C++ Library for the PsiSwarm Robot - Version 0.8
Dependents: PsiSwarm_V8_Blank_CPP Autonomia_RndmWlk
Fork of PsiSwarmV7_CPP by
eprom.h
00001 /* University of York Robotics Laboratory PsiSwarm Library: Eprom Functions Header File 00002 * 00003 * Copyright 2016 University of York 00004 * 00005 * Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at http://www.apache.org/licenses/LICENSE-2.0 00007 * Unless required by applicable law or agreed to in writing, software distributed under the License is distributed on an "AS IS" BASIS 00008 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00009 * See the License for the specific language governing permissions and limitations under the License. 00010 * 00011 * File: eprom.h 00012 * 00013 * (C) Dept. Electronics & Computer Science, University of York 00014 * James Hilder, Alan Millard, Alexander Horsfield, Homero Elizondo, Jon Timmis 00015 * 00016 * PsiSwarm Library Version: 0.8 00017 * 00018 * October 2016 00019 * 00020 */ 00021 00022 00023 #ifndef EPROM_H 00024 #define EPROM_H 00025 00026 /** Eprom Class 00027 * Functions for accessing the 64Kb EPROM chip and reading the reserved firmware block 00028 * 00029 * Example: 00030 * @code 00031 * #include "psiswarm.h" 00032 * 00033 * int main() { 00034 * init(); 00035 * eprom.write_eeprom_byte(0,0xDD); //Writes byte 0xDD in EPROM address 0 00036 * char c = eprom.read_eeprom_byte(0); //c will hold 0xDD 00037 * //Valid address range is from 0 to 65279 00038 * } 00039 * @endcode 00040 */ 00041 class Eprom 00042 { 00043 00044 public: 00045 00046 /** Write a single byte to the EPROM 00047 * 00048 * @param address The address to store the data, range 0-65279 00049 * @param data The character to store 00050 */ 00051 void write_eeprom_byte ( int address, char data ); 00052 00053 /** Read a single byte from the EPROM 00054 * 00055 * @param address The address to read from, range 0-65279 00056 * @return The character stored at address 00057 */ 00058 char read_eeprom_byte ( int address ); 00059 00060 /** Read the next byte from the EPROM, to be called after read_eeprom_byte 00061 * 00062 * @return The character stored at address after the previous one read from 00063 */ 00064 char read_next_eeprom_byte ( void ); 00065 00066 /** Read the data stored in the reserved firmware area of the EPROM 00067 * 00068 * @return 1 if a valid firmware is read, 0 otherwise 00069 */ 00070 char read_firmware ( void ); 00071 00072 }; 00073 00074 #endif
Generated on Tue Jul 12 2022 21:11:24 by
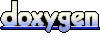