mbedtls ported to mbed-classic
Fork of mbedtls by
ecp.h File Reference
Elliptic curves over GF(p) More...
Go to the source code of this file.
Data Structures | |
struct | mbedtls_ecp_curve_info |
Curve information for use by other modules. More... | |
struct | mbedtls_ecp_point |
ECP point structure (jacobian coordinates) More... | |
struct | mbedtls_ecp_group |
ECP group structure. More... | |
struct | mbedtls_ecp_keypair |
ECP key pair structure. More... | |
Enumerations | |
enum | mbedtls_ecp_group_id { , MBEDTLS_ECP_DP_SECP192R1, MBEDTLS_ECP_DP_SECP224R1, MBEDTLS_ECP_DP_SECP256R1, MBEDTLS_ECP_DP_SECP384R1, MBEDTLS_ECP_DP_SECP521R1, MBEDTLS_ECP_DP_BP256R1, MBEDTLS_ECP_DP_BP384R1, MBEDTLS_ECP_DP_BP512R1, MBEDTLS_ECP_DP_CURVE25519, MBEDTLS_ECP_DP_SECP192K1, MBEDTLS_ECP_DP_SECP224K1, MBEDTLS_ECP_DP_SECP256K1 } |
Domain parameters (curve, subgroup and generator) identifiers. More... | |
Functions | |
const mbedtls_ecp_curve_info * | mbedtls_ecp_curve_list (void) |
Get the list of supported curves in order of preferrence (full information) | |
const mbedtls_ecp_group_id * | mbedtls_ecp_grp_id_list (void) |
Get the list of supported curves in order of preferrence (grp_id only) | |
const mbedtls_ecp_curve_info * | mbedtls_ecp_curve_info_from_grp_id (mbedtls_ecp_group_id grp_id) |
Get curve information from an internal group identifier. | |
const mbedtls_ecp_curve_info * | mbedtls_ecp_curve_info_from_tls_id (uint16_t tls_id) |
Get curve information from a TLS NamedCurve value. | |
const mbedtls_ecp_curve_info * | mbedtls_ecp_curve_info_from_name (const char *name) |
Get curve information from a human-readable name. | |
void | mbedtls_ecp_point_init (mbedtls_ecp_point *pt) |
Initialize a point (as zero) | |
void | mbedtls_ecp_group_init (mbedtls_ecp_group *grp) |
Initialize a group (to something meaningless) | |
void | mbedtls_ecp_keypair_init (mbedtls_ecp_keypair *key) |
Initialize a key pair (as an invalid one) | |
void | mbedtls_ecp_point_free (mbedtls_ecp_point *pt) |
Free the components of a point. | |
void | mbedtls_ecp_group_free (mbedtls_ecp_group *grp) |
Free the components of an ECP group. | |
void | mbedtls_ecp_keypair_free (mbedtls_ecp_keypair *key) |
Free the components of a key pair. | |
int | mbedtls_ecp_copy (mbedtls_ecp_point *P, const mbedtls_ecp_point *Q) |
Copy the contents of point Q into P. | |
int | mbedtls_ecp_group_copy (mbedtls_ecp_group *dst, const mbedtls_ecp_group *src) |
Copy the contents of a group object. | |
int | mbedtls_ecp_set_zero (mbedtls_ecp_point *pt) |
Set a point to zero. | |
int | mbedtls_ecp_is_zero (mbedtls_ecp_point *pt) |
Tell if a point is zero. | |
int | mbedtls_ecp_point_cmp (const mbedtls_ecp_point *P, const mbedtls_ecp_point *Q) |
Compare two points. | |
int | mbedtls_ecp_point_read_string (mbedtls_ecp_point *P, int radix, const char *x, const char *y) |
Import a non-zero point from two ASCII strings. | |
int | mbedtls_ecp_point_write_binary (const mbedtls_ecp_group *grp, const mbedtls_ecp_point *P, int format, size_t *olen, unsigned char *buf, size_t buflen) |
Export a point into unsigned binary data. | |
int | mbedtls_ecp_point_read_binary (const mbedtls_ecp_group *grp, mbedtls_ecp_point *P, const unsigned char *buf, size_t ilen) |
Import a point from unsigned binary data. | |
int | mbedtls_ecp_tls_read_point (const mbedtls_ecp_group *grp, mbedtls_ecp_point *pt, const unsigned char **buf, size_t len) |
