X_NUCLEO_NFC02A1 library for M24LR
Dependencies: ST_INTERFACES
Dependents: HelloWorld_NFC02A1_mbedOS HelloWorld_NFC02A1laatste HelloWorld_NFC02A1
Fork of X_NUCLEO_NFC02A1 by
DevI2C.h
00001 /** 00002 ****************************************************************************** 00003 * @file DevI2C.h 00004 * @author AST / EST / AMG-CL 00005 * @version V0.0.2 00006 * @date 25-July-2016 00007 * @brief Header file for a special I2C class DevI2C which provides some 00008 * helper function for on-board communication 00009 ****************************************************************************** 00010 * @attention 00011 * 00012 * <h2><center>© COPYRIGHT(c) 2014 STMicroelectronics</center></h2> 00013 * 00014 * Redistribution and use in source and binary forms, with or without modification, 00015 * are permitted provided that the following conditions are met: 00016 * 1. Redistributions of source code must retain the above copyright notice, 00017 * this list of conditions and the following disclaimer. 00018 * 2. Redistributions in binary form must reproduce the above copyright notice, 00019 * this list of conditions and the following disclaimer in the documentation 00020 * and/or other materials provided with the distribution. 00021 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00022 * may be used to endorse or promote products derived from this software 00023 * without specific prior written permission. 00024 * 00025 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00026 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00027 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00028 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00029 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00030 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00031 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00032 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00033 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00034 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00035 * 00036 ****************************************************************************** 00037 */ 00038 00039 /* Define to prevent from recursive inclusion --------------------------------*/ 00040 #ifndef __DEV_I2C_H 00041 #define __DEV_I2C_H 00042 00043 /* Includes ------------------------------------------------------------------*/ 00044 #include "mbed.h" 00045 00046 /* Classes -------------------------------------------------------------------*/ 00047 /** Helper class DevI2C providing functions for multi-register I2C communication 00048 * common for a series of I2C devices 00049 */ 00050 class DevI2C : public I2C 00051 { 00052 public: 00053 00054 /** Create a DevI2C Master interface, connected to the specified pins 00055 * 00056 * @param sda I2C data line pin 00057 * @param scl I2C clock line pin 00058 */ 00059 DevI2C(PinName sda, PinName scl) : I2C(sda, scl) {} 00060 00061 /** 00062 * @brief Writes a buffer towards the I2C peripheral device. 00063 * @param pBuffer pointer to the byte-array data to send 00064 * @param DeviceAddr specifies the peripheral device slave address. 00065 * @param RegisterAddr specifies the internal address register 00066 * where to start writing to (must be correctly masked). 00067 * @param NumByteToWrite number of bytes to be written. 00068 * @retval 0 if ok, 00069 * @retval -1 if an I2C error has occured, or 00070 * @retval -2 on temporary buffer overflow (i.e. NumByteToWrite was too high) 00071 * @note On some devices if NumByteToWrite is greater 00072 * than one, the RegisterAddr must be masked correctly! 00073 */ 00074 int i2c_write(uint8_t* pBuffer, uint8_t DeviceAddr, uint8_t RegisterAddr, 00075 uint16_t NumByteToWrite) 00076 { 00077 int ret; 00078 uint8_t tmp[TEMP_BUF_SIZE]; 00079 00080 if(NumByteToWrite >= TEMP_BUF_SIZE) return -2; 00081 00082 /* First, send device address. Then, send data and STOP condition */ 00083 tmp[0] = RegisterAddr; 00084 memcpy(tmp+1, pBuffer, NumByteToWrite); 00085 00086 ret = write(DeviceAddr, (const char*)tmp, NumByteToWrite+1, false); 00087 00088 if(ret) return -1; 00089 return 0; 00090 } 00091 00092 /** 00093 * @brief Writes a buffer towards the I2C peripheral device. 