Expansion SW library to control a bipolar stepper motor using X-NUCLEO-IHM05A1 expansion board based on L6208.
Dependencies: ST_INTERFACES
Dependents: HelloWorld_IHM05A1 TAU_ROTATING_PLATFORM_IHM05A1 Amaldi_13_Exercise_IHM05A1 Amaldi_13_Exercise_IHM05A1motore ... more
Fork of X-NUCLEO-IHM05A1 by
L6208.h
00001 /** 00002 ****************************************************************************** 00003 * @file L6208.h 00004 * @author IPC Rennes 00005 * @version V1.0.0 00006 * @date March 18th, 2016 00007 * @brief This file contains the class of a L6208 Motor Control component. 00008 * @note (C) COPYRIGHT 2016 STMicroelectronics 00009 ****************************************************************************** 00010 * @attention 00011 * 00012 * <h2><center>© COPYRIGHT(c) 2016 STMicroelectronics</center></h2> 00013 * 00014 * Redistribution and use in source and binary forms, with or without modification, 00015 * are permitted provided that the following conditions are met: 00016 * 1. Redistributions of source code must retain the above copyright notice, 00017 * this list of conditions and the following disclaimer. 00018 * 2. Redistributions in binary form must reproduce the above copyright notice, 00019 * this list of conditions and the following disclaimer in the documentation 00020 * and/or other materials provided with the distribution. 00021 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00022 * may be used to endorse or promote products derived from this software 00023 * without specific prior written permission. 00024 * 00025 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00026 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00027 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00028 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00029 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00030 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00031 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00032 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00033 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00034 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00035 * 00036 ****************************************************************************** 00037 */ 00038 00039 00040 /* Define to prevent recursive inclusion -------------------------------------*/ 00041 00042 #ifndef __L6208_CLASS_H 00043 #define __L6208_CLASS_H 00044 00045 00046 /* Includes ------------------------------------------------------------------*/ 00047 00048 /* ACTION 1 ------------------------------------------------------------------* 00049 * Include here platform specific header files. * 00050 *----------------------------------------------------------------------------*/ 00051 #include "mbed.h" 00052 00053 /* ACTION 2 ------------------------------------------------------------------* 00054 * Include here component specific header files. * 00055 *----------------------------------------------------------------------------*/ 00056 #include "L6208_def.h" 00057 /* ACTION 3 ------------------------------------------------------------------* 00058 * Include here interface specific header files. * 00059 * * 00060 * Example: * 00061 * #include "HumiditySensor.h" * 00062 * #include "TemperatureSensor.h" * 00063 *----------------------------------------------------------------------------*/ 00064 #include "StepperMotor.h" 00065 00066 00067 /* Classes -------------------------------------------------------------------*/ 00068 00069 /** 00070 * @brief Class representing a L6208 component. 00071 */ 00072 class L6208 : public StepperMotor 00073 { 00074 public: 00075 /*** Constructor and Destructor Methods ***/ 00076 00077 /** 00078 * @brief Constructor. 00079 * @param flag_and_enable_pin pin name of the EN pin of the component. 00080 * @param reset_pin pin name of the RESET pin of the component. 00081 * @param direction_pin pin name of the CW_CCW pin of the component. 00082 * @param half_full_pin pin name of the HALF_FULL pin of the component. 00083 * @param control_pin pin name of the CONTROL pin of the component. 00084 * @param clock_pin pin name of the CLOCK pin of the component. 00085 * @param vrefA_pwm_pin pin name of the PWM connected to the VREFA pin of the component. 00086 * @param vrefB_pwm_pin pin name of the PWM connected to the VREFB pin of the component. 