mbedtls ported to mbed-classic
Fork of mbedtls by
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 /* 00002 * Self-test demonstration program 00003 * 00004 * Copyright (C) 2006-2015, ARM Limited, All Rights Reserved 00005 * SPDX-License-Identifier: Apache-2.0 00006 * 00007 * Licensed under the Apache License, Version 2.0 (the "License"); you may 00008 * not use this file except in compliance with the License. 00009 * You may obtain a copy of the License at 00010 * 00011 * http://www.apache.org/licenses/LICENSE-2.0 00012 * 00013 * Unless required by applicable law or agreed to in writing, software 00014 * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT 00015 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00016 * See the License for the specific language governing permissions and 00017 * limitations under the License. 00018 * 00019 * This file is part of mbed TLS (https://tls.mbed.org) 00020 */ 00021 00022 #if !defined(POLARSSL_CONFIG_FILE) 00023 #include "mbedtls/config.h" 00024 #else 00025 #include MBEDTLS_CONFIG_FILE 00026 #endif 00027 00028 #include "mbedtls/entropy.h" 00029 #include "mbedtls/hmac_drbg.h" 00030 #include "mbedtls/ctr_drbg.h" 00031 #include "mbedtls/dhm.h" 00032 #include "mbedtls/gcm.h" 00033 #include "mbedtls/ccm.h" 00034 #include "mbedtls/md2.h" 00035 #include "mbedtls/md4.h" 00036 #include "mbedtls/md5.h" 00037 #include "mbedtls/ripemd160.h" 00038 #include "mbedtls/sha1.h" 00039 #include "mbedtls/sha256.h" 00040 #include "mbedtls/sha512.h" 00041 #include "mbedtls/arc4.h" 00042 #include "mbedtls/des.h" 00043 #include "mbedtls/aes.h" 00044 #include "mbedtls/camellia.h" 00045 #include "mbedtls/base64.h" 00046 #include "mbedtls/bignum.h" 00047 #include "mbedtls/rsa.h" 00048 #include "mbedtls/x509.h" 00049 #include "mbedtls/xtea.h" 00050 #include "mbedtls/pkcs5.h" 00051 #include "mbedtls/ecp.h" 00052 00053 #include <stdio.h> 00054 #include <string.h> 00055 00056 #if defined(MBEDTLS_PLATFORM_C) 00057 #include "mbedtls/platform.h" 00058 #else 00059 #include <stdio.h> 00060 #define mbedtls_printf printf 00061 #endif 00062 00063 #if defined(MBEDTLS_MEMORY_BUFFER_ALLOC_C) 00064 #include "mbedtls/memory_buffer_alloc.h" 00065 #endif 00066 00067 int selftest( int argc, char *argv[] ) 00068 { 00069 int ret = 0, v; 00070 #if defined(MBEDTLS_MEMORY_BUFFER_ALLOC_C) 00071 unsigned char buf[1000000]; 00072 #endif 00073 00074 if( argc == 2 && strcmp( argv[1], "-quiet" ) == 0 ) 00075 v = 0; 00076 else 00077 { 00078 v = 1; 00079 mbedtls_printf( "\n" ); 00080 } 00081 00082 #if defined(MBEDTLS_SELF_TEST) 00083 00084 #if defined(MBEDTLS_MEMORY_BUFFER_ALLOC_C) 00085 mbedtls_memory_buffer_alloc_init( buf, sizeof(buf) ); 00086 #endif 00087 00088 #if defined(MBEDTLS_MD2_C) 00089 if( ( ret = mbedtls_md2_self_test( v ) ) != 0 ) 00090 return( ret ); 00091 #endif 00092 00093 #if defined(MBEDTLS_MD4_C) 00094 if( ( ret = mbedtls_md4_self_test( v ) ) != 0 ) 00095 return( ret ); 00096 #endif 00097 00098 #if defined(MBEDTLS_MD5_C) 00099 if( ( ret = mbedtls_md5_self_test( v ) ) != 0 ) 00100 return( ret ); 00101 #endif 00102 00103 #if defined(MBEDTLS_RIPEMD160_C) 00104 if( ( ret = mbedtls_ripemd160_self_test( v ) ) != 0 ) 00105 return( ret ); 00106 #endif 00107 00108 #if defined(MBEDTLS_SHA1_C) 00109 if( ( ret = mbedtls_sha1_self_test( v ) ) != 0 ) 00110 return( ret ); 00111 #endif 00112 00113 #if defined(MBEDTLS_SHA256_C) 00114 if( ( ret = mbedtls_sha256_self_test( v ) ) != 0 ) 00115 return( ret ); 00116 #endif 00117 00118 #if defined(MBEDTLS_SHA512_C) 00119 if( ( ret = mbedtls_sha512_self_test( v ) ) != 0 ) 00120 return( ret ); 00121 #endif 00122 