Basic implementation of Xbus message parsing and generation for embedded processors. The code has no dependencies and should also work for other MCU architectures than ARM provided a C99 compiler is available.
Dependents: MTi-1_example_LPC1768 MTi-1_rikbeun MTi-1_example MTi-1_example ... more
xsdeviceid.c
00001 /*! 00002 * \file 00003 * \copyright Copyright (C) Xsens Technologies B.V., 2015. 00004 * 00005 * Licensed under the Apache License, Version 2.0 (the "License"); you may not 00006 * use this file except in compliance with the License. You may obtain a copy 00007 * of the License at 00008 * 00009 * http://www.apache.org/licenses/LICENSE-2.0 00010 * 00011 * Unless required by applicable law or agreed to in writing, software 00012 * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT 00013 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the 00014 * License for the specific language governing permissions and limitations 00015 * under the License. 00016 */ 00017 00018 #include "xsdeviceid.h " 00019 00020 /*! 00021 * \brief Return true if device ID corresponds to a MTi-1 series device. 00022 */ 00023 bool XsDeviceId_isMtMk4_X(uint32_t deviceId) 00024 { 00025 uint8_t deviceSeries = (deviceId >> 20) & 0xF; 00026 return ((deviceSeries == 0x8) || (deviceSeries == 0xC)); 00027 } 00028 00029 /*! 00030 * \brief Get a string describing the function of the MTi device. 00031 */ 00032 char const* XsDeviceId_functionDescription(enum DeviceFunction function) 00033 { 00034 switch (function) 00035 { 00036 case DF_IMU: 00037 return "Inertial Measurement Unit"; 00038 00039 case DF_VRU: 00040 return "Vertical Reference Unit"; 00041 00042 case DF_AHRS: 00043 return "Attitude Heading Reference System"; 00044 } 00045 00046 return "Unknown device function"; 00047 } 00048
Generated on Thu Jul 14 2022 08:57:02 by
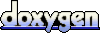