00087 */ 00088 L6208(PinName flag_and_enable_pin, PinName reset_pin, PinName direction_pin, PinName half_full_pin, PinName control_pin, PinName clock_pin, PinName vrefA_pwm_pin, PinName vrefB_pwm_pin) : StepperMotor(), 00089 flag_and_enable(flag_and_enable_pin), 00090 reset_pin(reset_pin), 00091 direction_pin(direction_pin), 00092 half_full_pin(half_full_pin), 00093 control_pin(control_pin), 00094 clock_pin(clock_pin), 00095 vrefA_pwm(vrefA_pwm_pin), 00096 vrefB_pwm(vrefB_pwm_pin) 00097 { 00098 /* Checking stackability. */ 00099 if (numberOfDevices!=0) 00100 error("Instantiation of the L6208 component failed: it can't be stacked on itself.\r\n"); 00101 00102 /* ACTION 4 ----------------------------------------------------------* 00103 * Initialize here the component's member variables, one variable per * 00104 * line. * 00105 * * 00106 * Example: * 00107 * measure = 0; * 00108 * instance_id = number_of_instances++; * 00109 *--------------------------------------------------------------------*/ 00110 errorHandlerCallback = 0; 00111 deviceInstance = numberOfDevices++; 00112 /* default tick frequency */ 00113 tickFreq = TIMER_TICK_FREQUENCY; 00114 /* waveform microstepping PWM period sample array, 90 deg shifted */ 00115 pMicroTable2 = &(microTable1[16]); 00116 } 00117 00118 /** 00119 * @brief Destructor. 00120 */ 00121 virtual ~L6208(void) {} 00122 00123 00124 /*** Public Component Related Methods ***/ 00125 00126 /* ACTION 5 --------------------------------------------------------------* 00127 * Implement here the component's public methods, as wrappers of the C * 00128 * component's functions. * 00129 * They should be: * 00130 * + Methods with the same name of the C component's virtual table's * 00131 * functions (1); * 00132 * + Methods with the same name of the C component's extended virtual * 00133 * table's functions, if any (2). * 00134 * * 00135 * Example: * 00136 * virtual int get_value(float *pData) //(1) * 00137 * { * 00138 * return COMPONENT_get_value(float *pfData); * 00139 * } * 00140 * * 00141 * virtual int enable_feature(void) //(2) * 00142 * { * 00143 * return COMPONENT_enable_feature(); * 00144 * } * 00145 *------------------------------------------------------------------------*/ 00146 00147 /** 00148 * @brief Public functions inherited from the Component Class 00149 */ 00150 00151 /** 00152 * @brief Initialize the component. 00153 * @param init Pointer to device specific initalization structure. 00154 * @retval "0" in case of success, an error code otherwise. 00155 */ 00156 virtual int init(void *init = NULL) 00157 { 00158 return (int) L6208_Init((void *) init); 00159 } 00160 00161 /** 00162 * @brief Getting the ID of the component. 00163 * @param id Pointer to an allocated variable to store the ID into. 00164 * @retval "0" in case of success, an error code otherwise. 00165 */ 00166 virtual int read_id(uint8_t *id = NULL) 00167 { 00168 return (int) L6208_ReadID((uint8_t *) id); 00169 } 00170 00171 /** 00172 * @brief Public functions inherited from the StepperMotor Class 00173 */ 00174 00175 /** 00176 * @brief Getting the value of the motor state . 00177 * @param None. 00178 * @retval The motor state accoring to motorState_t in motor.h 00179 */ 00180 virtual unsigned int get_status(void) 00181 { 00182 return (unsigned int) L6208_GetMotionState(); 00183 } 00184 00185 /** 00186 * @brief Getting the position. 00187 * @param None. 00188 * @retval The position. 00189 */ 00190 virtual signed int get_position(void) 00191 { 00192 return (signed int)L6208_GetPosition(); 00193 } 00194 00195 /** 00196 * @brief Getting the marked position. 00197 * @param None. 00198 * @retval The marked position. 00199 */ 00200 virtual signed int get_mark(void) 00201 { 00202 return (signed int)L6208_GetMark(); 00203 } 00204 00205 /** 00206 * @brief Getting the current speed in pps. 00207 * @param None. 00208 * @retval The current speed in pps. 00209 */ 00210 virtual unsigned int get_speed(void) 00211 { 00212 return (unsigned int)L6208_GetCurrentSpeed(); 00213 } 00214 00215 /** 00216 * @brief Getting the maximum speed in pps. 00217 * @param None. 00218 * @retval The maximum speed in pps. 00219 */ 00220 virtual unsigned int get_max_speed(void) 00221 { 00222 return (unsigned int)L6208_GetMaxSpeed(); 00223 } 00224 00225 /** 00226 * @brief Getting the minimum speed in pps. 00227 * @param None. 00228 * @retval The minimum speed in pps. 00229 */ 00230 virtual unsigned int get_min_speed(void) 00231 { 00232 return (unsigned int)L6208_GetMinSpeed(); 00233 } 00234 00235 /** 00236 * @brief Getting the acceleration in pps^2. 