mbedtls ported to mbed-classic
Fork of mbedtls by
Embed:
(wiki syntax)
Show/hide line numbers
ecp.h
Go to the documentation of this file.
00001 /** 00002 * \file ecp.h 00003 * 00004 * \brief Elliptic curves over GF(p) 00005 * 00006 * Copyright (C) 2006-2015, ARM Limited, All Rights Reserved 00007 * SPDX-License-Identifier: Apache-2.0 00008 * 00009 * Licensed under the Apache License, Version 2.0 (the "License"); you may 00010 * not use this file except in compliance with the License. 00011 * You may obtain a copy of the License at 00012 * 00013 * http://www.apache.org/licenses/LICENSE-2.0 00014 * 00015 * Unless required by applicable law or agreed to in writing, software 00016 * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT 00017 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00018 * See the License for the specific language governing permissions and 00019 * limitations under the License. 00020 * 00021 * This file is part of mbed TLS (https://tls.mbed.org) 00022 */ 00023 #ifndef MBEDTLS_ECP_H 00024 #define MBEDTLS_ECP_H 00025 00026 #include "bignum.h" 00027 00028 /* 00029 * ECP error codes 00030 */ 00031 #define MBEDTLS_ERR_ECP_BAD_INPUT_DATA -0x4F80 /**< Bad input parameters to function. */ 00032 #define MBEDTLS_ERR_ECP_BUFFER_TOO_SMALL -0x4F00 /**< The buffer is too small to write to. */ 00033 #define MBEDTLS_ERR_ECP_FEATURE_UNAVAILABLE -0x4E80 /**< Requested curve not available. */ 00034 #define MBEDTLS_ERR_ECP_VERIFY_FAILED -0x4E00 /**< The signature is not valid. */ 00035 #define MBEDTLS_ERR_ECP_ALLOC_FAILED -0x4D80 /**< Memory allocation failed. */ 00036 #define MBEDTLS_ERR_ECP_RANDOM_FAILED -0x4D00 /**< Generation of random value, such as (ephemeral) key, failed. */ 00037 #define MBEDTLS_ERR_ECP_INVALID_KEY -0x4C80 /**< Invalid private or public key. */ 00038 #define MBEDTLS_ERR_ECP_SIG_LEN_MISMATCH -0x4C00 /**< Signature is valid but shorter than the user-supplied length. */ 00039 00040 #ifdef __cplusplus 00041 extern "C" { 00042 #endif 00043 00044 /** 00045 * Domain parameters (curve, subgroup and generator) identifiers. 00046 * 00047 * Only curves over prime fields are supported. 00048 * 00049 * \warning This library does not support validation of arbitrary domain 00050 * parameters. Therefore, only well-known domain parameters from trusted 00051 * sources should be used. See mbedtls_ecp_group_load(). 00052 */ 00053 typedef enum 00054 { 00055 MBEDTLS_ECP_DP_NONE = 0, 00056 MBEDTLS_ECP_DP_SECP192R1 , /*!< 192-bits NIST curve */ 00057 MBEDTLS_ECP_DP_SECP224R1 , /*!< 224-bits NIST curve */ 00058 MBEDTLS_ECP_DP_SECP256R1 , /*!< 256-bits NIST curve */ 00059 MBEDTLS_ECP_DP_SECP384R1 , /*!< 384-bits NIST curve */ 00060 MBEDTLS_ECP_DP_SECP521R1 , /*!< 521-bits NIST curve */ 00061 MBEDTLS_ECP_DP_BP256R1 , /*!< 256-bits Brainpool curve */ 00062 MBEDTLS_ECP_DP_BP384R1 , /*!< 384-bits Brainpool curve */ 00063 MBEDTLS_ECP_DP_BP512R1 , /*!< 512-bits Brainpool curve */ 00064 MBEDTLS_ECP_DP_CURVE25519 , /*!< Curve25519 */ 00065 MBEDTLS_ECP_DP_SECP192K1 , /*!< 192-bits "Koblitz" curve */ 00066 MBEDTLS_ECP_DP_SECP224K1 , /*!< 224-bits "Koblitz" curve */ 00067 MBEDTLS_ECP_DP_SECP256K1 , /*!