polymorphic base structures for easily implementing different gaits. Triangle wave is already in there
Dependents: robotic_fish_ver_4_9_pixy
fishgait.h
00001 #pragma once 00002 #include "mbed.h" 00003 #define MAXGAITS 10 00004 00005 class fishgait 00006 { 00007 protected: 00008 Timer* t; 00009 public: 00010 fishgait(); //give the constructor a timer to use 00011 ~fishgait(); 00012 void setTimer(Timer* tObject); //give the instance a timer to base its calculations off of instead of making a new one 00013 virtual float compute()=0; //asbtract function to compute output duty cycle. Must redefine in child classes 00014 }; 00015 /* 00016 class mixedGait : public fishgait 00017 { 00018 private: 00019 fishgait gaits[MAXGAITS]; 00020 float weights[MAXGAITS]; 00021 int count; 00022 public: 00023 mixedGait(); 00024 void attach(fishgait gait, float weight); //gait to attach and its weight in the overall result. weights will be normalized 00025 float compute(); //return the mixed signal pattern 00026 }; 00027 */ 00028 class triangleGait: public fishgait 00029 { 00030 private: 00031 float frq; 00032 float amp; 00033 public: 00034 triangleGait(float freq, float amplitude); 00035 //~triangleGait(); 00036 float compute(); //this does a triangle wave pattern. frequency and amplitude controlled 00037 }; 00038 00039 class squareGait: public fishgait 00040 { 00041 private: 00042 float frq; 00043 float amp; 00044 public: 00045 squareGait(float freq, float amplitude); 00046 //~triangleGait(); 00047 float compute(); //this does a triangle wave pattern. frequency and amplitude controlled 00048 }; 00049 00050 class sawGait: public fishgait 00051 { 00052 private: 00053 float frq; 00054 float amp; 00055 public: 00056 sawGait(float freq, float amplitude); 00057 //~triangleGait(); 00058 float compute(); //this does a triangle wave pattern. frequency and amplitude controlled 00059 };
Generated on Tue Jul 12 2022 16:19:49 by
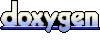