00237 * @param None. 00238 * @retval The acceleration in pps^2. 00239 */ 00240 virtual unsigned int get_acceleration(void) 00241 { 00242 return (unsigned int)L6208_GetAcceleration(); 00243 } 00244 00245 /** 00246 * @brief Getting the deceleration in pps^2. 00247 * @param None. 00248 * @retval The deceleration in pps^2. 00249 */ 00250 virtual unsigned int get_deceleration(void) 00251 { 00252 return (unsigned int)L6208_GetDeceleration(); 00253 } 00254 00255 /** 00256 * @brief Getting the direction of rotation. 00257 * @param None. 00258 * @retval The direction of rotation. 00259 */ 00260 virtual direction_t get_direction(void) 00261 { 00262 if (L6208_GetDirection()!=BACKWARD) 00263 { 00264 return FWD; 00265 } 00266 else 00267 { 00268 return BWD; 00269 } 00270 } 00271 00272 /** 00273 * @brief Setting the current position to be the home position. 00274 * @param None. 00275 * @retval None. 00276 */ 00277 virtual void set_home(void) 00278 { 00279 L6208_SetHome(); 00280 } 00281 00282 /** 00283 * @brief Setting the current position to be the marked position. 00284 * @param None. 00285 * @retval None. 00286 */ 00287 virtual void set_mark(void) 00288 { 00289 L6208_SetMark(); 00290 } 00291 00292 /** 00293 * @brief Setting the maximum speed in pps. 00294 * @param speed The maximum speed in pps. 00295 * @retval "true" in case of success, "false" otherwise. 00296 */ 00297 virtual bool set_max_speed(unsigned int speed) 00298 { 00299 if (speed <= 0xFFFF) 00300 { 00301 return L6208_SetMaxSpeed((uint16_t) speed); 00302 } 00303 else 00304 { 00305 return false; 00306 } 00307 } 00308 00309 /** 00310 * @brief Setting the minimum speed in pps. 00311 * @param speed The minimum speed in pps. 00312 * @retval "true" in case of success, "false" otherwise. 00313 */ 00314 virtual bool set_min_speed(unsigned int speed) 00315 { 00316 if (speed <= 0xFFFF) 00317 { 00318 return L6208_SetMinSpeed((uint16_t) speed); 00319 } 00320 else 00321 { 00322 return false; 00323 } 00324 } 00325 00326 /** 00327 * @brief Setting the acceleration in pps^2. 00328 * @param acceleration The acceleration in pps/s^2. 00329 * @retval "true" in case of success, "false" otherwise. 00330 */ 00331 virtual bool set_acceleration(unsigned int acceleration) 00332 { 00333 if (acceleration <= 0xFFFF) 00334 { 00335 return L6208_SetAcceleration((uint16_t) acceleration); 00336 } 00337 else 00338 { 00339 return false; 00340 } 00341 } 00342 00343 /** 00344 * @brief Setting the deceleration in pps^2. 00345 * @param deceleration The deceleration in pps^2. 00346 * @retval "true" in case of success, "false" otherwise. 00347 */ 00348 virtual bool set_deceleration(unsigned int deceleration) 00349 { 00350 if (deceleration <= 0xFFFF) 00351 { 00352 return L6208_SetDeceleration((uint16_t) deceleration); 00353 } 00354 else 00355 { 00356 return false; 00357 } 00358 } 00359 00360 /** 00361 * @brief Setting the Step Mode. 00362 * @param step_mode The Step Mode. 00363 * @retval "true" in case of success, "false" otherwise. 00364 * @note step_mode can be one of the following: 00365 * + STEP_MODE_FULL 00366 * + STEP_MODE_WAVE 00367 * + STEP_MODE_HALF 00368 * + STEP_MODE_1_4 00369 * + STEP_MODE_1_8 00370 * + STEP_MODE_1_16 00371 */ 00372 virtual bool set_step_mode(step_mode_t step_mode) 00373 { 00374 return L6208_SetStepMode((motorStepMode_t) step_mode); 00375 } 00376 00377 /** 00378 * @brief Going to a specified position. 00379 * @param position The desired position. 00380 * @retval None. 00381 */ 00382 virtual void go_to(signed int position) 00383 { 00384 L6208_GoTo((int32_t)position); 00385 } 00386 00387 /** 00388 * @brief Going to the home position. 00389 * @param None. 00390 * @retval None. 00391 */ 00392 virtual void go_home(void) 00393 { 00394 L6208_GoHome(); 00395 } 00396 00397 /** 00398 * @brief Going to the marked position. 00399 * @param None. 00400 * @retval None. 00401 */ 00402 virtual void go_mark(void) 00403 { 00404 L6208_GoMark(); 00405 } 00406 00407 /** 00408 * @brief Running the motor towards a specified direction. 00409 * @param direction The direction of rotation. 00410 * @retval None. 00411 */ 00412 virtual void run(direction_t direction) 00413 { 00414 L6208_Run((motorDir_t) (direction == StepperMotor::FWD ? FORWARD : BACKWARD)); 00415 } 00416 00417 /** 00418 * @brief Moving the motor towards a specified direction for a certain number of steps. 