Library to handle the X-NUCLEO-6180XA1 Proximity and ambient light sensor expansion board based on VL6180X.
Dependencies: X_NUCLEO_COMMON ST_INTERFACES
Dependents: HelloWorld_6180XA1 SunTracker_BLE Servo_6180XA1 BLE_HR_Light ... more
Fork of X_NUCLEO_6180XA1 by
VL6180X Class Reference
Class representing a VL6180X sensor component. More...
#include <VL6180X.h>
Public Member Functions | |
VL6180X (DevI2C &i2c, DigitalOut &pin, PinName pin_gpio1, uint8_t DevAddr=DEFAULT_DEVICE_ADDRESS) | |
Constructor 1 (DigitalOut) | |
VL6180X (DevI2C &i2c, STMPE1600DigiOut &pin, PinName pin_gpio1, uint8_t DevAddr=DEFAULT_DEVICE_ADDRESS) | |
Constructor 2 (STMPE1600DigiOut) | |
virtual | ~VL6180X () |
Destructor. | |
void | on (void) |
PowerOn the sensor. | |
void | off (void) |
PowerOff the sensor. | |
int | start_measurement (operating_mode_t operating_mode, void(*fptr)(void), uint16_t low, uint16_t high) |
Start the measure indicated by operating mode. | |
int | get_measurement (operating_mode_t operating_mode, measure_data_t *Data) |
Get results for the measure indicated by operating mode. | |
int | stop_measurement (operating_mode_t operating_mode) |
Stop the currently running measure indicate by operating_mode. | |
int | handle_irq (operating_mode_t operating_mode, measure_data_t *Data) |
Interrupt handling func to be called by user after an INT is occourred. | |
void | enable_interrupt_measure_detection_irq (void) |
Enable interrupt measure IRQ. | |
void | disable_interrupt_measure_detection_irq (void) |
Disable interrupt measure IRQ. | |
void | attach_interrupt_measure_detection_irq (void(*fptr)(void)) |
Attach a function to call when an interrupt is detected, i.e. | |
unsigned | present () |
Check the sensor presence. | |
int | wait_device_booted () |
Wait for device booted after chip enable (hardware standby) | |
virtual int | init (void *NewAddr) |
One time device initialization. | |
int | setup_gpio_1 (uint8_t InitFunction, int ActiveHigh) |
Configure GPIO1 function and set polarity. | |
int | prepare () |
Prepare device for operation. | |
int | range_start_continuous_mode () |
Start continuous ranging mode. | |
int | range_start_single_shot () |
Start single shot ranging measure. | |
int | range_set_max_convergence_time (uint8_t MaxConTime_msec) |
Set maximum convergence time. | |
int | range_poll_measurement (VL6180X_RangeData_t *pRangeData) |
Single shot Range measurement in polling mode. | |
int | _range_get_measurement_if_ready (VL6180X_RangeData_t *pRangeData) |
Check for measure readiness and get it if ready. | |
int | range_get_measurement (VL6180X_RangeData_t *pRangeData) |
Retrieve range measurements set from device. | |
virtual int | get_distance (uint32_t *pi_data) |
Get a single distance measure result. | |
int | range_config_interrupt (uint8_t ConfigGpioInt) |
Configure ranging interrupt reported to application. | |
int | range_get_interrupt_status (uint8_t *pIntStatus) |
Return ranging error interrupt status. | |
int | als_poll_measurement (VL6180X_AlsData_t *pAlsData) |
Run a single ALS measurement in single shot polling mode. | |
int | als_get_measurement (VL6180X_AlsData_t *pAlsData) |
Get actual ALS measurement. | |
int | als_config_interrupt (uint8_t ConfigGpioInt) |
Configure ALS interrupts provide to application. | |
int | als_set_integration_period (uint16_t period_ms) |
Set ALS integration period. | |
int | als_set_inter_measurement_period (uint16_t intermeasurement_period_ms) |
Set ALS "inter-measurement period". | |
int | als_set_analogue_gain (uint8_t gain) |
Set ALS analog gain code. | |
int | als_set_thresholds (uint16_t lux_threshold_low, uint16_t lux_threshold_high) |
Set thresholds for ALS continuous mode. | |
int | als_get_interrupt_status (uint8_t *pIntStatus) |
Read ALS interrupt status. | |
int | static_init () |
Low level ranging and ALS register static settings (you should call VL6180X_Prepare() function instead) | |
int | range_wait_device_ready (int MaxLoop) |
Wait for device to be ready (before a new ranging command can be issued by application) | |
int | range_set_inter_meas_period (uint32_t InterMeasTime_msec) |
Program Inter measurement period (used only in continuous mode) | |
int | upscale_set_scaling (uint8_t scaling) |
