C++ Library for the PsiSwarm Robot - Version 0.8
Dependents: PsiSwarm_V8_Blank_CPP Autonomia_RndmWlk
Fork of PsiSwarmV7_CPP by
Sensors Class Reference
Sensors class Functions to read values from the Psi Swarm infrared, ultrasonic, temperature and power sensors. More...
#include <sensors.h>
Public Member Functions | |
float | get_battery_voltage (void) |
Returns the current battery voltage for the robot. | |
float | get_current (void) |
Returns the current being used by the robot. | |
float | get_dc_voltage (void) |
Returns the voltage sensed from the DC input (post rectification) | |
float | get_temperature (void) |
Returns the temperature sensed by the digital thermometer placed near the front of the MBED socket. | |
void | enable_ultrasonic_ticker (void) |
Enables a 10Hz ticker that automatically takes readings from the SRF-02 ultrasonic sensor (if fitted) | |
void | disable_ultrasonic_ticker (void) |
Disables the ultrasonic ticker. | |
void | update_ultrasonic_measure (void) |
Sends a message to SRF-02 ultrasonic sensor (if fitted) to instruct it to take a new reading. | |
void | store_background_raw_ir_values (void) |
Stores the raw ADC values for all 8 IR side-facing sensors without enabling IR emitters. | |
void | store_illuminated_raw_ir_values (void) |
Stores the raw ADC values for all 8 IR side-facing sensors after enabling IR emitters. | |
void | store_ir_values (void) |
Stores the raw ADC values for all 8 IR side-facing sensors both before and after enabling IR emitters Calls store_background_raw_ir_values() then store_illuminated_raw_ir_values() | |
char | get_background_raw_ir_value (char index) |
Returns the stored value of the non-illuminated side-facing IR sensor value based on last call of store_background_raw_ir_values Call either store_ir_values() or store_background_raw_ir_values() before using this function to update reading. | |
unsigned short | get_illuminated_raw_ir_value (char index) |
Returns the stored value of the illuminated side-facing IR sensor value based on last call of store_illuminated_raw_ir_values Call either store_ir_values() or store_illuminated_raw_ir_values() before using this function to update reading. | |
unsigned short | calculate_side_ir_value (char index) |
Returns the subtraction of the background side IR value from the reflection based on last call of store_ir_values() For most purposes this is the best method of detected obstacles etc as it mitigates for varying background levels of IR. | |
unsigned short | read_illuminated_raw_ir_value (char index) |
Enables the IR emitter then returns the value of the given side-facing IR sensor This function is used when just one sensor is needed to be read; in general using store_ir_values() and get_illuminated_raw_ir_value(index) is preferable. | |
void | store_background_base_ir_values (void) |
Stores the raw ADC values for all 5 base IR sensors without enabling IR emitters. | |
void | store_illuminated_base_ir_values (void) |
Stores the raw ADC values for all 5 base IR sensors after enabling IR emitters. | |
void | store_base_ir_values (void) |
Stores the raw ADC values for all 5 base IR sensors both before and after enabling IR emitters Calls store_background_base_ir_values() then store_illuminated_base_ir_values() | |
unsigned short | get_background_base_ir_value (char index) |
Returns the stored value of the non-illuminated base IR sensor value based on last call of store_background_base_ir_values Call either store_base_ir_values() or store_background_base_ir_values() before using this function to update reading. | |
unsigned short | get_illuminated_base_ir_value (char index) |
Returns the stored value of the illuminated base IR sensor value based on last call of store_illuminated_base_ir_values Call either store_base_ir_values() or store_illuminated_base_ir_values() before using this function to update reading. | |
unsigned short | calculate_base_ir_value (char index) |
Returns the subtraction of the background base IR value from the reflection based on last call of store_base_ir_values() For most purposes this is the best method of getting values from the base IR sensor as it mitigates for background levels of IR. |
Detailed Description
Sensors class Functions to read values from the Psi Swarm infrared, ultrasonic, temperature and power sensors.
Example:
#include "psiswarm.h" int main() { init(); }
Definition at line 39 of file sensors.h.
Member Function Documentation
unsigned short calculate_base_ir_value | ( | char | index ) |
Returns the subtraction of the background base IR value from the reflection based on last call of store_base_ir_values() For most purposes this is the best method of getting values from the base IR sensor as it mitigates for background levels of IR.
- Parameters:
-
index - The index of the sensor to read (range 0 to 4, sensor from left to right viewed from above - 2 is in middle of front)
- Returns:
- Unsigned short of compensated ir value (illuminated value - background value) (range 0 to 4095)
Definition at line 407 of file sensors.cpp.
unsigned short calculate_side_ir_value | ( | char | index ) |
Returns the subtraction of the background side IR value from the reflection based on last call of store_ir_values() For most purposes this is the best method of detected obstacles etc as it mitigates for varying background levels of IR.
- Parameters:
-
index - The index of the sensor to read (range 0 to 7, clockwise around robot from front-right)
- Returns:
- Unsigned short of compensated ir value (illuminated value - background value) (range 0 to 4095)
Definition at line 420 of file sensors.cpp.
void disable_ultrasonic_ticker | ( | void | ) |
Disables the ultrasonic ticker.