00419 * @param direction The direction of rotation. 00420 * @param steps The desired number of steps. 00421 * @retval None. 00422 */ 00423 virtual void move(direction_t direction, unsigned int steps) 00424 { 00425 L6208_Move((motorDir_t) (direction == StepperMotor::FWD ? FORWARD : BACKWARD), (uint32_t)steps); 00426 } 00427 00428 /** 00429 * @brief Stopping the motor through an immediate deceleration up to zero speed. 00430 * @param None. 00431 * @retval None. 00432 */ 00433 virtual void soft_stop(void) 00434 { 00435 L6208_SoftStop(); 00436 } 00437 00438 /** 00439 * @brief Stopping the motor through an immediate infinite deceleration. 00440 * @param None. 00441 * @retval None. 00442 */ 00443 virtual void hard_stop(void) 00444 { 00445 L6208_HardStop(); 00446 } 00447 00448 /** 00449 * @brief Disabling the power bridge after performing a deceleration to zero. 00450 * @param None. 00451 * @retval None. 00452 */ 00453 virtual void soft_hiz(void) 00454 { 00455 motorStopMode_t stopMode = L6208_GetStopMode(); 00456 if (stopMode==HIZ_MODE) 00457 { 00458 L6208_SoftStop(); 00459 } 00460 else 00461 { 00462 L6208_SetStopMode(HIZ_MODE); 00463 L6208_SoftStop(); 00464 L6208_SetStopMode(stopMode); 00465 } 00466 } 00467 00468 /** 00469 * @brief Disabling the power bridge immediately. 00470 * @param None. 00471 * @retval None. 00472 */ 00473 virtual void hard_hiz(void) 00474 { 00475 L6208_HardHiZ(); 00476 } 00477 00478 /** 00479 * @brief Waiting while the motor is active. 00480 * @param None. 00481 * @retval None. 00482 */ 00483 virtual void wait_while_active(void) 00484 { 00485 L6208_WaitWhileActive(); 00486 } 00487 00488 /** 00489 * @brief Public functions NOT inherited 00490 */ 00491 00492 /** 00493 * @brief Attaching an error handler. 00494 * @param fptr An error handler. 00495 * @retval None. 00496 */ 00497 virtual void attach_error_handler(void (*fptr)(uint16_t error)) 00498 { 00499 L6208_AttachErrorHandler((void (*)(uint16_t error)) fptr); 00500 } 00501 00502 /** 00503 * @brief Checks if the device is disabled or/and has an alarm flag set 00504 * by reading the EN pin position. 00505 * @param None. 00506 * @retval One if the EN pin is low (the device is disabled or/and 00507 * has an alarm flag set), otherwise zero. 00508 */ 00509 virtual unsigned int check_status_hw(void) 00510 { 00511 if (!flag_and_enable.read()) 00512 { 00513 return 0x01; 00514 } 00515 else 00516 { 00517 return 0x00; 00518 } 00519 } 00520 00521 /** 00522 * @brief Disabling the device. 00523 * @param None. 00524 * @retval None. 00525 */ 00526 virtual void disable(void) 00527 { 00528 L6208_Disable(); 00529 } 00530 00531 /** 00532 * @brief Enabling the device. 00533 * @param None. 00534 * @retval None. 00535 */ 00536 virtual void enable(void) 00537 { 00538 L6208_Enable(); 00539 } 00540 00541 /** 00542 * @brief Getting the motor decay mode. 00543 * @param None. 00544 * @retval The motor decay mode. 00545 */ 00546 virtual motorDecayMode_t get_decay_mode() 00547 { 00548 return L6208_get_decay_mode(); 00549 } 00550 00551 /** 00552 * @brief Set the frequency of the VREFA and VREFB PWM 00553 * @param frequency in Hz 00554 * @retval None. 00555 */ 00556 virtual uint32_t get_freq_vref_pwm(void) 00557 { 00558 return L6208_VrefPwmGetFreq(); 00559 } 00560 00561 /** 00562 * @brief Getting the version of the firmware. 00563 * @param None. 00564 * @retval The version of the firmware. 00565 */ 00566 virtual unsigned int get_fw_version(void) 00567 { 00568 return (unsigned int) L6208_GetFwVersion(); 00569 } 00570 00571 /** 00572 * @brief Getting the motor step mode. 00573 * @param None. 00574 * @retval The motor step mode. 00575 */ 00576 virtual step_mode_t get_step_mode(void) 00577 { 00578 return (step_mode_t) L6208_GetStepMode(); 00579 } 00580 00581 /** 00582 * @brief Getting the motor stop mode. 00583 * @param None. 00584 * @retval The motor stop mode. 00585 */ 00586 virtual motorStopMode_t get_stop_mode(void) 00587 { 00588 return L6208_GetStopMode(); 00589 } 00590 00591 /** 00592 * @brief Going to a specified position with a specificied direction. 00593 * @param direction The desired direction. 00594 * @param position The desired position. 00595 * @retval None. 00596 */ 00597 virtual void go_to(direction_t direction, signed int position) 00598 { 00599 L6208_GoToDir((motorDir_t) (direction == StepperMotor::FWD ? FORWARD : BACKWARD),(int32_t)position); 00600 } 00601 00602 /** 00603 * @brief Release the L6208 reset (Reset pin set to high level). 