mbedtls ported to mbed-classic
Fork of mbedtls by
aes.h File Reference
AES block cipher. More...
Go to the source code of this file.
Data Structures | |
struct | mbedtls_aes_context |
AES context structure. More... | |
Functions | |
void | mbedtls_aes_init (mbedtls_aes_context *ctx) |
Initialize AES context. | |
void | mbedtls_aes_free (mbedtls_aes_context *ctx) |
Clear AES context. | |
int | mbedtls_aes_setkey_enc (mbedtls_aes_context *ctx, const unsigned char *key, unsigned int keybits) |
AES key schedule (encryption) | |
int | mbedtls_aes_setkey_dec (mbedtls_aes_context *ctx, const unsigned char *key, unsigned int keybits) |
AES key schedule (decryption) | |
int | mbedtls_aes_crypt_ecb (mbedtls_aes_context *ctx, int mode, const unsigned char input[16], unsigned char output[16]) |
AES-ECB block encryption/decryption. | |
int | mbedtls_aes_crypt_cbc (mbedtls_aes_context *ctx, int mode, size_t length, unsigned char iv[16], const unsigned char *input, unsigned char *output) |
AES-CBC buffer encryption/decryption Length should be a multiple of the block size (16 bytes) | |
int | mbedtls_aes_crypt_cfb128 (mbedtls_aes_context *ctx, int mode, size_t length, size_t *iv_off, unsigned char iv[16], const unsigned char *input, unsigned char *output) |
AES-CFB128 buffer encryption/decryption. | |
int | mbedtls_aes_crypt_cfb8 (mbedtls_aes_context *ctx, int mode, size_t length, unsigned char iv[16], const unsigned char *input, unsigned char *output) |
AES-CFB8 buffer encryption/decryption. | |
int | mbedtls_aes_crypt_ctr (mbedtls_aes_context *ctx, size_t length, size_t *nc_off, unsigned char nonce_counter[16], unsigned char stream_block[16], const unsigned char *input, unsigned char *output) |
AES-CTR buffer encryption/decryption. | |
void | mbedtls_aes_encrypt (mbedtls_aes_context *ctx, const unsigned char input[16], unsigned char output[16]) |
Internal AES block encryption function (Only exposed to allow overriding it, see MBEDTLS_AES_ENCRYPT_ALT) | |
void | mbedtls_aes_decrypt (mbedtls_aes_context *ctx, const unsigned char input[16], unsigned char output[16]) |
Internal AES block decryption function (Only exposed to allow overriding it, see MBEDTLS_AES_DECRYPT_ALT) | |
int | mbedtls_aes_self_test (int verbose) |
Checkup routine. |
Detailed Description
AES block cipher.
Copyright (C) 2006-2015, ARM Limited, All Rights Reserved SPDX-License-Identifier: Apache-2.0
Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance with the License. You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software distributed under the License is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions and limitations under the License.
This file is part of mbed TLS (https://tls.mbed.org)
Definition in file aes.h.
Function Documentation
int mbedtls_aes_crypt_cbc | ( | mbedtls_aes_context * | ctx, |
int | mode, | ||
size_t | length, | ||
unsigned char | iv[16], | ||
const unsigned char * | input, | ||
unsigned char * | output | ||
) |
AES-CBC buffer encryption/decryption Length should be a multiple of the block size (16 bytes)
- Note:
- Upon exit, the content of the IV is updated so that you can call the function same function again on the following block(s) of data and get the same result as if it was encrypted in one call. This allows a "streaming" usage. If on the other hand you need to retain the contents of the IV, you should either save it manually or use the cipher module instead.
- Parameters:
-
ctx AES context mode MBEDTLS_AES_ENCRYPT or MBEDTLS_AES_DECRYPT length length of the input data iv initialization vector (updated after use) input buffer holding the input data output buffer holding the output data
- Returns:
- 0 if successful, or MBEDTLS_ERR_AES_INVALID_INPUT_LENGTH
int mbedtls_aes_crypt_cfb128 | ( | mbedtls_aes_context * | ctx, |
int | mode, | ||
size_t | length, | ||
size_t * | iv_off, | ||
unsigned char | iv[16], | ||
const unsigned char * | input, | ||
unsigned char * | output | ||
) |
AES-CFB128 buffer encryption/decryption.