Import a point from a TLS ECPoint record. | |
int | mbedtls_ecp_tls_write_point (const mbedtls_ecp_group *grp, const mbedtls_ecp_point *pt, int format, size_t *olen, unsigned char *buf, size_t blen) |
Export a point as a TLS ECPoint record. | |
int | mbedtls_ecp_group_load (mbedtls_ecp_group *grp, mbedtls_ecp_group_id index) |
Set a group using well-known domain parameters. | |
int | mbedtls_ecp_tls_read_group (mbedtls_ecp_group *grp, const unsigned char **buf, size_t len) |
Set a group from a TLS ECParameters record. | |
int | mbedtls_ecp_tls_write_group (const mbedtls_ecp_group *grp, size_t *olen, unsigned char *buf, size_t blen) |
Write the TLS ECParameters record for a group. | |
int | mbedtls_ecp_mul (mbedtls_ecp_group *grp, mbedtls_ecp_point *R, const mbedtls_mpi *m, const mbedtls_ecp_point *P, int(*f_rng)(void *, unsigned char *, size_t), void *p_rng) |
Multiplication by an integer: R = m * P (Not thread-safe to use same group in multiple threads) | |
int | mbedtls_ecp_muladd (mbedtls_ecp_group *grp, mbedtls_ecp_point *R, const mbedtls_mpi *m, const mbedtls_ecp_point *P, const mbedtls_mpi *n, const mbedtls_ecp_point *Q) |
Multiplication and addition of two points by integers: R = m * P + n * Q (Not thread-safe to use same group in multiple threads) | |
int | mbedtls_ecp_check_pubkey (const mbedtls_ecp_group *grp, const mbedtls_ecp_point *pt) |
Check that a point is a valid public key on this curve. | |
int | mbedtls_ecp_check_privkey (const mbedtls_ecp_group *grp, const mbedtls_mpi *d) |
Check that an mbedtls_mpi is a valid private key for this curve. | |
int | mbedtls_ecp_gen_keypair_base (mbedtls_ecp_group *grp, const mbedtls_ecp_point *G, mbedtls_mpi *d, mbedtls_ecp_point *Q, int(*f_rng)(void *, unsigned char *, size_t), void *p_rng) |
Generate a keypair with configurable base point. | |
int | mbedtls_ecp_gen_keypair (mbedtls_ecp_group *grp, mbedtls_mpi *d, mbedtls_ecp_point *Q, int(*f_rng)(void *, unsigned char *, size_t), void *p_rng) |
Generate a keypair. | |
int | mbedtls_ecp_gen_key (mbedtls_ecp_group_id grp_id, mbedtls_ecp_keypair *key, int(*f_rng)(void *, unsigned char *, size_t), void *p_rng) |
Generate a keypair. | |
int | mbedtls_ecp_check_pub_priv (const mbedtls_ecp_keypair *pub, const mbedtls_ecp_keypair *prv) |
Check a public-private key pair. | |
int | mbedtls_ecp_self_test (int verbose) |
Checkup routine. |
Detailed Description
Elliptic curves over GF(p)
Copyright (C) 2006-2015, ARM Limited, All Rights Reserved SPDX-License-Identifier: Apache-2.0
Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance with the License. You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software distributed under the License is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions and limitations under the License.
This file is part of mbed TLS (https://tls.mbed.org)
Definition in file ecp.h.
Enumeration Type Documentation
enum mbedtls_ecp_group_id |
Domain parameters (curve, subgroup and generator) identifiers.
Only curves over prime fields are supported.
- Warning:
- This library does not support validation of arbitrary domain parameters. Therefore, only well-known domain parameters from trusted sources should be used. See mbedtls_ecp_group_load().
- Enumerator:
Function Documentation
int mbedtls_ecp_check_privkey | ( | const mbedtls_ecp_group * | grp, |
const mbedtls_mpi * | d | ||
) |
Check that an mbedtls_mpi is a valid private key for this curve.
