I2C-Library for various sensors
Embed:
(wiki syntax)
Show/hide line numbers
twi_master.h
Go to the documentation of this file.
00001 /** 00002 * @brief 00003 * 00004 * @file twi_master.h 00005 * @author Joel von Rotz 00006 * @date 18.07.2018 00007 */ 00008 #ifndef TWI_MASTER_H 00009 #define TWI_MASTER_H 00010 #include "mbed.h" 00011 00012 /** 00013 * @brief <strong>TWI_Master</strong> is used for interacting with different libraries of I2C sensors, which I wrote. 00014 * This library has been inspired by the <a href="https://github.com/adafruit/Adafruit_Sensor">Adafruit Sensor-Library</a> 00015 * and the <a href="https://github.com/jrowberg/i2cdevlib/">I2Cdevlib</a> by jrowberg. 00016 * \n\n 00017 * It is not an I2C driver, since the existing I2C functions are used and new things, such as masking and individual 00018 * bit changes, are built in around them. 00019 * \n\n 00020 */ 00021 class TWI_Master 00022 { 00023 public: 00024 TWI_Master(PinName sda, PinName scl, uint32_t frequency = 400000); 00025 void i2c_write(uint8_t i2c_address, uint8_t slave_address, const uint8_t data); 00026 void i2c_writeBits(uint8_t i2c_address, uint8_t slave_address, const uint8_t data, uint8_t mask); 00027 uint8_t i2c_readBits(uint8_t i2c_address, uint8_t slave_address, uint8_t mask); 00028 void i2c_readSeries(uint8_t i2c_address, uint8_t slave_address, uint8_t *data, uint8_t length); 00029 uint8_t i2c_read(uint8_t i2c_address, uint8_t slave_address); 00030 00031 private: 00032 I2C m_i2c_sensor; 00033 uint32_t m_i2c_frequency; 00034 }; 00035 00036 #endif 00037
Generated on Fri Jul 15 2022 19:49:03 by
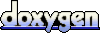