Note: Due to the nature of CFB you should use the same key schedule for both encryption and decryption. So a context initialized with mbedtls_aes_setkey_enc() for both MBEDTLS_AES_ENCRYPT and MBEDTLS_AES_DECRYPT.
- Note:
- Upon exit, the content of the IV is updated so that you can call the function same function again on the following block(s) of data and get the same result as if it was encrypted in one call. This allows a "streaming" usage. If on the other hand you need to retain the contents of the IV, you should either save it manually or use the cipher module instead.
- Parameters:
-
ctx AES context mode MBEDTLS_AES_ENCRYPT or MBEDTLS_AES_DECRYPT length length of the input data iv_off offset in IV (updated after use) iv initialization vector (updated after use) input buffer holding the input data output buffer holding the output data
- Returns:
- 0 if successful
int mbedtls_aes_crypt_cfb8 | ( | mbedtls_aes_context * | ctx, |
int | mode, | ||
size_t | length, | ||
unsigned char | iv[16], | ||
const unsigned char * | input, | ||
unsigned char * | output | ||
) |
AES-CFB8 buffer encryption/decryption.
Note: Due to the nature of CFB you should use the same key schedule for both encryption and decryption. So a context initialized with mbedtls_aes_setkey_enc() for both MBEDTLS_AES_ENCRYPT and MBEDTLS_AES_DECRYPT.
- Note:
- Upon exit, the content of the IV is updated so that you can call the function same function again on the following block(s) of data and get the same result as if it was encrypted in one call. This allows a "streaming" usage. If on the other hand you need to retain the contents of the IV, you should either save it manually or use the cipher module instead.
- Parameters:
-
ctx AES context mode MBEDTLS_AES_ENCRYPT or MBEDTLS_AES_DECRYPT length length of the input data iv initialization vector (updated after use) input buffer holding the input data output buffer holding the output data
- Returns:
- 0 if successful
int mbedtls_aes_crypt_ctr | ( | mbedtls_aes_context * | ctx, |
size_t | length, | ||
size_t * | nc_off, | ||
unsigned char | nonce_counter[16], | ||
unsigned char | stream_block[16], | ||
const unsigned char * | input, | ||
unsigned char * | output | ||
) |
AES-CTR buffer encryption/decryption.
Warning: You have to keep the maximum use of your counter in mind!
Note: Due to the nature of CTR you should use the same key schedule for both encryption and decryption. So a context initialized with mbedtls_aes_setkey_enc() for both MBEDTLS_AES_ENCRYPT and MBEDTLS_AES_DECRYPT.
- Parameters:
-
ctx AES context length The length of the data nc_off The offset in the current stream_block (for resuming within current cipher stream). The offset pointer to should be 0 at the start of a stream. nonce_counter The 128-bit nonce and counter. stream_block The saved stream-block for resuming. Is overwritten by the function. input The input data stream output The output data stream
- Returns:
- 0 if successful
int mbedtls_aes_crypt_ecb | ( | mbedtls_aes_context * | ctx, |
int | mode, | ||
const unsigned char | input[16], | ||
unsigned char | output[16] | ||
) |
void mbedtls_aes_decrypt | ( | mbedtls_aes_context * | ctx, |
const unsigned char | input[16], | ||
unsigned char | output[16] | ||
) |
void mbedtls_aes_encrypt | ( | mbedtls_aes_context * | ctx, |
const unsigned char | input[16], | ||
unsigned char | output[16] | ||
) |
void mbedtls_aes_free | ( | mbedtls_aes_context * | ctx ) |
void mbedtls_aes_init | ( | mbedtls_aes_context * | ctx ) |
int mbedtls_aes_self_test | ( | int | verbose ) |
int mbedtls_aes_setkey_dec | ( | mbedtls_aes_context * | ctx, |
const unsigned char * | key, | ||
unsigned int | keybits | ||
) |
int mbedtls_aes_setkey_enc | ( | mbedtls_aes_context * | ctx, |
const unsigned char * | key, | ||
unsigned int | keybits | ||
) |
Generated on Tue Jul 12 2022 12:52:50 by
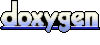