- Parameters:
-
grp Group used d Integer to check
- Returns:
- 0 if point is a valid private key, MBEDTLS_ERR_ECP_INVALID_KEY otherwise.
- Note:
- Uses bare components rather than an mbedtls_ecp_keypair structure in order to ease use with other structures such as mbedtls_ecdh_context of mbedtls_ecdsa_context.
int mbedtls_ecp_check_pub_priv | ( | const mbedtls_ecp_keypair * | pub, |
const mbedtls_ecp_keypair * | prv | ||
) |
Check a public-private key pair.
- Parameters:
-
pub Keypair structure holding a public key prv Keypair structure holding a private (plus public) key
- Returns:
- 0 if successful (keys are valid and match), or MBEDTLS_ERR_ECP_BAD_INPUT_DATA, or a MBEDTLS_ERR_ECP_XXX or MBEDTLS_ERR_MPI_XXX code.
int mbedtls_ecp_check_pubkey | ( | const mbedtls_ecp_group * | grp, |
const mbedtls_ecp_point * | pt | ||
) |
Check that a point is a valid public key on this curve.
- Parameters:
-
grp Curve/group the point should belong to pt Point to check
- Returns:
- 0 if point is a valid public key, MBEDTLS_ERR_ECP_INVALID_KEY otherwise.
- Note:
- This function only checks the point is non-zero, has valid coordinates and lies on the curve, but not that it is indeed a multiple of G. This is additional check is more expensive, isn't required by standards, and shouldn't be necessary if the group used has a small cofactor. In particular, it is useless for the NIST groups which all have a cofactor of 1.
- Uses bare components rather than an mbedtls_ecp_keypair structure in order to ease use with other structures such as mbedtls_ecdh_context of mbedtls_ecdsa_context.
int mbedtls_ecp_copy | ( | mbedtls_ecp_point * | P, |
const mbedtls_ecp_point * | Q | ||
) |
const mbedtls_ecp_curve_info* mbedtls_ecp_curve_info_from_grp_id | ( | mbedtls_ecp_group_id | grp_id ) |
const mbedtls_ecp_curve_info* mbedtls_ecp_curve_info_from_name | ( | const char * | name ) |
const mbedtls_ecp_curve_info* mbedtls_ecp_curve_info_from_tls_id | ( | uint16_t | tls_id ) |
const mbedtls_ecp_curve_info* mbedtls_ecp_curve_list | ( | void | ) |
int mbedtls_ecp_gen_key | ( | mbedtls_ecp_group_id | grp_id, |
mbedtls_ecp_keypair * | key, | ||
int(*)(void *, unsigned char *, size_t) | f_rng, | ||
void * | p_rng | ||
) |
int mbedtls_ecp_gen_keypair | ( | mbedtls_ecp_group * | grp, |
mbedtls_mpi * | d, | ||
mbedtls_ecp_point * | Q, | ||
int(*)(void *, unsigned char *, size_t) | f_rng, | ||
void * | p_rng | ||
) |
Generate a keypair.
- Parameters:
-
grp ECP group d Destination MPI (secret part) Q Destination point (public part) f_rng RNG function p_rng RNG parameter
- Returns:
- 0 if successful, or a MBEDTLS_ERR_ECP_XXX or MBEDTLS_MPI_XXX error code
- Note:
- Uses bare components rather than an mbedtls_ecp_keypair structure in order to ease use with other structures such as mbedtls_ecdh_context of mbedtls_ecdsa_context.
int mbedtls_ecp_gen_keypair_base | ( | mbedtls_ecp_group * | grp, |
const mbedtls_ecp_point * | G, | ||
mbedtls_mpi * | d, | ||
mbedtls_ecp_point * | Q, | ||
int(*)(void *, unsigned char *, size_t) | f_rng, | ||
void * | p_rng | ||
) |
Generate a keypair with configurable base point.