00123 #if defined(MBEDTLS_ARC4_C) 00124 if( ( ret = mbedtls_arc4_self_test( v ) ) != 0 ) 00125 return( ret ); 00126 #endif 00127 00128 #if defined(MBEDTLS_DES_C) 00129 if( ( ret = mbedtls_des_self_test( v ) ) != 0 ) 00130 return( ret ); 00131 #endif 00132 00133 #if defined(MBEDTLS_AES_C) 00134 if( ( ret = mbedtls_aes_self_test( v ) ) != 0 ) 00135 return( ret ); 00136 #endif 00137 00138 #if defined(MBEDTLS_GCM_C) && defined(MBEDTLS_AES_C) 00139 if( ( ret = mbedtls_gcm_self_test( v ) ) != 0 ) 00140 return( ret ); 00141 #endif 00142 00143 #if defined(MBEDTLS_CCM_C) && defined(MBEDTLS_AES_C) 00144 if( ( ret = mbedtls_ccm_self_test( v ) ) != 0 ) 00145 return( ret ); 00146 #endif 00147 00148 #if defined(MBEDTLS_BASE64_C) 00149 if( ( ret = mbedtls_base64_self_test( v ) ) != 0 ) 00150 return( ret ); 00151 #endif 00152 00153 #if defined(MBEDTLS_BIGNUM_C) 00154 if( ( ret = mbedtls_mpi_self_test( v ) ) != 0 ) 00155 return( ret ); 00156 #endif 00157 00158 #if defined(MBEDTLS_RSA_C) 00159 if( ( ret = mbedtls_rsa_self_test( v ) ) != 0 ) 00160 return( ret ); 00161 #endif 00162 00163 #if defined(MBEDTLS_X509_USE_C) 00164 if( ( ret = mbedtls_x509_self_test( v ) ) != 0 ) 00165 return( ret ); 00166 #endif 00167 00168 #if defined(MBEDTLS_XTEA_C) 00169 if( ( ret = mbedtls_xtea_self_test( v ) ) != 0 ) 00170 return( ret ); 00171 #endif 00172 00173 #if defined(MBEDTLS_CAMELLIA_C) 00174 if( ( ret = mbedtls_camellia_self_test( v ) ) != 0 ) 00175 return( ret ); 00176 #endif 00177 00178 #if defined(MBEDTLS_CTR_DRBG_C) 00179 if( ( ret = mbedtls_ctr_drbg_self_test( v ) ) != 0 ) 00180 return( ret ); 00181 #endif 00182 00183 #if defined(MBEDTLS_HMAC_DRBG_C) 00184 if( ( ret = mbedtls_hmac_drbg_self_test( v ) ) != 0 ) 00185 return( ret ); 00186 #endif 00187 00188 #if defined(MBEDTLS_ECP_C) 00189 if( ( ret = mbedtls_ecp_self_test( v ) ) != 0 ) 00190 return( ret ); 00191 #endif 00192 00193 #if defined(MBEDTLS_DHM_C) 00194 if( ( ret = mbedtls_dhm_self_test( v ) ) != 0 ) 00195 return( ret ); 00196 #endif 00197 00198 #if defined(MBEDTLS_ENTROPY_C) 00199 if( ( ret = mbedtls_entropy_self_test( v ) ) != 0 ) 00200 return( ret ); 00201 #endif 00202 00203 #if defined(MBEDTLS_PKCS5_C) 00204 if( ( ret = mbedtls_pkcs5_self_test( v ) ) != 0 ) 00205 return( ret ); 00206 #endif 00207 00208 #if defined(MBEDTLS_TIMING_C) 00209 if( ( ret = mbedtls_timing_self_test( v ) ) != 0 ) 00210 return( ret ); 00211 #endif 00212 00213 #else 00214 mbedtls_printf( " POLARSSL_SELF_TEST not defined.\n" ); 00215 #endif 00216 00217 if( v != 0 ) 00218 { 00219 #if defined(MBEDTLS_MEMORY_BUFFER_ALLOC_C) && defined(MBEDTLS_MEMORY_DEBUG) 00220 mbedtls_memory_buffer_alloc_status(); 00221 #endif 00222 } 00223 00224 #if defined(MBEDTLS_MEMORY_BUFFER_ALLOC_C) 00225 mbedtls_memory_buffer_alloc_free(); 00226 00227 if( ( ret = mbedtls_memory_buffer_alloc_self_test( v ) ) != 0 ) 00228 return( ret ); 00229 #endif 00230 00231 if( v != 0 ) 00232 { 00233 mbedtls_printf( " [ All tests passed ]\n\n" ); 00234 #if defined(_WIN32) 00235 mbedtls_printf( " Press Enter to exit this program.\n" ); 00236 fflush( stdout ); getchar(); 00237 #endif 00238 } 00239 00240 return( ret ); 00241 } 00242 00243 #if defined(TARGET_LIKE_MBED) 00244 00245 #include "test_env.h" 00246 00247 int main() { 00248 greentea_send_kv("description", "mbed TLS selftest program"); 00249 GREENTEA_SETUP(40, "default_auto"); 00250 GREENTEA_TESTSUITE_RESULT(selftest(0, NULL) == 0); 00251 } 00252 00253 #else 00254 00255 int main() { 00256 return selftest(0, NULL); 00257 } 00258 00259 #endif
Generated on Tue Jul 12 2022 12:52:43 by
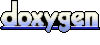