00604 * @param None. 00605 * @retval None. 00606 */ 00607 virtual void release_reset(void) 00608 { 00609 L6208_ReleaseReset(); 00610 } 00611 00612 /** 00613 * @brief Reset the device with current step mode, resets current speed, 00614 * positions and microstep variables. 00615 * @param None. 00616 * @retval None. 00617 */ 00618 virtual void reset(void) 00619 { 00620 L6208_Reset(); 00621 } 00622 00623 /** 00624 * @brief Reset the L6208 (Reset pin set to low level). 00625 * @param None. 00626 * @retval None. 00627 */ 00628 virtual void reset_device(void) 00629 { 00630 L6208_ResetDevice(); 00631 } 00632 00633 /** 00634 * @brief Set the motor decay mode. 00635 * @param decayMode The desired decay mode (SLOW_DECAY or FAST_DECAY). 00636 * @retval None. 00637 */ 00638 virtual void set_decay_mode(motorDecayMode_t decayMode) 00639 { 00640 L6208_SetDecayMode(decayMode); 00641 } 00642 00643 /** 00644 * @brief Set the motor direction. 00645 * @param direction The desired direction. 00646 * @retval None. 00647 */ 00648 virtual void set_direction(direction_t direction) 00649 { 00650 L6208_SetDirection((motorDir_t) (direction == StepperMotor::FWD ? FORWARD : BACKWARD)); 00651 } 00652 00653 /** 00654 * @brief Set the frequency of the VREFA and VREFB PWM 00655 * @param frequency in Hz 00656 * @retval None. 00657 */ 00658 virtual void set_freq_vref_pwm(uint32_t frequency) 00659 { 00660 L6208_VrefPwmSetFreq(frequency); 00661 } 00662 00663 /** 00664 * @brief Set the motor stop mode. 00665 * @param stopMode The desired stop mode (HOLD_MODE or HIZ_MODE). 00666 * @retval None. 00667 */ 00668 virtual void set_stop_mode(motorStopMode_t stopMode) 00669 { 00670 L6208_SetStopMode(stopMode); 00671 } 00672 00673 /*** Public Interrupt Related Methods ***/ 00674 00675 /* ACTION 6 --------------------------------------------------------------* 00676 * Implement here interrupt related methods, if any. * 00677 * Note that interrupt handling is platform dependent, e.g.: * 00678 * + mbed: * 00679 * InterruptIn feature_irq(pin); //Interrupt object. * 00680 * feature_irq.rise(callback); //Attach a callback. * 00681 * feature_irq.mode(PullNone); //Set interrupt mode. * 00682 * feature_irq.enable_irq(); //Enable interrupt. * 00683 * feature_irq.disable_irq(); //Disable interrupt. * 00684 * + Arduino: * 00685 * attachInterrupt(pin, callback, RISING); //Attach a callback. * 00686 * detachInterrupt(pin); //Detach a callback. * 00687 * * 00688 * Example (mbed): * 00689 * void attach_feature_irq(void (*fptr) (void)) * 00690 * { * 00691 * feature_irq.rise(fptr); * 00692 * } * 00693 * * 00694 * void enable_feature_irq(void) * 00695 * { * 00696 * feature_irq.enable_irq(); * 00697 * } * 00698 * * 00699 * void disable_feature_irq(void) * 00700 * { * 00701 * feature_irq.disable_irq(); * 00702 * } * 00703 *------------------------------------------------------------------------*/ 00704 /** 00705 * @brief Attaching an interrupt handler to the FLAG interrupt. 00706 * @param fptr An interrupt handler. 00707 * @retval None. 00708 */ 00709 00710 void attach_flag_irq(void (*fptr)(void)) 00711 { 00712 flag_and_enable.mode(PullDown); 00713 flag_and_enable.fall(fptr); 00714 } 00715 00716 /** 00717 * @brief Enabling the FLAG interrupt handling. 00718 * @param None. 00719 * @retval None. 00720 */ 00721 void enable_flag_irq(void) 00722 { 00723 flag_and_enable.enable_irq(); 00724 } 00725 00726 protected: 00727 00728 /*** Protected Component Related Methods ***/ 00729 00730 /* ACTION 7 --------------------------------------------------------------* 00731 * Declare here the component's specific methods. * 00732 * They should be: * 00733 * + Methods with the same name of the C component's virtual table's * 00734 * functions (1); * 00735 * + Methods with the same name of the C component's extended virtual * 00736 * table's functions, if any (2); * 00737 * + Helper methods, if any, like functions declared in the component's * 00738 * source files but not pointed by the component's virtual table (3). * 00739 * * 00740 * Example: * 00741 * status_t COMPONENT_get_value(float *f); //(1) * 00742 * status_t COMPONENT_enable_feature(void); //(2) * 00743 * status_t COMPONENT_compute_average(void); //(3) * 00744 *------------------------------------------------------------------------*/ 00745 status_t L6208_Init(void *init); 00746 status_t L6208_ReadID(uint8_t *id); 00747 void L6208_AttachErrorHandler(void (*callback)(uint16_t