- Parameters:
-
grp ECP group G Chosen base point d Destination MPI (secret part) Q Destination point (public part) f_rng RNG function p_rng RNG parameter
- Returns:
- 0 if successful, or a MBEDTLS_ERR_ECP_XXX or MBEDTLS_MPI_XXX error code
- Note:
- Uses bare components rather than an mbedtls_ecp_keypair structure in order to ease use with other structures such as mbedtls_ecdh_context of mbedtls_ecdsa_context.
int mbedtls_ecp_group_copy | ( | mbedtls_ecp_group * | dst, |
const mbedtls_ecp_group * | src | ||
) |
void mbedtls_ecp_group_free | ( | mbedtls_ecp_group * | grp ) |
void mbedtls_ecp_group_init | ( | mbedtls_ecp_group * | grp ) |
int mbedtls_ecp_group_load | ( | mbedtls_ecp_group * | grp, |
mbedtls_ecp_group_id | index | ||
) |
Set a group using well-known domain parameters.
- Parameters:
-
grp Destination group index Index in the list of well-known domain parameters
- Returns:
- 0 if successful, MBEDTLS_ERR_MPI_XXX if initialization failed MBEDTLS_ERR_ECP_FEATURE_UNAVAILABLE for unkownn groups
- Note:
- Index should be a value of RFC 4492's enum NamedCurve, usually in the form of a MBEDTLS_ECP_DP_XXX macro.
Definition at line 691 of file ecp_curves.c.
const mbedtls_ecp_group_id* mbedtls_ecp_grp_id_list | ( | void | ) |
int mbedtls_ecp_is_zero | ( | mbedtls_ecp_point * | pt ) |
void mbedtls_ecp_keypair_free | ( | mbedtls_ecp_keypair * | key ) |
void mbedtls_ecp_keypair_init | ( | mbedtls_ecp_keypair * | key ) |
int mbedtls_ecp_mul | ( | mbedtls_ecp_group * | grp, |
mbedtls_ecp_point * | R, | ||
const mbedtls_mpi * | m, | ||
const mbedtls_ecp_point * | P, | ||
int(*)(void *, unsigned char *, size_t) | f_rng, | ||
void * | p_rng | ||
) |
Multiplication by an integer: R = m * P (Not thread-safe to use same group in multiple threads)
- Note:
- In order to prevent timing attacks, this function executes the exact same sequence of (base field) operations for any valid m. It avoids any if-branch or array index depending on the value of m.
- If f_rng is not NULL, it is used to randomize intermediate results in order to prevent potential timing attacks targeting these results. It is recommended to always provide a non-NULL f_rng (the overhead is negligible).
- Parameters:
-
grp ECP group R Destination point m Integer by which to multiply P Point to multiply f_rng RNG function (see notes) p_rng RNG parameter
- Returns:
- 0 if successful, MBEDTLS_ERR_ECP_INVALID_KEY if m is not a valid privkey or P is not a valid pubkey, MBEDTLS_ERR_MPI_ALLOC_FAILED if memory allocation failed
int mbedtls_ecp_muladd | ( | mbedtls_ecp_group * | grp, |
mbedtls_ecp_point * | R, | ||
const mbedtls_mpi * | m, | ||
const mbedtls_ecp_point * | P, | ||
const mbedtls_mpi * | n, | ||
const mbedtls_ecp_point * | Q | ||
) |
Multiplication and addition of two points by integers: R = m * P + n * Q (Not thread-safe to use same group in multiple threads)
- Note:
- In contrast to mbedtls_ecp_mul(), this function does not guarantee a constant execution flow and timing.
- Parameters:
-
grp ECP group R Destination point m Integer by which to multiply P P Point to multiply by m n Integer by which to multiply Q Q Point to be multiplied by n
- Returns:
- 0 if successful, MBEDTLS_ERR_ECP_INVALID_KEY if m or n is not a valid privkey or P or Q is not a valid pubkey, MBEDTLS_ERR_MPI_ALLOC_FAILED if memory allocation failed
int mbedtls_ecp_point_cmp | ( | const mbedtls_ecp_point * | P, |
const mbedtls_ecp_point * | Q | ||
) |
Compare two points.
- Note:
- This assumes the points are normalized. Otherwise, they may compare as "not equal" even if they are.
- Parameters:
-
P First point to compare Q Second point to compare
- Returns:
- 0 if the points are equal, MBEDTLS_ERR_ECP_BAD_INPUT_DATA otherwise
void mbedtls_ecp_point_free | ( | mbedtls_ecp_point * | pt ) |
void mbedtls_ecp_point_init | ( | mbedtls_ecp_point * | pt ) |
int mbedtls_ecp_point_read_binary | ( | const mbedtls_ecp_group * | grp, |
mbedtls_ecp_point * | P, | ||
const unsigned char * | buf, | ||
size_t | ilen | ||
) |
Import a point from unsigned binary data.
