mbedtls ported to mbed-classic
Fork of mbedtls by
bignum.h File Reference
Multi-precision integer library. More...
Go to the source code of this file.
Data Structures | |
struct | mbedtls_mpi |
MPI structure. More... | |
Functions | |
void | mbedtls_mpi_init (mbedtls_mpi *X) |
Initialize one MPI (make internal references valid) This just makes it ready to be set or freed, but does not define a value for the MPI. | |
void | mbedtls_mpi_free (mbedtls_mpi *X) |
Unallocate one MPI. | |
int | mbedtls_mpi_grow (mbedtls_mpi *X, size_t nblimbs) |
Enlarge to the specified number of limbs. | |
int | mbedtls_mpi_shrink (mbedtls_mpi *X, size_t nblimbs) |
Resize down, keeping at least the specified number of limbs. | |
int | mbedtls_mpi_copy (mbedtls_mpi *X, const mbedtls_mpi *Y) |
Copy the contents of Y into X. | |
void | mbedtls_mpi_swap (mbedtls_mpi *X, mbedtls_mpi *Y) |
Swap the contents of X and Y. | |
int | mbedtls_mpi_safe_cond_assign (mbedtls_mpi *X, const mbedtls_mpi *Y, unsigned char assign) |
Safe conditional assignement X = Y if assign is 1. | |
int | mbedtls_mpi_safe_cond_swap (mbedtls_mpi *X, mbedtls_mpi *Y, unsigned char assign) |
Safe conditional swap X <-> Y if swap is 1. | |
int | mbedtls_mpi_lset (mbedtls_mpi *X, mbedtls_mpi_sint z) |
Set value from integer. | |
int | mbedtls_mpi_get_bit (const mbedtls_mpi *X, size_t pos) |
Get a specific bit from X. | |
int | mbedtls_mpi_set_bit (mbedtls_mpi *X, size_t pos, unsigned char val) |
Set a bit of X to a specific value of 0 or 1. | |
size_t | mbedtls_mpi_lsb (const mbedtls_mpi *X) |
Return the number of zero-bits before the least significant '1' bit. | |
size_t | mbedtls_mpi_bitlen (const mbedtls_mpi *X) |
Return the number of bits up to and including the most significant '1' bit'. | |
size_t | mbedtls_mpi_size (const mbedtls_mpi *X) |
Return the total size in bytes. | |
int | mbedtls_mpi_read_string (mbedtls_mpi *X, int radix, const char *s) |
Import from an ASCII string. | |
int | mbedtls_mpi_write_string (const mbedtls_mpi *X, int radix, char *buf, size_t buflen, size_t *olen) |
Export into an ASCII string. | |
int | mbedtls_mpi_read_file (mbedtls_mpi *X, int radix, FILE *fin) |
Read X from an opened file. | |
int | mbedtls_mpi_write_file (const char *p, const mbedtls_mpi *X, int radix, FILE *fout) |
Write X into an opened file, or stdout if fout is NULL. | |
int | mbedtls_mpi_read_binary (mbedtls_mpi *X, const unsigned char *buf, size_t buflen) |
Import X from unsigned binary data, big endian. | |
int | mbedtls_mpi_write_binary (const mbedtls_mpi *X, unsigned char *buf, size_t buflen) |
Export X into unsigned binary data, big endian. | |
int | mbedtls_mpi_shift_l (mbedtls_mpi *X, size_t count) |
Left-shift: X <<= count. | |
int | mbedtls_mpi_shift_r (mbedtls_mpi *X, size_t count) |
Right-shift: X >>= count. | |
int | mbedtls_mpi_cmp_abs (const mbedtls_mpi *X, const mbedtls_mpi *Y) |
Compare unsigned values. | |
int | mbedtls_mpi_cmp_mpi (const mbedtls_mpi *X, const mbedtls_mpi *Y) |
Compare signed values. | |