< 256-bits "Koblitz" curve */ 00068 } mbedtls_ecp_group_id; 00069 00070 /** 00071 * Number of supported curves (plus one for NONE). 00072 * 00073 * (Montgomery curves excluded for now.) 00074 */ 00075 #define MBEDTLS_ECP_DP_MAX 12 00076 00077 /** 00078 * Curve information for use by other modules 00079 */ 00080 typedef struct 00081 { 00082 mbedtls_ecp_group_id grp_id ; /*!< Internal identifier */ 00083 uint16_t tls_id ; /*!< TLS NamedCurve identifier */ 00084 uint16_t bit_size ; /*!< Curve size in bits */ 00085 const char *name ; /*!< Human-friendly name */ 00086 } mbedtls_ecp_curve_info; 00087 00088 /** 00089 * \brief ECP point structure (jacobian coordinates) 00090 * 00091 * \note All functions expect and return points satisfying 00092 * the following condition: Z == 0 or Z == 1. (Other 00093 * values of Z are used by internal functions only.) 00094 * The point is zero, or "at infinity", if Z == 0. 00095 * Otherwise, X and Y are its standard (affine) coordinates. 00096 */ 00097 typedef struct 00098 { 00099 mbedtls_mpi X ; /*!< the point's X coordinate */ 00100 mbedtls_mpi Y ; /*!< the point's Y coordinate */ 00101 mbedtls_mpi Z ; /*!< the point's Z coordinate */ 00102 } 00103 mbedtls_ecp_point; 00104 00105 /** 00106 * \brief ECP group structure 00107 * 00108 * We consider two types of curves equations: 00109 * 1. Short Weierstrass y^2 = x^3 + A x + B mod P (SEC1 + RFC 4492) 00110 * 2. Montgomery, y^2 = x^3 + A x^2 + x mod P (Curve25519 + draft) 00111 * In both cases, a generator G for a prime-order subgroup is fixed. In the 00112 * short weierstrass, this subgroup is actually the whole curve, and its 00113 * cardinal is denoted by N. 00114 * 00115 * In the case of Short Weierstrass curves, our code requires that N is an odd 00116 * prime. (Use odd in mbedtls_ecp_mul() and prime in mbedtls_ecdsa_sign() for blinding.) 00117 * 00118 * In the case of Montgomery curves, we don't store A but (A + 2) / 4 which is 00119 * the quantity actually used in the formulas. Also, nbits is not the size of N 00120 * but the required size for private keys. 00121 * 00122 * If modp is NULL, reduction modulo P is done using a generic algorithm. 00123 * Otherwise, it must point to a function that takes an mbedtls_mpi in the range 00124 * 0..2^(2*pbits)-1 and transforms it in-place in an integer of little more 00125 * than pbits, so that the integer may be efficiently brought in the 0..P-1 00126 * range by a few additions or substractions. It must return 0 on success and 00127 * non-zero on failure. 00128 */ 00129 typedef struct 00130 { 00131 mbedtls_ecp_group_id id ; /*!< internal group identifier */ 00132 mbedtls_mpi P ; /*!< prime modulus of the base field */ 00133 mbedtls_mpi A ; /*!< 1. A in the equation, or 2. (A + 2) / 4 */ 00134 mbedtls_mpi B ; /*!< 1. B in the equation, or 2. unused */ 00135 mbedtls_ecp_point G ; /*!< generator of the (sub)group used */ 00136 mbedtls_mpi N ; /*!< 1. the order of G, or 2. unused */ 00137 size_t pbits ; /*!< number of bits in P */ 00138 size_t nbits ; /*!< number of bits in 1. P, or 2. private keys */ 00139 unsigned int h ; /*!< internal: 1 if the constants are static */ 00140 int (*modp)(mbedtls_mpi *); /*!