X_NUCLEO_NFC02A1 library for M24LR
Dependencies: ST_INTERFACES
Dependents: HelloWorld_NFC02A1_mbedOS HelloWorld_NFC02A1laatste HelloWorld_NFC02A1
Fork of X_NUCLEO_NFC02A1 by
XNucleoNFC02A1.h
00001 /** 00002 ****************************************************************************** 00003 * @file X_NUCLEO_NFC02A1.cpp 00004 * @author AMG Central Lab 00005 * @version V2.0.0 00006 * @date 19 May 2017 00007 * @brief Singleton class that controls all the electronics inside the 00008 * X_NUCLEO_NFC02A1 expansion board. 00009 ****************************************************************************** 00010 * @attention 00011 * 00012 * <h2><center>© COPYRIGHT(c) 2016 STMicroelectronics</center></h2> 00013 * 00014 * Redistribution and use in source and binary forms, with or without modification, 00015 * are permitted provided that the following conditions are met: 00016 * 1. Redistributions of source code must retain the above copyright notice, 00017 * this list of conditions and the following disclaimer. 00018 * 2. Redistributions in binary form must reproduce the above copyright notice, 00019 * this list of conditions and the following disclaimer in the documentation 00020 * and/or other materials provided with the distribution. 00021 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00022 * may be used to endorse or promote products derived from this software 00023 * without specific prior written permission. 00024 * 00025 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00026 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00027 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00028 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00029 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00030 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00031 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00032 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00033 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00034 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00035 * 00036 ****************************************************************************** 00037 */ 00038 00039 #ifndef X_NUCLEO_NFC02A1_H_ 00040 #define X_NUCLEO_NFC02A1_H_ 00041 #include <stdint.h> 00042 00043 #include "mbed.h" 00044 00045 #include "m24lr/M24LR.h" 00046 00047 /** 00048 * Singleton class that controls all the electronics inside the X_NUCLEO_NFC02A1 expansion board. 00049 */ 00050 class XNucleoNFC02A1 { 00051 00052 public: 00053 00054 private: 00055 /** 00056 * Pointer to the singleton instance, NULL if not allocated. 00057 */ 00058 static XNucleoNFC02A1 *mInstance; 00059 /** 00060 * I2C address of the M24LR chip. 00061 */ 00062 static const uint8_t M24LR_ADDR; 00063 00064 static const uint8_t M24LR_ADDR_DATA; 00065 00066 /** 00067 * Constructor 00068 * @param devI2C I2C channel used to communicate with the board. 00069 * @param eventCallback Function that will be called when the gpo pin status change. 00070 * @param gpoName Name of the gpio pin of the M24LR chip. 00071 * @param RFDisableName Pin that controls the rf antenna status. 00072 * @param led1Name Pin to control the led1 status. 00073 * @param led2Name Pin to control the led1 status. 00074 * @param led3Name Pin to control the led1 status. 00075 */ 00076 XNucleoNFC02A1(DevI2C &devI2C, 00077 const PinName &gpoName, const PinName &RFDisableName, 00078 const PinName &led1Name, const PinName &led2Name, 00079 const PinName &led3Name): 00080 mM24LR(M24LR_ADDR, M24LR_ADDR_DATA, devI2C), 00081 mNfcLed1(led1Name),mNfcLed2(led2Name),mNfcLed3(led3Name){} 00082 00083 public: 00084 static const PinName DEFAULT_SDA_PIN; //!< Default pin used for the M24LR SDA signal. 00085 static const PinName DEFAULT_SDL_PIN; //!< Default pin used for the M24LR SDL signal. 00086 static const PinName DEFAULT_GPO_PIN; //!< Default pin used for the M24LR GPO signal. 00087 static const PinName DEFAULT_RF_DISABLE_PIN; //!< Default pin used for M24LR RF_DISABLE signal. 00088 static const PinName DEFAULT_LED1_PIN; //!< Default pin to control the led 1. 00089 static const PinName DEFAULT_LED2_PIN; //!< Default pin to control the led 2. 00090 static const PinName DEFAULT_LED3_PIN; //!< Default pin to control the led 3. 00091 00092 /** 00093 * Create or return an instance of X_NUCLEO_NFC02A1. 00094 * @param devI2C I2C channel used to communicate with the board. 00095 * @param eventCallback Function that will be called when the gpo pin status change. 00096 * @param gpoName Name of the gpio pin of the M24LR chip. 00097 * @param RFDisableName Pin that controls the rf antenna status. 00098 * @param led1Name Pin to control the led1 status. 00099 * @param led2Name Pin to control the led1 status. 00100 * @param led3Name Pin to control the led1 status. 00101 */ 00102 static XNucleoNFC02A1* instance(DevI2C &devI2C, 00103 const PinName &gpoName = DEFAULT_GPO_PIN, 00104 const PinName &RFDisableName = DEFAULT_RF_DISABLE_PIN, 00105 const PinName &led1Name = DEFAULT_LED1_PIN, 00106 const PinName &led2Name = DEFAULT_LED2_PIN, 00107 const PinName &led3Name = DEFAULT_LED3_PIN); 00108 00109 /** 00110 * @return board led1. 00111 */ 00112 DigitalOut& get_led1 () { 00113 return mNfcLed1; 00114 } 00115 00116 /** 00117 * @return board led2. 00118 */ 00119 DigitalOut& get_led2 () { 00120 return mNfcLed2; 00121 } 00122 00123 /** 00124 * @return board led3. 00125 */ 00126 DigitalOut& get_led3 () { 00127 return mNfcLed3; 00128 } 00129 00130 /** 00131 * @return NFC Chip. 00132 */ 00133 M24LR& get_M24LR () { 00134 return mM24LR; 00135 } 00136 00137 virtual ~XNucleoNFC02A1() { 00138 } 00139 00140 private: 00141 M24LR mM24LR; 00142 00143 DigitalOut mNfcLed1; 00144 DigitalOut mNfcLed2; 00145 DigitalOut mNfcLed3; 00146 00147 }; 00148 #endif /* X_NUCLEO_NFC02A1_H_ */
Generated on Wed Jul 13 2022 03:37:30 by
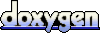