Set device ranging scaling factor. | |
int | upscale_get_scaling () |
Get current ranging scaling factor. | |
uint16_t | get_upper_limit () |
Get the maximal distance for actual scaling. | |
int | range_set_thresholds (uint16_t low, uint16_t high, int SafeHold) |
Apply low and high ranging thresholds that are considered only in continuous mode. | |
int | range_get_thresholds (uint16_t *low, uint16_t *high) |
Get scaled high and low threshold from device. | |
int | range_set_raw_thresholds (uint8_t low, uint8_t high) |
Set ranging raw thresholds (scaling not considered so not recommended to use it) | |
int | range_set_ece_factor (uint16_t FactorM, uint16_t FactorD) |
Set Early Convergence Estimate ratio. | |
int | range_set_ece_state (int enable) |
Set Early Convergence Estimate state (See SYSRANGE_RANGE_CHECK_ENABLES register) | |
int | flter_set_state (int state) |
Set activation state of the wrap around filter. | |
int | filter_get_state () |
Get activation state of the wrap around filter. | |
int | d_max_set_state (int state) |
Set activation state of DMax computation. | |
int | d_max_get_state () |
Get activation state of DMax computation. | |
int | range_set_system_mode (uint8_t mode) |
Set ranging mode and start/stop measure (use high level functions instead : VL6180X_RangeStartSingleShot() or VL6180X_RangeStartContinuousMode()) | |
int8_t | get_offset_calibration_data () |
Get part to part calibration offset. | |
void | set_offset_calibration_data (int8_t offset) |
Set or over-write part to part calibration offset. | |
int | set_x_talk_compensation_rate (FixPoint97_t Rate) |
Set Cross talk compensation rate. | |
int | als_wait_device_ready (int MaxLoop) |
Wait for device to be ready for new als operation or max pollign loop (time out) | |
int | als_set_system_mode (uint8_t mode) |
Set ALS system mode and start/stop measure. | |
int | set_group_param_hold (int Hold) |
Set Group parameter Hold state. | |
int | set_i2c_address (int NewAddr) |
Set new device i2c address. | |
int | setup_gpio_x (int pin, uint8_t IntFunction, int ActiveHigh) |
Fully configure gpio 0/1 pin : polarity and functionality. | |
int | set_gpio_x_polarity (int pin, int active_high) |
Set interrupt pin polarity for the given GPIO. | |
int | set_gpio_x_functionality (int pin, uint8_t functionality) |
Select interrupt functionality for the given GPIO. | |
int | disable_gpio_x_out (int pin) |
brief Disable and turn to Hi-Z gpio output pin | |
int | get_interrupt_status (uint8_t *status) |
Get all interrupts cause. | |
int | clear_interrupt (uint8_t IntClear) |
Clear given system interrupt condition. | |
virtual int | get_lux (uint32_t *pi_data) |
Get a single light (in Lux) measure result. | |
int | als_start_continuous_mode () |
Start the ALS (light) measure in continous mode. | |
int | als_start_single_shot () |
Start the ALS (light) measure in single shot mode. |
Detailed Description
Class representing a VL6180X sensor component.
Definition at line 124 of file VL6180X.h.
Constructor & Destructor Documentation
VL6180X | ( | DevI2C & | i2c, |
DigitalOut & | pin, | ||
PinName | pin_gpio1, | ||
uint8_t | DevAddr = DEFAULT_DEVICE_ADDRESS |
||
) |
Constructor 1 (DigitalOut)
- Parameters:
-
[in] &i2c device I2C to be used for communication [in] &pin Mbed DigitalOut pin to be used as component GPIO_0 CE [in] &pin_gpio1 pin Mbed InterruptIn PinName to be used as component GPIO_1 INT [in] DevAddr device address, 0x29 by default
VL6180X | ( | DevI2C & | i2c, |
STMPE1600DigiOut & | pin, | ||
PinName | pin_gpio1, | ||
uint8_t | DevAddr = DEFAULT_DEVICE_ADDRESS |
||
) |
Constructor 2 (STMPE1600DigiOut)
- Parameters:
-
[in] i2c device I2C to be used for communication [in] &pin Gpio Expander STMPE1600DigiOut pin to be used as component GPIO_0 CE [in] pin_gpio1 pin Mbed InterruptIn PinName to be used as component GPIO_1 INT [in] device address, 0x29 by default
Member Function Documentation
void attach_interrupt_measure_detection_irq | ( | void(*)(void) | fptr ) |
void disable_interrupt_measure_detection_irq | ( | void | ) |
void enable_interrupt_measure_detection_irq | ( | void | ) |
Generated on Wed Jul 13 2022 23:03:14 by
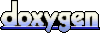