< function for fast reduction mod P */ 00141 int (*t_pre)(mbedtls_ecp_point *, void *); /*!< unused */ 00142 int (*t_post)(mbedtls_ecp_point *, void *); /*!< unused */ 00143 void *t_data ; /*!< unused */ 00144 mbedtls_ecp_point *T ; /*!< pre-computed points for ecp_mul_comb() */ 00145 size_t T_size ; /*!< number for pre-computed points */ 00146 } 00147 mbedtls_ecp_group; 00148 00149 /** 00150 * \brief ECP key pair structure 00151 * 00152 * A generic key pair that could be used for ECDSA, fixed ECDH, etc. 00153 * 00154 * \note Members purposefully in the same order as struc mbedtls_ecdsa_context. 00155 */ 00156 typedef struct 00157 { 00158 mbedtls_ecp_group grp ; /*!< Elliptic curve and base point */ 00159 mbedtls_mpi d ; /*!< our secret value */ 00160 mbedtls_ecp_point Q ; /*!< our public value */ 00161 } 00162 mbedtls_ecp_keypair; 00163 00164 /** 00165 * \name SECTION: Module settings 00166 * 00167 * The configuration options you can set for this module are in this section. 00168 * Either change them in config.h or define them on the compiler command line. 00169 * \{ 00170 */ 00171 00172 #if !defined(MBEDTLS_ECP_MAX_BITS) 00173 /** 00174 * Maximum size of the groups (that is, of N and P) 00175 */ 00176 #define MBEDTLS_ECP_MAX_BITS 521 /**< Maximum bit size of groups */ 00177 #endif 00178 00179 #define MBEDTLS_ECP_MAX_BYTES ( ( MBEDTLS_ECP_MAX_BITS + 7 ) / 8 ) 00180 #define MBEDTLS_ECP_MAX_PT_LEN ( 2 * MBEDTLS_ECP_MAX_BYTES + 1 ) 00181 00182 #if !defined(MBEDTLS_ECP_WINDOW_SIZE) 00183 /* 00184 * Maximum "window" size used for point multiplication. 00185 * Default: 6. 00186 * Minimum value: 2. Maximum value: 7. 00187 * 00188 * Result is an array of at most ( 1 << ( MBEDTLS_ECP_WINDOW_SIZE - 1 ) ) 00189 * points used for point multiplication. This value is directly tied to EC 00190 * peak memory usage, so decreasing it by one should roughly cut memory usage 00191 * by two (if large curves are in use). 00192 * 00193 * Reduction in size may reduce speed, but larger curves are impacted first. 00194 * Sample performances (in ECDHE handshakes/s, with FIXED_POINT_OPTIM = 1): 00195 * w-size: 6 5 4 3 2 00196 * 521 145 141 135 120 97 00197 * 384 214 209 198 177 146 00198 * 256 320 320 303 262 226 00199 00200 * 224 475 475 453 398 342 00201 * 192 640 640 633 587 476 00202 */ 00203 #define MBEDTLS_ECP_WINDOW_SIZE 6 /**< Maximum window size used */ 00204 #endif /* MBEDTLS_ECP_WINDOW_SIZE */ 00205 00206 #if !defined(MBEDTLS_ECP_FIXED_POINT_OPTIM) 00207 /* 00208 * Trade memory for speed on fixed-point multiplication. 00209 * 00210 * This speeds up repeated multiplication of the generator (that is, the 00211 * multiplication in ECDSA signatures, and half of the multiplications in 00212 * ECDSA verification and ECDHE) by a factor roughly 3 to 4. 00213 * 00214 * The cost is increasing EC peak memory usage by a factor roughly 2. 00215 * 00216 * Change this value to 0 to reduce peak memory usage. 