error)); 00748 void L6208_Disable(void); 00749 void L6208_ErrorHandler(uint16_t error); 00750 void L6208_Enable(void); 00751 uint16_t L6208_GetAcceleration(void); 00752 uint16_t L6208_GetCurrentSpeed(void); 00753 uint16_t L6208_GetDeceleration(void); 00754 motorDecayMode_t L6208_get_decay_mode(void); 00755 motorDir_t L6208_GetDirection(void); 00756 uint32_t L6208_GetFwVersion(void); 00757 int32_t L6208_GetMark(void); 00758 uint16_t L6208_GetMaxSpeed(void); 00759 uint16_t L6208_GetMinSpeed(void); 00760 motorState_t L6208_GetMotionState(void); 00761 int32_t L6208_GetPosition(void); 00762 motorStepMode_t L6208_GetStepMode(void); 00763 motorStopMode_t L6208_GetStopMode(void); 00764 void L6208_GoHome(void); 00765 void L6208_GoMark(void); 00766 void L6208_GoTo(int32_t targetPosition); 00767 void L6208_GoToDir(motorDir_t direction, int32_t targetPosition); 00768 void L6208_HardHiZ(void); 00769 void L6208_HardStop(void); 00770 void L6208_Move(motorDir_t direction, uint32_t stepCount); 00771 void L6208_ReleaseReset(void); 00772 void L6208_Reset(void); 00773 void L6208_ResetDevice(void); 00774 void L6208_Run(motorDir_t direction); 00775 bool L6208_SetAcceleration(uint16_t newAcc); 00776 void L6208_SetDecayMode(motorDecayMode_t decayMode); 00777 bool L6208_SetDeceleration(uint16_t newDec); 00778 void L6208_SetDirection(motorDir_t direction); 00779 void L6208_SetHome(void); 00780 void L6208_SetMark(void); 00781 bool L6208_SetMaxSpeed(uint16_t volatile newSpeed); 00782 bool L6208_SetMinSpeed(uint16_t volatile newSpeed); 00783 bool L6208_SetStepMode(motorStepMode_t stepMode); 00784 void L6208_SetStopMode(motorStopMode_t stopMode); 00785 bool L6208_SoftStop(void); 00786 void L6208_TickHandler(void); 00787 uint32_t L6208_VrefPwmGetFreq(void); 00788 void L6208_VrefPwmSetFreq(uint32_t newFreq); 00789 void L6208_WaitWhileActive(void); 00790 00791 /*** Functions intended to be used only internally ***/ 00792 00793 void L6208_ClearSysFlag(uint32_t mask); 00794 uint32_t L6208_ComputeNbAccOrDecSteps(uint16_t accOrDecRate); 00795 uint16_t L6208_ConvertAcceDecelRateValue(uint16_t newAccOrDecRate); 00796 void L6208_DoAccel(void); 00797 void L6208_DoDecel(void); 00798 void L6208_DoRun(void); 00799 uint8_t L6208_GetMicrostepSample2Scale(void); 00800 void L6208_Indexmodeinit(void); 00801 bool L6208_IsSysFlag(uint32_t mask); 00802 void L6208_ResetSteps(void); 00803 uint32_t L6208_ScaleWaveformSample(uint8_t sampleIndex); 00804 void L6208_ScaleWaveformTable(void); 00805 void L6208_SetDeviceParamsToGivenValues(l6208_init_t* pInitDevicePrm); 00806 void L6208_SetDeviceParamsToPredefinedValues(void); 00807 void L6208_SetMicrostepSample2Scale(uint8_t value); 00808 void L6208_SetMicrostepSample2Update(uint8_t value); 00809 void L6208_SetMotionState(motorState_t newMotionState); 00810 bool L6208_SetSpeed(uint16_t newSpeed, uint32_t volatile *pSpeed); 00811 void L6208_SetSysFlag(uint32_t mask); 00812 bool L6208_StartMovement(void); 00813 void L6208_UpdateScanWaveformTable(void); 00814 void L6208_UstepWaveformHandling(void); 00815 bool L6208_VectorCalc(uint8_t newTorque); 00816 bool L6208_VrefPwmComputePulseWidth(uint8_t bridgeId, uint16_t value, bool valueIsPwmDutyCycle); 00817 void L6208_VrefPwmUpdatePulseWidth(void); 00818 00819 /*** Component's I/O Methods ***/ 00820 00821 /* ACTION 8 --------------------------------------------------------------* 00822 * Implement here other I/O methods beyond those already implemented * 00823 * above, which are declared extern within the component's header file. * 00824 *------------------------------------------------------------------------*/ 00825 /** 00826 * @brief Reset the clock pin. 00827 * @param None. 00828 * @retval None. 00829 */ 00830 void L6208_Board_CLOCK_PIN_Reset(void) 00831 { 00832 clock_pin = 0; 00833 } 00834 /** 00835 * @brief Set the clock pin. 00836 * @param None. 00837 * @retval None. 00838 */ 00839 void L6208_Board_CLOCK_PIN_Set(void) 00840 { 00841 clock_pin = 1; 00842 } 00843 00844 /** 00845 * @brief Set the control pin. 00846 * @param None. 00847 * @retval None. 00848 */ 00849 void L6208_Board_CONTROL_PIN_Set(void) 00850 { 00851 control_pin = 1; 00852 } 00853 00854 /** 00855 * @brief Reset the control pin. 00856 * @param None. 00857 * @retval None. 00858 */ 00859 void L6208_Board_CONTROL_PIN_Reset(void) 00860 { 00861 control_pin = 0; 00862 } 00863 00864 /** 00865 * @brief Making the CPU wait. 00866 * @param None. 00867 * @retval None. 00868 */ 00869 void L6208_Board_Delay(uint32_t delay) 00870 { 00871 wait_ms(delay); 00872 } 00873 00874 /** 00875 * @brief Reset the dir pin. 00876 * @param None. 00877 * @retval None. 00878 */ 00879 void L6208_Board_DIR_PIN_Reset(void) 00880 { 00881 direction_pin = 0; 00882 } 00883 00884 /** 00885 * @brief Set the dir pin. 00886 * @param None. 00887 * @retval None. 00888 */ 00889 void L6208_Board_DIR_PIN_Set(void) 00890 { 00891 direction_pin = 1; 00892 } 00893 00894 /** 00895 * @brief Disable the power bridges (leave the output bridges HiZ). 