int | mbedtls_mpi_cmp_int (const mbedtls_mpi *X, mbedtls_mpi_sint z) |
Compare signed values. | |
int | mbedtls_mpi_add_abs (mbedtls_mpi *X, const mbedtls_mpi *A, const mbedtls_mpi *B) |
Unsigned addition: X = |A| + |B|. | |
int | mbedtls_mpi_sub_abs (mbedtls_mpi *X, const mbedtls_mpi *A, const mbedtls_mpi *B) |
Unsigned subtraction: X = |A| - |B|. | |
int | mbedtls_mpi_add_mpi (mbedtls_mpi *X, const mbedtls_mpi *A, const mbedtls_mpi *B) |
Signed addition: X = A + B. | |
int | mbedtls_mpi_sub_mpi (mbedtls_mpi *X, const mbedtls_mpi *A, const mbedtls_mpi *B) |
Signed subtraction: X = A - B. | |
int | mbedtls_mpi_add_int (mbedtls_mpi *X, const mbedtls_mpi *A, mbedtls_mpi_sint b) |
Signed addition: X = A + b. | |
int | mbedtls_mpi_sub_int (mbedtls_mpi *X, const mbedtls_mpi *A, mbedtls_mpi_sint b) |
Signed subtraction: X = A - b. | |
int | mbedtls_mpi_mul_mpi (mbedtls_mpi *X, const mbedtls_mpi *A, const mbedtls_mpi *B) |
Baseline multiplication: X = A * B. | |
int | mbedtls_mpi_mul_int (mbedtls_mpi *X, const mbedtls_mpi *A, mbedtls_mpi_uint b) |
Baseline multiplication: X = A * b. | |
int | mbedtls_mpi_div_mpi (mbedtls_mpi *Q, mbedtls_mpi *R, const mbedtls_mpi *A, const mbedtls_mpi *B) |
Division by mbedtls_mpi: A = Q * B + R. | |
int | mbedtls_mpi_div_int (mbedtls_mpi *Q, mbedtls_mpi *R, const mbedtls_mpi *A, mbedtls_mpi_sint b) |
Division by int: A = Q * b + R. | |
int | mbedtls_mpi_mod_mpi (mbedtls_mpi *R, const mbedtls_mpi *A, const mbedtls_mpi *B) |
Modulo: R = A mod B. | |
int | mbedtls_mpi_mod_int (mbedtls_mpi_uint *r, const mbedtls_mpi *A, mbedtls_mpi_sint b) |
Modulo: r = A mod b. | |
int | mbedtls_mpi_exp_mod (mbedtls_mpi *X, const mbedtls_mpi *A, const mbedtls_mpi *E, const mbedtls_mpi *N, mbedtls_mpi *_RR) |
Sliding-window exponentiation: X = A^E mod N. | |
int | mbedtls_mpi_fill_random (mbedtls_mpi *X, size_t size, int(*f_rng)(void *, unsigned char *, size_t), void *p_rng) |
Fill an MPI X with size bytes of random. | |
int | mbedtls_mpi_gcd (mbedtls_mpi *G, const mbedtls_mpi *A, const mbedtls_mpi *B) |
Greatest common divisor: G = gcd(A, B) | |
int | mbedtls_mpi_inv_mod (mbedtls_mpi *X, const mbedtls_mpi *A, const mbedtls_mpi *N) |
Modular inverse: X = A^-1 mod N. | |
int | mbedtls_mpi_is_prime (const mbedtls_mpi *X, int(*f_rng)(void *, unsigned char *, size_t), void *p_rng) |
Miller-Rabin primality test. | |
int | mbedtls_mpi_gen_prime (mbedtls_mpi *X, size_t nbits, int dh_flag, int(*f_rng)(void *, unsigned char *, size_t), void *p_rng) |
Prime number generation. | |
int | mbedtls_mpi_self_test (int verbose) |
Checkup routine. |
Detailed Description
Multi-precision integer library.
Copyright (C) 2006-2015, ARM Limited, All Rights Reserved SPDX-License-Identifier: Apache-2.0
Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance with the License. You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software distributed under the License is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions and limitations under the License.
This file is part of mbed TLS (https://tls.mbed.org)
Definition in file bignum.h.