00217 */ 00218 #define MBEDTLS_ECP_FIXED_POINT_OPTIM 1 /**< Enable fixed-point speed-up */ 00219 #endif /* MBEDTLS_ECP_FIXED_POINT_OPTIM */ 00220 00221 /* \} name SECTION: Module settings */ 00222 00223 /* 00224 * Point formats, from RFC 4492's enum ECPointFormat 00225 */ 00226 #define MBEDTLS_ECP_PF_UNCOMPRESSED 0 /**< Uncompressed point format */ 00227 #define MBEDTLS_ECP_PF_COMPRESSED 1 /**< Compressed point format */ 00228 00229 /* 00230 * Some other constants from RFC 4492 00231 */ 00232 #define MBEDTLS_ECP_TLS_NAMED_CURVE 3 /**< ECCurveType's named_curve */ 00233 00234 /** 00235 * \brief Get the list of supported curves in order of preferrence 00236 * (full information) 00237 * 00238 * \return A statically allocated array, the last entry is 0. 00239 */ 00240 const mbedtls_ecp_curve_info *mbedtls_ecp_curve_list( void ); 00241 00242 /** 00243 * \brief Get the list of supported curves in order of preferrence 00244 * (grp_id only) 00245 * 00246 * \return A statically allocated array, 00247 * terminated with MBEDTLS_ECP_DP_NONE. 00248 */ 00249 const mbedtls_ecp_group_id *mbedtls_ecp_grp_id_list( void ); 00250 00251 /** 00252 * \brief Get curve information from an internal group identifier 00253 * 00254 * \param grp_id A MBEDTLS_ECP_DP_XXX value 00255 * 00256 * \return The associated curve information or NULL 00257 */ 00258 const mbedtls_ecp_curve_info *mbedtls_ecp_curve_info_from_grp_id( mbedtls_ecp_group_id grp_id ); 00259 00260 /** 00261 * \brief Get curve information from a TLS NamedCurve value 00262 * 00263 * \param tls_id A MBEDTLS_ECP_DP_XXX value 00264 * 00265 * \return The associated curve information or NULL 00266 */ 00267 const mbedtls_ecp_curve_info *mbedtls_ecp_curve_info_from_tls_id( uint16_t tls_id ); 00268 00269 /** 00270 * \brief Get curve information from a human-readable name 00271 * 00272 * \param name The name 00273 * 00274 * \return The associated curve information or NULL 00275 */ 00276 const mbedtls_ecp_curve_info *mbedtls_ecp_curve_info_from_name( const char *name ); 00277 00278 /** 00279 * \brief Initialize a point (as zero) 00280 */ 00281 void mbedtls_ecp_point_init( mbedtls_ecp_point *pt ); 00282 00283 /** 00284 * \brief Initialize a group (to something meaningless) 00285 */ 00286 void mbedtls_ecp_group_init( mbedtls_ecp_group *grp ); 00287 00288 /** 00289 * \brief Initialize a key pair (as an invalid one) 00290 */ 00291 void mbedtls_ecp_keypair_init( mbedtls_ecp_keypair *key ); 00292 00293 /** 00294 * \brief Free the components of a point 00295 */ 00296 void mbedtls_ecp_point_free( mbedtls_ecp_point *pt ); 00297 00298 /** 00299 * \brief Free the components of an ECP group 00300 */ 00301 void mbedtls_ecp_group_free( mbedtls_ecp_group *grp ); 00302 00303 /** 00304 * \brief Free the components of a key pair 00305 */ 00306 void mbedtls_ecp_keypair_free( mbedtls_ecp_keypair *key ); 00307 00308 /** 00309 * \brief Copy the contents of point Q into P 00310 * 00311 * \param P Destination point 00312 * \param Q Source point 00313 * 00314 * \return 0 if successful, 00315 * MBEDTLS_ERR_MPI_ALLOC_FAILED if memory allocation failed 00316 */ 00317 int mbedtls_ecp_copy( mbedtls_ecp_point *P, const mbedtls_ecp_point *Q ); 00318 00319 /** 00320 * \brief Copy the contents of a group object 00321 * 00322 * \param dst Destination group 00323 * \param src Source group 00324 * 00325 * \return 0 if successful, 00326 * MBEDTLS_ERR_MPI_ALLOC_FAILED