00896 * @param None. 00897 * @retval None. 00898 */ 00899 void L6208_Board_Disable(void) 00900 { 00901 flag_and_enable.disable_irq(); 00902 flag_and_enable.mode(PullDown); 00903 } 00904 00905 /** 00906 * @brief Disabling interrupts. 00907 * @param None. 00908 * @retval None. 00909 */ 00910 void L6208_Board_DisableIrq(void) 00911 { 00912 __disable_irq(); 00913 } 00914 00915 /** 00916 * @brief Enable the power bridges (leave the output bridges HiZ). 00917 * @param None. 00918 * @retval None. 00919 */ 00920 void L6208_Board_Enable(void) 00921 { 00922 flag_and_enable.mode(PullUp); 00923 flag_and_enable.enable_irq(); 00924 } 00925 00926 /** 00927 * @brief Enabling interrupts. 00928 * @param None. 00929 * @retval None. 00930 */ 00931 void L6208_Board_EnableIrq(void) 00932 { 00933 __enable_irq(); 00934 } 00935 00936 /** 00937 * @brief Reset the half full pin. 00938 * @param None. 00939 * @retval None. 00940 */ 00941 void L6208_Board_HALF_FULL_PIN_Reset(void) 00942 { 00943 half_full_pin = 0; 00944 } 00945 00946 /** 00947 * @brief Set the half full pin. 00948 * @param None. 00949 * @retval None. 00950 */ 00951 void L6208_Board_HALF_FULL_PIN_Set(void) 00952 { 00953 half_full_pin = 1; 00954 } 00955 00956 /** 00957 * @brief Initialising the the VREFA or VREFB PWM. 00958 * @param None. 00959 * @retval None. 00960 */ 00961 void L6208_Board_VrefPwmInit(uint8_t bridgeId, uint32_t pwmFreq) {} 00962 00963 /** 00964 * @brief Exit the device from reset mode. 00965 * @param None. 00966 * @retval None. 00967 */ 00968 void L6208_Board_ReleaseReset(void) 00969 { 00970 reset_pin = 1; 00971 } 00972 00973 /** 00974 * @brief Put the device in reset mode. 00975 * @param None. 00976 * @retval None. 00977 */ 00978 void L6208_Board_Reset(void) 00979 { 00980 reset_pin = 0; 00981 } 00982 00983 /** 00984 * @brief Get the tick timer frequency in Hz. 00985 * @param None. 00986 * @retval The tick timer frequency in Hz. 00987 */ 00988 uint32_t L6208_Board_TickGetFreq(void) 00989 { 00990 return TIMER_TICK_FREQUENCY; 00991 } 00992 00993 /** 00994 * @brief Initialising the tick. 00995 * @param None. 00996 * @retval None. 00997 */ 00998 void L6208_Board_TickInit(void) {} 00999 01000 /** 01001 * @brief Starting the tick timer, setting its frequency 01002 * and attaching a tick handler function to it. 01003 * @param frequency the frequency of the tick. 01004 * @retval None. 01005 */ 01006 void L6208_Board_TickStart(uint16_t frequency) 01007 { 01008 /* Computing the period of the tick. */ 01009 double period = 1.0f / frequency; 01010 01011 /* Attaching a tick handler function which updates */ 01012 /* the state machine every elapsed period time. */ 01013 ticker.attach(this, &L6208::L6208_TickHandler, period); 01014 } 01015 01016 /** 01017 * @brief Stopping the tick. 01018 * @param None. 01019 * @retval None. 01020 */ 01021 void L6208_Board_TickStop(void) 01022 { 01023 ticker.detach(); 01024 } 01025 01026 /** 01027 * @brief Set the pulse width of the VREFA PWM. 01028 * @param[in] pulseWidthInUs pulse width of the PWM in microsecond. 01029 * @retval None. 01030 */ 01031 void L6208_Board_VrefPwmSetPulseWidthA(uint8_t pulseWidthInUs) 01032 { 01033 vrefA_pwm.pulsewidth_us(pulseWidthInUs); 01034 } 01035 01036 /** 01037 * @brief Set the pulse width of the VREFB PWM. 01038 * @param[in] pulseWidthInUs pulse width of the PWM in microsecond. 01039 * @retval None. 01040 */ 01041 void L6208_Board_VrefPwmSetPulseWidthB(uint8_t pulseWidthInUs) 01042 { 01043 vrefB_pwm.pulsewidth_us(pulseWidthInUs); 01044 } 01045 01046 /** 01047 * @brief Start the timer for the VREFA or VREFB PWM. 01048 * @param[in] bridgeId 01049 * 0 for BRIDGE_A 01050 * 1 for BRIDGE_B 01051 * @param[in] pwmPeriod period of the PWM used to generate the reference 01052 * voltage for the bridge. 01053 * @retval "true" in case of success, "false" otherwise. 