- Parameters:
-
grp Group to which the point should belong P Point to import buf Input buffer ilen Actual length of input
- Returns:
- 0 if successful, MBEDTLS_ERR_ECP_BAD_INPUT_DATA if input is invalid, MBEDTLS_ERR_MPI_ALLOC_FAILED if memory allocation failed, MBEDTLS_ERR_ECP_FEATURE_UNAVAILABLE if the point format is not implemented.
- Note:
- This function does NOT check that the point actually belongs to the given group, see mbedtls_ecp_check_pubkey() for that.
int mbedtls_ecp_point_read_string | ( | mbedtls_ecp_point * | P, |
int | radix, | ||
const char * | x, | ||
const char * | y | ||
) |
Import a non-zero point from two ASCII strings.
- Parameters:
-
P Destination point radix Input numeric base x First affine coordinate as a null-terminated string y Second affine coordinate as a null-terminated string
- Returns:
- 0 if successful, or a MBEDTLS_ERR_MPI_XXX error code
int mbedtls_ecp_point_write_binary | ( | const mbedtls_ecp_group * | grp, |
const mbedtls_ecp_point * | P, | ||
int | format, | ||
size_t * | olen, | ||
unsigned char * | buf, | ||
size_t | buflen | ||
) |
Export a point into unsigned binary data.
- Parameters:
-
grp Group to which the point should belong P Point to export format Point format, should be a MBEDTLS_ECP_PF_XXX macro olen Length of the actual output buf Output buffer buflen Length of the output buffer
- Returns:
- 0 if successful, or MBEDTLS_ERR_ECP_BAD_INPUT_DATA or MBEDTLS_ERR_ECP_BUFFER_TOO_SMALL
int mbedtls_ecp_self_test | ( | int | verbose ) |
int mbedtls_ecp_set_zero | ( | mbedtls_ecp_point * | pt ) |
int mbedtls_ecp_tls_read_group | ( | mbedtls_ecp_group * | grp, |
const unsigned char ** | buf, | ||
size_t | len | ||
) |
Set a group from a TLS ECParameters record.
- Parameters:
-
grp Destination group buf &(Start of input buffer) len Buffer length
- Note:
- buf is updated to point right after ECParameters on exit
- Returns:
- 0 if successful, MBEDTLS_ERR_MPI_XXX if initialization failed MBEDTLS_ERR_ECP_BAD_INPUT_DATA if input is invalid
int mbedtls_ecp_tls_read_point | ( | const mbedtls_ecp_group * | grp, |
mbedtls_ecp_point * | pt, | ||
const unsigned char ** | buf, | ||
size_t | len | ||
) |
Import a point from a TLS ECPoint record.
- Parameters:
-
grp ECP group used pt Destination point buf $(Start of input buffer) len Buffer length
- Note:
- buf is updated to point right after the ECPoint on exit
- Returns:
- 0 if successful, MBEDTLS_ERR_MPI_XXX if initialization failed MBEDTLS_ERR_ECP_BAD_INPUT_DATA if input is invalid
int mbedtls_ecp_tls_write_group | ( | const mbedtls_ecp_group * | grp, |
size_t * | olen, | ||
unsigned char * | buf, | ||
size_t | blen | ||
) |
int mbedtls_ecp_tls_write_point | ( | const mbedtls_ecp_group * | grp, |
const mbedtls_ecp_point * | pt, | ||
int | format, | ||
size_t * | olen, | ||
unsigned char * | buf, | ||
size_t | blen | ||
) |
Export a point as a TLS ECPoint record.
- Parameters:
-
grp ECP group used pt Point to export format Export format olen length of data written buf Buffer to write to blen Buffer length
- Returns:
- 0 if successful, or MBEDTLS_ERR_ECP_BAD_INPUT_DATA or MBEDTLS_ERR_ECP_BUFFER_TOO_SMALL
Generated on Tue Jul 12 2022 12:52:51 by
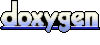