C++ Library for the PsiSwarm Robot - Version 0.8
Dependents: PsiSwarm_V8_Blank_CPP Autonomia_RndmWlk
Fork of PsiSwarmV7_CPP by
sensors.h
00001 /* University of York Robotics Laboratory PsiSwarm Library: Sensor Functions Header File 00002 * 00003 * Copyright 2016 University of York 00004 * 00005 * Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at http://www.apache.org/licenses/LICENSE-2.0 00007 * Unless required by applicable law or agreed to in writing, software distributed under the License is distributed on an "AS IS" BASIS 00008 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00009 * See the License for the specific language governing permissions and limitations under the License. 00010 * 00011 * File: sensors.h 00012 * 00013 * (C) Dept. Electronics & Computer Science, University of York 00014 * James Hilder, Alan Millard, Alexander Horsfield, Homero Elizondo, Jon Timmis 00015 * 00016 * PsiSwarm Library Version: 0.8 00017 * 00018 * October 2016 00019 * 00020 */ 00021 00022 #ifndef SENSORS_H 00023 #define SENSORS_H 00024 00025 /** 00026 * Sensors class 00027 * Functions to read values from the Psi Swarm infrared, ultrasonic, temperature and power sensors 00028 * 00029 * Example: 00030 * @code 00031 * #include "psiswarm.h" 00032 * 00033 * int main() { 00034 * init(); 00035 * 00036 * } 00037 * @endcode 00038 */ 00039 class Sensors 00040 { 00041 public: 00042 00043 /** Returns the current battery voltage for the robot 00044 * @return The voltage (in V); this should range between 3.5V for a discharged battery and 4.2V for a fully charged battery 00045 */ 00046 float get_battery_voltage ( void ); 00047 00048 /** Returns the current being used by the robot 00049 * @return The current (in A) 00050 */ 00051 float get_current ( void ); 00052 00053 /** Returns the voltage sensed from the DC input (post rectification) 00054 * @return The voltage (in V); note some back-voltage from the battery is expected even when no DC input is detected 00055 */ 00056 float get_dc_voltage ( void ); 00057 00058 /** Returns the temperature sensed by the digital thermometer placed near the front of the MBED socket 00059 * @return The temperature (in degrees C) 00060 */ 00061 float get_temperature ( void ); 00062 00063 /** Enables a 10Hz ticker that automatically takes readings from the SRF-02 ultrasonic sensor (if fitted) 00064 */ 00065 void enable_ultrasonic_ticker( void ); 00066 00067 /** Disables the ultrasonic ticker 00068 */ 00069 void disable_ultrasonic_ticker( void ); 00070 00071 /** Sends a message to SRF-02 ultrasonic sensor (if fitted) to instruct it to take a new reading. The result is available approx 70ms later 00072 */ 00073 void update_ultrasonic_measure ( void ); 00074 void IF_read_ultrasonic_measure ( void ); 00075 00076 /** Stores the raw ADC values for all 8 IR side-facing sensors without enabling IR emitters 00077 */ 00078 void store_background_raw_ir_values ( void ); 00079 00080 /** Stores the raw ADC values for all 8 IR side-facing sensors after enabling IR emitters 00081 */ 00082 void store_illuminated_raw_ir_values ( void ); 00083 00084 /** Stores the raw ADC values for all 8 IR side-facing sensors both before and after enabling IR emitters 00085 * Calls store_background_raw_ir_values() then store_illuminated_raw_ir_values() 00086 */ 00087 void store_ir_values ( void ); 00088 00089 /** Returns the stored value of the non-illuminated side-facing IR sensor value based on last call of store_background_raw_ir_values 00090 * Call either store_ir_values() or store_background_raw_ir_values() before using this function to update reading 00091 * @param index - The index of the sensor to read (range 0 to 7, clockwise around robot from front-right) 00092 * @return Unsigned short of background IR reading (range 0 to 4095) 00093 */ 00094 char get_background_raw_ir_value ( char index ); 00095 00096 /** Returns the stored value of the illuminated side-facing IR sensor value based on last call of store_illuminated_raw_ir_values 00097 * Call either store_ir_values() or store_illuminated_raw_ir_values() before using this function to update reading 00098 * @param index - The index of the sensor to read (range 0 to 7, clockwise around robot from