00094 * @param pBuffer pointer to the byte-array data to send 00095 * @param DeviceAddr specifies the peripheral device slave address. 00096 * @param RegisterAddr specifies the internal address register 00097 * where to start writing to (must be correctly masked). 00098 * @param NumByteToWrite number of bytes to be written. 00099 * @retval 0 if ok, 00100 * @retval -1 if an I2C error has occured, or 00101 * @retval -2 on temporary buffer overflow (i.e. NumByteToWrite was too high) 00102 * @note On some devices if NumByteToWrite is greater 00103 * than one, the RegisterAddr must be masked correctly! 00104 */ 00105 int i2c_write(uint8_t* pBuffer, uint8_t DeviceAddr, uint16_t RegisterAddr, 00106 uint16_t NumByteToWrite) 00107 { 00108 int ret; 00109 uint8_t tmp[TEMP_BUF_SIZE]; 00110 00111 if(NumByteToWrite >= TEMP_BUF_SIZE) return -2; 00112 00113 /* First, send device address. Then, send data and STOP condition */ 00114 tmp[0] = (RegisterAddr >> 8) & 0xFF; 00115 tmp[1] = (RegisterAddr) & 0xFF; 00116 memcpy(tmp+2, pBuffer, NumByteToWrite); 00117 00118 ret = write(DeviceAddr, (const char*)tmp, NumByteToWrite+2, false); 00119 00120 if(ret) return -1; 00121 return 0; 00122 } 00123 /** 00124 * @brief Reads a buffer from the I2C peripheral device. 00125 * @param pBuffer pointer to the byte-array to read data in to 00126 * @param DaviceAddr specifies the peripheral device slave address. 00127 * @param RegisterAddr specifies the internal address register 00128 * where to start reading from (must be correctly masked). 00129 * @param NumByteToRead number of bytes to be read. 00130 * @retval 0 if ok, 00131 * @retval -1 if an I2C error has occured 00132 * @note On some devices if NumByteToWrite is greater 00133 * than one, the RegisterAddr must be masked correctly! 00134 */ 00135 int i2c_read(uint8_t* pBuffer, uint8_t DeviceAddr, uint16_t RegisterAddr, 00136 uint16_t NumByteToRead) 00137 { 00138 int ret; 00139 uint8_t reg_addr[2]; 00140 reg_addr[0] = (RegisterAddr >> 8) & 0xFF; 00141 reg_addr[1] = (RegisterAddr) & 0xFF; 00142 /* Send device address, with no STOP condition */ 00143 ret = write(DeviceAddr, (const char*)reg_addr, 2, true); 00144 if(!ret) { 00145 /* Read data, with STOP condition */ 00146 ret = read(DeviceAddr, (char*)pBuffer, NumByteToRead, false); 00147 } 00148 00149 if(ret) 00150 return -1; 00151 else 00152 return 0; 00153 } 00154 00155 /** 00156 * @brief Reads a buffer from the I2C peripheral device. 00157 * @param pBuffer pointer to the byte-array to read data in to 00158 * @param DaviceAddr specifies the peripheral device slave address. 00159 * @param RegisterAddr specifies the internal address register 00160 * where to start reading from (must be correctly masked). 00161 * @param NumByteToRead number of bytes to be read. 00162 * @retval 0 if ok, 00163 * @retval -1 if an I2C error has occured 00164 * @note On some devices if NumByteToWrite is greater 00165 * than one, the RegisterAddr must be masked correctly! 00166 */ 00167 int i2c_read(uint8_t* pBuffer, uint8_t DeviceAddr, uint8_t RegisterAddr, 00168 uint16_t NumByteToRead) 00169 { 00170 int ret; 00171 00172 /* Send device address, with no STOP condition */ 00173 ret = write(DeviceAddr, (const char*)&RegisterAddr, 1, true); 00174 if(!ret) { 00175 /* Read data, with STOP condition */ 00176 ret = read(DeviceAddr, (char*)pBuffer, NumByteToRead, false); 00177 } 00178 00179 if(ret) return -1; 00180 return 0; 00181 } 00182 00183 private: 00184 static const unsigned int TEMP_BUF_SIZE = 32; 00185 }; 00186 00187 #endif /* __DEV_I2C_H */
Generated on Wed Jul 13 2022 03:37:30 by
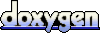