401 if( memcmp( p, hash, hashlen ) != 0 ) 01402 return( MBEDTLS_ERR_RSA_VERIFY_FAILED ); 01403 01404 p += hashlen; 01405 01406 if( p != end ) 01407 return( MBEDTLS_ERR_RSA_VERIFY_FAILED ); 01408 01409 return( 0 ); 01410 } 01411 #endif /* MBEDTLS_PKCS1_V15 */ 01412 01413 /* 01414 * Do an RSA operation and check the message digest 01415 */ 01416 int mbedtls_rsa_pkcs1_verify( mbedtls_rsa_context *ctx, 01417 int (*f_rng)(void *, unsigned char *, size_t), 01418 void *p_rng, 01419 int mode, 01420 mbedtls_md_type_t md_alg, 01421 unsigned int hashlen, 01422 const unsigned char *hash, 01423 const unsigned char *sig ) 01424 { 01425 switch( ctx->padding ) 01426 { 01427 #if defined(MBEDTLS_PKCS1_V15) 01428 case MBEDTLS_RSA_PKCS_V15: 01429 return mbedtls_rsa_rsassa_pkcs1_v15_verify( ctx, f_rng, p_rng, mode, md_alg, 01430 hashlen, hash, sig ); 01431 #endif 01432 01433 #if defined(MBEDTLS_PKCS1_V21) 01434 case MBEDTLS_RSA_PKCS_V21: 01435 return mbedtls_rsa_rsassa_pss_verify( ctx, f_rng, p_rng, mode, md_alg, 01436 hashlen, hash, sig ); 01437 #endif 01438 01439 default: 01440 return( MBEDTLS_ERR_RSA_INVALID_PADDING ); 01441 } 01442 } 01443 01444 /* 01445 * Copy the components of an RSA key 01446 */ 01447 int mbedtls_rsa_copy( mbedtls_rsa_context *dst, const mbedtls_rsa_context *src ) 01448 { 01449 int ret; 01450 01451 dst->ver = src->ver ; 01452 dst->len = src->len ; 01453 01454 MBEDTLS_MPI_CHK( mbedtls_mpi_copy( &dst->N , &src->N ) ); 01455 MBEDTLS_MPI_CHK( mbedtls_mpi_copy( &dst->E , &src->E ) ); 01456 01457 MBEDTLS_MPI_CHK( mbedtls_mpi_copy( &dst->D , &src->D ) ); 01458 MBEDTLS_MPI_CHK( mbedtls_mpi_copy( &dst->P , &src->P ) ); 01459 MBEDTLS_MPI_CHK( mbedtls_mpi_copy( &dst->Q , &src->Q ) ); 01460 MBEDTLS_MPI_CHK( mbedtls_mpi_copy( &dst->DP , &src->DP ) ); 01461 MBEDTLS_MPI_CHK( mbedtls_mpi_copy( &dst->DQ , &src->DQ ) ); 01462 MBEDTLS_MPI_CHK( mbedtls_mpi_copy( &dst->QP , &src->QP ) ); 01463 01464 MBEDTLS_MPI_CHK( mbedtls_mpi_copy( &dst->RN , &src->RN ) ); 01465 MBEDTLS_MPI_CHK( mbedtls_mpi_copy( &dst->RP , &src->RP ) ); 01466 MBEDTLS_MPI_CHK( mbedtls_mpi_copy( &dst->RQ , &src->RQ ) ); 01467 01468 MBEDTLS_MPI_CHK( mbedtls_mpi_copy( &dst->Vi , &src->Vi ) ); 01469 MBEDTLS_MPI_CHK( mbedtls_mpi_copy( &dst->Vf , &src->Vf ) ); 01470 01471 dst->padding = src->padding ; 01472 dst->hash_id = src->hash_id ; 01473 01474 cleanup: 01475 if( ret != 0 ) 01476 mbedtls_rsa_free( dst ); 01477 01478 return( ret ); 01479 } 01480 01481 /* 01482 * Free the components of an RSA key 01483 */ 01484 void mbedtls_rsa_free( mbedtls_rsa_context *ctx ) 01485 { 01486 mbedtls_mpi_free( &ctx->Vi ); mbedtls_mpi_free( &ctx->Vf ); 01487 mbedtls_mpi_free( &ctx->RQ ); mbedtls_mpi_free( &ctx->RP ); mbedtls_mpi_free( &ctx->RN ); 01488 mbedtls_mpi_free( &ctx->QP ); mbedtls_mpi_free( &ctx->DQ ); mbedtls_mpi_free( &ctx->DP ); 01489 mbedtls_mpi_free( &ctx->Q ); mbedtls_mpi_free( &ctx->P ); mbedtls_mpi_free( &ctx->D ); 01490 mbedtls_mpi_free( &ctx->E ); mbedtls_mpi_free( &ctx->N ); 01491 01492 #if defined(MBEDTLS_THREADING_C) 01493 mbedtls_mutex_free( &ctx->mutex ); 01494 #endif 01495 } 01496 01497 #if defined(MBEDTLS_SELF_TEST) 01498 01499 #include "mbedtls/sha1.h" 01500 01501 /* 01502 * Example RSA-1024 keypair, for test purposes 01503 */ 01504 #define KEY_LEN 128 01505 01506 #define RSA_N "9292758453063D803DD603D5E777D788" \ 01507 "8ED1D5BF35786190FA2F23EBC0848AEA" \ 01508 "DDA92CA6C3D80B32C4D109BE0F36D6AE" \ 01509 "7130B9CED7ACDF54CFC7555AC14EEBAB" \ 01510 "93A89813FBF3C4F8066D2D800F7C38A8" \ 01511 "1AE31942917403FF4946B0A83D3D3E05" \ 01512 "EE57C6F5F5606FB5D4BC6CD34EE0801A" \ 01513 "5E94BB77B07507233A0BC7BAC8F90F79" 01514 01515 #define RSA_E "10001" 01516 01517 #define RSA_D "24BF6185468786FDD303083D25E64EFC" \ 01518 "66CA472BC44D253102F8B4A9D3BFA750" \ 01519 "91386C0077937FE33FA3252D28855837" \ 01520 "AE1B484A8A9A45F7EE8C0C634F99E8CD" \ 01521 "DF79C5CE07EE72C7F123142198164234" \ 01522 "CABB724CF78B8173B9F880FC86322407" \ 01523 "AF1FEDFDDE2BEB674CA15F3E81A1521E" \ 01524 "071513A1E85B5DFA031F21ECAE91A34D" 01525 01526 #define RSA_P "C36D0EB7FCD285223CFB5AABA5BDA3D8" \ 01527 "2C01CAD19EA484A87EA4377637E75500" \ 01528 "FCB2005C5C7DD6EC4AC023CDA285D796" \ 01529 "C3D9E75E1EFC42488BB4F1D13AC30A57" 01530 01531 #define RSA_Q "C000DF51A7C77AE8D7C7370C1FF55B69" \ 01532 "E211C2B9E5DB1ED0BF61D0D9899620F4" \ 01533 "910E4168387E3C30AA1E00C339A79508" \ 01534 "8452DD96A9A5EA5D9DCA68DA636032AF" 01535 01536 #define RSA_DP "C1ACF567564274FB07A0BBAD5D26E298" \ 01537 "3C94D22288ACD763FD8E5600ED4A702D" \ 01538 "F84198A5F06C2E72236AE490C93F07F8" \ 01539 "3CC559CD27BC2D1CA488811730BB5725" 01540 01541 #define RSA_DQ "4959CBF6F8FEF750AEE6977C155579C7" \ 01542 "D8AAEA56749EA28623272E4F7D0592AF" \ 01543 "7C1F1313CAC9471B5C523BFE592F517B" \ 01544 "407A1BD76C164B93DA2D32A383E58357" 01545 01546 #define RSA_QP "9AE7FBC99546432DF71896FC239EADAE" \ 01547 "F38D18D2B2F0E2DD275AA977E2BF4411" \ 01548 "F5A3B2A5D33605AEBBCCBA7FEB9F2D2F" \ 01549 "A74206CEC169D74BF5A8C50D6F48EA08" 01550 01551 #define PT_LEN 24 01552 #define RSA_PT "\xAA\xBB\xCC\x03\x02\x01\x00\xFF\xFF\xFF\xFF\xFF" \ 01553 "\x11\x22\x33\x0A\x0B\x0C\xCC\xDD\xDD\xDD\xDD\xDD" 01554 01555 #if defined(MBEDTLS_PKCS1_V15) 01556 static int myrand( void *rng_state, unsigned char *output, size_t len ) 01557 { 01558 #if !defined(__OpenBSD__) 01559 size_t i; 01560 01561 if( rng_state != NULL ) 01562 rng_state = NULL; 01563 01564 for( i = 0; i < len; ++i ) 01565 output[i] = rand(); 01566 #else 01567 if( rng_state != NULL ) 01568 rng_state = NULL; 01569 01570 arc4random_buf( output, len ); 01571 #endif /* !OpenBSD */ 01572 01573 return( 0 ); 01574 } 01575 #endif /* MBEDTLS_PKCS1_V15 */ 01576 01577 /* 01578 * Checkup routine 01579 */ 01580 int mbedtls_rsa_self_test( int verbose ) 01581 { 01582 int ret = 0; 01583 #if defined(MBEDTLS_PKCS1_V15) 01584 size_t len; 01585 mbedtls_rsa_context rsa; 01586 unsigned char rsa_plaintext[PT_LEN]; 01587 unsigned char rsa_decrypted[PT_LEN]; 01588 unsigned char rsa_ciphertext[KEY_LEN]; 01589 #if defined(MBEDTLS_SHA1_C) 01590 unsigned char sha1sum[20]; 01591 #endif 01592 01593 mbedtls_rsa_init( &rsa, MBEDTLS_RSA_PKCS_V15, 0 ); 01594 01595 rsa.len = KEY_LEN; 