if memory allocation failed 00327 */ 00328 int mbedtls_ecp_group_copy( mbedtls_ecp_group *dst, const mbedtls_ecp_group *src ); 00329 00330 /** 00331 * \brief Set a point to zero 00332 * 00333 * \param pt Destination point 00334 * 00335 * \return 0 if successful, 00336 * MBEDTLS_ERR_MPI_ALLOC_FAILED if memory allocation failed 00337 */ 00338 int mbedtls_ecp_set_zero( mbedtls_ecp_point *pt ); 00339 00340 /** 00341 * \brief Tell if a point is zero 00342 * 00343 * \param pt Point to test 00344 * 00345 * \return 1 if point is zero, 0 otherwise 00346 */ 00347 int mbedtls_ecp_is_zero( mbedtls_ecp_point *pt ); 00348 00349 /** 00350 * \brief Compare two points 00351 * 00352 * \note This assumes the points are normalized. Otherwise, 00353 * they may compare as "not equal" even if they are. 00354 * 00355 * \param P First point to compare 00356 * \param Q Second point to compare 00357 * 00358 * \return 0 if the points are equal, 00359 * MBEDTLS_ERR_ECP_BAD_INPUT_DATA otherwise 00360 */ 00361 int mbedtls_ecp_point_cmp( const mbedtls_ecp_point *P, 00362 const mbedtls_ecp_point *Q ); 00363 00364 /** 00365 * \brief Import a non-zero point from two ASCII strings 00366 * 00367 * \param P Destination point 00368 * \param radix Input numeric base 00369 * \param x First affine coordinate as a null-terminated string 00370 * \param y Second affine coordinate as a null-terminated string 00371 * 00372 * \return 0 if successful, or a MBEDTLS_ERR_MPI_XXX error code 00373 */ 00374 int mbedtls_ecp_point_read_string( mbedtls_ecp_point *P, int radix, 00375 const char *x, const char *y ); 00376 00377 /** 00378 * \brief Export a point into unsigned binary data 00379 * 00380 * \param grp Group to which the point should belong 00381 * \param P Point to export 00382 * \param format Point format, should be a MBEDTLS_ECP_PF_XXX macro 00383 * \param olen Length of the actual output 00384 * \param buf Output buffer 00385 * \param buflen Length of the output buffer 00386 * 00387 * \return 0 if successful, 00388 * or MBEDTLS_ERR_ECP_BAD_INPUT_DATA 00389 * or MBEDTLS_ERR_ECP_BUFFER_TOO_SMALL 00390 */ 00391 int mbedtls_ecp_point_write_binary( const mbedtls_ecp_group *grp, const mbedtls_ecp_point *P, 00392 int format, size_t *olen, 00393 unsigned char *buf, size_t buflen ); 00394 00395 /** 00396 * \brief Import a point from unsigned binary data 00397 * 00398 * \param grp Group to which the point should belong 00399 * \param P Point to import 00400 * \param buf Input buffer 00401 * \param ilen Actual length of input 00402 * 00403 * \return 0 if successful, 00404 * MBEDTLS_ERR_ECP_BAD_INPUT_DATA if input is invalid, 00405 * MBEDTLS_ERR_MPI_ALLOC_FAILED if memory allocation failed, 00406 * MBEDTLS_ERR_ECP_FEATURE_UNAVAILABLE if the point format 00407 * is not implemented. 