front-right) 00099 * @return Unsigned short of illuminated IR reading (range 0 to 4095) 00100 */ 00101 unsigned short get_illuminated_raw_ir_value ( char index ); 00102 00103 /** Returns the subtraction of the background side IR value from the reflection based on last call of store_ir_values() 00104 * For most purposes this is the best method of detected obstacles etc as it mitigates for varying background levels of IR 00105 * @param index - The index of the sensor to read (range 0 to 7, clockwise around robot from front-right) 00106 * @return Unsigned short of compensated ir value (illuminated value - background value) (range 0 to 4095) 00107 */ 00108 unsigned short calculate_side_ir_value ( char index ); 00109 00110 /** Enables the IR emitter then returns the value of the given side-facing IR sensor 00111 * This function is used when just one sensor is needed to be read; in general using store_ir_values() and get_illuminated_raw_ir_value(index) is preferable 00112 * @param index - The index of the sensor to read (range 0 to 7, clockwise around robot from front-right) 00113 * @return Unsigned short of illuminated IR reading (range 0 to 4095) 00114 */ 00115 unsigned short read_illuminated_raw_ir_value ( char index ) ; 00116 00117 /** Stores the raw ADC values for all 5 base IR sensors without enabling IR emitters 00118 */ 00119 void store_background_base_ir_values ( void ); 00120 00121 /** Stores the raw ADC values for all 5 base IR sensors after enabling IR emitters 00122 */ 00123 void store_illuminated_base_ir_values ( void ); 00124 00125 /** Stores the raw ADC values for all 5 base IR sensors both before and after enabling IR emitters 00126 * Calls store_background_base_ir_values() then store_illuminated_base_ir_values() 00127 */ 00128 void store_base_ir_values ( void ); 00129 00130 /** Returns the stored value of the non-illuminated base IR sensor value based on last call of store_background_base_ir_values 00131 * Call either store_base_ir_values() or store_background_base_ir_values() before using this function to update reading 00132 * @param index - The index of the sensor to read (range 0 to 4, sensor from left to right viewed from above - 2 is in middle of front) 00133 * @return Unsigned short of background IR reading (range 0 to 4095) 00134 */ 00135 unsigned short get_background_base_ir_value ( char index ); 00136 00137 /** Returns the stored value of the illuminated base IR sensor value based on last call of store_illuminated_base_ir_values 00138 * Call either store_base_ir_values() or store_illuminated_base_ir_values() before using this function to update reading 00139 * @param index - The index of the sensor to read (range 0 to 4, sensor from left to right viewed from above - 2 is in middle of front) 00140 * @return Unsigned short of illuminated IR reading (range 0 to 4095) 00141 */ 00142 unsigned short get_illuminated_base_ir_value ( char index ); 00143 00144 00145 /** Returns the subtraction of the background base IR value from the reflection based on last call of store_base_ir_values() 00146 * For most purposes this is the best method of getting values from the base IR sensor as it mitigates for background levels of IR 00147 * @param index - The index of the sensor to read (range 0 to 4, sensor from left to right viewed from above - 2 is in middle of front) 00148 * @return Unsigned short of compensated ir value (illuminated value - background value) (range 0 to 4095) 00149 */ 00150 unsigned short calculate_base_ir_value ( char index ); 00151 00152 // The following functions are used for special programs or to retain backwards compatability but are not documented as part of the API 00153 00154 void store_reflected_ir_distances ( void ); 00155 float read_reflected_ir_distance ( char index ); 00156 float get_reflected_ir_distance ( char index ); 00157 float calculate_reflected_distance ( unsigned short background_value, unsigned short illuminated_value ); 00158 int get_bearing_from_ir_array ( unsigned short * ir_sensor_readings); 00159 void store_line_position ( void ); 00160 void calibrate_base_ir_sensors ( void ); 00161 00162 }; 00163 #endif
Generated on Tue Jul 12 2022 21:11:24 by
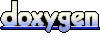