C++ Library for the PsiSwarm Robot - Version 0.8
Dependents: PsiSwarm_V8_Blank_CPP Autonomia_RndmWlk
Fork of PsiSwarmV7_CPP by
Eprom Class Reference
Eprom Class Functions for accessing the 64Kb EPROM chip and reading the reserved firmware block. More...
#include <eprom.h>
Public Member Functions | |
void | write_eeprom_byte (int address, char data) |
Write a single byte to the EPROM. | |
char | read_eeprom_byte (int address) |
Read a single byte from the EPROM. | |
char | read_next_eeprom_byte (void) |
Read the next byte from the EPROM, to be called after read_eeprom_byte. | |
char | read_firmware (void) |
Read the data stored in the reserved firmware area of the EPROM. |
Detailed Description
Eprom Class Functions for accessing the 64Kb EPROM chip and reading the reserved firmware block.
Example:
#include "psiswarm.h" int main() { init(); eprom.write_eeprom_byte(0,0xDD); //Writes byte 0xDD in EPROM address 0 char c = eprom.read_eeprom_byte(0); //c will hold 0xDD //Valid address range is from 0 to 65279 }
Definition at line 41 of file eprom.h.
Member Function Documentation
char read_eeprom_byte | ( | int | address ) |
char read_firmware | ( | void | ) |
char read_next_eeprom_byte | ( | void | ) |
void write_eeprom_byte | ( | int | address, |
char | data | ||
) |
Write a single byte to the EPROM.
University of York Robotics Laboratory PsiSwarm Library: Eprom Functions Source File.
- Parameters:
-
address The address to store the data, range 0-65279 data The character to store
Copyright 2016 University of York
Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance with the License. You may obtain a copy of the License at http://www.apache.org/licenses/LICENSE-2.0 Unless required by applicable law or agreed to in writing, software distributed under the License is distributed on an "AS IS" BASIS WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions and limitations under the License.
File: eprom.cpp
(C) Dept. Electronics & Computer Science, University of York James Hilder, Alan Millard, Alexander Horsfield, Homero Elizondo, Jon Timmis
PsiSwarm Library Version: 0.8
October 2016
Functions for accessing the 64Kb EPROM chip and reading the reserved firmware block
Example:
#include "psiswarm.h" int main() { init(); eprom.write_eeprom_byte(0,0xDD); //Writes byte 0xDD in EPROM address 0 char c = eprom.read_eeprom_byte(0); //c will hold 0xDD //Valid address range is from 0 to 65279 }
Write a single byte to the EPROM
- Parameters:
-
address The address to store the data, range 0-65279 data The character to store
Generated on Tue Jul 12 2022 21:11:24 by
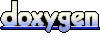