00408 * 00409 * \note This function does NOT check that the point actually 00410 * belongs to the given group, see mbedtls_ecp_check_pubkey() for 00411 * that. 00412 */ 00413 int mbedtls_ecp_point_read_binary( const mbedtls_ecp_group *grp, mbedtls_ecp_point *P, 00414 const unsigned char *buf, size_t ilen ); 00415 00416 /** 00417 * \brief Import a point from a TLS ECPoint record 00418 * 00419 * \param grp ECP group used 00420 * \param pt Destination point 00421 * \param buf $(Start of input buffer) 00422 * \param len Buffer length 00423 * 00424 * \note buf is updated to point right after the ECPoint on exit 00425 * 00426 * \return 0 if successful, 00427 * MBEDTLS_ERR_MPI_XXX if initialization failed 00428 * MBEDTLS_ERR_ECP_BAD_INPUT_DATA if input is invalid 00429 */ 00430 int mbedtls_ecp_tls_read_point( const mbedtls_ecp_group *grp, mbedtls_ecp_point *pt, 00431 const unsigned char **buf, size_t len ); 00432 00433 /** 00434 * \brief Export a point as a TLS ECPoint record 00435 * 00436 * \param grp ECP group used 00437 * \param pt Point to export 00438 * \param format Export format 00439 * \param olen length of data written 00440 * \param buf Buffer to write to 00441 * \param blen Buffer length 00442 * 00443 * \return 0 if successful, 00444 * or MBEDTLS_ERR_ECP_BAD_INPUT_DATA 00445 * or MBEDTLS_ERR_ECP_BUFFER_TOO_SMALL 00446 */ 00447 int mbedtls_ecp_tls_write_point( const mbedtls_ecp_group *grp, const mbedtls_ecp_point *pt, 00448 int format, size_t *olen, 00449 unsigned char *buf, size_t blen ); 00450 00451 /** 00452 * \brief Set a group using well-known domain parameters 00453 * 00454 * \param grp Destination group 00455 * \param index Index in the list of well-known domain parameters 00456 * 00457 * \return 0 if successful, 00458 * MBEDTLS_ERR_MPI_XXX if initialization failed 00459 * MBEDTLS_ERR_ECP_FEATURE_UNAVAILABLE for unkownn groups 00460 * 00461 * \note Index should be a value of RFC 4492's enum NamedCurve, 00462 * usually in the form of a MBEDTLS_ECP_DP_XXX macro. 00463 */ 00464 int mbedtls_ecp_group_load( mbedtls_ecp_group *grp, mbedtls_ecp_group_id index ); 00465 00466 /** 00467 * \brief Set a group from a TLS ECParameters record 00468 * 00469 * \param grp Destination group 00470 * \param buf &(Start of input buffer) 00471 * \param len Buffer length 00472 * 00473 * \note buf is updated to point right after ECParameters on exit 00474 * 00475 * \return 0 if successful, 00476 * MBEDTLS_ERR_MPI_XXX if initialization failed 00477 * MBEDTLS_ERR_ECP_BAD_INPUT_DATA if input is invalid 00478 */ 00479 int mbedtls_ecp_tls_read_group( mbedtls_ecp_group *grp, const unsigned char **buf, size_t len ); 00480 00481 /** 00482 * \brief Write the TLS ECParameters record for a group 00483 * 00484 * \param grp ECP group used 00485 * \param olen Number of bytes actually written 00486 * \param buf Buffer to write to 00487 * \param blen Buffer length 00488 * 00489 * \return 0 if successful, 00490 * or MBEDTLS_ERR_ECP_BUFFER_TOO_SMALL 00491 */ 00492 int mbedtls_ecp_tls_write_group( const mbedtls_ecp_group *grp, size_t *olen, 00493 unsigned char *buf, size_t blen ); 00494 00495 /** 00496 * \brief Multiplication by an integer: R = m * P 00497 * (Not thread-safe to use same group in multiple threads) 00498 * 00499 * \note In order to prevent timing attacks, this function 00500 * executes the exact same sequence of (base field) 00501 * operations for any valid m. It avoids any if-branch or 00502 * array index depending on the value of m. 00503 * 00504 * \note If f_rng is not NULL, it is used to randomize intermediate 00505 * results in order to prevent potential timing attacks 00506 * targeting these results. It is recommended to always 00507 * provide a non-NULL f_rng (the overhead is negligible). 