Function Documentation
int mbedtls_mpi_add_abs | ( | mbedtls_mpi * | X, |
const mbedtls_mpi * | A, | ||
const mbedtls_mpi * | B | ||
) |
int mbedtls_mpi_add_int | ( | mbedtls_mpi * | X, |
const mbedtls_mpi * | A, | ||
mbedtls_mpi_sint | b | ||
) |
int mbedtls_mpi_add_mpi | ( | mbedtls_mpi * | X, |
const mbedtls_mpi * | A, | ||
const mbedtls_mpi * | B | ||
) |
size_t mbedtls_mpi_bitlen | ( | const mbedtls_mpi * | X ) |
int mbedtls_mpi_cmp_abs | ( | const mbedtls_mpi * | X, |
const mbedtls_mpi * | Y | ||
) |
int mbedtls_mpi_cmp_int | ( | const mbedtls_mpi * | X, |
mbedtls_mpi_sint | z | ||
) |
int mbedtls_mpi_cmp_mpi | ( | const mbedtls_mpi * | X, |
const mbedtls_mpi * | Y | ||
) |
int mbedtls_mpi_copy | ( | mbedtls_mpi * | X, |
const mbedtls_mpi * | Y | ||
) |
int mbedtls_mpi_div_int | ( | mbedtls_mpi * | Q, |
mbedtls_mpi * | R, | ||
const mbedtls_mpi * | A, | ||
mbedtls_mpi_sint | b | ||
) |
Division by int: A = Q * b + R.
- Parameters:
-
Q Destination MPI for the quotient R Destination MPI for the rest value A Left-hand MPI b Integer to divide by
- Returns:
- 0 if successful, MBEDTLS_ERR_MPI_ALLOC_FAILED if memory allocation failed, MBEDTLS_ERR_MPI_DIVISION_BY_ZERO if b == 0
- Note:
- Either Q or R can be NULL.
int mbedtls_mpi_div_mpi | ( | mbedtls_mpi * | Q, |
mbedtls_mpi * | R, | ||
const mbedtls_mpi * | A, | ||
const mbedtls_mpi * | B | ||
) |
Division by mbedtls_mpi: A = Q * B + R.
- Parameters:
-
Q Destination MPI for the quotient R Destination MPI for the rest value A Left-hand MPI B Right-hand MPI
- Returns:
- 0 if successful, MBEDTLS_ERR_MPI_ALLOC_FAILED if memory allocation failed, MBEDTLS_ERR_MPI_DIVISION_BY_ZERO if B == 0
- Note:
- Either Q or R can be NULL.
int mbedtls_mpi_exp_mod | ( | mbedtls_mpi * | X, |
const mbedtls_mpi * | A, | ||
const mbedtls_mpi * | E, | ||
const mbedtls_mpi * | N, | ||
mbedtls_mpi * | _RR | ||
) |
Sliding-window exponentiation: X = A^E mod N.
- Parameters:
-
X Destination MPI A Left-hand MPI E Exponent MPI N Modular MPI _RR Speed-up MPI used for recalculations
- Returns:
- 0 if successful, MBEDTLS_ERR_MPI_ALLOC_FAILED if memory allocation failed, MBEDTLS_ERR_MPI_BAD_INPUT_DATA if N is negative or even or if E is negative
- Note:
- _RR is used to avoid re-computing R*R mod N across multiple calls, which speeds up things a bit. It can be set to NULL if the extra performance is unneeded.
int mbedtls_mpi_fill_random | ( | mbedtls_mpi * | X, |
size_t | size, | ||
int(*)(void *, unsigned char *, size_t) | f_rng, | ||
void * | p_rng | ||
) |
void mbedtls_mpi_free | ( | mbedtls_mpi * | X ) |
int mbedtls_mpi_gcd | ( | mbedtls_mpi * | G, |
const mbedtls_mpi * | A, | ||
const mbedtls_mpi * | B | ||
) |
int mbedtls_mpi_gen_prime | ( | mbedtls_mpi * | X, |
size_t | nbits, | ||
int | dh_flag, | ||
int(*)(void *, unsigned char *, size_t) | f_rng, | ||
void * | p_rng | ||
) |
Prime number generation.
