I2C-Library for various sensors
TWI_Master Class Reference
TWI_Master is used for interacting with different libraries of I2C sensors, which I wrote. More...
#include <twi_master.h>
Public Member Functions | |
TWI_Master (PinName sda, PinName scl, uint32_t frequency=400000) | |
Construct a new TWI_Master object. | |
void | i2c_write (uint8_t i2c_address, uint8_t slave_address, const uint8_t data) |
Writes data into specified address (changes every bit) | |
void | i2c_writeBits (uint8_t i2c_address, uint8_t slave_address, const uint8_t data, uint8_t mask) |
Writes bits by masking the necessary bits. | |
uint8_t | i2c_readBits (uint8_t i2c_address, uint8_t slave_address, uint8_t mask) |
Reads one register from the specified address and masks it with the given mask. | |
void | i2c_readSeries (uint8_t i2c_address, uint8_t slave_address, uint8_t *data, uint8_t length) |
Reads multiple registers. | |
uint8_t | i2c_read (uint8_t i2c_address, uint8_t slave_address) |
Reads one register from the specified register-address. |
Detailed Description
TWI_Master is used for interacting with different libraries of I2C sensors, which I wrote.
This library has been inspired by the Adafruit Sensor-Library and the I2Cdevlib by jrowberg.
It is not an I2C driver, since the existing I2C functions are used and new things, such as masking and individual bit changes, are built in around them.
Definition at line 21 of file twi_master.h.
Constructor & Destructor Documentation
TWI_Master | ( | PinName | sda, |
PinName | scl, | ||
uint32_t | frequency = 400000 |
||
) |
Construct a new TWI_Master object.
The given parameters should be all the necessary things to start interacting with the other libraries.
- Parameters:
-
sda SDA-Pin scl SCL-Pin frequency I2C-frequency (default: 400kHz)
Definition at line 19 of file twi_master.cpp.
Member Function Documentation
uint8_t i2c_read | ( | uint8_t | i2c_address, |
uint8_t | slave_address | ||
) |
Reads one register from the specified register-address.
- Parameters:
-
slave_address the desired register-address for reading
- Returns:
- uint8_t returns read data
Definition at line 85 of file twi_master.cpp.
uint8_t i2c_readBits | ( | uint8_t | i2c_address, |
uint8_t | slave_address, | ||
uint8_t | mask | ||
) |
Reads one register from the specified address and masks it with the given mask.
- Parameters:
-
slave_address the desired register-address for reading mask the mask for the desired bits
- Returns:
- uint8_t returns the masked data
Definition at line 102 of file twi_master.cpp.
void i2c_readSeries | ( | uint8_t | i2c_address, |
uint8_t | slave_address, | ||
uint8_t * | data, | ||
uint8_t | length | ||
) |
Reads multiple registers.
- Parameters:
-
slave_address the start register-address data array, which the functions saves the data to length the length of the read-sequence
Definition at line 68 of file twi_master.cpp.
void i2c_write | ( | uint8_t | i2c_address, |
uint8_t | slave_address, | ||
const uint8_t | data | ||
) |
Writes data into specified address (changes every bit)
- Parameters:
-
slave_address register-address which will be written to data 8-bit value that overwrites the register-value
Definition at line 31 of file twi_master.cpp.
void i2c_writeBits | ( | uint8_t | i2c_address, |
uint8_t | slave_address, | ||
const uint8_t | data, | ||
uint8_t | mask | ||
) |
Writes bits by masking the necessary bits.
The data variables will be normally masked with the mask (data & mask)
, the register will be masked by the inverted mask (register_value & ~mask)
. What will be written to the register will be new_register_data = (data | register_value)
.
- Parameters:
-
slave_address register-address that will be edited data 8-bit value that edit the editable bits (which are determined by the mask) mask Bits that want to be changed, all the other bits will be saved.
Definition at line 46 of file twi_master.cpp.
Generated on Fri Jul 15 2022 19:49:03 by
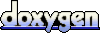