00508 * 00509 * \param grp ECP group 00510 * \param R Destination point 00511 * \param m Integer by which to multiply 00512 * \param P Point to multiply 00513 * \param f_rng RNG function (see notes) 00514 * \param p_rng RNG parameter 00515 * 00516 * \return 0 if successful, 00517 * MBEDTLS_ERR_ECP_INVALID_KEY if m is not a valid privkey 00518 * or P is not a valid pubkey, 00519 * MBEDTLS_ERR_MPI_ALLOC_FAILED if memory allocation failed 00520 */ 00521 int mbedtls_ecp_mul( mbedtls_ecp_group *grp, mbedtls_ecp_point *R, 00522 const mbedtls_mpi *m, const mbedtls_ecp_point *P, 00523 int (*f_rng)(void *, unsigned char *, size_t), void *p_rng ); 00524 00525 /** 00526 * \brief Multiplication and addition of two points by integers: 00527 * R = m * P + n * Q 00528 * (Not thread-safe to use same group in multiple threads) 00529 * 00530 * \note In contrast to mbedtls_ecp_mul(), this function does not guarantee 00531 * a constant execution flow and timing. 00532 * 00533 * \param grp ECP group 00534 * \param R Destination point 00535 * \param m Integer by which to multiply P 00536 * \param P Point to multiply by m 00537 * \param n Integer by which to multiply Q 00538 * \param Q Point to be multiplied by n 00539 * 00540 * \return 0 if successful, 00541 * MBEDTLS_ERR_ECP_INVALID_KEY if m or n is not a valid privkey 00542 * or P or Q is not a valid pubkey, 00543 * MBEDTLS_ERR_MPI_ALLOC_FAILED if memory allocation failed 00544 */ 00545 int mbedtls_ecp_muladd( mbedtls_ecp_group *grp, mbedtls_ecp_point *R, 00546 const mbedtls_mpi *m, const mbedtls_ecp_point *P, 00547 const mbedtls_mpi *n, const mbedtls_ecp_point *Q ); 00548 00549 /** 00550 * \brief Check that a point is a valid public key on this curve 00551 * 00552 * \param grp Curve/group the point should belong to 00553 * \param pt Point to check 00554 * 00555 * \return 0 if point is a valid public key, 00556 * MBEDTLS_ERR_ECP_INVALID_KEY otherwise. 00557 * 00558 * \note This function only checks the point is non-zero, has valid 00559 * coordinates and lies on the curve, but not that it is 00560 * indeed a multiple of G. This is additional check is more 00561 * expensive, isn't required by standards, and shouldn't be 00562 * necessary if the group used has a small cofactor. In 00563 * particular, it is useless for the NIST groups which all 00564 * have a cofactor of 1. 00565 * 00566 * \note Uses bare components rather than an mbedtls_ecp_keypair structure 00567 * in order to ease use with other structures such as 00568 * mbedtls_ecdh_context of mbedtls_ecdsa_context. 00569 */ 00570 int mbedtls_ecp_check_pubkey( const mbedtls_ecp_group *grp, const mbedtls_ecp_point *pt ); 00571 00572 /** 00573 * \brief Check that an mbedtls_mpi is a valid private key for this curve 00574 * 00575 * \param grp Group used 00576 * \param d Integer to check 00577 * 00578 * \return 0 if point is a valid private key, 00579 * MBEDTLS_ERR_ECP_INVALID_KEY otherwise. 