Definition at line 37 of file sensors.cpp.
void enable_ultrasonic_ticker | ( | void | ) |
Enables a 10Hz ticker that automatically takes readings from the SRF-02 ultrasonic sensor (if fitted)
Definition at line 32 of file sensors.cpp.
unsigned short get_background_base_ir_value | ( | char | index ) |
Returns the stored value of the non-illuminated base IR sensor value based on last call of store_background_base_ir_values Call either store_base_ir_values() or store_background_base_ir_values() before using this function to update reading.
- Parameters:
-
index - The index of the sensor to read (range 0 to 4, sensor from left to right viewed from above - 2 is in middle of front)
- Returns:
- Unsigned short of background IR reading (range 0 to 4095)
Definition at line 299 of file sensors.cpp.
char get_background_raw_ir_value | ( | char | index ) |
Returns the stored value of the non-illuminated side-facing IR sensor value based on last call of store_background_raw_ir_values Call either store_ir_values() or store_background_raw_ir_values() before using this function to update reading.
- Parameters:
-
index - The index of the sensor to read (range 0 to 7, clockwise around robot from front-right)
- Returns:
- Unsigned short of background IR reading (range 0 to 4095)
Definition at line 175 of file sensors.cpp.
float get_battery_voltage | ( | void | ) |
Returns the current battery voltage for the robot.
- Returns:
- The voltage (in V); this should range between 3.5V for a discharged battery and 4.2V for a fully charged battery
Definition at line 87 of file sensors.cpp.
float get_current | ( | void | ) |
Returns the current being used by the robot.
- Returns:
- The current (in A)
Definition at line 93 of file sensors.cpp.
float get_dc_voltage | ( | void | ) |
Returns the voltage sensed from the DC input (post rectification)
- Returns:
- The voltage (in V); note some back-voltage from the battery is expected even when no DC input is detected
Definition at line 101 of file sensors.cpp.
unsigned short get_illuminated_base_ir_value | ( | char | index ) |
Returns the stored value of the illuminated base IR sensor value based on last call of store_illuminated_base_ir_values Call either store_base_ir_values() or store_illuminated_base_ir_values() before using this function to update reading.
- Parameters:
-
index - The index of the sensor to read (range 0 to 4, sensor from left to right viewed from above - 2 is in middle of front)
- Returns:
- Unsigned short of illuminated IR reading (range 0 to 4095)
Definition at line 307 of file sensors.cpp.
unsigned short get_illuminated_raw_ir_value | ( | char | index ) |
Returns the stored value of the illuminated side-facing IR sensor value based on last call of store_illuminated_raw_ir_values Call either store_ir_values() or store_illuminated_raw_ir_values() before using this function to update reading.
- Parameters:
-
index - The index of the sensor to read (range 0 to 7, clockwise around robot from front-right)
- Returns:
- Unsigned short of illuminated IR reading (range 0 to 4095)
Definition at line 183 of file sensors.cpp.
float get_temperature | ( | void | ) |
Returns the temperature sensed by the digital thermometer placed near the front of the MBED socket.
- Returns:
- The temperature (in degrees C)
Definition at line 77 of file sensors.cpp.
unsigned short read_illuminated_raw_ir_value | ( | char | index ) |
Enables the IR emitter then returns the value of the given side-facing IR sensor This function is used when just one sensor is needed to be read; in general using store_ir_values() and get_illuminated_raw_ir_value(index) is preferable.
- Parameters:
-
index - The index of the sensor to read (range 0 to 7, clockwise around robot from front-right)
- Returns:
- Unsigned short of illuminated IR reading (range 0 to 4095)
Definition at line 256 of file sensors.cpp.
void store_background_base_ir_values | ( | void | ) |
Stores the raw ADC values for all 5 base IR sensors without enabling IR emitters.
Definition at line 325 of file sensors.cpp.
void store_background_raw_ir_values | ( | void | ) |
Stores the raw ADC values for all 8 IR side-facing sensors without enabling IR emitters.
Definition at line 207 of file sensors.cpp.
void store_base_ir_values | ( | void | ) |
Stores the raw ADC values for all 5 base IR sensors both before and after enabling IR emitters Calls store_background_base_ir_values() then store_illuminated_base_ir_values()
Definition at line 315 of file sensors.cpp.
void store_illuminated_base_ir_values | ( | void | ) |
Stores the raw ADC values for all 5 base IR sensors after enabling IR emitters.
Definition at line 334 of file sensors.cpp.
void store_illuminated_raw_ir_values | ( | void | ) |
Stores the raw ADC values for all 8 IR side-facing sensors after enabling IR emitters.
Definition at line 216 of file sensors.cpp.
void store_ir_values | ( | void | ) |
Stores the raw ADC values for all 8 IR side-facing sensors both before and after enabling IR emitters Calls store_background_raw_ir_values() then store_illuminated_raw_ir_values()
Definition at line 200 of file sensors.cpp.
void update_ultrasonic_measure | ( | void | ) |
Sends a message to SRF-02 ultrasonic sensor (if fitted) to instruct it to take a new reading.
The result is available approx 70ms later
Definition at line 42 of file sensors.cpp.
Generated on Tue Jul 12 2022 21:11:24 by
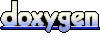