- Parameters:
-
X Destination MPI nbits Required size of X in bits ( 3 <= nbits <= MBEDTLS_MPI_MAX_BITS ) dh_flag If 1, then (X-1)/2 will be prime too f_rng RNG function p_rng RNG parameter
- Returns:
- 0 if successful (probably prime), MBEDTLS_ERR_MPI_ALLOC_FAILED if memory allocation failed, MBEDTLS_ERR_MPI_BAD_INPUT_DATA if nbits is < 3
int mbedtls_mpi_get_bit | ( | const mbedtls_mpi * | X, |
size_t | pos | ||
) |
int mbedtls_mpi_grow | ( | mbedtls_mpi * | X, |
size_t | nblimbs | ||
) |
void mbedtls_mpi_init | ( | mbedtls_mpi * | X ) |
int mbedtls_mpi_inv_mod | ( | mbedtls_mpi * | X, |
const mbedtls_mpi * | A, | ||
const mbedtls_mpi * | N | ||
) |
Modular inverse: X = A^-1 mod N.
- Parameters:
-
X Destination MPI A Left-hand MPI N Right-hand MPI
- Returns:
- 0 if successful, MBEDTLS_ERR_MPI_ALLOC_FAILED if memory allocation failed, MBEDTLS_ERR_MPI_BAD_INPUT_DATA if N is negative or nil MBEDTLS_ERR_MPI_NOT_ACCEPTABLE if A has no inverse mod N
int mbedtls_mpi_is_prime | ( | const mbedtls_mpi * | X, |
int(*)(void *, unsigned char *, size_t) | f_rng, | ||
void * | p_rng | ||
) |
size_t mbedtls_mpi_lsb | ( | const mbedtls_mpi * | X ) |
int mbedtls_mpi_lset | ( | mbedtls_mpi * | X, |
mbedtls_mpi_sint | z | ||
) |
int mbedtls_mpi_mod_int | ( | mbedtls_mpi_uint * | r, |
const mbedtls_mpi * | A, | ||
mbedtls_mpi_sint | b | ||
) |
Modulo: r = A mod b.
- Parameters:
-
r Destination mbedtls_mpi_uint A Left-hand MPI b Integer to divide by
- Returns:
- 0 if successful, MBEDTLS_ERR_MPI_ALLOC_FAILED if memory allocation failed, MBEDTLS_ERR_MPI_DIVISION_BY_ZERO if b == 0, MBEDTLS_ERR_MPI_NEGATIVE_VALUE if b < 0
int mbedtls_mpi_mod_mpi | ( | mbedtls_mpi * | R, |
const mbedtls_mpi * | A, | ||
const mbedtls_mpi * | B | ||
) |
Modulo: R = A mod B.
- Parameters:
-
R Destination MPI for the rest value A Left-hand MPI B Right-hand MPI
- Returns:
- 0 if successful, MBEDTLS_ERR_MPI_ALLOC_FAILED if memory allocation failed, MBEDTLS_ERR_MPI_DIVISION_BY_ZERO if B == 0, MBEDTLS_ERR_MPI_NEGATIVE_VALUE if B < 0
int mbedtls_mpi_mul_int | ( | mbedtls_mpi * | X, |
const mbedtls_mpi * | A, | ||
mbedtls_mpi_uint | b | ||
) |
int mbedtls_mpi_mul_mpi | ( | mbedtls_mpi * | X, |
const mbedtls_mpi * | A, | ||
const mbedtls_mpi * | B | ||
) |
int mbedtls_mpi_read_binary | ( | mbedtls_mpi * | X, |
const unsigned char * | buf, | ||
size_t | buflen | ||
) |
int mbedtls_mpi_read_file | ( | mbedtls_mpi * | X, |
int | radix, | ||
FILE * | fin | ||
) |
int mbedtls_mpi_read_string | ( | mbedtls_mpi * | X, |
int | radix, | ||
const char * | s | ||
) |
int mbedtls_mpi_safe_cond_assign | ( | mbedtls_mpi * | X, |
const mbedtls_mpi * | Y, | ||
unsigned char | assign | ||
) |
Safe conditional assignement X = Y if assign is 1.
- Parameters:
-
X MPI to conditionally assign to Y Value to be assigned assign 1: perform the assignment, 0: keep X's original value
- Returns:
- 0 if successful, MBEDTLS_ERR_MPI_ALLOC_FAILED if memory allocation failed,
- Note:
- This function is equivalent to if( assign ) mbedtls_mpi_copy( X, Y ); except that it avoids leaking any information about whether the assignment was done or not (the above code may leak information through branch prediction and/or memory access patterns analysis).
int mbedtls_mpi_safe_cond_swap | ( | mbedtls_mpi * | X, |
mbedtls_mpi * | Y, | ||
unsigned char | assign | ||
) |
Safe conditional swap X <-> Y if swap is 1.