01596 MBEDTLS_MPI_CHK( mbedtls_mpi_read_string( &rsa.N , 16, RSA_N ) ); 01597 MBEDTLS_MPI_CHK( mbedtls_mpi_read_string( &rsa.E , 16, RSA_E ) ); 01598 MBEDTLS_MPI_CHK( mbedtls_mpi_read_string( &rsa.D , 16, RSA_D ) ); 01599 MBEDTLS_MPI_CHK( mbedtls_mpi_read_string( &rsa.P , 16, RSA_P ) ); 01600 MBEDTLS_MPI_CHK( mbedtls_mpi_read_string( &rsa.Q , 16, RSA_Q ) ); 01601 MBEDTLS_MPI_CHK( mbedtls_mpi_read_string( &rsa.DP , 16, RSA_DP ) ); 01602 MBEDTLS_MPI_CHK( mbedtls_mpi_read_string( &rsa.DQ , 16, RSA_DQ ) ); 01603 MBEDTLS_MPI_CHK( mbedtls_mpi_read_string( &rsa.QP , 16, RSA_QP ) ); 01604 01605 if( verbose != 0 ) 01606 mbedtls_printf( " RSA key validation: " ); 01607 01608 if( mbedtls_rsa_check_pubkey( &rsa ) != 0 || 01609 mbedtls_rsa_check_privkey( &rsa ) != 0 ) 01610 { 01611 if( verbose != 0 ) 01612 mbedtls_printf( "failed\n" ); 01613 01614 return( 1 ); 01615 } 01616 01617 if( verbose != 0 ) 01618 mbedtls_printf( "passed\n PKCS#1 encryption : " ); 01619 01620 memcpy( rsa_plaintext, RSA_PT, PT_LEN ); 01621 01622 if( mbedtls_rsa_pkcs1_encrypt( &rsa, myrand, NULL, MBEDTLS_RSA_PUBLIC, PT_LEN, 01623 rsa_plaintext, rsa_ciphertext ) != 0 ) 01624 { 01625 if( verbose != 0 ) 01626 mbedtls_printf( "failed\n" ); 01627 01628 return( 1 ); 01629 } 01630 01631 if( verbose != 0 ) 01632 mbedtls_printf( "passed\n PKCS#1 decryption : " ); 01633 01634 if( mbedtls_rsa_pkcs1_decrypt( &rsa, myrand, NULL, MBEDTLS_RSA_PRIVATE, &len, 01635 rsa_ciphertext, rsa_decrypted, 01636 sizeof(rsa_decrypted) ) != 0 ) 01637 { 01638 if( verbose != 0 ) 01639 mbedtls_printf( "failed\n" ); 01640 01641 return( 1 ); 01642 } 01643 01644 if( memcmp( rsa_decrypted, rsa_plaintext, len ) != 0 ) 01645 { 01646 if( verbose != 0 ) 01647 mbedtls_printf( "failed\n" ); 01648 01649 return( 1 ); 01650 } 01651 01652 if( verbose != 0 ) 01653 mbedtls_printf( "passed\n" ); 01654 01655 #if defined(MBEDTLS_SHA1_C) 01656 if( verbose != 0 ) 01657 mbedtls_printf( "PKCS#1 data sign : " ); 01658 01659 mbedtls_sha1( rsa_plaintext, PT_LEN, sha1sum ); 01660 01661 if( mbedtls_rsa_pkcs1_sign( &rsa, myrand, NULL, MBEDTLS_RSA_PRIVATE, MBEDTLS_MD_SHA1, 0, 01662 sha1sum, rsa_ciphertext ) != 0 ) 01663 { 01664 if( verbose != 0 ) 01665 mbedtls_printf( "failed\n" ); 01666 01667 return( 1 ); 01668 } 01669 01670 if( verbose != 0 ) 01671 mbedtls_printf( "passed\n PKCS#1 sig. verify: " ); 01672 01673 if( mbedtls_rsa_pkcs1_verify( &rsa, NULL, NULL, MBEDTLS_RSA_PUBLIC, MBEDTLS_MD_SHA1, 0, 01674 sha1sum, rsa_ciphertext ) != 0 ) 01675 { 01676 if( verbose != 0 ) 01677 mbedtls_printf( "failed\n" ); 01678 01679 return( 1 ); 01680 } 01681 01682 if( verbose != 0 ) 01683 mbedtls_printf( "passed\n" ); 01684 #endif /* MBEDTLS_SHA1_C */ 01685 01686 if( verbose != 0 ) 01687 mbedtls_printf( "\n" ); 01688 01689 cleanup: 01690 mbedtls_rsa_free( &rsa ); 01691 #else /* MBEDTLS_PKCS1_V15 */ 01692 ((void) verbose); 01693 #endif /* MBEDTLS_PKCS1_V15 */ 01694 return( ret ); 01695 } 01696 01697 #endif /* MBEDTLS_SELF_TEST */ 01698 01699 #endif /* MBEDTLS_RSA_C */
Generated on Tue Jul 12 2022 12:52:46 by
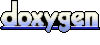