I2C-Library for various sensors
Embed:
(wiki syntax)
Show/hide line numbers
twi_master.cpp
Go to the documentation of this file.
00001 /** 00002 * @brief Implementation of TWI_Master class. 00003 * 00004 * @file twi_master.cpp 00005 * @author Joel von Rotz 00006 * @date 18.07.2018 00007 */ 00008 #include "mbed.h" 00009 #include "twi_master.h " 00010 00011 /** 00012 * @brief Construct a new TWI_Master object. The given parameters should be all the necessary things to start 00013 * interacting with the other libraries. 00014 * 00015 * @param sda SDA-Pin 00016 * @param scl SCL-Pin 00017 * @param frequency I2C-frequency (default: 400kHz) 00018 */ 00019 TWI_Master::TWI_Master(PinName sda, PinName scl, uint32_t frequency) : 00020 m_i2c_sensor(sda, scl) 00021 { 00022 m_i2c_sensor.frequency(frequency); 00023 } 00024 00025 /** 00026 * @brief Writes data into specified address (changes every bit) 00027 * 00028 * @param slave_address register-address which will be written to 00029 * @param data 8-bit value that overwrites the register-value 00030 */ 00031 void TWI_Master::i2c_write(uint8_t i2c_address, uint8_t slave_address, const uint8_t data) 00032 { 00033 char writeArray[2] = {slave_address, data}; 00034 m_i2c_sensor.write(i2c_address, writeArray, 2, false); 00035 } 00036 00037 /** 00038 * @brief Writes bits by masking the necessary bits. The data variables will be normally masked with the mask 00039 * <code><strong>(data & mask)</strong></code>, the register will be masked by the inverted mask <code><strong>(register_value & ~mask)</strong></code>. 00040 * What will be written to the register will be <code><strong> new_register_data = (data | register_value) </strong></code>. 00041 * 00042 * @param slave_address register-address that will be edited 00043 * @param data 8-bit value that edit the editable bits (which are determined by the mask) 00044 * @param mask Bits that want to be changed, all the other bits will be saved. 00045 */ 00046 void TWI_Master::i2c_writeBits(uint8_t i2c_address, uint8_t slave_address, const uint8_t data, uint8_t mask) 00047 { 00048 char current_value; 00049 char writeArray[2] = {slave_address, data}; 00050 00051 //Get current value of desired register and mask it with mask-value 00052 current_value = i2c_read(i2c_address, slave_address); 00053 current_value &= ~mask; 00054 00055 //Combine Data with the new data (additionaly masking the data) and send it to the Sensor 00056 writeArray[1] = current_value | (data & mask); 00057 00058 m_i2c_sensor.write(i2c_address, writeArray, 2, false); 00059 } 00060 00061 /** 00062 * @brief Reads multiple registers 00063 * 00064 * @param slave_address the start register-address 00065 * @param data array, which the functions saves the data to 00066 * @param length the length of the read-sequence 00067 */ 00068 void TWI_Master::i2c_readSeries(uint8_t i2c_address, uint8_t slave_address, uint8_t *data, uint8_t length) 00069 { 00070 char *data_array; 00071 data_array = reinterpret_cast<char*>(data); 00072 00073 const char temp_address = slave_address; 00074 00075 m_i2c_sensor.write(i2c_address, &temp_address, 1, true); 00076 m_i2c_sensor.read(i2c_address, data_array,length, false); 00077 } 00078 00079 /** 00080 * @brief Reads one register from the specified register-address. 00081 * 00082 * @param slave_address the desired register-address for reading 00083 * @return uint8_t returns read data 00084 */ 00085 uint8_t TWI_Master::i2c_read(uint8_t i2c_address, uint8_t slave_address) 00086 { 00087 char data; 00088 const char temp_address = slave_address; 00089 m_i2c_sensor.write(i2c_address, &temp_address, 1, true); 00090 m_i2c_sensor.read(i2c_address, &data, 1, false); 00091 return data; 00092 } 00093 00094 /** 00095 * @brief Reads one register from the specified address and masks it with 00096 * the given mask. 00097 * 00098 * @param slave_address the desired register-address for reading 00099 * @param mask the mask for the desired bits 00100 * @return uint8_t returns the masked data 00101 */ 00102 uint8_t TWI_Master::i2c_readBits(uint8_t i2c_address, uint8_t slave_address, uint8_t mask) 00103 { 00104 char data; 00105 const char temp_address = slave_address; 00106 m_i2c_sensor.write(i2c_address, &temp_address, 1, true); 00107 m_i2c_sensor.read(i2c_address, &data, 1, false); 00108 return data & mask; 00109 } 00110
Generated on Fri Jul 15 2022 19:49:03 by
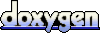