- Parameters:
-
X First mbedtls_mpi value Y Second mbedtls_mpi value assign 1: perform the swap, 0: keep X and Y's original values
- Returns:
- 0 if successful, MBEDTLS_ERR_MPI_ALLOC_FAILED if memory allocation failed,
- Note:
- This function is equivalent to if( assign ) mbedtls_mpi_swap( X, Y ); except that it avoids leaking any information about whether the assignment was done or not (the above code may leak information through branch prediction and/or memory access patterns analysis).
int mbedtls_mpi_self_test | ( | int | verbose ) |
int mbedtls_mpi_set_bit | ( | mbedtls_mpi * | X, |
size_t | pos, | ||
unsigned char | val | ||
) |
Set a bit of X to a specific value of 0 or 1.
- Note:
- Will grow X if necessary to set a bit to 1 in a not yet existing limb. Will not grow if bit should be set to 0
- Parameters:
-
X MPI to use pos Zero-based index of the bit in X val The value to set the bit to (0 or 1)
- Returns:
- 0 if successful, MBEDTLS_ERR_MPI_ALLOC_FAILED if memory allocation failed, MBEDTLS_ERR_MPI_BAD_INPUT_DATA if val is not 0 or 1
int mbedtls_mpi_shift_l | ( | mbedtls_mpi * | X, |
size_t | count | ||
) |
int mbedtls_mpi_shift_r | ( | mbedtls_mpi * | X, |
size_t | count | ||
) |
int mbedtls_mpi_shrink | ( | mbedtls_mpi * | X, |
size_t | nblimbs | ||
) |
size_t mbedtls_mpi_size | ( | const mbedtls_mpi * | X ) |
int mbedtls_mpi_sub_abs | ( | mbedtls_mpi * | X, |
const mbedtls_mpi * | A, | ||
const mbedtls_mpi * | B | ||
) |
int mbedtls_mpi_sub_int | ( | mbedtls_mpi * | X, |
const mbedtls_mpi * | A, | ||
mbedtls_mpi_sint | b | ||
) |
int mbedtls_mpi_sub_mpi | ( | mbedtls_mpi * | X, |
const mbedtls_mpi * | A, | ||
const mbedtls_mpi * | B | ||
) |
void mbedtls_mpi_swap | ( | mbedtls_mpi * | X, |
mbedtls_mpi * | Y | ||
) |
int mbedtls_mpi_write_binary | ( | const mbedtls_mpi * | X, |
unsigned char * | buf, | ||
size_t | buflen | ||
) |
Export X into unsigned binary data, big endian.
Always fills the whole buffer, which will start with zeros if the number is smaller.
- Parameters:
-
X Source MPI buf Output buffer buflen Output buffer size
- Returns:
- 0 if successful, MBEDTLS_ERR_MPI_BUFFER_TOO_SMALL if buf isn't large enough
int mbedtls_mpi_write_file | ( | const char * | p, |
const mbedtls_mpi * | X, | ||
int | radix, | ||
FILE * | fout | ||
) |
Write X into an opened file, or stdout if fout is NULL.
- Parameters:
-
p Prefix, can be NULL X Source MPI radix Output numeric base fout Output file handle (can be NULL)
- Returns:
- 0 if successful, or a MBEDTLS_ERR_MPI_XXX error code
- Note:
- Set fout == NULL to print X on the console.
int mbedtls_mpi_write_string | ( | const mbedtls_mpi * | X, |
int | radix, | ||
char * | buf, | ||
size_t | buflen, | ||
size_t * | olen | ||
) |
Export into an ASCII string.
- Parameters:
-
X Source MPI radix Output numeric base buf Buffer to write the string to buflen Length of buf olen Length of the string written, including final NUL byte
- Returns:
- 0 if successful, or a MBEDTLS_ERR_MPI_XXX error code. *olen is always updated to reflect the amount of data that has (or would have) been written.
- Note:
- Call this function with buflen = 0 to obtain the minimum required buffer size in *olen.
Generated on Tue Jul 12 2022 12:52:51 by
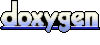