01054 * @note the unit is 1/256th of a microsecond. The VREFA PWM must be started 01055 * before the VREFB PWM. 01056 */ 01057 bool L6208_Board_VrefPwmStart(uint8_t bridgeId, uint16_t pwmPeriod) 01058 { 01059 pwmPeriod>>=8; 01060 /* Setting the period and the duty-cycle of PWM. */ 01061 if (bridgeId == 0) 01062 { 01063 vrefA_pwm.period_us(pwmPeriod); 01064 } 01065 else if (bridgeId == 1) 01066 { 01067 vrefB_pwm.period_us(pwmPeriod); 01068 } 01069 else 01070 { 01071 return false; 01072 } 01073 return true; 01074 } 01075 01076 /** 01077 * @brief Stop the timer for the VREFA or VREFB PWM. 01078 * @param[in] bridgeId 01079 * 0 for BRIDGE_A 01080 * 1 for BRIDGE_B 01081 * @retval "true" in case of success, "false" otherwise. 01082 */ 01083 bool L6208_Board_VrefPwmStop(uint8_t bridgeId) 01084 { 01085 if (bridgeId == 0) 01086 { 01087 vrefA_pwm.period_us(0); 01088 } 01089 else if (bridgeId == 1) 01090 { 01091 vrefB_pwm.period_us(0); 01092 } 01093 else 01094 { 01095 return false; 01096 } 01097 return true; 01098 } 01099 01100 protected: 01101 01102 /*** Component's Instance Variables ***/ 01103 01104 /* ACTION 9 --------------------------------------------------------------* 01105 * Declare here interrupt related variables, if needed. * 01106 * Note that interrupt handling is platform dependent, see * 01107 * "Interrupt Related Methods" above. * 01108 * * 01109 * Example: * 01110 * + mbed: * 01111 * InterruptIn feature_irq; * 01112 *------------------------------------------------------------------------*/ 01113 /* Flag Interrupt and chip enable. */ 01114 InterruptIn flag_and_enable; 01115 01116 /* ACTION 10 -------------------------------------------------------------* 01117 * Declare here other pin related variables, if needed. * 01118 * * 01119 * Example: * 01120 * + mbed: * 01121 * DigitalOut standby_reset; * 01122 *------------------------------------------------------------------------*/ 01123 /* RESET pin. */ 01124 DigitalOut reset_pin; 01125 /* CW_CCW pin. */ 01126 DigitalOut direction_pin; 01127 /* HALF_FULL pin */ 01128 DigitalOut half_full_pin; 01129 /* CONTROL pin */ 01130 DigitalOut control_pin; 01131 /* CLOCK pin */ 01132 DigitalOut clock_pin; 01133 01134 /* Pulse Width Modulation pin for VREFA pin */ 01135 PwmOut vrefA_pwm; 01136 /* Pulse Width Modulation pin for VREFA pin */ 01137 PwmOut vrefB_pwm; 01138 01139 /* Timer for the tick */ 01140 Ticker ticker; 01141 01142 /* ACTION 11 -------------------------------------------------------------* 01143 * Declare here communication related variables, if needed. * 01144 * * 01145 * Example: * 01146 * + mbed: * 01147 * DigitalOut ssel; * 01148 * DevSPI &dev_spi; * 01149 *------------------------------------------------------------------------*/ 01150 /* Configuration. */ 01151 01152 /* IO Device. */ 01153 01154 /* ACTION 12 -------------------------------------------------------------* 01155 * Declare here identity related variables, if needed. * 01156 * Note that there should be only a unique identifier for each component, * 01157 * which should be the "who_am_i" parameter. * 01158 *------------------------------------------------------------------------*/ 01159 /* Identity */ 01160 uint8_t who_am_i; 01161 01162 /* ACTION 13 -------------------------------------------------------------* 01163 * Declare here the component's static and non-static data, one variable * 01164 * per line. * 01165 * * 01166 * Example: * 01167 * float measure; * 01168 * int instance_id; * 01169 * static int number_of_instances; * 01170 *------------------------------------------------------------------------*/ 01171 /* Data. */ 01172 void (*errorHandlerCallback)(uint16_t error); 01173 deviceParams_t devicePrm; 01174 uint8_t deviceInstance; 01175 uint32_t tickFreq; 01176 /// microstepping PWM period and torque scaled waveform samples array 01177 uint16_t updatedMicroTable[L6208_USTEPS_PER_QUARTER_PERIOD+1]; 01178 /// waveform scanning microstepping PWM period sample arrays for VREFA wave 01179 uint16_t microTable1[L6208_USTEPS_PER_QUARTER_PERIOD*3+1]; 01180 /// waveform scanning microstepping PWM period sample array for VREFB wave 01181 uint16_t *pMicroTable2; 01182 01183 /* Static data. */ 01184 static uint8_t numberOfDevices; 01185 static const uint16_t RefMicroTable[L6208_USTEPS_PER_QUARTER_PERIOD*3]; 01186 }; 01187 01188 #endif // __L6208_CLASS_H 01189 01190 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Thu Jul 14 2022 21:58:52 by
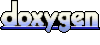