00580 * 00581 * \note Uses bare components rather than an mbedtls_ecp_keypair structure 00582 * in order to ease use with other structures such as 00583 * mbedtls_ecdh_context of mbedtls_ecdsa_context. 00584 */ 00585 int mbedtls_ecp_check_privkey( const mbedtls_ecp_group *grp, const mbedtls_mpi *d ); 00586 00587 /** 00588 * \brief Generate a keypair with configurable base point 00589 * 00590 * \param grp ECP group 00591 * \param G Chosen base point 00592 * \param d Destination MPI (secret part) 00593 * \param Q Destination point (public part) 00594 * \param f_rng RNG function 00595 * \param p_rng RNG parameter 00596 * 00597 * \return 0 if successful, 00598 * or a MBEDTLS_ERR_ECP_XXX or MBEDTLS_MPI_XXX error code 00599 * 00600 * \note Uses bare components rather than an mbedtls_ecp_keypair structure 00601 * in order to ease use with other structures such as 00602 * mbedtls_ecdh_context of mbedtls_ecdsa_context. 00603 */ 00604 int mbedtls_ecp_gen_keypair_base( mbedtls_ecp_group *grp, 00605 const mbedtls_ecp_point *G, 00606 mbedtls_mpi *d, mbedtls_ecp_point *Q, 00607 int (*f_rng)(void *, unsigned char *, size_t), 00608 void *p_rng ); 00609 00610 /** 00611 * \brief Generate a keypair 00612 * 00613 * \param grp ECP group 00614 * \param d Destination MPI (secret part) 00615 * \param Q Destination point (public part) 00616 * \param f_rng RNG function 00617 * \param p_rng RNG parameter 00618 * 00619 * \return 0 if successful, 00620 * or a MBEDTLS_ERR_ECP_XXX or MBEDTLS_MPI_XXX error code 00621 * 00622 * \note Uses bare components rather than an mbedtls_ecp_keypair structure 00623 * in order to ease use with other structures such as 00624 * mbedtls_ecdh_context of mbedtls_ecdsa_context. 00625 */ 00626 int mbedtls_ecp_gen_keypair( mbedtls_ecp_group *grp, mbedtls_mpi *d, mbedtls_ecp_point *Q, 00627 int (*f_rng)(void *, unsigned char *, size_t), 00628 void *p_rng ); 00629 00630 /** 00631 * \brief Generate a keypair 00632 * 00633 * \param grp_id ECP group identifier 00634 * \param key Destination keypair 00635 * \param f_rng RNG function 00636 * \param p_rng RNG parameter 00637 * 00638 * \return 0 if successful, 00639 * or a MBEDTLS_ERR_ECP_XXX or MBEDTLS_MPI_XXX error code 00640 */ 00641 int mbedtls_ecp_gen_key( mbedtls_ecp_group_id grp_id, mbedtls_ecp_keypair *key, 00642 int (*f_rng)(void *, unsigned char *, size_t), void *p_rng ); 00643 00644 /** 00645 * \brief Check a public-private key pair 00646 * 00647 * \param pub Keypair structure holding a public key 00648 * \param prv Keypair structure holding a private (plus public) key 00649 * 00650 * \return 0 if successful (keys are valid and match), or 00651 * MBEDTLS_ERR_ECP_BAD_INPUT_DATA, or 00652 * a MBEDTLS_ERR_ECP_XXX or MBEDTLS_ERR_MPI_XXX code. 00653 */ 00654 int mbedtls_ecp_check_pub_priv( const mbedtls_ecp_keypair *pub, const mbedtls_ecp_keypair *prv ); 00655 00656 #if defined(MBEDTLS_SELF_TEST) 00657 /** 00658 * \brief Checkup routine 00659 * 00660 * \return 0 if successful, or 1 if a test failed 00661 */ 00662 int mbedtls_ecp_self_test( int verbose ); 00663 #endif 00664 00665 #ifdef __cplusplus 00666 } 00667 #endif 00668 00669 #endif /* ecp.h */
Generated on Tue Jul 12 2022 12:52:43 by
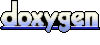